最大化给定数组及其反转数组的相应索引处的元素总和
给定一个包含N个整数的数组arr[] ,任务是找到通过将原始数组和反转数组的相同索引处的元素相加获得的最大和。
例子:
Input: arr[]={ 1, 8, 9, 5, 4, 6 }
Output: 14
Explanation:
Original array : {1, 8, 9, 5, 4, 6}
Reversed array: {6, 4, 5, 9, 8, 1}
Adding corresponding indexes element:
{1+6=7, 8+4=12, 9+5=14, 5+9=14, 4+8=12, 6+1=7}
So, The Maximum sum is 14.
Input: arr[]={-31, 5, -1, 7, -5}
Output: 12
朴素的做法:创建一个反转数组,并在添加相应的索引元素后返回最大和。
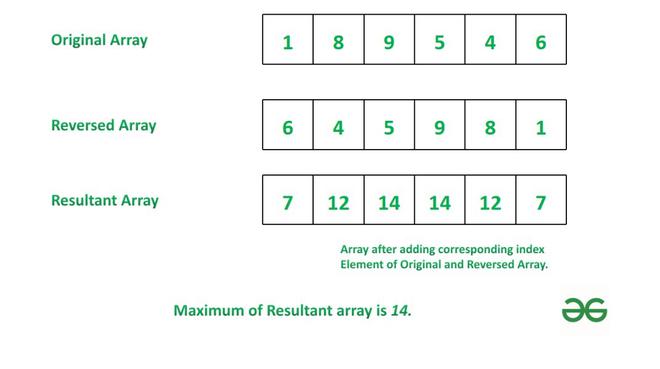
添加对应的反转数组元素后的最大和
下面是上述方法的实现
C++
// C++ program for the above approach
#include
using namespace std;
// Function to find the maximum
// sum obtained by adding the
// elements at the same index of
// the original array and of
// the reversed array
int maximumSum(int arr[], int n)
{
int c = 0;
// Creating reversed array
int reversed[n];
for (int i = n - 1; i >= 0; i--)
reversed = arr[i];
int res = INT_MIN;
// Adding corresponding
// indexes of original
// and reversed array
for (int i = 0; i < n; i++) {
res = std::max(res,
arr[i] + reversed[i]);
}
return res;
}
// Driver Code
int main()
{
int arr[] = { 1, 8, 9, 5, 4, 6 };
int n = sizeof(arr) / sizeof(arr[0]);
cout << maximumSum(arr, n);
return 0;
}
Java
/*package whatever //do not write package name here */
import java.io.*;
class GFG {
// Function to find the maximum
// sum obtained by adding the
// elements at the same index of
// the original array and of
// the reversed array
static int maximumSum(int[] arr, int n)
{
int c = 0;
// Creating reversed array
int[] reversed = new int[n];
for (int i = n - 1; i >= 0; i--)
reversed = arr[i];
int res = Integer.MIN_VALUE;
// Adding corresponding
// indexes of original
// and reversed array
for (int i = 0; i < n; i++) {
res = Math.max(res, arr[i] + reversed[i]);
}
return res;
}
// Driver Code
public static void main(String[] args)
{
int arr[] = { 1, 8, 9, 5, 4, 6 };
int n = arr.length;
System.out.println(maximumSum(arr, n));
}
}
// This code is contributed by maddler.
Python3
# Python 3 program for the above approach
import sys
# Function to find the maximum
# sum obtained by adding the
# elements at the same index of
# the original array and of
# the reversed array
def maximumSum(arr, n):
c = 0
# Creating reversed array
reversed = [0]*n
for i in range(n - 1, -1, -1):
reversed = arr[i]
c += 1
res = -sys.maxsize - 1
# Adding corresponding
# indexes of original
# and reversed array
for i in range(n):
res = max(res,
arr[i] + reversed[i])
return res
# Driver Code
if __name__ == "__main__":
arr = [1, 8, 9, 5, 4, 6]
n = len(arr)
print(maximumSum(arr, n))
# This code is contributed by ukasp.
C#
/*package whatever //do not write package name here */
using System;
public class GFG {
// Function to find the maximum
// sum obtained by adding the
// elements at the same index of
// the original array and of
// the reversed array
static int maximumSum(int[] arr, int n) {
int c = 0;
// Creating reversed array
int[] reversed = new int[n];
for (int i = n - 1; i >= 0; i--)
reversed = arr[i];
int res = int.MinValue;
// Adding corresponding
// indexes of original
// and reversed array
for (int i = 0; i < n; i++) {
res = Math.Max(res, arr[i] + reversed[i]);
}
return res;
}
// Driver Code
public static void Main(String[] args) {
int []arr = { 1, 8, 9, 5, 4, 6 };
int n = arr.Length;
Console.WriteLine(maximumSum(arr, n));
}
}
// This code is contributed by gauravrajput1
Javascript
C++
// C++ program for the above approach
#include
using namespace std;
// Function to find the maximum
// sum obtained by adding the
// elements at the same index of
// the original array and of
// the reversed array
int maximumSum(int arr[], int n)
{
// Creating i as front pointer
// and j as rear pointer
int i = 0, j = n - 1;
int max = INT_MIN;
while (i <= j) {
if (max < arr[i] + arr[j])
max = arr[i] + arr[j];
i++;
j--;
}
// Returning the maximum value
return max;
}
// Driver Code
int main()
{
int arr[] = { 1, 8, 9, 5, 4, 6 };
int n = sizeof(arr) / sizeof(arr[0]);
cout << maximumSum(arr, n);
return 0;
}
Java
// Java program for the above approach
import java.util.*;
public class GFG {
// Function to find the maximum
// sum obtained by adding the
// elements at the same index of
// the original array and of
// the reversed array
static int maximumSum(int []arr, int n)
{
// Creating i as front pointer
// and j as rear pointer
int i = 0, j = n - 1;
int max = Integer.MIN_VALUE;
while (i <= j) {
if (max < arr[i] + arr[j])
max = arr[i] + arr[j];
i++;
j--;
}
// Returning the maximum value
return max;
}
// Driver Code
public static void main(String args[])
{
int []arr = { 1, 8, 9, 5, 4, 6 };
int n = arr.length;
System.out.println(maximumSum(arr, n));
}
}
// This code is contributed by Samim Hossain Mondal.
Python3
# python program for the above approach
INT_MIN = -2147483647 - 1
# Function to find the maximum
# sum obtained by adding the
# elements at the same index of
# the original array and of
# the reversed array
def maximumSum(arr, n):
# Creating i as front pointer
# and j as rear pointer
i = 0
j = n - 1
max = INT_MIN
while (i <= j):
if (max < arr[i] + arr[j]):
max = arr[i] + arr[j]
i += 1
j -= 1
# Returning the maximum value
return max
# Driver Code
if __name__ == "__main__":
arr = [1, 8, 9, 5, 4, 6]
n = len(arr)
print(maximumSum(arr, n))
# This code is contributed by rakeshsahni
C#
// C# program for the above approach
using System;
class GFG
{
// Function to find the maximum
// sum obtained by adding the
// elements at the same index of
// the original array and of
// the reversed array
static int maximumSum(int []arr, int n)
{
// Creating i as front pointer
// and j as rear pointer
int i = 0, j = n - 1;
int max = Int32.MinValue;
while (i <= j) {
if (max < arr[i] + arr[j])
max = arr[i] + arr[j];
i++;
j--;
}
// Returning the maximum value
return max;
}
// Driver Code
public static void Main()
{
int []arr = { 1, 8, 9, 5, 4, 6 };
int n = arr.Length;
Console.Write(maximumSum(arr, n));
}
}
// This code is contributed by Samim Hossain Mondal.
Javascript
输出:
14
时间复杂度: O(N)
辅助空间: O(N)
有效方法:这个问题可以使用两指针算法来解决。因此,请按照以下步骤找到答案:
- 创建一个指向数组中第一个元素的前指针和一个指向最后一个元素的后指针。
- 现在运行一个循环,直到这两个指针相互交叉。在每次迭代中:
- 添加前后指针指向的元素。这是原始数组和反转数组中相应元素的总和。
- 前指针加 1,后指针减 1。
- 循环结束后,返回得到的最大和。
下面是上述方法的实现
C++
// C++ program for the above approach
#include
using namespace std;
// Function to find the maximum
// sum obtained by adding the
// elements at the same index of
// the original array and of
// the reversed array
int maximumSum(int arr[], int n)
{
// Creating i as front pointer
// and j as rear pointer
int i = 0, j = n - 1;
int max = INT_MIN;
while (i <= j) {
if (max < arr[i] + arr[j])
max = arr[i] + arr[j];
i++;
j--;
}
// Returning the maximum value
return max;
}
// Driver Code
int main()
{
int arr[] = { 1, 8, 9, 5, 4, 6 };
int n = sizeof(arr) / sizeof(arr[0]);
cout << maximumSum(arr, n);
return 0;
}
Java
// Java program for the above approach
import java.util.*;
public class GFG {
// Function to find the maximum
// sum obtained by adding the
// elements at the same index of
// the original array and of
// the reversed array
static int maximumSum(int []arr, int n)
{
// Creating i as front pointer
// and j as rear pointer
int i = 0, j = n - 1;
int max = Integer.MIN_VALUE;
while (i <= j) {
if (max < arr[i] + arr[j])
max = arr[i] + arr[j];
i++;
j--;
}
// Returning the maximum value
return max;
}
// Driver Code
public static void main(String args[])
{
int []arr = { 1, 8, 9, 5, 4, 6 };
int n = arr.length;
System.out.println(maximumSum(arr, n));
}
}
// This code is contributed by Samim Hossain Mondal.
Python3
# python program for the above approach
INT_MIN = -2147483647 - 1
# Function to find the maximum
# sum obtained by adding the
# elements at the same index of
# the original array and of
# the reversed array
def maximumSum(arr, n):
# Creating i as front pointer
# and j as rear pointer
i = 0
j = n - 1
max = INT_MIN
while (i <= j):
if (max < arr[i] + arr[j]):
max = arr[i] + arr[j]
i += 1
j -= 1
# Returning the maximum value
return max
# Driver Code
if __name__ == "__main__":
arr = [1, 8, 9, 5, 4, 6]
n = len(arr)
print(maximumSum(arr, n))
# This code is contributed by rakeshsahni
C#
// C# program for the above approach
using System;
class GFG
{
// Function to find the maximum
// sum obtained by adding the
// elements at the same index of
// the original array and of
// the reversed array
static int maximumSum(int []arr, int n)
{
// Creating i as front pointer
// and j as rear pointer
int i = 0, j = n - 1;
int max = Int32.MinValue;
while (i <= j) {
if (max < arr[i] + arr[j])
max = arr[i] + arr[j];
i++;
j--;
}
// Returning the maximum value
return max;
}
// Driver Code
public static void Main()
{
int []arr = { 1, 8, 9, 5, 4, 6 };
int n = arr.Length;
Console.Write(maximumSum(arr, n));
}
}
// This code is contributed by Samim Hossain Mondal.
Javascript
输出:
14
时间复杂度: O(N)
辅助空间: O(1)。