使用 Pandas 进行数据分析
Pandas是最流行的用于数据分析的Python库。它提供高度优化的性能,后端源代码完全用C或Python编写。
We can analyze data in pandas with:
SeriesDataFrames
系列:
Series是 pandas 中定义的一维(1-D)数组,可用于存储任何数据类型。
代码 #1:创建系列
# Program to create series
# Import Panda Library
import pandas as pd
# Create series with Data, and Index
a = pd.Series(Data, index = Index)
在这里,数据可以是:
- 一个标量值,可以是 integerValue、字符串
- 可以是键值对的Python字典
- 一个Ndarray
注意:默认情况下,索引从 0、1、2、...(n-1) 开始,其中 n 是数据长度。代码 #2:当 Data 包含标量值时
# Program to Create series with scalar values
# Numeric data
Data =[1, 3, 4, 5, 6, 2, 9]
# Creating series with default index values
s = pd.Series(Data)
# predefined index values
Index =['a', 'b', 'c', 'd', 'e', 'f', 'g']
# Creating series with predefined index values
si = pd.Series(Data, Index)
输出:
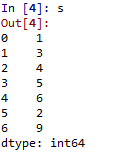
具有默认索引的标量数据
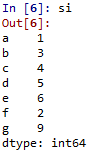
带索引的标量数据
代码#3:当数据包含字典时
# Program to Create Dictionary series
dictionary ={'a':1, 'b':2, 'c':3, 'd':4, 'e':5}
# Creating series of Dictionary type
sd = pd.Series(dictionary)
输出:
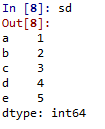
字典类型数据
代码 #4:当 Data 包含 Ndarray
# Program to Create ndarray series
# Defining 2darray
Data =[[2, 3, 4], [5, 6, 7]]
# Creating series of 2darray
snd = pd.Series(Data)
输出:
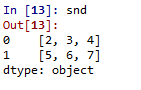
数据作为 Ndarray
数据框:
DataFrames是 pandas 中定义的二维(2-D)数据结构,由行和列组成。
代码 #1:创建 DataFrame
# Program to Create DataFrame
# Import Library
import pandas as pd
# Create DataFrame with Data
a = pd.DataFrame(Data)
在这里,数据可以是:
- 一本或多本词典
- 一个或多个系列
- 2D-numpy Ndarray
代码 #2:当数据是字典时
# Program to Create Data Frame with two dictionaries
# Define Dictionary 1
dict1 ={'a':1, 'b':2, 'c':3, 'd':4}
# Define Dictionary 2
dict2 ={'a':5, 'b':6, 'c':7, 'd':8, 'e':9}
# Define Data with dict1 and dict2
Data = {'first':dict1, 'second':dict2}
# Create DataFrame
df = pd.DataFrame(Data)
输出:
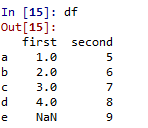
带有两个字典的 DataFrame
代码 #3:当数据是系列时
# Program to create Dataframe of three series
import pandas as pd
# Define series 1
s1 = pd.Series([1, 3, 4, 5, 6, 2, 9])
# Define series 2
s2 = pd.Series([1.1, 3.5, 4.7, 5.8, 2.9, 9.3])
# Define series 3
s3 = pd.Series(['a', 'b', 'c', 'd', 'e'])
# Define Data
Data ={'first':s1, 'second':s2, 'third':s3}
# Create DataFrame
dfseries = pd.DataFrame(Data)
输出:
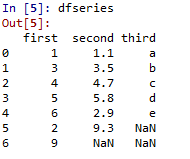
三个系列的DataFrame
代码 #4:当 Data 是 2D-numpy ndarray
注意:在创建二维数组的 DataFrame 时必须保持一个约束——二维数组的维度必须相同。
# Program to create DataFrame from 2D array
# Import Library
import pandas as pd
# Define 2d array 1
d1 =[[2, 3, 4], [5, 6, 7]]
# Define 2d array 2
d2 =[[2, 4, 8], [1, 3, 9]]
# Define Data
Data ={'first': d1, 'second': d2}
# Create DataFrame
df2d = pd.DataFrame(Data)
输出:
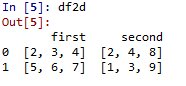
带有 2d ndarray 的 DataFrame