使用Python将高斯滤波器应用于图像
高斯滤波器是一种低通滤波器,用于减少噪声(高频分量)和模糊图像区域。过滤器被实现为奇数大小的对称内核(矩阵的 DIP 版本),它通过感兴趣区域的每个像素以获得所需的效果。内核对于剧烈的颜色变化(边缘)并不困难,因为内核中心的像素比外围具有更多的权重。高斯滤波器可以被认为是高斯函数(数学)的近似。在本文中,我们将学习使用Python编程语言利用高斯滤波器来减少图像中的噪声的方法。
我们将使用以下图像进行演示:

一段windows资源管理器截图
应用高斯滤波器的过程
在对图像使用高斯滤波器的过程中,我们首先定义将用于消除图像的内核/矩阵的大小。大小通常是奇数,即可以在中心像素上计算整体结果。此外,内核是对称的,因此具有相同的行数和列数。内核内部的值由高斯函数计算,如下所示:
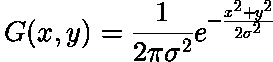
二维高斯函数
Where,
x → X coordinate value
y → Y coordinate value
???? → Mathematical Constant PI (value = 3.13)
σ → Standard Deviation
使用上述函数,可以通过为其提供适当的值来计算任何大小的高斯核。一个标准偏差为 1 的 3×3 高斯核近似(二维),如下所示
在Python中实现高斯核
我们将使用名为filter()的 PIL(Python成像库)函数通过预定义的高斯内核传递我们的整个图像。函数帮助页面如下:
Syntax: Filter(Kernel)
Takes in a kernel (predefined or custom) and each pixel of the image through it (Kernel Convolution).
Parameter: Filter Kernel
Return: Image Object
在以下示例中,我们将模糊上述图像。
Python3
# ImageFilter for using filter() function
from PIL import Image, ImageFilter
# Opening the image
# (R prefixed to string in order to deal with '\' in paths)
image = Image.open(r"IMAGE_PATH")
# Blurring image by sending the ImageFilter.
# GaussianBlur predefined kernel argument
image = image.filter(ImageFilter.GaussianBlur)
# Displaying the image
image.show()
Python3
from PIL import Image, ImageFilter
image = Image.open(r"FILE_PATH")
# Cropping the image
smol_image = image.crop((0, 0, 150, 150))
# Blurring on the cropped image
blurred_image = smol_image.filter(ImageFilter.GaussianBlur)
# Pasting the blurred image on the original image
image.paste(blurred_image, (0,0))
# Displaying the image
image.save('output.png')
输出:

图像模糊
解释:
首先,我们导入了 PIL 库的 Image 和 ImageFilter(用于使用filter() )模块。然后我们通过在路径IMAGE_PATH (用户定义)打开图像来创建一个图像对象。之后我们通过过滤器函数过滤图像,并提供ImageFilter.GaussianBlur (在 ImageFilter 模块中预定义)作为它的参数。 ImageFilter.GaussianBlur 的内核尺寸为 5×5。最后我们展示了图像。
Note: The size of kernel could be manipulated by passing as parameter (optional) the radius of the kernel. This changes the following line from.
image = image.filter(ImageFilter.GaussianBlur)
to
image = image.filter(ImageFilter.GaussianBlur(radius=x))
where x => blur radius (size of kernel in one direction, from the center pixel)
模糊图像中的一个小区域:
除了整个图像,它的某些部分也可以被选择性地模糊。这可以通过首先裁剪图像的所需区域,然后将其传递给 filter()函数。其输出(模糊的子图像)将粘贴在原始图像的顶部。这将为我们提供所需的输出。
其代码如下:
蟒蛇3
from PIL import Image, ImageFilter
image = Image.open(r"FILE_PATH")
# Cropping the image
smol_image = image.crop((0, 0, 150, 150))
# Blurring on the cropped image
blurred_image = smol_image.filter(ImageFilter.GaussianBlur)
# Pasting the blurred image on the original image
image.paste(blurred_image, (0,0))
# Displaying the image
image.save('output.png')
输出:

只有图像的左上角区域模糊