给定N边的多边形,我们需要找到通过将给定多边形的顶点与正好是公共的一侧连接起来而形成的三角形的数量。
例子:
Input : 6
Output : 12
The image below is of a triangle forming inside a Hexagon by joining vertices as shown above. The two triangles formed has one side (AB) common with that of a polygon.It depicts that with one edge of a hexagon we can make two triangles with one side common. We can’t take C or F as our third vertex as it will make 2 sides common with the hexagon.
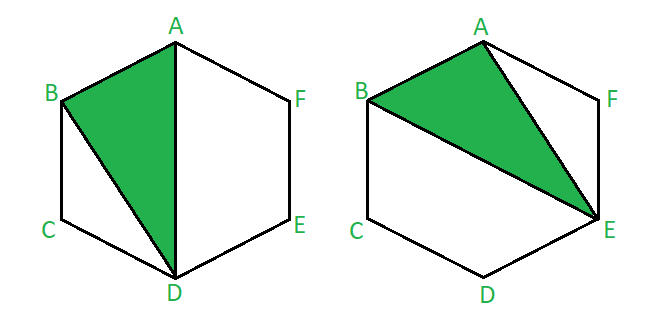
Triangle with one side common of a hexagon
No of triangles formed ie equal to 12 as there are 6 edges in a hexagon.
Input : 5
Output : 5
方法 :
- 若要使一个边与多边形共同的三角形,不能将与所选共同顶点相邻的两个顶点视为三角形的第三个顶点。
- 首先从多边形中选择任意一条边。将此边缘视为公共边缘。在多边形中选择边的方法数将等于n。
- 现在,要形成一个三角形,请选择任意(n-4)个顶点。不能考虑公共边的两个顶点和与公共边相邻的两个顶点。
- 通过将一个n边多边形的顶点与一个边共同连接而形成的三角形数将等于n *(n-4)。
下面是上述方法的实现:
C++
// C++ program to implement
// the above problem
#include
using namespace std;
// Function to find the number of triangles
void findTriangles(int n)
{
int num;
num = n * (n - 4);
// print the number of triangles
cout << num;
}
// Driver code
int main()
{
// initialize the number of sides of a polygon
int n;
n = 6;
findTriangles(n);
return 0;
}
Java
// Java program to implement
// the above problem
class GFG
{
// Function to find the number of triangles
static void findTriangles(int n)
{
int num;
num = n * (n - 4);
// print the number of triangles
System.out.println(num);
}
// Driver code
public static void main(String [] args)
{
// initialize the number of sides of a polygon
int n;
n = 6;
findTriangles(n);
}
}
// This code is contributed by ihritik
Python3
# Python3 program to implement
# the above problem
# Function to find the number of triangles
def findTriangles(n):
num = n * (n - 4)
# print the number of triangles
print(num)
# Driver code
# initialize the number of sides of a polygon
n = 6
findTriangles(n)
# This codee is contributed by mohit kumar 29
C#
// C# program to implement
// the above problem
using System;
class GFG
{
// Function to find the number of triangles
static void findTriangles(int n)
{
int num;
num = n * (n - 4);
// print the number of triangles
Console.WriteLine(num);
}
// Driver code
public static void Main()
{
// initialize the number of sides of a polygon
int n;
n = 6;
findTriangles(n);
}
}
// This code is contributed by ihritik
Javascript
输出:
12
时间复杂度: O(1)