给定由 M对组成的两个数组 A[]和B[] ,根据层序遍历表示 N 个不同节点的两棵二叉树的边,任务是检查树是否是彼此的镜像。
例子:
Input: N = 6, M = 5, A[][2] = {{1, 5}, {1, 4}, {5, 7}, {5, 8}, {4, 9}}, B[][2] = {{1, 4}, {1, 5}, {4, 9}, {5, 8}, {5, 7}}
Output: Yes
Explanation:
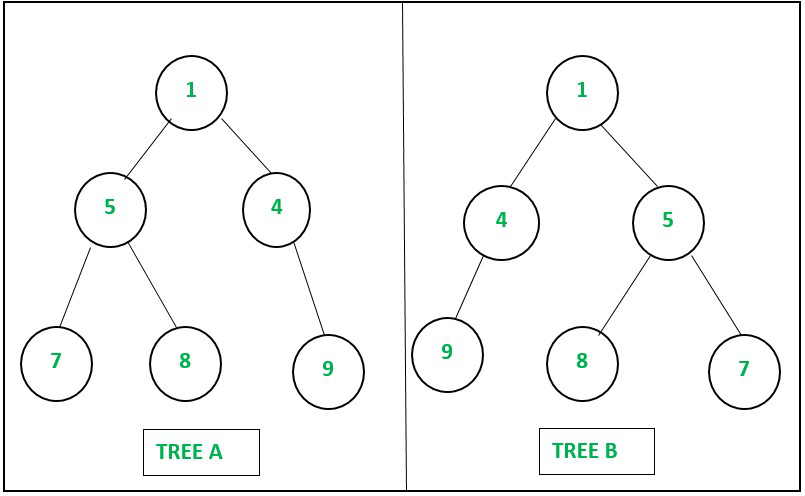
Example 1
Input: N = 5, M = 4, A[][2] = {{10, 20}, {10, 30}, {20, 40}, {20, 50}}, B[][2] = {{10, 30}, {10, 20}, {20, 40}, {20, 50}}
Output: No
Explanation:
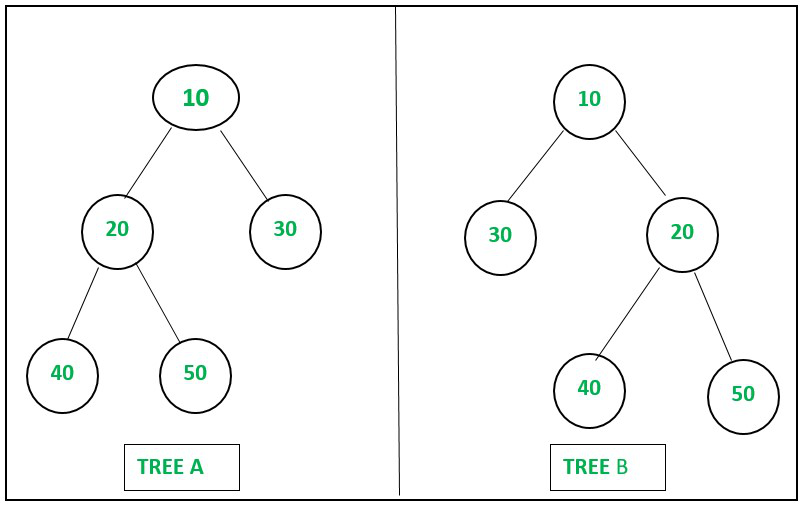
Example 2
本文的 Set 1 和 Set 2 在之前的文章中已经讨论过。
方法:可以使用 Map 和 Set 数据结构解决给定的问题。请按照以下步骤解决问题:
- 初始化两个,向量的映射说T1和T2分别存储树A和B的邻接表。
- 初始化一个集合称St来存储所有唯一节点的值。
- 使用变量i遍历数组A[] ,并执行以下步骤:
- 将值A[i][1]推入向量T1[A[i][0]] 中,然后将A[i][0]和A[i][1]附加到集合St 。
- 由于边是根据层序遍历给出的,因此在所有节点的树 A中,首先插入左子节点,然后插入右子节点。
- 使用变量 i以相反的顺序遍历数组B[]并执行以下步骤:
- 将值B[i][1]推入向量T2[B[i][0]] 中,然后将B[i][0]和B[i][1]附加到集合St 。
- 由于数组B[]是反向遍历的,因此在所有节点的树 B中,首先插入右孩子,然后插入左孩子。
- 现在迭代集合St并检查树 A中当前节点的孩子的向量是否不等于树 B 中当前节点的孩子的向量,然后打印“否”作为答案,然后返回。
- 最后,如果以上情况都不满足,则打印“是”作为答案。
下面是上述方法的实现:
C++
// C++ program for the above approach
#include
using namespace std;
// Function to check whether two binary
// trees are mirror image of each other
// or not
string checkMirrorTree(int N, int M,
int A[][2], int B[][2])
{
// Stores the adjacency list
// of tree A
map > T1;
// Stores the adjacency list
// of tree B
map > T2;
// Stores all distinct nodes
set st;
// Traverse the array A[]
for (int i = 0; i < M; i++) {
// Push A[i][1] in the
// vector T1[A[i][0]]
T1[A[i][0]].push_back(A[i][1]);
// Insert A[i][0] in the
// set st
st.insert(A[i][0]);
// Insert A[i][1] in the
// set st
st.insert(A[i][1]);
}
// Traverse the array B[] in
// reverse
for (int i = M - 1; i >= 0; i--) {
// Push B[i][1] in the
// vector T2[B[i][0]]
T2[B[i][0]].push_back(B[i][1]);
// Insert B[i][0] in the
// set st
st.insert(B[i][0]);
// Insert B[i][0] in the
// set st
st.insert(B[i][1]);
}
// Iterate over the set st
for (auto node : st) {
// If vector T1[node] is
// not equals to T2[node]
if (T1[node] != T2[node])
return "No";
}
// Return "Yes" as
// the answer
return "Yes";
}
// Driver Code
int main()
{
// Given Input
int N = 6;
int M = 5;
int A[][2] = {
{ 1, 5 }, { 1, 4 }, { 5, 7 }, { 5, 8 }, { 4, 9 }
};
int B[][2] = {
{ 1, 4 }, { 1, 5 }, { 4, 9 }, { 5, 8 }, { 5, 7 }
};
// Function Call
cout << checkMirrorTree(N, M, A, B);
return 0;
}
Python3
# Py program for the above approach
# Function to check whether two binary
# trees are mirror image of each other
# or not
def checkMirrorTree(N, M, A, B):
# Stores the adjacency list
# of tree A
T1 = [[] for i in range(100)]
# Stores the adjacency list
# of tree B
T2 = [[] for i in range(100)]
# Stores all distinct nodes
st = {}
# Traverse the array A[]
for i in range(M):
# Push A[i][1] in the
# vector T1[A[i][0]]
T1[A[i][0]].append(A[i][1])
# Insert A[i][0] in the
# set st
st[A[i][0]] = 1
# Insert A[i][1] in the
# set st
st[A[i][1]] = 1
# Traverse the array B[] in
# reverse
for i in range(M - 1, -1, -1):
# Push B[i][1] in the
# vector T2[B[i][0]]
T2[B[i][0]].append(B[i][1])
# Insert B[i][0] in the
# set st
st[B[i][0]] = 1
# Insert B[i][0] in the
# set st
st[B[i][1]] = 1
# Iterate over the set st
for node in st:
# If vector T1[node] is
# not equals to T2[node]
if (T1[node] != T2[node]):
return "No"
# Return "Yes" as
# the answer
return "Yes"
# Driver Code
if __name__ == '__main__':
# Given Input
N = 6
M = 5
A =[ [1, 5], [1, 4], [5, 7], [5, 8], [4, 9]]
B =[ [ 1, 4 ],[ 1, 5 ],[ 4, 9 ],[ 5, 8 ],[ 5, 7 ]]
# Function Call
print (checkMirrorTree(N, M, A, B))
# This code is contributed by mohit kumar 29.
输出
Yes
时间复杂度: O((N+M)*log(N))
辅助空间: O(N+M)
如果您希望与专家一起参加现场课程,请参阅DSA 现场工作专业课程和学生竞争性编程现场课程。