将矩阵顺时针旋转 90 度而不使用任何额外空间
给定一个方阵,在不使用任何额外空间的情况下,将其顺时针方向旋转 90 度。
例子:
Input:
1 2 3
4 5 6
7 8 9
Output:
7 4 1
8 5 2
9 6 3
Input:
1 2
3 4
Output:
3 1
4 2
方法一
方法:方法类似于 Inplace 旋转方阵 90 度 |设置 1。唯一不同的是以顺时针方向打印循环的元素,即 N x N 矩阵将具有 floor(N/2) 平方循环。
例如,一个 3 X 3 矩阵将有 1 个循环。循环由其第一行、最后一列、最后一行和第一列构成。
对于每个方形循环,我们以顺时针方向交换矩阵中与相应单元格相关的元素。为此,我们只需要一个临时变量。
解释:
Let size of row and column be 3.
During first iteration –
a[i][j] = Element at first index (leftmost corner top)= 1.
a[j][n-1-i]= Rightmost corner top Element = 3.
a[n-1-i][n-1-j] = Rightmost corner bottom element = 9.
a[n-1-j][i] = Leftmost corner bottom element = 7.
Move these elements in the clockwise direction.
During second iteration –
a[i][j] = 2.
a[j][n-1-j] = 6.
a[n-1-i][n-1-j] = 8.
a[n-1-j][i] = 4.
Similarly, move these elements in the clockwise direction.
下面是上述方法的实现:
C++
// C++ implementation of above approach
#include
using namespace std;
#define N 4
// Function to rotate the matrix 90 degree clockwise
void rotate90Clockwise(int a[N][N])
{
// Traverse each cycle
for (int i = 0; i < N / 2; i++) {
for (int j = i; j < N - i - 1; j++) {
// Swap elements of each cycle
// in clockwise direction
int temp = a[i][j];
a[i][j] = a[N - 1 - j][i];
a[N - 1 - j][i] = a[N - 1 - i][N - 1 - j];
a[N - 1 - i][N - 1 - j] = a[j][N - 1 - i];
a[j][N - 1 - i] = temp;
}
}
}
// Function for print matrix
void printMatrix(int arr[N][N])
{
for (int i = 0; i < N; i++) {
for (int j = 0; j < N; j++)
cout << arr[i][j] << " ";
cout << '\n';
}
}
// Driver code
int main()
{
int arr[N][N] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90Clockwise(arr);
printMatrix(arr);
return 0;
}
Java
// Java implementation of above approach
import java.io.*;
class GFG
{
static int N = 4;
// Function to rotate the matrix 90 degree clockwise
static void rotate90Clockwise(int a[][])
{
// Traverse each cycle
for (int i = 0; i < N / 2; i++)
{
for (int j = i; j < N - i - 1; j++)
{
// Swap elements of each cycle
// in clockwise direction
int temp = a[i][j];
a[i][j] = a[N - 1 - j][i];
a[N - 1 - j][i] = a[N - 1 - i][N - 1 - j];
a[N - 1 - i][N - 1 - j] = a[j][N - 1 - i];
a[j][N - 1 - i] = temp;
}
}
}
// Function for print matrix
static void printMatrix(int arr[][])
{
for (int i = 0; i < N; i++)
{
for (int j = 0; j < N; j++)
System.out.print( arr[i][j] + " ");
System.out.println();
}
}
// Driver code
public static void main (String[] args)
{
int arr[][] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90Clockwise(arr);
printMatrix(arr);
}
}
// This code has been contributed by inder_verma.
Python
# Function to rotate the matrix
# 90 degree clockwise
def rotate90Clockwise(A):
N = len(A[0])
for i in range(N // 2):
for j in range(i, N - i - 1):
temp = A[i][j]
A[i][j] = A[N - 1 - j][i]
A[N - 1 - j][i] = A[N - 1 - i][N - 1 - j]
A[N - 1 - i][N - 1 - j] = A[j][N - 1 - i]
A[j][N - 1 - i] = temp
# Function to print the matrix
def printMatrix(A):
N = len(A[0])
for i in range(N):
print(A[i])
# Driver code
A = [[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12],
[13, 14, 15, 16]]
rotate90Clockwise(A)
printMatrix(A)
# This code was contributed
# by pk_tautolo
C#
// C# implementation of above approach
using System;
class GFG
{
static int N = 4;
// Function to rotate the matrix
// 90 degree clockwise
static void rotate90Clockwise(int[,] a)
{
// Traverse each cycle
for (int i = 0; i < N / 2; i++)
{
for (int j = i; j < N - i - 1; j++)
{
// Swap elements of each cycle
// in clockwise direction
int temp = a[i, j];
a[i, j] = a[N - 1 - j, i];
a[N - 1 - j, i] = a[N - 1 - i, N - 1 - j];
a[N - 1 - i, N - 1 - j] = a[j, N - 1 - i];
a[j, N - 1 - i] = temp;
}
}
}
// Function for print matrix
static void printMatrix(int[,] arr)
{
for (int i = 0; i < N; i++)
{
for (int j = 0; j < N; j++)
Console.Write( arr[i, j] + " ");
Console.Write("\n");
}
}
// Driver code
public static void Main ()
{
int [,]arr = {{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12},
{13, 14, 15, 16}};
rotate90Clockwise(arr);
printMatrix(arr);
}
}
// This code is contributed
// by ChitraNayal
PHP
Javascript
C++
// C++ implementation of above approach
#include
using namespace std;
#define N 4
// Function to rotate the matrix 90 degree clockwise
void rotate90Clockwise(int arr[N][N])
{
// printing the matrix on the basis of
// observations made on indices.
for (int j = 0; j < N; j++)
{
for (int i = N - 1; i >= 0; i--)
cout << arr[i][j] << " ";
cout << '\n';
}
}
// Driver code
int main()
{
int arr[N][N] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90Clockwise(arr);
return 0;
}
// This code is contributed by yashbeersingh42
Java
// Java implementation of above approach
import java.io.*;
class GFG {
static int N = 4;
// Function to rotate the matrix 90 degree clockwise
static void rotate90Clockwise(int arr[][])
{
// printing the matrix on the basis of
// observations made on indices.
for (int j = 0; j < N; j++)
{
for (int i = N - 1; i >= 0; i--)
System.out.print(arr[i][j] + " ");
System.out.println();
}
}
public static void main(String[] args)
{
int arr[][] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90Clockwise(arr);
}
}
// This code is contributed by yashbeersingh42
Python3
# Python3 implementation of above approach
N = 4
# Function to rotate the matrix 90 degree clockwise
def rotate90Clockwise(arr) :
global N
# printing the matrix on the basis of
# observations made on indices.
for j in range(N) :
for i in range(N - 1, -1, -1) :
print(arr[i][j], end = " ")
print()
# Driver code
arr = [ [ 1, 2, 3, 4 ],
[ 5, 6, 7, 8 ],
[ 9, 10, 11, 12 ],
[ 13, 14, 15, 16 ] ]
rotate90Clockwise(arr);
# This code is contributed by divyesh072019.
C#
// C# implementation of above approach
using System;
class GFG {
static int N = 4;
// Function to rotate the matrix 90 degree clockwise
static void rotate90Clockwise(int[,] arr)
{
// printing the matrix on the basis of
// observations made on indices.
for (int j = 0; j < N; j++)
{
for (int i = N - 1; i >= 0; i--)
Console.Write(arr[i, j] + " ");
Console.WriteLine();
}
}
// Driver code
static void Main() {
int[,] arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90Clockwise(arr);
}
}
// This code is contributed by divyeshrabadiya07.
Javascript
C++
#include
using namespace std;
#define N 4
void print(int arr[N][N])
{
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < N; ++j)
cout << arr[i][j] << " ";
cout << '\n';
}
}
void rotate(int arr[N][N])
{
// First rotation
// with respect to main diagonal
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < i; ++j)
{
int temp = arr[i][j];
arr[i][j] = arr[j][i];
arr[j][i] = temp;
}
}
// Second rotation
// with respect to middle column
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < N / 2; ++j)
{
int temp = arr[i][j];
arr[i][j] = arr[i][N - j - 1];
arr[i][N - j - 1] = temp;
}
}
}
// Driver code
int main()
{
int arr[N][N] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate(arr);
print(arr);
return 0;
}
// This code is contributed by Rahul Verma
Java
import java.io.*;
class GFG {
static void rotate(int[][] arr) {
int n=arr.length;
// first rotation
// with respect to main diagonal
for(int i=0;i
C#
using System;
using System.Collections.Generic;
public class GFG {
static void rotate(int[,] arr) {
int n=arr.GetLength(0);
// first rotation
// with respect to main diagonal
for(int i=0;i
Javascript
C++
#include
using namespace std;
#define N 4
void print(int arr[N][N])
{
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < N; ++j)
cout << arr[i][j] << " ";
cout << '\n';
}
}
void rotate(int arr[N][N])
{
// First rotation
// with respect to Secondary diagonal
for(int i = 0; i < N; i++)
{
for(int j = 0; j < N - i; j++)
{
int temp = arr[i][j];
arr[i][j] = arr[N - 1 - j][N - 1 - i];
arr[N - 1 - j][N - 1 - i] = temp;
}
}
// Second rotation
// with respect to middle row
for(int i = 0; i < N / 2; i++)
{
for(int j = 0; j < N; j++)
{
int temp = arr[i][j];
arr[i][j] = arr[N - 1 - i][j];
arr[N - 1 - i][j] = temp;
}
}
}
// Driver code
int main()
{
int arr[N][N] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate(arr);
print(arr);
return 0;
}
// This code is contributed by Rahul Verma
Java
import java.io.*;
class GFG {
static void rotate(int[][] arr)
{
int n = arr.length;
// first rotation
// with respect to Secondary diagonal
for (int i = 0; i < n; i++) {
for (int j = 0; j < n - i; j++) {
int temp = arr[i][j];
arr[i][j] = arr[n - 1 - j][n - 1 - i];
arr[n - 1 - j][n - 1 - i] = temp;
}
}
// Second rotation
// with respect to middle row
for (int i = 0; i < n / 2; i++) {
for (int j = 0; j < n; j++) {
int temp = arr[i][j];
arr[i][j] = arr[n - 1 - i][j];
arr[n - 1 - i][j] = temp;
}
}
}
// to print matrix
static void printMatrix(int arr[][])
{
int n = arr.length;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++)
System.out.print(arr[i][j] + " ");
System.out.println();
}
}
// Driver code
public static void main(String[] args)
{
int arr[][] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate(arr);
printMatrix(arr);
}
}
// This code is contributed by Rahul Verma
C#
using System;
using System.Collections.Generic;
class GFG{
static void rotate(int[,] arr)
{
int n = arr.GetLength(0);
// First rotation
// with respect to Secondary diagonal
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n - i; j++)
{
int temp = arr[i, j];
arr[i, j] = arr[n - 1 - j, n - 1 - i];
arr[n - 1 - j, n - 1 - i] = temp;
}
}
// Second rotation
// with respect to middle row
for(int i = 0; i < n / 2; i++)
{
for(int j = 0; j < n; j++)
{
int temp = arr[i, j];
arr[i, j] = arr[n - 1 - i, j];
arr[n - 1 - i, j] = temp;
}
}
}
// To print matrix
static void printMatrix(int [,]arr)
{
int n = arr.GetLength(0);
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n; j++)
Console.Write(arr[i, j] + " ");
Console.WriteLine();
}
}
// Driver code
public static void Main(String[] args)
{
int [,]arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate(arr);
printMatrix(arr);
}
}
// This code is contributed by aashish1995
13 9 5 1
14 10 6 2
15 11 7 3
16 12 8 4
方法二:
方法:该方法基于旋转矩阵后由索引构成的模式。考虑下图可以清楚地了解它。
考虑具有如下索引 (i, j) 的 3 x 3 矩阵。
00 01 02
10 11 12
20 21 22
将矩阵顺时针旋转 90 度后,索引转换为
20 10 00 current_row_index = 0, i = 2, 1, 0
21 11 01 current_row_index = 1, i = 2, 1, 0
22 12 02 current_row_index = 2, i = 2, 1, 0
观察:在任何行中,对于每一个递减的行索引i ,都存在一个常量列索引j , 使得j = current_row_index 。
可以使用 2 个嵌套循环打印此图案。
(这种写入索引的模式是通过写入所需元素的确切索引来实现的
它们实际存在于原始数组中的位置。)
下面是上述方法的实现:
C++
// C++ implementation of above approach
#include
using namespace std;
#define N 4
// Function to rotate the matrix 90 degree clockwise
void rotate90Clockwise(int arr[N][N])
{
// printing the matrix on the basis of
// observations made on indices.
for (int j = 0; j < N; j++)
{
for (int i = N - 1; i >= 0; i--)
cout << arr[i][j] << " ";
cout << '\n';
}
}
// Driver code
int main()
{
int arr[N][N] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90Clockwise(arr);
return 0;
}
// This code is contributed by yashbeersingh42
Java
// Java implementation of above approach
import java.io.*;
class GFG {
static int N = 4;
// Function to rotate the matrix 90 degree clockwise
static void rotate90Clockwise(int arr[][])
{
// printing the matrix on the basis of
// observations made on indices.
for (int j = 0; j < N; j++)
{
for (int i = N - 1; i >= 0; i--)
System.out.print(arr[i][j] + " ");
System.out.println();
}
}
public static void main(String[] args)
{
int arr[][] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90Clockwise(arr);
}
}
// This code is contributed by yashbeersingh42
蟒蛇3
# Python3 implementation of above approach
N = 4
# Function to rotate the matrix 90 degree clockwise
def rotate90Clockwise(arr) :
global N
# printing the matrix on the basis of
# observations made on indices.
for j in range(N) :
for i in range(N - 1, -1, -1) :
print(arr[i][j], end = " ")
print()
# Driver code
arr = [ [ 1, 2, 3, 4 ],
[ 5, 6, 7, 8 ],
[ 9, 10, 11, 12 ],
[ 13, 14, 15, 16 ] ]
rotate90Clockwise(arr);
# This code is contributed by divyesh072019.
C#
// C# implementation of above approach
using System;
class GFG {
static int N = 4;
// Function to rotate the matrix 90 degree clockwise
static void rotate90Clockwise(int[,] arr)
{
// printing the matrix on the basis of
// observations made on indices.
for (int j = 0; j < N; j++)
{
for (int i = N - 1; i >= 0; i--)
Console.Write(arr[i, j] + " ");
Console.WriteLine();
}
}
// Driver code
static void Main() {
int[,] arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90Clockwise(arr);
}
}
// This code is contributed by divyeshrabadiya07.
Javascript
13 9 5 1
14 10 6 2
15 11 7 3
16 12 8 4
方法三:
方法:方法是将给定的矩阵旋转两次,第一次相对于主对角线,下一次相对于中间列旋转合成矩阵,考虑下图可以清楚地了解它。
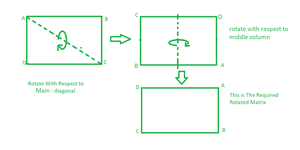
将方阵顺时针旋转 90 度
下面是上述方法的实现:
C++
#include
using namespace std;
#define N 4
void print(int arr[N][N])
{
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < N; ++j)
cout << arr[i][j] << " ";
cout << '\n';
}
}
void rotate(int arr[N][N])
{
// First rotation
// with respect to main diagonal
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < i; ++j)
{
int temp = arr[i][j];
arr[i][j] = arr[j][i];
arr[j][i] = temp;
}
}
// Second rotation
// with respect to middle column
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < N / 2; ++j)
{
int temp = arr[i][j];
arr[i][j] = arr[i][N - j - 1];
arr[i][N - j - 1] = temp;
}
}
}
// Driver code
int main()
{
int arr[N][N] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate(arr);
print(arr);
return 0;
}
// This code is contributed by Rahul Verma
Java
import java.io.*;
class GFG {
static void rotate(int[][] arr) {
int n=arr.length;
// first rotation
// with respect to main diagonal
for(int i=0;i
C#
using System;
using System.Collections.Generic;
public class GFG {
static void rotate(int[,] arr) {
int n=arr.GetLength(0);
// first rotation
// with respect to main diagonal
for(int i=0;i
Javascript
方法四:
方法:这种方法与方法 3 类似,唯一的区别是在第一次旋转时,我们围绕第二对角线旋转,然后围绕中间行旋转。
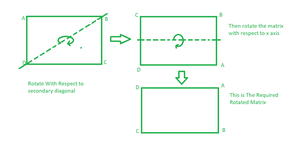
将方阵顺时针旋转 90 度
下面是上述方法的实现:
C++
#include
using namespace std;
#define N 4
void print(int arr[N][N])
{
for(int i = 0; i < N; ++i)
{
for(int j = 0; j < N; ++j)
cout << arr[i][j] << " ";
cout << '\n';
}
}
void rotate(int arr[N][N])
{
// First rotation
// with respect to Secondary diagonal
for(int i = 0; i < N; i++)
{
for(int j = 0; j < N - i; j++)
{
int temp = arr[i][j];
arr[i][j] = arr[N - 1 - j][N - 1 - i];
arr[N - 1 - j][N - 1 - i] = temp;
}
}
// Second rotation
// with respect to middle row
for(int i = 0; i < N / 2; i++)
{
for(int j = 0; j < N; j++)
{
int temp = arr[i][j];
arr[i][j] = arr[N - 1 - i][j];
arr[N - 1 - i][j] = temp;
}
}
}
// Driver code
int main()
{
int arr[N][N] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate(arr);
print(arr);
return 0;
}
// This code is contributed by Rahul Verma
Java
import java.io.*;
class GFG {
static void rotate(int[][] arr)
{
int n = arr.length;
// first rotation
// with respect to Secondary diagonal
for (int i = 0; i < n; i++) {
for (int j = 0; j < n - i; j++) {
int temp = arr[i][j];
arr[i][j] = arr[n - 1 - j][n - 1 - i];
arr[n - 1 - j][n - 1 - i] = temp;
}
}
// Second rotation
// with respect to middle row
for (int i = 0; i < n / 2; i++) {
for (int j = 0; j < n; j++) {
int temp = arr[i][j];
arr[i][j] = arr[n - 1 - i][j];
arr[n - 1 - i][j] = temp;
}
}
}
// to print matrix
static void printMatrix(int arr[][])
{
int n = arr.length;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++)
System.out.print(arr[i][j] + " ");
System.out.println();
}
}
// Driver code
public static void main(String[] args)
{
int arr[][] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate(arr);
printMatrix(arr);
}
}
// This code is contributed by Rahul Verma
C#
using System;
using System.Collections.Generic;
class GFG{
static void rotate(int[,] arr)
{
int n = arr.GetLength(0);
// First rotation
// with respect to Secondary diagonal
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n - i; j++)
{
int temp = arr[i, j];
arr[i, j] = arr[n - 1 - j, n - 1 - i];
arr[n - 1 - j, n - 1 - i] = temp;
}
}
// Second rotation
// with respect to middle row
for(int i = 0; i < n / 2; i++)
{
for(int j = 0; j < n; j++)
{
int temp = arr[i, j];
arr[i, j] = arr[n - 1 - i, j];
arr[n - 1 - i, j] = temp;
}
}
}
// To print matrix
static void printMatrix(int [,]arr)
{
int n = arr.GetLength(0);
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n; j++)
Console.Write(arr[i, j] + " ");
Console.WriteLine();
}
}
// Driver code
public static void Main(String[] args)
{
int [,]arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate(arr);
printMatrix(arr);
}
}
// This code is contributed by aashish1995
如果您希望与专家一起参加现场课程,请参阅DSA 现场工作专业课程和学生竞争性编程现场课程。