在不使用任何额外空间的情况下将矩阵旋转 90 度 |设置 2
给定一个方阵,在不使用任何额外空间的情况下将其逆时针方向旋转 90 度。
例子:
Input:
1 2 3
4 5 6
7 8 9
Output:
3 6 9
2 5 8
1 4 7
Rotated the input matrix by
90 degrees in anti-clockwise direction.
Input:
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
Output:
4 8 12 16
3 7 11 15
2 6 10 14
1 5 9 13
Rotated the input matrix by
90 degrees in anti-clockwise direction.
需要额外空间的方法已经在另一篇文章中讨论过:
原地旋转方阵 90 度 |设置 1
这篇文章使用空间优化的不同方法讨论了相同的问题。
方法:想法是找到矩阵的转置,然后反转转置矩阵的列。
下面是一个例子来展示它是如何工作的。
算法:
- 为了解决给定的问题,有两个任务。第一个是找到转置,第二个是在不使用额外空间的情况下反转列
- 矩阵的转置是指矩阵在其对角线上翻转,即元素的行索引变为列索引,反之亦然。因此,要找到转置,将位置 (i, j) 处的元素与 (j, i) 互换。运行两个循环,外循环从 0 到行数,内循环从 0 到外循环的索引。
- 要反转转置矩阵的列,请运行两个嵌套循环,外循环从 0 到列数,内循环从 0 到行数/2,将 (i, j) 处的元素与 (i, row[count-1 -j]),其中 i 和 j 分别是内循环和外循环的索引。
C++
// C++ program for left
// rotation of matrix by 90 degree
// without using extra space
#include
using namespace std;
#define R 4
#define C 4
// After transpose we swap
// elements of column
// one by one for finding left
// rotation of matrix
// by 90 degree
void reverseColumns(int arr[R][C])
{
for (int i = 0; i < C; i++)
for (int j = 0, k = C - 1; j < k; j++, k--)
swap(arr[j][i], arr[k][i]);
}
// Function for do transpose of matrix
void transpose(int arr[R][C])
{
for (int i = 0; i < R; i++)
for (int j = i; j < C; j++)
swap(arr[i][j], arr[j][i]);
}
// Function for print matrix
void printMatrix(int arr[R][C])
{
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++)
cout << arr[i][j] << " ";
cout << '\n';
}
}
// Function to anticlockwise
// rotate matrix by 90 degree
void rotate90(int arr[R][C])
{
transpose(arr);
reverseColumns(arr);
}
// Driven code
int main()
{
int arr[R][C] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90(arr);
printMatrix(arr);
return 0;
}
Java
// JAVA Code for left Rotation of a
// matrix by 90 degree without using
// any extra space
import java.util.*;
class GFG {
// After transpose we swap elements of
// column one by one for finding left
// rotation of matrix by 90 degree
static void reverseColumns(int arr[][])
{
for (int i = 0; i < arr[0].length; i++)
for (int j = 0, k = arr[0].length - 1; j < k;
j++, k--) {
int temp = arr[j][i];
arr[j][i] = arr[k][i];
arr[k][i] = temp;
}
}
// Function for do transpose of matrix
static void transpose(int arr[][])
{
for (int i = 0; i < arr.length; i++)
for (int j = i; j < arr[0].length; j++) {
int temp = arr[j][i];
arr[j][i] = arr[i][j];
arr[i][j] = temp;
}
}
// Function for print matrix
static void printMatrix(int arr[][])
{
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[0].length; j++)
System.out.print(arr[i][j] + " ");
System.out.println("");
}
}
// Function to anticlockwise rotate
// matrix by 90 degree
static void rotate90(int arr[][])
{
transpose(arr);
reverseColumns(arr);
}
/* Driver program to test above function */
public static void main(String[] args)
{
int arr[][] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90(arr);
printMatrix(arr);
}
}
// This code is contributed by Arnav Kr. Mandal.
C#
// C# program for left rotation
// of matrix by 90 degree
// without using extra space
using System;
class GFG {
static int R = 4;
static int C = 4;
// After transpose we swap
// elements of column one
// by one for finding left
// rotation of matrix by
// 90 degree
static void reverseColumns(int[, ] arr)
{
for (int i = 0; i < C; i++)
for (int j = 0, k = C - 1; j < k; j++, k--) {
int temp = arr[j, i];
arr[j, i] = arr[k, i];
arr[k, i] = temp;
}
}
// Function for do
// transpose of matrix
static void transpose(int[, ] arr)
{
for (int i = 0; i < R; i++)
for (int j = i; j < C; j++) {
int temp = arr[j, i];
arr[j, i] = arr[i, j];
arr[i, j] = temp;
}
}
// Function for print matrix
static void printMatrix(int[, ] arr)
{
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++)
Console.Write(arr[i, j] + " ");
Console.WriteLine("");
}
}
// Function to anticlockwise
// rotate matrix by 90 degree
static void rotate90(int[, ] arr)
{
transpose(arr);
reverseColumns(arr);
}
// Driver code
static void Main()
{
int[, ] arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotate90(arr);
printMatrix(arr);
}
// This code is contributed
// by Sam007
}
Python3
# Python 3 program for left rotation of matrix by 90
# degree without using extra space
R = 4
C = 4
# After transpose we swap elements of column
# one by one for finding left rotation of matrix
# by 90 degree
def reverseColumns(arr):
for i in range(C):
j = 0
k = C-1
while j < k:
t = arr[j][i]
arr[j][i] = arr[k][i]
arr[k][i] = t
j += 1
k -= 1
# Function for do transpose of matrix
def transpose(arr):
for i in range(R):
for j in range(i, C):
t = arr[i][j]
arr[i][j] = arr[j][i]
arr[j][i] = t
# Function for print matrix
def printMatrix(arr):
for i in range(R):
for j in range(C):
print(str(arr[i][j]), end=" ")
print()
# Function to anticlockwise rotate matrix
# by 90 degree
def rotate90(arr):
transpose(arr)
reverseColumns(arr)
# Driven code
arr = [[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12],
[13, 14, 15, 16]
]
rotate90(arr)
printMatrix(arr)
PHP
Javascript
C++14
// C++ program for left rotation of matrix
// by 90 degree without using extra space
#include
using namespace std;
#define R 4
#define C 4
// Function to rotate matrix anticlockwise by 90 degrees.
void rotateby90(int arr[R][C])
{
int n = R; // n=size of the square matrix
int a = 0, b = 0, c = 0, d = 0;
// iterate over all the boundaries of the matrix
for (int i = 0; i <= n / 2 - 1; i++) {
// for each boundary, keep on taking 4 elements (one
// each along the 4 edges) and swap them in
// anticlockwise manner
for (int j = 0; j <= n - 2 * i - 2; j++) {
a = arr[i + j][i];
b = arr[n - 1 - i][i + j];
c = arr[n - 1 - i - j][n - 1 - i];
d = arr[i][n - 1 - i - j];
arr[i + j][i] = d;
arr[n - 1 - i][i + j] = a;
arr[n - 1 - i - j][n - 1 - i] = b;
arr[i][n - 1 - i - j] = c;
}
}
}
// Function for print matrix
void printMatrix(int arr[R][C])
{
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++)
cout << arr[i][j] << " ";
cout << '\n';
}
}
// Driven code
int main()
{
int arr[R][C] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotateby90(arr);
printMatrix(arr);
return 0;
}
// This code is contributed by Md. Enjamum
// Hossain(enja_2001)
Java
// JAVA Code for left Rotation of a matrix
// by 90 degree without using any extra space
import java.util.*;
class GFG {
// Function to rotate matrix anticlockwise by 90
// degrees.
static void rotateby90(int arr[][])
{
int n = arr.length;
int a = 0, b = 0, c = 0, d = 0;
// iterate over all the boundaries of the matrix
for (int i = 0; i <= n / 2 - 1; i++) {
// for each boundary, keep on taking 4 elements
// (one each along the 4 edges) and swap them in
// anticlockwise manner
for (int j = 0; j <= n - 2 * i - 2; j++) {
a = arr[i + j][i];
b = arr[n - 1 - i][i + j];
c = arr[n - 1 - i - j][n - 1 - i];
d = arr[i][n - 1 - i - j];
arr[i + j][i] = d;
arr[n - 1 - i][i + j] = a;
arr[n - 1 - i - j][n - 1 - i] = b;
arr[i][n - 1 - i - j] = c;
}
}
}
// Function for print matrix
static void printMatrix(int arr[][])
{
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[0].length; j++)
System.out.print(arr[i][j] + " ");
System.out.println("");
}
}
/* Driver program to test above function */
public static void main(String[] args)
{
int arr[][] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotateby90(arr);
printMatrix(arr);
}
}
// This code is contributed by Md. Enjamum
// Hossain(enja_2001)
C#
// C# Code for left Rotation of a matrix
// by 90 degree without using any extra space
using System;
using System.Collections.Generic;
public class GFG {
// Function to rotate matrix anticlockwise by 90
// degrees.
static void rotateby90(int [,]arr)
{
int n = arr.GetLength(0);
int a = 0, b = 0, c = 0, d = 0;
// iterate over all the boundaries of the matrix
for (int i = 0; i <= n / 2 - 1; i++) {
// for each boundary, keep on taking 4 elements
// (one each along the 4 edges) and swap them in
// anticlockwise manner
for (int j = 0; j <= n - 2 * i - 2; j++) {
a = arr[i + j,i];
b = arr[n - 1 - i,i + j];
c = arr[n - 1 - i - j,n - 1 - i];
d = arr[i,n - 1 - i - j];
arr[i + j,i] = d;
arr[n - 1 - i,i + j] = a;
arr[n - 1 - i - j,n - 1 - i] = b;
arr[i,n - 1 - i - j] = c;
}
}
}
// Function for print matrix
static void printMatrix(int [,]arr)
{
for (int i = 0; i < arr.GetLength(0); i++) {
for (int j = 0; j < arr.GetLength(1); j++)
Console.Write(arr[i,j] + " ");
Console.WriteLine("");
}
}
/* Driver program to test above function */
public static void Main(String[] args)
{
int [,]arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotateby90(arr);
printMatrix(arr);
}
}
// This code is contributed by umadevi9616
Javascript
Python3
# Alternative implementation using numpy
import numpy
# Driven code
arr = [[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12],
[13, 14, 15, 16]
]
# Define flip algorithm (Note numpy.flip is a builtin f
# function for versions > v.1.12.0)
def flip(m, axis):
if not hasattr(m, 'ndim'):
m = asarray(m)
indexer = [slice(None)] * m.ndim
try:
indexer[axis] = slice(None, None, -1)
except IndexError:
raise ValueError("axis =% i is invalid for the % i-dimensional input array"
% (axis, m.ndim))
return m[tuple(indexer)]
# Transpose the matrix
trans = numpy.transpose(arr)
# Flip the matrix anti-clockwise (1 for clockwise)
flipmat = flip(trans, 0)
print("\nnumpy implementation\n")
print(flipmat)
C++
#include
using namespace std;
//Function to rotate matrix anticlockwise by 90 degrees.
void rotateby90(vector >& matrix)
{
int n=matrix.size();
int mid;
if(n%2==0)
mid=n/2-1;
else
mid=n/2;
for(int i=0,j=n-1;i<=mid;i++,j--)
{
for(int k=0;k>& arr)
{
int n=arr.size();
for(int i=0;i> arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotateby90(arr);
printMatrix(arr);
return 0;
}
Java
import java.util.*;
class GFG{
private static int[][] swap(int[][] matrix, int x1,int x2,int y1, int y2) {
int temp = matrix[x1][x2] ;
matrix[x1][x2] = matrix[y1][y2];
matrix[y1][y2] = temp;
return matrix;
}
//Function to rotate matrix anticlockwise by 90 degrees.
static int[][] rotateby90(int[][] matrix)
{
int n=matrix.length;
int mid;
if(n % 2 == 0)
mid = n / 2 - 1;
else
mid = n / 2;
for(int i = 0, j = n - 1; i <= mid; i++, j--)
{
for(int k = 0; k < j - i; k++)
{
matrix= swap(matrix, i, j - k, j, i + k); //ru ld
matrix= swap(matrix, i + k, i, j, i + k); //lu ld
matrix= swap(matrix, i, j - k, j - k, j); //ru rd
}
}
return matrix;
}
static void printMatrix(int[][] arr)
{
int n = arr.length;
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n; j++)
{
System.out.print(arr[i][j] + " ");
}
System.out.println();
}
}
public static void main(String[] args) {
int[][] arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
arr = rotateby90(arr);
printMatrix(arr);
}
}
// This code is contributed by umadevi9616
C#
using System;
public class GFG{
private static int[,] swap(int[,] matrix, int x1,int x2,int y1, int y2) {
int temp = matrix[x1,x2] ;
matrix[x1,x2] = matrix[y1,y2];
matrix[y1,y2] = temp;
return matrix;
}
//Function to rotate matrix anticlockwise by 90 degrees.
static int[,] rotateby90(int[,] matrix)
{
int n=matrix.GetLength(0);
int mid;
if(n % 2 == 0)
mid = (n / 2 - 1);
else
mid = (n / 2);
for(int i = 0, j = n - 1; i <= mid; i++, j--)
{
for(int k = 0; k < j - i; k++)
{
matrix= swap(matrix, i, j - k, j, i + k); //ru ld
matrix= swap(matrix, i + k, i, j, i + k); //lu ld
matrix= swap(matrix, i, j - k, j - k, j); //ru rd
}
}
return matrix;
}
static void printMatrix(int[,] arr)
{
int n = arr.GetLength(0);
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n; j++)
{
Console.Write(arr[i,j] + " ");
}
Console.WriteLine();
}
}
public static void Main(String[] args) {
int[,] arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
arr = rotateby90(arr);
printMatrix(arr);
}
}
// This code is contributed by Rajput-Ji
Javascript
4 8 12 16
3 7 11 15
2 6 10 14
1 5 9 13
复杂性分析:
- 时间复杂度: O(R*C)。
矩阵被遍历了两次,所以复杂度是 O(R*C)。 - 空间复杂度: O(1)。
空间复杂度是恒定的,因为不需要额外的空间。
另一种使用矩阵单次遍历的方法:
这个想法是沿着矩阵的边界遍历,并在每个边界逆时针方向移动元素的位置 90 0 。矩阵中有这样的 (n/2-1) 个边界。
算法:
- 遍历矩阵中的所有边界。共有 (n/2-1) 个边界
- 对于每个边界,取 4 个角元素并交换它们,使 4 个角元素沿逆时针方向旋转。然后沿边缘(左、右、上、下)取下 4 个元素并以逆时针方向交换它们。只要该特定边界中的所有元素都沿逆时针方向旋转 90 0就可以继续。
- 然后移动到下一个内边界并继续该过程,只要整个矩阵逆时针方向旋转 90 0即可。

原始数组
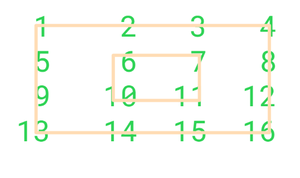
总共 n/2-1 个边界

对于最外边界
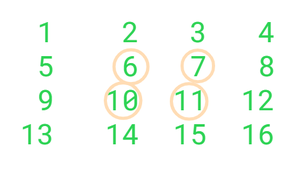
对于下一个内边界
C++14
// C++ program for left rotation of matrix
// by 90 degree without using extra space
#include
using namespace std;
#define R 4
#define C 4
// Function to rotate matrix anticlockwise by 90 degrees.
void rotateby90(int arr[R][C])
{
int n = R; // n=size of the square matrix
int a = 0, b = 0, c = 0, d = 0;
// iterate over all the boundaries of the matrix
for (int i = 0; i <= n / 2 - 1; i++) {
// for each boundary, keep on taking 4 elements (one
// each along the 4 edges) and swap them in
// anticlockwise manner
for (int j = 0; j <= n - 2 * i - 2; j++) {
a = arr[i + j][i];
b = arr[n - 1 - i][i + j];
c = arr[n - 1 - i - j][n - 1 - i];
d = arr[i][n - 1 - i - j];
arr[i + j][i] = d;
arr[n - 1 - i][i + j] = a;
arr[n - 1 - i - j][n - 1 - i] = b;
arr[i][n - 1 - i - j] = c;
}
}
}
// Function for print matrix
void printMatrix(int arr[R][C])
{
for (int i = 0; i < R; i++) {
for (int j = 0; j < C; j++)
cout << arr[i][j] << " ";
cout << '\n';
}
}
// Driven code
int main()
{
int arr[R][C] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotateby90(arr);
printMatrix(arr);
return 0;
}
// This code is contributed by Md. Enjamum
// Hossain(enja_2001)
Java
// JAVA Code for left Rotation of a matrix
// by 90 degree without using any extra space
import java.util.*;
class GFG {
// Function to rotate matrix anticlockwise by 90
// degrees.
static void rotateby90(int arr[][])
{
int n = arr.length;
int a = 0, b = 0, c = 0, d = 0;
// iterate over all the boundaries of the matrix
for (int i = 0; i <= n / 2 - 1; i++) {
// for each boundary, keep on taking 4 elements
// (one each along the 4 edges) and swap them in
// anticlockwise manner
for (int j = 0; j <= n - 2 * i - 2; j++) {
a = arr[i + j][i];
b = arr[n - 1 - i][i + j];
c = arr[n - 1 - i - j][n - 1 - i];
d = arr[i][n - 1 - i - j];
arr[i + j][i] = d;
arr[n - 1 - i][i + j] = a;
arr[n - 1 - i - j][n - 1 - i] = b;
arr[i][n - 1 - i - j] = c;
}
}
}
// Function for print matrix
static void printMatrix(int arr[][])
{
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[0].length; j++)
System.out.print(arr[i][j] + " ");
System.out.println("");
}
}
/* Driver program to test above function */
public static void main(String[] args)
{
int arr[][] = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotateby90(arr);
printMatrix(arr);
}
}
// This code is contributed by Md. Enjamum
// Hossain(enja_2001)
C#
// C# Code for left Rotation of a matrix
// by 90 degree without using any extra space
using System;
using System.Collections.Generic;
public class GFG {
// Function to rotate matrix anticlockwise by 90
// degrees.
static void rotateby90(int [,]arr)
{
int n = arr.GetLength(0);
int a = 0, b = 0, c = 0, d = 0;
// iterate over all the boundaries of the matrix
for (int i = 0; i <= n / 2 - 1; i++) {
// for each boundary, keep on taking 4 elements
// (one each along the 4 edges) and swap them in
// anticlockwise manner
for (int j = 0; j <= n - 2 * i - 2; j++) {
a = arr[i + j,i];
b = arr[n - 1 - i,i + j];
c = arr[n - 1 - i - j,n - 1 - i];
d = arr[i,n - 1 - i - j];
arr[i + j,i] = d;
arr[n - 1 - i,i + j] = a;
arr[n - 1 - i - j,n - 1 - i] = b;
arr[i,n - 1 - i - j] = c;
}
}
}
// Function for print matrix
static void printMatrix(int [,]arr)
{
for (int i = 0; i < arr.GetLength(0); i++) {
for (int j = 0; j < arr.GetLength(1); j++)
Console.Write(arr[i,j] + " ");
Console.WriteLine("");
}
}
/* Driver program to test above function */
public static void Main(String[] args)
{
int [,]arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotateby90(arr);
printMatrix(arr);
}
}
// This code is contributed by umadevi9616
Javascript
4 8 12 16
3 7 11 15
2 6 10 14
1 5 9 13
复杂性分析:
时间复杂度:O(R*C)。矩阵只遍历一次,所以时间复杂度为 O(R*C)。
空间复杂度:O(1) 。空间复杂度为 O(1),因为不需要额外的空间。
实现:让我们看看Python numpy 的一种方法,它可以用来得出特定的解决方案。
Python3
# Alternative implementation using numpy
import numpy
# Driven code
arr = [[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12],
[13, 14, 15, 16]
]
# Define flip algorithm (Note numpy.flip is a builtin f
# function for versions > v.1.12.0)
def flip(m, axis):
if not hasattr(m, 'ndim'):
m = asarray(m)
indexer = [slice(None)] * m.ndim
try:
indexer[axis] = slice(None, None, -1)
except IndexError:
raise ValueError("axis =% i is invalid for the % i-dimensional input array"
% (axis, m.ndim))
return m[tuple(indexer)]
# Transpose the matrix
trans = numpy.transpose(arr)
# Flip the matrix anti-clockwise (1 for clockwise)
flipmat = flip(trans, 0)
print("\nnumpy implementation\n")
print(flipmat)
输出:
numpy implementation
[[ 4 8 12 16]
[ 3 7 11 15]
[ 2 6 10 14]
[ 1 5 9 13]]
注意:上述步骤/程序向左(或逆时针)旋转。让我们看看如何正确旋转或顺时针旋转。该方法将是类似的。找到矩阵的转置,然后反转转置矩阵的行。
这就是它的完成方式。
沿边界旋转
我们可以从给定矩阵的前 4 个角开始,然后不断增加行和列索引以移动。
在任何给定时刻,我们都会有四个角 lu(左上)、ld(左下)、ru(右上)、rd(右下)。
向左旋转,我们将首先交换 ru 和 ld,然后是 lu 和 ld,最后是 ru 和 rd。 ru lu rd ld
C++
#include
using namespace std;
//Function to rotate matrix anticlockwise by 90 degrees.
void rotateby90(vector >& matrix)
{
int n=matrix.size();
int mid;
if(n%2==0)
mid=n/2-1;
else
mid=n/2;
for(int i=0,j=n-1;i<=mid;i++,j--)
{
for(int k=0;k>& arr)
{
int n=arr.size();
for(int i=0;i> arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
rotateby90(arr);
printMatrix(arr);
return 0;
}
Java
import java.util.*;
class GFG{
private static int[][] swap(int[][] matrix, int x1,int x2,int y1, int y2) {
int temp = matrix[x1][x2] ;
matrix[x1][x2] = matrix[y1][y2];
matrix[y1][y2] = temp;
return matrix;
}
//Function to rotate matrix anticlockwise by 90 degrees.
static int[][] rotateby90(int[][] matrix)
{
int n=matrix.length;
int mid;
if(n % 2 == 0)
mid = n / 2 - 1;
else
mid = n / 2;
for(int i = 0, j = n - 1; i <= mid; i++, j--)
{
for(int k = 0; k < j - i; k++)
{
matrix= swap(matrix, i, j - k, j, i + k); //ru ld
matrix= swap(matrix, i + k, i, j, i + k); //lu ld
matrix= swap(matrix, i, j - k, j - k, j); //ru rd
}
}
return matrix;
}
static void printMatrix(int[][] arr)
{
int n = arr.length;
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n; j++)
{
System.out.print(arr[i][j] + " ");
}
System.out.println();
}
}
public static void main(String[] args) {
int[][] arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
arr = rotateby90(arr);
printMatrix(arr);
}
}
// This code is contributed by umadevi9616
C#
using System;
public class GFG{
private static int[,] swap(int[,] matrix, int x1,int x2,int y1, int y2) {
int temp = matrix[x1,x2] ;
matrix[x1,x2] = matrix[y1,y2];
matrix[y1,y2] = temp;
return matrix;
}
//Function to rotate matrix anticlockwise by 90 degrees.
static int[,] rotateby90(int[,] matrix)
{
int n=matrix.GetLength(0);
int mid;
if(n % 2 == 0)
mid = (n / 2 - 1);
else
mid = (n / 2);
for(int i = 0, j = n - 1; i <= mid; i++, j--)
{
for(int k = 0; k < j - i; k++)
{
matrix= swap(matrix, i, j - k, j, i + k); //ru ld
matrix= swap(matrix, i + k, i, j, i + k); //lu ld
matrix= swap(matrix, i, j - k, j - k, j); //ru rd
}
}
return matrix;
}
static void printMatrix(int[,] arr)
{
int n = arr.GetLength(0);
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n; j++)
{
Console.Write(arr[i,j] + " ");
}
Console.WriteLine();
}
}
public static void Main(String[] args) {
int[,] arr = { { 1, 2, 3, 4 },
{ 5, 6, 7, 8 },
{ 9, 10, 11, 12 },
{ 13, 14, 15, 16 } };
arr = rotateby90(arr);
printMatrix(arr);
}
}
// This code is contributed by Rajput-Ji
Javascript
输出:
4 8 12 16
3 7 11 15
2 6 10 14
1 5 9 13
时间复杂度:O(n^2)
空间复杂度:O(1)