完整图的最大可能边不相交生成树
给出一个包含 N 个顶点的完整图。任务是找出可能的边不相交生成树的最大数量。
边不相交生成树是一种生成树,其中集合中没有两棵树有共同的边。
例子:
Input : N = 4
Output : 2
Input : N = 5
Output : 2
从具有 N 个顶点的完整图中,可能的边不相交生成树的最大数量可以给出为,
Max Edge-disjoint spanning tree = floor(N / 2)
让我们看一些例子:
示例 1 :
具有 4 个顶点的完整图
上图所有可能的边不相交生成树是:
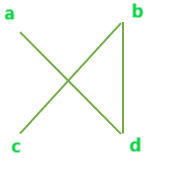
一种
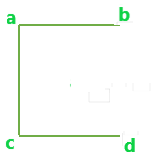
乙
示例 2 :
具有 5 个顶点的完整图
上图所有可能的边不相交生成树是:
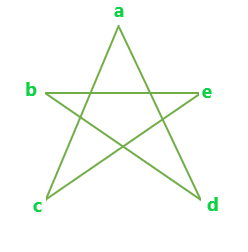
一种
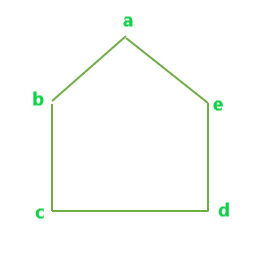
乙
下面是找到可能的边不相交生成树的最大数量的程序。
C++
// C++ program to find the maximum number of
// Edge-Disjoint Spanning tree possible
#include
using namespace std;
// Function to calculate max number of
// Edge-Disjoint Spanning tree possible
float edgeDisjoint(int n)
{
float result = 0;
result = floor(n / 2);
return result;
}
// Driver code
int main()
{
int n = 4;
cout << edgeDisjoint(n);
return 0;
}
Java
// Java program to find the maximum
// number of Edge-Disjoint Spanning
// tree possible
import java.io.*;
class GFG
{
// Function to calculate max number
// of Edge-Disjoint Spanning tree
// possible
static double edgeDisjoint(int n)
{
double result = 0;
result = Math.floor(n / 2);
return result;
}
// Driver Code
public static void main(String[] args)
{
int n = 4;
System.out.println((int)edgeDisjoint(n));
}
}
// This code is contributed
// by Naman_Garg
Python3
# Python 3 to find the maximum
# number of Edge-Disjoint
# Spanning tree possible
import math
# Function to calculate max
# number of Edge-Disjoint
# Spanning tree possible
def edgeDisjoint(n):
result = 0
result = math.floor(n / 2)
return result
# Driver Code
if __name__ == "__main__" :
n = 4
print(int(edgeDisjoint(n)))
# This Code is contributed
# by Naman_Garg
C#
// C# program to find the maximum number of
// Edge-Disjoint Spanning tree possible
using System;
class GFG
{
// Function to calculate max number of
// Edge-Disjoint Spanning tree possible
static double edgeDisjoint(double n)
{
double result = 0;
result = Math.Floor(n / 2);
return result;
}
// Driver Code
public static void Main()
{
int n = 4;
Console.Write(edgeDisjoint(n));
}
}
// This code is contributed
// by Sanjit_Prasad
PHP
Javascript
输出:
2