给定一个由N 个整数组成的数组arr[] ,任务是使用慢排序按升序对给定数组进行排序。
例子:
Input: arr[] = {6, 8, 9, 4, 12, 1}
Output: 1 4 6 8 9 12
Input: arr[] = {5, 4, 3, 2, 1}
Output: 1 2 3 4 5
方法:与归并排序一样,慢排序是一种分而治之的算法。它将输入数组分成两半,称自己为两半,然后比较两半的最大元素。它将子数组的最大元素存储在子数组的顶部位置,然后递归调用没有最大元素的子数组。请按照以下步骤解决问题:
慢排序(arr[], l, r):
- 如果r >= l ,请执行以下步骤:
- 找到数组的中间值m = (l + r) / 2 。
- 递归调用函数SlowSort 来查找前半个元素的最大值: SlowSort(arr, l, m)
- 递归调用函数SlowSort 求后半元素的最大值: SlowSort(arr, m + 1, r)
- 最后将上述函数调用返回的两个最大值中的最大值存储为arr[r] = max(arr[m], arr[r])
- 递归调用函数SlowSort,没有上一步得到的最大值: SlowSort(arr, l, r-1)
下图显示了完整的慢排序过程。例如,数组{9, 6, 8, 4, 1, 3, 7, 2} 。从图中可以看出,数组被递归分成两半,直到大小变为1。一旦大小变为1,比较过程就开始了。
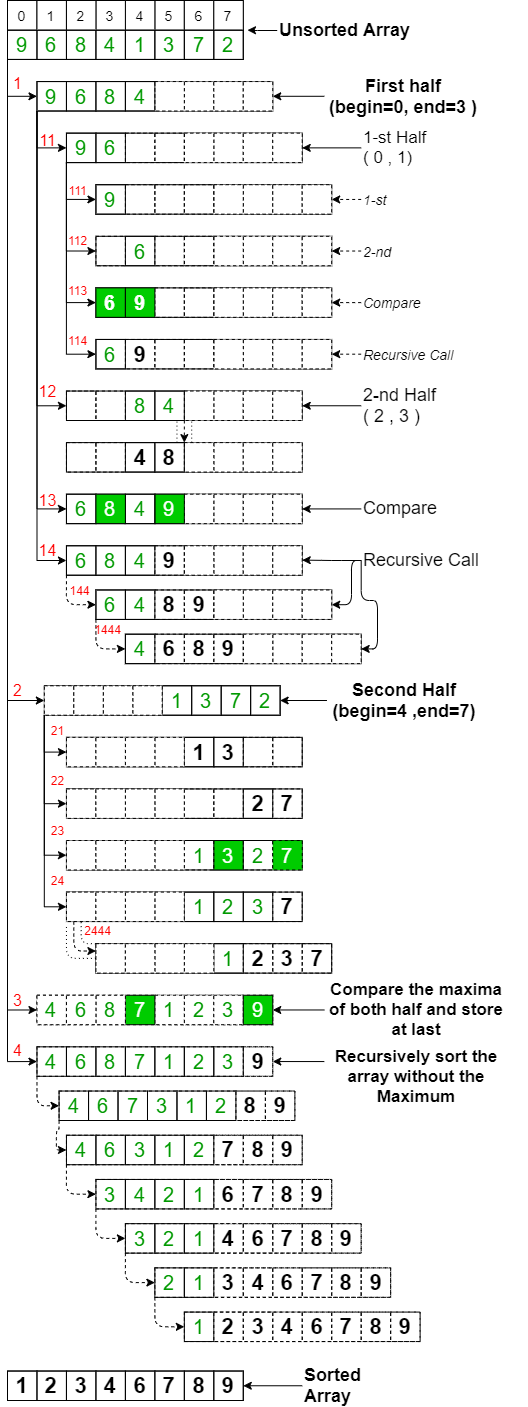
慢排序
下面是上述方法的实现:
C++
// C++ program for the above approach
#include
using namespace std;
// Function to swap two elements
void swap(int* xp, int* yp)
{
int temp = *xp;
*xp = *yp;
*yp = temp;
}
// Function to sort the array using
// the Slow sort
void slow_sort(int A[], int i, int j)
{
// Recursion break condition
if (i >= j)
return;
// Store the middle value
int m = (i + j) / 2;
// Recursively call with the
// left half
slow_sort(A, i, m);
// Recursively call with the
// right half
slow_sort(A, m + 1, j);
// Swap if the first element is
// lower than second
if (A[j] < A[m]) {
swap(&A[j], &A[m]);
}
// Recursively call with the
// array excluding the maximum
// element
slow_sort(A, i, j - 1);
}
// Function to print the array
void printArray(int arr[], int size)
{
int i;
for (i = 0; i < size; i++)
cout << arr[i] << " ";
cout << endl;
}
// Driver Code
int main()
{
// Given Input
int arr[] = { 6, 8, 9, 4, 12, 1 };
int n = sizeof(arr) / sizeof(arr[0]);
// Function Call
slow_sort(arr, 0, n - 1);
// Print the sorted array
printArray(arr, n);
return 0;
}
Java
// Java program for the above approach
class GFG{
// Function to sort the array using
// the Slow sort
static void slow_sort(int A[], int i, int j)
{
// Recursion break condition
if (i >= j)
return;
// Store the middle value
int m = (i + j) / 2;
// Recursively call with the
// left half
slow_sort(A, i, m);
// Recursively call with the
// right half
slow_sort(A, m + 1, j);
// Swap if the first element is
// lower than second
if (A[j] < A[m])
{
int temp = A[j];
A[j] = A[m];
A[m] = temp;
}
// Recursively call with the
// array excluding the maximum
// element
slow_sort(A, i, j - 1);
}
// Function to print the array
static void printArray(int arr[], int size)
{
int i;
for(i = 0; i < size; i++)
System.out.print(arr[i] + " ");
System.out.println();
}
// Driver code
public static void main(String[] args)
{
int arr[] = { 6, 8, 9, 4, 12, 1 };
int n = arr.length;
// Function Call
slow_sort(arr, 0, n - 1);
// Print the sorted array
printArray(arr, n);
}
}
// This code is contributed by abhinavjain194
Python3
# Python3 program for the above approach
# Function to sort the array using
# the Slow sort
def slow_sort(A, i, j):
# Recursion break condition
if (i >= j):
return
# Store the middle value
m = (i + j) // 2
# Recursively call with the
# left half
slow_sort(A, i, m)
# Recursively call with the
# right half
slow_sort(A, m + 1, j)
# Swap if the first element is
# lower than second
if (A[j] < A[m]):
temp = A[m]
A[m] = A[j]
A[j] = temp
# Recursively call with the
# array excluding the maximum
# element
slow_sort(A, i, j - 1)
# Function to print the array
def printArray(arr, size):
for i in range(size):
print(arr[i], end = " ")
# Driver Code
if __name__ == '__main__':
arr = [ 6, 8, 9, 4, 12, 1 ]
n = len(arr)
# Function Call
slow_sort(arr, 0, n - 1)
# Print the sorted array
printArray(arr, n)
# This code is contributed by SoumikMondal
C#
// C# implementation of the approach
using System;
class GFG
{
// Function to sort the array using
// the Slow sort
static void slow_sort(int[] A, int i, int j)
{
// Recursion break condition
if (i >= j)
return;
// Store the middle value
int m = (i + j) / 2;
// Recursively call with the
// left half
slow_sort(A, i, m);
// Recursively call with the
// right half
slow_sort(A, m + 1, j);
// Swap if the first element is
// lower than second
if (A[j] < A[m])
{
int temp = A[j];
A[j] = A[m];
A[m] = temp;
}
// Recursively call with the
// array excluding the maximum
// element
slow_sort(A, i, j - 1);
}
// Function to print the array
static void printArray(int[] arr, int size)
{
int i;
for(i = 0; i < size; i++)
Console.Write(arr[i] + " ");
Console.WriteLine();
}
// Driver code
public static void Main()
{
int[] arr = { 6, 8, 9, 4, 12, 1 };
int n = arr.Length;
// Function Call
slow_sort(arr, 0, n - 1);
// Print the sorted array
printArray(arr, n);
}
}
// this code is contributed by sanjoy_62.
Javascript
输出:
1 4 6 8 9 12
最佳案例时间复杂度: , 其中 e > 0
平均案例时间复杂度:
辅助空间: O(1)
如果您希望与专家一起参加现场课程,请参阅DSA 现场工作专业课程和学生竞争性编程现场课程。