对话框是一种出现在窗口或屏幕上的小部件,它包含任何关键信息或可以要求任何决定。当弹出对话框时,所有其他功能都将被禁用,直到您关闭对话框或提供答案。我们对不同类型的条件使用对话框,例如警报通知、显示不同选项的简单通知,或者我们也可以制作一个对话框,它可以用作显示对话框的选项卡。
flutter的对话框类型
- 警报对话框
- 简单对话框
- 显示对话框
警报对话框:
警报对话框告诉用户任何需要识别的情况。警报对话框包含一个可选的标题和一个可选的操作列表。我们有不同的行动作为我们的要求。有时内容与屏幕尺寸相比太大,因此为了解决这个问题,我们可能不得不使用扩展类。
特性:
- 标题:始终建议使我们的对话框标题尽可能短。这对用户来说很容易理解。
- 动作:用于显示必须执行的动作的内容。
- 内容: alertDialog小部件的主体由内容定义。
- Shape:用于定义我们对话框的形状,无论是圆形、曲线等等。
构造函数:
AlertDialog(
{
Key key,
Widget title,
EdgeInsetsGeometry titlePadding,
TextStyle titleTextStyle,
Widget content,
EdgeInsetsGeometry contentPadding: const EdgeInsets.fromLTRB(24.0, 20.0, 24.0, 24.0),
TextStyle contentTextStyle,
List actions,
EdgeInsetsGeometry actionsPadding: EdgeInsets.zero,
VerticalDirection actionsOverflowDirection,
double actionsOverflowButtonSpacing,
EdgeInsetsGeometry buttonPadding,
Color backgroundColor,
double elevation,
String semanticLabel,
EdgeInsets insetPadding: _defaultInsetPadding,
Clip clipBehavior: Clip.none,
ShapeBorder shape,
bool scrollable: false
}
)
这是用于创建对话框的片段代码。
Dart
AlertDialog(
title: Text('Welcome'), // To display the title it is optional
content: Text('GeeksforGeeks'), // Message which will be pop up on the screen
// Action widget which will provide the user to acknowledge the choice
actions: [
FlatButton( // FlatButton widget is used to make a text to work like a button
textColor: Colors.black,
onPressed: () {}, // function used to perform after pressing the button
child: Text('CANCEL'),
),
FlatButton(
textColor: Colors.black,
onPressed: () {},
child: Text('ACCEPT'),
),
],
),
Dart
SimpleDialog(
title:const Text('GeeksforGeeks'),
children: [
SimpleDialogOption(
onPressed: () { },
child:const Text('Option 1'),
),
SimpleDialogOption(
onPressed: () { },
child: const Text('Option 2'),
),
],
),
Dart
showDialog(
context: context,
builder: (BuildContext context) {
return Expanded(
child: AlertDialog(
title: Text('Welcome'),
content: Text('GeeksforGeeks'),
actions: [
FlatButton(
textColor: Colors.black,
onPressed: () {},
child: Text('CANCEL'),
),
FlatButton(
textColor: Colors.black,
onPressed: () {},
child: Text('ACCEPT'),
),
],
),
);
},
);
输出:
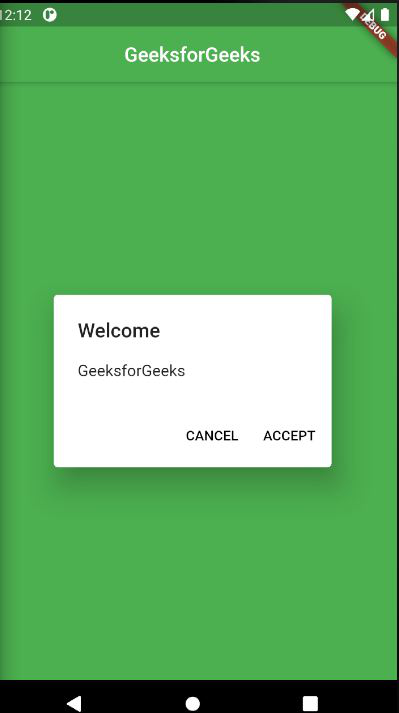
警报对话框
简单对话框:
一个简单的对话框允许用户从不同的选项中进行选择。它包含可选的标题,并显示在选项上方。我们也可以使用填充来显示选项。填充用于使小部件更灵活。
特性:
- 标题:始终建议使我们的对话框标题尽可能短。这对用户来说很容易理解。
- Shape:用于定义我们对话框的形状,无论是圆形、曲线等等。
- backgroundcolor:用于设置我们对话框的背景颜色。
- TextStyle:用于改变我们文本的样式。
构造函数:
const SimpleDialog(
{
Key key,
Widget title,
EdgeInsetsGeometry titlePadding: const EdgeInsets.fromLTRB(24.0, 24.0, 24.0, 0.0),
TextStyle titleTextStyle,
List children,
EdgeInsetsGeometry contentPadding: const EdgeInsets.fromLTRB(0.0, 12.0, 0.0, 16.0),
Color backgroundColor,
double elevation,
String semanticLabel,
ShapeBorder shape
}
)
这是简单对话框的片段代码
Dart
SimpleDialog(
title:const Text('GeeksforGeeks'),
children: [
SimpleDialogOption(
onPressed: () { },
child:const Text('Option 1'),
),
SimpleDialogOption(
onPressed: () { },
child: const Text('Option 2'),
),
],
),
输出:
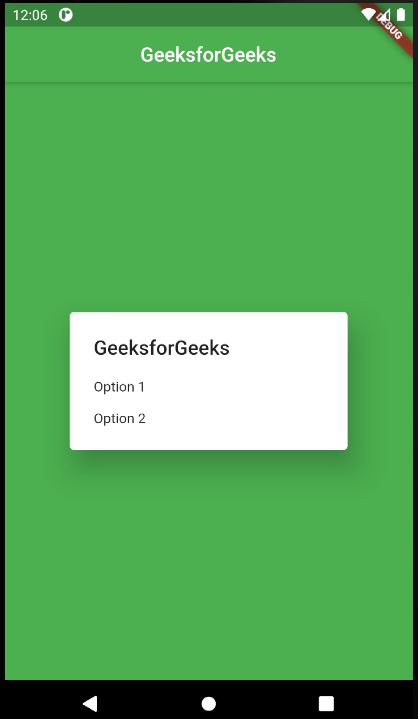
简单对话框
显示对话框:
它基本上用于更改我们应用程序的当前屏幕以显示对话框弹出窗口。您必须在弹出对话框之前调用。它退出当前动画并呈现新的屏幕动画。当我们想要显示一个会弹出任何类型对话框的选项卡时,我们使用这个对话框,或者我们创建一个前端选项卡来显示后台进程。
特性:
- Builder:它返回子对象而不是创建子参数。
- Barriercolor:它定义了使对话框中的所有内容变暗的模式屏障颜色。
- useSafeArea:确保对话框只使用屏幕的安全区域,不与屏幕区域重叠。
构造函数
Future showDialog (
{
@required BuildContext context,
WidgetBuilder builder,
bool barrierDismissible: true,
Color barrierColor,
bool useSafeArea: true,
bool useRootNavigator: true,
RouteSettings routeSettings,
@Deprecated(It returns the child from the closure provided to the builder class ) Widget child
}
)
这是showDialog函数的片段。
Dart
showDialog(
context: context,
builder: (BuildContext context) {
return Expanded(
child: AlertDialog(
title: Text('Welcome'),
content: Text('GeeksforGeeks'),
actions: [
FlatButton(
textColor: Colors.black,
onPressed: () {},
child: Text('CANCEL'),
),
FlatButton(
textColor: Colors.black,
onPressed: () {},
child: Text('ACCEPT'),
),
],
),
);
},
);
主要区别:
警报对话框
- 用于显示任何类型的警报通知。
- 我们可以选择对警报弹出窗口做出反应,例如接受按钮或拒绝按钮。
简单对话框
- 用于将简单选项显示为对话框。
- 有不同的选项可以根据它选择和执行功能。该选项可以很简单,例如在不同的电子邮件之间进行选择。
显示对话框
- 用于创建一个会弹出对话框的选项。
- 通过使用它,我们可以弹出不同类型的对话框,例如alertDialog 、 SimpleDialog作为子小部件。
想要一个更快节奏和更具竞争力的环境来学习 Android 的基础知识吗?
单击此处前往由我们的专家精心策划的指南,旨在让您立即做好行业准备!