将图像旋转 90 度
给定一张图片,你将如何将它旋转 90 度?一个模糊的问题。最小化浏览器并在继续之前尝试您的解决方案。
可以将图像视为可以存储在缓冲区中的二维矩阵。我们提供了矩阵维度和它的基地址。我们怎么转呢?
例如看下图,
* * * ^ * * *
* * * | * * *
* * * | * * *
* * * | * * *
向右旋转后出现(注意箭头方向)
* * * *
* * * *
* * * *
-- - - >
* * * *
* * * *
* * * *
这个想法很简单。将源矩阵的每一行转换为最终图像的所需列。我们将使用辅助缓冲区来转换图像。
从上图我们可以观察到
first row of source ------> last column of destination
second row of source ------> last but-one column of destination
so ... on
last row of source ------> first column of destination
以图形的形式,我们可以将上述 (mxn) 矩阵转换为 (nxm) 矩阵,
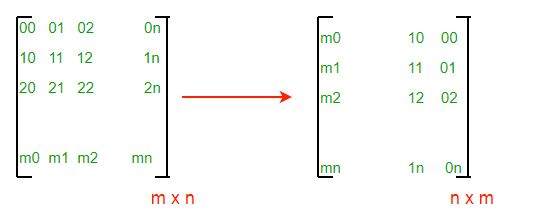
转型
如果你还没有尝试过,至少现在试试你的伪代码。
编写我们的伪代码将很容易。在 C/C++ 中,我们通常会按行主要顺序遍历矩阵。每一行都被转换成最终图像的不同列。我们需要构建最终图像的列。见以下算法(变换)
for (r = 0; r < m; r++)
{
for (c = 0; c < n; c++)
{
// Hint: Map each source element indices into
// indices of destination matrix element.
dest_buffer [ c ] [ m - r - 1 ] = source_buffer [ r ] [ c ];
}
}
请注意,有多种方法可以实现基于矩阵遍历、行主要或列主要顺序的算法。我们有两个矩阵和两种方法(行和列主要)来遍历每个矩阵。因此,至少可以有 4 种不同的方式将源矩阵转换为最终矩阵。
C++
// C++ program to turn an
// image by 90 Degree
#include
using namespace std;
void displayMatrix(unsigned int const *p,
unsigned int row,
unsigned int col);
void rotate(unsigned int *pS,
unsigned int *pD,
unsigned int row,
unsigned int col);
void displayMatrix(unsigned int const *p,
unsigned int r,
unsigned int c)
{
unsigned int row, col;
cout << "\n\n";
for (row = 0; row < r; row++)
{
for (col = 0; col < c; col++)
cout << * (p + row * c + col) << "\t";
cout << "\n";
}
cout << "\n\n";
}
void rotate(unsigned int *pS,
unsigned int *pD,
unsigned int row,
unsigned int col)
{
unsigned int r, c;
for (r = 0; r < row; r++)
{
for (c = 0; c < col; c++)
{
*(pD + c * row + (row - r - 1)) =
*(pS + r * col + c);
}
}
}
// Driver Code
int main()
{
// declarations
unsigned int image[][4] = {{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}};
unsigned int *pSource;
unsigned int *pDestination;
unsigned int m, n;
// setting initial values
// and memory allocation
m = 3, n = 4, pSource = (unsigned int *)image;
pDestination = (unsigned int *)malloc
(sizeof(int) * m * n);
// process each buffer
displayMatrix(pSource, m, n);
rotate(pSource, pDestination, m, n);
displayMatrix(pDestination, n, m);
free(pDestination);
return 0;
}
// This code is contributed by rathbhupendra
C
// C program to turn an
// image by 90 Degree
#include
#include
void displayMatrix(unsigned int const *p,
unsigned int row,
unsigned int col);
void rotate(unsigned int *pS,
unsigned int *pD,
unsigned int row,
unsigned int col);
void displayMatrix(unsigned int const *p,
unsigned int r,
unsigned int c)
{
unsigned int row, col;
printf("\n\n");
for (row = 0; row < r; row++)
{
for (col = 0; col < c; col++)
printf("%d\t", * (p + row * c + col));
printf("\n");
}
printf("\n\n");
}
void rotate(unsigned int *pS,
unsigned int *pD,
unsigned int row,
unsigned int col)
{
unsigned int r, c;
for (r = 0; r < row; r++)
{
for (c = 0; c < col; c++)
{
*(pD + c * row + (row - r - 1)) =
*(pS + r * col + c);
}
}
}
// Driver Code
int main()
{
// declarations
unsigned int image[][4] = {{1,2,3,4},
{5,6,7,8},
{9,10,11,12}};
unsigned int *pSource;
unsigned int *pDestination;
unsigned int m, n;
// setting initial values
// and memory allocation
m = 3, n = 4, pSource = (unsigned int *)image;
pDestination =
(unsigned int *)malloc
(sizeof(int) * m * n);
// process each buffer
displayMatrix(pSource, m, n);
rotate(pSource, pDestination, m, n);
displayMatrix(pDestination, n, m);
free(pDestination);
getchar();
return 0;
}
输出 :
1 2 3 4
5 6 7 8
9 10 11 12
9 5 1
10 6 2
11 7 3
12 8 4
将方阵原地旋转 90 度