在给定的二叉搜索树中查找所有偶数路径
给定具有N个节点的二叉搜索树,任务是找到 所有从根开始到任何叶子结束并且总和为偶数的路径。
例子:
Input:
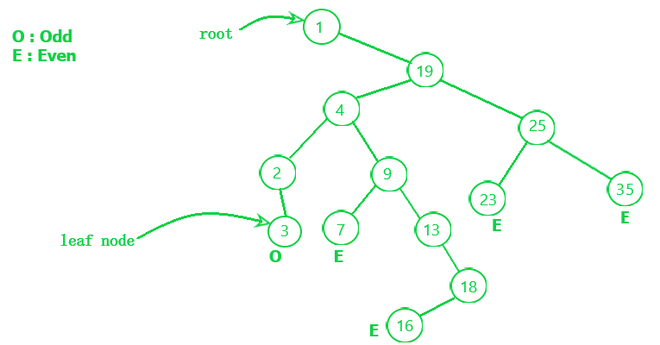
Img-Btree
Output:
Even sum Paths are:
1st) 1 -> 19 -> 4 -> 9 -> 7 = sum(40)
2nd) 1 -> 19 -> 4 -> 9 -> 13 -> 18 -> 16 = sum(80)
3rd) 1 -> 19 -> 25 -> 35 = sum(68)
4th) 1 -> 19 -> 25 -> 23 = sum(80)
Explanation: When we start traversing form root node to the leaf node then we have different path are (1, 19, 4, 9, 7), (1, 19, 4, 9, 13, 18, 16), (1, 19, 25, 35), (1, 19, 25, 23) and (1, 19, 4, 2, 3). And after finding the sum of every path we found that all are even. Here we found one path as odd which are 1 -> 19 -> 4 -> 2 -> 3 = sum(29).
Input: 2
/ \
1 3
Output: No path
Explanation: There is no path which has even sum.
方法:可以使用堆栈来解决问题,基于以下思想:
Traverse the whole tree and keep storing the path in the stack. When the leaf is reached check if the sum of the path is even or not.
按照下面提到的步骤来实施该方法:
- 取一个堆栈(比如path )来存储当前 path 。
- 递归遍历树并在每次迭代中:
- 存储该路径上所有节点的总和(例如sum )并将该节点数据也存储在路径中。
- 遍历左孩子。
- 遍历正确的孩子。
- 如果到达叶节点,则检查路径的总和是偶数还是奇数。
- 返回所有此类路径。
下面是更好理解的代码:
Java
// Java code to implement the approach
import java.io.*;
import java.lang.*;
import java.util.*;
class GFG {
static int count = 0;
// Structure of a tree node
static class Node {
Node left;
int val;
Node right;
Node(int val)
{
this.right = right;
this.val = val;
this.left = left;
}
}
// Function to find the paths
static void cntEvenPath(Node root, int sum,
Stack path)
{
// Add the value of node in sum
sum += root.val;
// Keep storing the node element
// in the path
path.push(root.val);
// If node has no left and right child
// and also the sum is even
// then return the path
if ((sum & 1) == 0
&& (root.left == null
&& root.right == null)) {
count++;
System.out.println("Sum Of"
+ path + " = "
+ sum);
return;
}
// Check left child
if (root.left != null) {
cntEvenPath(root.left, sum, path);
path.pop();
}
// Check right child
if (root.right != null) {
cntEvenPath(root.right, sum, path);
path.pop();
}
}
// Driver code
public static void main(String[] args)
{
// Build a tree
Node root = new Node(1);
root.right = new Node(19);
root.right.right = new Node(25);
root.right.left = new Node(4);
root.right.left.left = new Node(2);
root.right.left.right = new Node(9);
root.right.right.left = new Node(23);
root.right.right.right
= new Node(35);
root.right.left.left.right
= new Node(3);
root.right.left.right.left
= new Node(7);
root.right.left.right.right
= new Node(13);
root.right.left.right.right.right
= new Node(18);
root.right.left.right.right.right.left
= new Node(16);
System.out.println();
// Stack to store path
Stack path = new Stack<>();
cntEvenPath(root, 0, path);
if (count == 0)
System.out.println("No path");
}
}
Sum Of[1, 19, 4, 9, 7] = 40
Sum Of[1, 19, 4, 9, 13, 18, 16] = 80
Sum Of[1, 19, 25, 23] = 68
Sum Of[1, 19, 25, 35] = 80
时间复杂度: O(N)
辅助空间: O(N)