如何在 MATLAB 中创建下拉菜单
在本文中,我们将了解下拉菜单组件、如何创建它以及它的重要属性是什么。然后我们将创建一个简单的 Matlab Gui 应用程序。
在 MATLAB 中创建下拉菜单
下拉菜单是一个 UI 组件,可帮助用户选择给定选项之一或键入文本。 Matlab 提供了一个名为uidropdown的函数来创建下拉菜单的实例。
创建下拉菜单的三种方式如下:
- dropdownObject = uidropdown; (不带任何参数)
- dropdownObject = uidropdown(parent);
- dropdownObject = uidropdown(parent, NameOfProperty, Value ...);
特性
此 UI 组件的一些重要属性如下:
- 项目:下拉菜单中可用的选项是使用此属性设置的。
- 值:访问或更改组件当前选择的值。
- Text: text 属性用于控制标签的内容。默认值为“标签”。
- 解释器:此属性允许我们使用不同的解释器来解释标签的文本。就像我们可以使用 Latex 代码编写方程式或使用 HTML 格式化文本并将解释器设置为“latex”或“HTML”。默认为“无”。
- HorizontalAlignment:控制标签组件内文本的水平对齐方式。默认为“左”。
- VerticalAlignment:控制组件内的垂直对齐方式。默认值为“中心”。
- 自动换行:将文本换行以适应组件的宽度。默认为“关闭”。
- FontName:更改文本的字体。
- FontSize:控制字体大小。
- 字体粗细:控制文本的粗细。
- FontAngle:控制字体角度。
- 字体颜色:字体的颜色。
- BackgroundColor:改变标签的背景颜色。
- 可见性:此属性控制组件的可见性。默认为“开”。
- 启用:启用或禁用外观。默认为“开”。
- TootTip:用于指导组件用途的文本。默认为“。
- 位置:一个 4 值列表,用于控制组件在父窗口中的位置和组件的大小。
让我们看一些演示语法使用的示例。
下拉菜单()
此函数不需要任何参数,但由于每个组件都必须在父窗口内,因此 Matlab 创建一个图形并将组件分配给它。
例子:
Matlab
% MATLAB code for uidropdown()
% create a uidropdown object using the api
dropdownObject = uidropdown;
Matlab
% MATLAB code for uidropdown(parent)
% create a figure window
fig = uifigure;
% create a drop down menu and pass the fig as parent
dropdownObject = uidropdown(fig);
Matlab
% MATLAB code for uidropdown(parent, NameOfProperty, Value ...)
% create a figure window
fig = uifigure;
% create a drop down menu and pass the fig as
% parent as well as set the properties.
dropdownObject = uidropdown(fig, 'Items', {'Mango','Guava','Orange','Apple'},
'Value', 'Apple');
Matlab
% MATLAB code for create a figure
fig = uifigure('Position', [100 100 300 275]);
% create a label
label = uilabel(fig, 'Position', [100 120, 120 40],...
'FontSize', 30,...
'FontWeight', 'bold');
% create a dropdownObject and pass the figure as parent
dropdownObject = uidropdown(fig,...
'Items', {'Mango','Guava','Orange','Apple'},...
'Value', 'Apple',...
'Editable', 'on',...
'Position', [84 204 100 20],...
'ValueChangedFcn', @(dd, event) fruitSelected(dd, label));
% function to call when option is selected (callback)
function fruitSelected(dd, label)
% read the value from the dropdown
val = dd.Value;
% set the text property of label
label.Text = val;
end
输出:
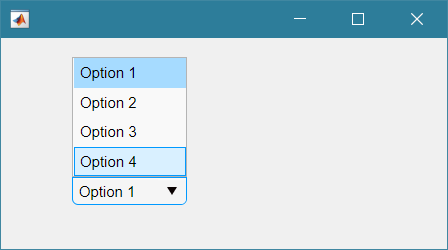
一个下拉菜单
默认情况下,组件将项目保存为“选项 1”、“选项 2”等。
uidropdown(父)
方法uidropdown还接受一个可选的父窗口来保存组件。
例子:
MATLAB
% MATLAB code for uidropdown(parent)
% create a figure window
fig = uifigure;
% create a drop down menu and pass the fig as parent
dropdownObject = uidropdown(fig);
输出:
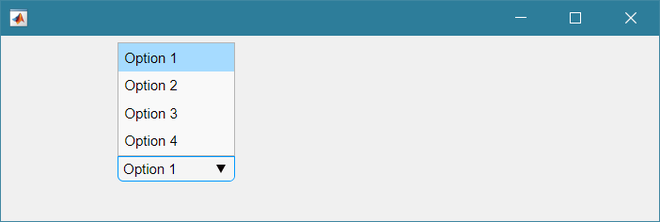
使用自定义窗口作为父级的下拉组件
uidropdown(parent, NameOfProperty, Value ...)
Matlab 还为我们提供了在实例化时通过传递属性名称及其值来设置下拉菜单属性的选项。
例子:
MATLAB
% MATLAB code for uidropdown(parent, NameOfProperty, Value ...)
% create a figure window
fig = uifigure;
% create a drop down menu and pass the fig as
% parent as well as set the properties.
dropdownObject = uidropdown(fig, 'Items', {'Mango','Guava','Orange','Apple'},
'Value', 'Apple');
输出:
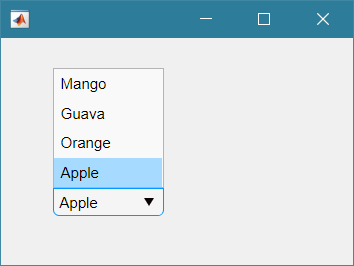
带有自定义选项的下拉菜单
在方法 uidropdown 中,设置了 'Items' 属性,它是组件将显示的所有选项。 'value' 属性描述了默认选择的选项。
现在我们创建一个应用程序,在该应用程序中,从下拉菜单中选择一个选项将显示在具有更大字体的标签中。
例子:
MATLAB
% MATLAB code for create a figure
fig = uifigure('Position', [100 100 300 275]);
% create a label
label = uilabel(fig, 'Position', [100 120, 120 40],...
'FontSize', 30,...
'FontWeight', 'bold');
% create a dropdownObject and pass the figure as parent
dropdownObject = uidropdown(fig,...
'Items', {'Mango','Guava','Orange','Apple'},...
'Value', 'Apple',...
'Editable', 'on',...
'Position', [84 204 100 20],...
'ValueChangedFcn', @(dd, event) fruitSelected(dd, label));
% function to call when option is selected (callback)
function fruitSelected(dd, label)
% read the value from the dropdown
val = dd.Value;
% set the text property of label
label.Text = val;
end
输出:
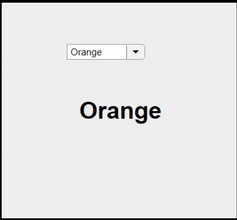
带有字体属性的下拉菜单
我们首先创建一个图形来保存所有 UI 组件,并设置它的 Position 属性来控制它的位置和大小。然后创建一个字体大小为 30 且字体粗细设置为粗体的标签。我们创建了一个下拉菜单并将其属性设置为可编辑,使用户能够输入一个值而不是选择一个选项。 'ValueChangedFcn' 属性设置一旦下拉菜单的值发生变化将调用的函数回调。