查找第一位和最后一位数字总和的Python程序
给定一个正整数 N(至少包含两位数字)。任务是编写一个Python程序,将给定数字 N 的第一位和最后一位相加。
例子:
Input: N = 1247
Output: 8
Explanation: First digit is 1 and Last digit is 7. So, addition of these two (1 + 7) is equal to 8.
Input: N = 73
Output: 10
方法一:字符串实现
- 以字符串的形式输入输入或在字符串中对给定输入进行类型转换。
- 现在选择 String 的第 0 个索引并将其类型转换为 Integer 并将其存储在变量中。
- 与 -1st 索引相同,也存储在另一个变量中。
- 现在添加这两个变量和
- 将它们打印为输出。
注意:我们可以使用字符串[0]访问 String 的第一个元素,使用字符串[-1]访问 String 的最后一个元素。
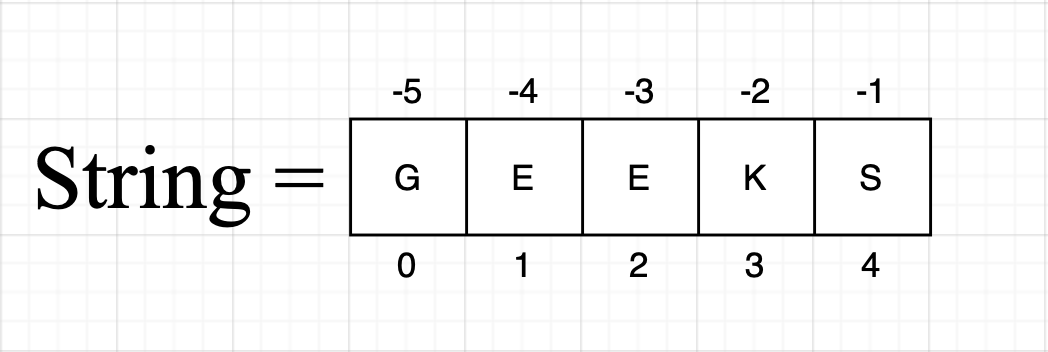
字符串表示
Python3
# We have a number
number = 1247
# We are type casting it in string
number = str(number)
# Storing first and last digit in a variable
# after type casting into Integer.
first_digit = int(number[0])
last_digit = int(number[-1])
# Adding these two variables
addition = first_digit + last_digit
# Display our output
print('Addition of first and last digit of the number is',
addition)
Python3
# We have a number.
number = 1247
# Assigning last digit of the number in res
# variable.
res = number % 10
# Now, continue a loop until
# the number becomes less than 9.
while number > 9:
# integer division of the number and reassigning
# it.
number = number // 10
# Here, our number only contain one digit.
# So, add this number in res variable.
res += number
# Now, display our output
print('Addition of first and last digit of number is', res)
输出:
Addition of first and last digit of the number is 8
方法二:用整数求解
- 我们给出了一个正整数。
- 除以 10 后,将余数存储在结果变量中。
- 继续循环,直到数字小于 9。
- 每次在循环中,将数字除以 10(整数除法)。
- 循环结束后。
- 在结果变量中添加数字。
- 将结果变量显示为输出。
注意:每当我们将任何数字除以 10 时,我们都会得到最后一位作为余数。如果我们将任何数字除以 100,我们将得到最后两位数作为余数。
蟒蛇3
# We have a number.
number = 1247
# Assigning last digit of the number in res
# variable.
res = number % 10
# Now, continue a loop until
# the number becomes less than 9.
while number > 9:
# integer division of the number and reassigning
# it.
number = number // 10
# Here, our number only contain one digit.
# So, add this number in res variable.
res += number
# Now, display our output
print('Addition of first and last digit of number is', res)
输出:
Addition of first and last digit of the number is 8
时间复杂度: O(n),其中 n 是给定数字中有多少位数字。