检查是否有可能在无限矩阵中以偶数步到达目的地
给定一个包含无限行和列的矩阵[][]中的源和目的地,任务是找出是否有可能以偶数步从源到达目的地。此外,您只能向上、向下、向左和向右移动。
例子:
Input: Source = {2, 1}, Destination = {1, 4}
Output: Yes
Input: Source = {2, 2}, Destination = {1, 4}
Output: No
观察:
观察结果是,如果在最短路径上到达目的地所需的步数是偶数,那么在所有其他路线上到达它所需的步数将始终是偶数。此外,可以有无数种方法到达目标点。下面给出了在 4 x 5 矩阵中从 (1, 2) 到达 (4, 1) 的一些路径:
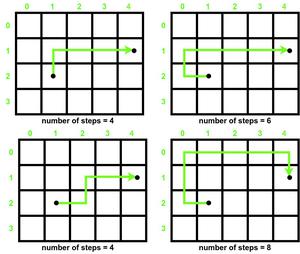
所需的最少步数 =4
因此,我们的问题减少了在矩阵中找到从源到达目的地所需的最小步数,这可以通过简单地将X坐标和Y坐标之间的差值的绝对值之和轻松计算出来。
下面是上述方法的实现:
C++
// C++ Program for the above approach
#include
using namespace std;
// Function to check destination can be
// reached from source in even number of
// steps
void IsEvenPath(int Source[], int Destination[])
{
// Coordinates differences
int x_dif = abs(Source[0] - Destination[0]);
int y_dif = abs(Source[1] - Destination[1]);
// minimum number of steps required
int minsteps = x_dif + y_dif;
// Minsteps is even
if (minsteps % 2 == 0)
cout << "Yes";
// Minsteps is odd
else
cout << "No";
}
// Driver Code
int main()
{
// Given Input
int Source[] = { 2, 1 };
int Destination[] = { 1, 4 };
// Function Call
IsEvenPath(Source, Destination);
return 0;
}
Java
// Java program for the above approach
import java.lang.*;
import java.util.*;
class GFG{
// Function to check destination can be
// reached from source in even number of
// steps
static void IsEvenPath(int Source[], int Destination[])
{
// Coordinates differences
int x_dif = Math.abs(Source[0] - Destination[0]);
int y_dif = Math.abs(Source[1] - Destination[1]);
// Minimum number of steps required
int minsteps = x_dif + y_dif;
// Minsteps is even
if (minsteps % 2 == 0)
System.out.println("Yes");
// Minsteps is odd
else
System.out.println("No");
}
// Driver code
public static void main(String[] args)
{
// Given Input
int Source[] = { 2, 1 };
int Destination[] = { 1, 4 };
// Function Call
IsEvenPath(Source, Destination);
}
}
// This code is contributed by sanjoy_62
Python3
# Python3 program for the above approach
# Function to check destination can be
# reached from source in even number of
# steps
def IsEvenPath(Source, Destination):
# Coordinates differences
x_dif = abs(Source[0] - Destination[0])
y_dif = abs(Source[1] - Destination[1])
# Minimum number of steps required
minsteps = x_dif + y_dif
# Minsteps is even
if (minsteps % 2 == 0):
print("Yes")
# Minsteps is odd
else:
print("No")
# Driver Code
if __name__ == '__main__':
# Given Input
Source = [ 2, 1 ]
Destination = [ 1, 4 ]
# Function Call
IsEvenPath(Source, Destination)
# This code is contributed by mohit kumar 29
C#
// C# program for the above approach
using System;
class GFG{
// Function to check destination can be
// reached from source in even number of
// steps
static void IsEvenPath(int[] Source, int[] Destination)
{
// Coordinates differences
int x_dif = Math.Abs(Source[0] - Destination[0]);
int y_dif = Math.Abs(Source[1] - Destination[1]);
// Minimum number of steps required
int minsteps = x_dif + y_dif;
// Minsteps is even
if (minsteps % 2 == 0)
Console.WriteLine("Yes");
// Minsteps is odd
else
Console.WriteLine("No");
}
// Driver code
public static void Main(string[] args)
{
// Given Input
int[] Source = { 2, 1 };
int[] Destination = { 1, 4 };
// Function Call
IsEvenPath(Source, Destination);
}
}
// This code is contributed by code_hunt.
Javascript
输出
Yes
时间复杂度: O(1)
辅助空间: O(1)