Java中的关联、组合和聚合
关联是通过它们的对象建立的两个独立类之间的关系。关联可以是一对一、一对多、多对一、多对多。在面向对象的编程中,一个对象与另一个对象通信以使用该对象提供的功能和服务。组合和聚合是关联的两种形式。
例子:
Java
// Java Program to illustrate the
// Concept of Association
// Importing required classes
import java.io.*;
// Class 1
// Bank class
class Bank {
// Attributes of bank
private String name;
// Constructor of this class
Bank(String name)
{
// this keyword refers to current instance itself
this.name = name;
}
// Method of Bank class
public String getBankName()
{
// Returning name of bank
return this.name;
}
}
// Class 2
// Employee class
class Employee {
// Attributes of employee
private String name;
// Employee name
Employee(String name)
{
// This keyword refers to current instance itself
this.name = name;
}
// Method of Employee class
public String getEmployeeName()
{
// returning the name of employee
return this.name;
}
}
// Class 3
// Association between both the
// classes in main method
class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating objects of bank and Employee class
Bank bank = new Bank("ICICI");
Employee emp = new Employee("Ridhi");
// Print and display name and
// corresponding bank of employee
System.out.println(emp.getEmployeeName()
+ " is employee of "
+ bank.getBankName());
}
}
Java
// Java program to illustrate Concept of Aggregation
// Importing required classes
import java.io.*;
import java.util.*;
// Class 1
// Student class
class Student {
// Attributes of student
String name;
int id;
String dept;
// Constructor of student class
Student(String name, int id, String dept)
{
// This keyword refers to current instance itself
this.name = name;
this.id = id;
this.dept = dept;
}
}
// Class 2
// Department class contains list of student objects
// It is associated with student class through its Objects
class Department {
// Attributes of Department class
String name;
private List students;
Department(String name, List students)
{
// this keyword refers to current instance itself
this.name = name;
this.students = students;
}
// Method of Department class
public List getStudents()
{
// Returning list of user defined type
// Student type
return students;
}
}
// Class 3
// Institute class contains list of Department
// Objects. It is associated with Department
// class through its Objects
class Institute {
// Attributes of Institute
String instituteName;
private List departments;
// Constructor of institute class
Institute(String instituteName,List departments)
{
// This keyword refers to current instance itself
this.instituteName = instituteName;
this.departments = departments;
}
// Method of Institute class
// Counting total students of all departments
// in a given institute
public int getTotalStudentsInInstitute()
{
int noOfStudents = 0;
List students;
for (Department dept : departments) {
students = dept.getStudents();
for (Student s : students) {
noOfStudents++;
}
}
return noOfStudents;
}
}
// Class 4
// main class
class GFG {
// main driver method
public static void main(String[] args)
{
// Creating object of Student class inside main()
Student s1 = new Student("Mia", 1, "CSE");
Student s2 = new Student("Priya", 2, "CSE");
Student s3 = new Student("John", 1, "EE");
Student s4 = new Student("Rahul", 2, "EE");
// Creating a List of CSE Students
List cse_students = new ArrayList();
// Adding CSE students
cse_students.add(s1);
cse_students.add(s2);
// Creating a List of EE Students
List ee_students
= new ArrayList();
// Adding EE students
ee_students.add(s3);
ee_students.add(s4);
// Creating objects of EE and CSE class inside
// main()
Department CSE = new Department("CSE", cse_students);
Department EE = new Department("EE", ee_students);
List departments = new ArrayList();
departments.add(CSE);
departments.add(EE);
// Lastly creating an instance of Institute
Institute institute = new Institute("BITS", departments);
// Display message for better readability
System.out.print("Total students in institute: ");
// Calling method to get total number of students
// in institute and printing on console
System.out.print(institute.getTotalStudentsInInstitute());
}
}
Java
// Java program to illustrate
// the concept of Composition
// Importing required classes
import java.io.*;
import java.util.*;
// Class 1
// Book
class Book {
// Attributes of book
public String title;
public String author;
// Constructor of Book class
Book(String title, String author)
{
// This keyword refers to current instance itself
this.title = title;
this.author = author;
}
}
// Class 2
class Library {
// Reference to refer to list of books
private final List books;
// Library class contains list of books
Library(List books)
{
// Referring to same book as
// this keyword refers to same instance itself
this.books = books;
}
// Method
// To get total number of books in library
public List getTotalBooksInLibrary()
{
return books;
}
}
// Class 3
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating objects of Book class inside main()
// method Custom inputs
Book b1
= new Book("EffectiveJ Java", "Joshua Bloch");
Book b2
= new Book("Thinking in Java", "Bruce Eckel");
Book b3 = new Book("Java: The Complete Reference",
"Herbert Schildt");
// Creating the list which contains number of books
List books = new ArrayList();
// Adding books
// using add() method
books.add(b1);
books.add(b2);
books.add(b3);
Library library = new Library(books);
// Calling method to get total books in library
// and storing it in list of user0defined type -
// Books
List bks = library.getTotalBooksInLibrary();
// Iterating over books using for each loop
for (Book bk : bks) {
// Printing the title and author name of book on
// console
System.out.println("Title : " + bk.title
+ " and "
+ " Author : " + bk.author);
}
}
}
Java
// Java Program to Illustrate Difference between
// Aggregation and Composition
// Importing I/O classes
import java.io.*;
// Class 1
// Engine class which will
// be used by car. so 'Car'
// class will have a field
// of Engine type.
class Engine {
// Method to starting an engine
public void work()
{
// Print statement whenever this method is called
System.out.println(
"Engine of car has been started ");
}
}
// Class 2
// Engine class
final class Car {
// For a car to move,
// it needs to have an engine.
// Composition
private final Engine engine;
// Note: Uncommented part refers to Aggregation
// private Engine engine;
// Constructor of this class
Car(Engine engine)
{
// This keywords refers to same instance
this.engine = engine;
}
// Method
// Car start moving by starting engine
public void move()
{
// if(engine != null)
{
// Calling method for working of engine
engine.work();
// Print statement
System.out.println("Car is moving ");
}
}
}
// Class 3
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Making an engine by creating
// an instance of Engine class.
Engine engine = new Engine();
// Making a car with engine so we are
// passing a engine instance as an argument
// while creating instance of Car
Car car = new Car(engine);
// Making car to move by calling
// move() method inside main()
car.move();
}
}
Ridhi is employee of ICICI
输出说明:在上面的示例中,两个单独的类 Bank 和 Employee 通过它们的 Objects 关联。银行可以有很多员工,所以是一对多的关系。
聚合
这是一种特殊的协会形式,其中:
- 它代表 Has-A 的关系。
- 它是一种单向关联,即单向关系。例如,一个部门可以有学生,但反之亦然是不可能的,因此本质上是单向的。
- 在聚合中,两个条目都可以单独存在,这意味着结束一个实体不会影响另一个实体。
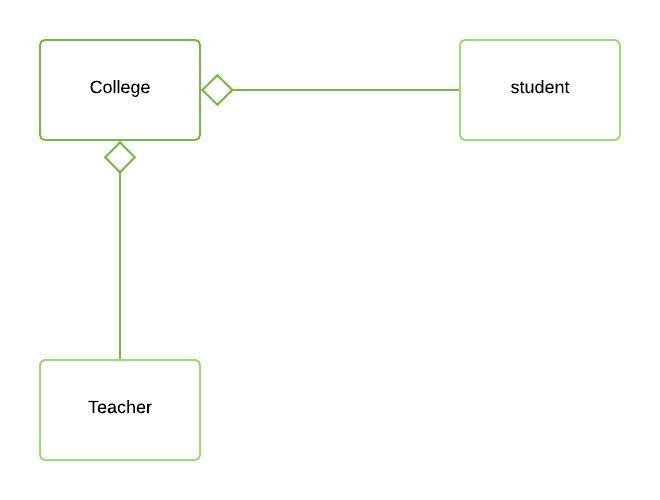
聚合
例子
Java
// Java program to illustrate Concept of Aggregation
// Importing required classes
import java.io.*;
import java.util.*;
// Class 1
// Student class
class Student {
// Attributes of student
String name;
int id;
String dept;
// Constructor of student class
Student(String name, int id, String dept)
{
// This keyword refers to current instance itself
this.name = name;
this.id = id;
this.dept = dept;
}
}
// Class 2
// Department class contains list of student objects
// It is associated with student class through its Objects
class Department {
// Attributes of Department class
String name;
private List students;
Department(String name, List students)
{
// this keyword refers to current instance itself
this.name = name;
this.students = students;
}
// Method of Department class
public List getStudents()
{
// Returning list of user defined type
// Student type
return students;
}
}
// Class 3
// Institute class contains list of Department
// Objects. It is associated with Department
// class through its Objects
class Institute {
// Attributes of Institute
String instituteName;
private List departments;
// Constructor of institute class
Institute(String instituteName,List departments)
{
// This keyword refers to current instance itself
this.instituteName = instituteName;
this.departments = departments;
}
// Method of Institute class
// Counting total students of all departments
// in a given institute
public int getTotalStudentsInInstitute()
{
int noOfStudents = 0;
List students;
for (Department dept : departments) {
students = dept.getStudents();
for (Student s : students) {
noOfStudents++;
}
}
return noOfStudents;
}
}
// Class 4
// main class
class GFG {
// main driver method
public static void main(String[] args)
{
// Creating object of Student class inside main()
Student s1 = new Student("Mia", 1, "CSE");
Student s2 = new Student("Priya", 2, "CSE");
Student s3 = new Student("John", 1, "EE");
Student s4 = new Student("Rahul", 2, "EE");
// Creating a List of CSE Students
List cse_students = new ArrayList();
// Adding CSE students
cse_students.add(s1);
cse_students.add(s2);
// Creating a List of EE Students
List ee_students
= new ArrayList();
// Adding EE students
ee_students.add(s3);
ee_students.add(s4);
// Creating objects of EE and CSE class inside
// main()
Department CSE = new Department("CSE", cse_students);
Department EE = new Department("EE", ee_students);
List departments = new ArrayList();
departments.add(CSE);
departments.add(EE);
// Lastly creating an instance of Institute
Institute institute = new Institute("BITS", departments);
// Display message for better readability
System.out.print("Total students in institute: ");
// Calling method to get total number of students
// in institute and printing on console
System.out.print(institute.getTotalStudentsInInstitute());
}
}
Total students in institute: 4
输出解释:在这个例子中,有一个研究所没有。 CSE、EE 等部门。每个部门都没有。学生的。因此,我们创建了一个引用 Object 或 no 的 Institute 类。部门类的对象(即对象列表)。这意味着 Institute 类通过其 Object(s) 与 Department 类相关联。并且 Department 类还引用了 Student 类的一个或多个对象(即对象列表),这意味着它通过其 Object(s) 与 Student 类相关联。
它代表了一种Has-A关系。在上面的例子中:Student Has-A name。学生有身份证。 Student Has-A Dept. Department Has-A学生如下图所示。
When do we use Aggregation ??
Code reuse is best achieved by aggregation.
概念 3:组合
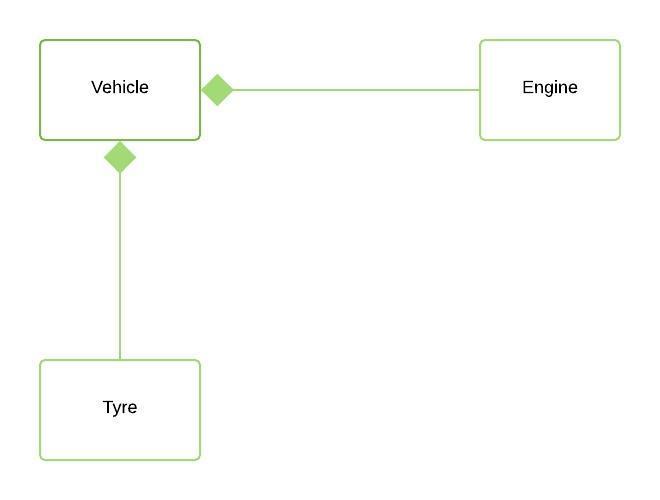
作品
组合是聚合的一种受限形式,其中两个实体高度依赖于彼此。
- 它代表关系的一部分。
- 在组合中,两个实体相互依赖。
- 当两个实体之间存在组合时,组合对象不能没有另一个实体而存在。
示例库
Java
// Java program to illustrate
// the concept of Composition
// Importing required classes
import java.io.*;
import java.util.*;
// Class 1
// Book
class Book {
// Attributes of book
public String title;
public String author;
// Constructor of Book class
Book(String title, String author)
{
// This keyword refers to current instance itself
this.title = title;
this.author = author;
}
}
// Class 2
class Library {
// Reference to refer to list of books
private final List books;
// Library class contains list of books
Library(List books)
{
// Referring to same book as
// this keyword refers to same instance itself
this.books = books;
}
// Method
// To get total number of books in library
public List getTotalBooksInLibrary()
{
return books;
}
}
// Class 3
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating objects of Book class inside main()
// method Custom inputs
Book b1
= new Book("EffectiveJ Java", "Joshua Bloch");
Book b2
= new Book("Thinking in Java", "Bruce Eckel");
Book b3 = new Book("Java: The Complete Reference",
"Herbert Schildt");
// Creating the list which contains number of books
List books = new ArrayList();
// Adding books
// using add() method
books.add(b1);
books.add(b2);
books.add(b3);
Library library = new Library(books);
// Calling method to get total books in library
// and storing it in list of user0defined type -
// Books
List bks = library.getTotalBooksInLibrary();
// Iterating over books using for each loop
for (Book bk : bks) {
// Printing the title and author name of book on
// console
System.out.println("Title : " + bk.title
+ " and "
+ " Author : " + bk.author);
}
}
}
Title : EffectiveJ Java and Author : Joshua Bloch
Title : Thinking in Java and Author : Bruce Eckel
Title : Java: The Complete Reference and Author : Herbert Schildt
输出说明:在上面的例子中,一个库可以没有。相同或不同主题的书籍。因此,如果图书馆被摧毁,那么该特定图书馆内的所有书籍都将被摧毁。即没有图书馆,书籍就无法存在。这就是为什么它是组合。书是图书馆的一部分。
聚合与组合
1. 依赖关系:聚合意味着子节点可以独立于父节点而存在的关系。比如Bank and Employee,删除Bank and Employee 仍然存在。而组合意味着孩子不能独立于父母而存在的关系。示例:人与心,心与人不分离
2. 关系类型:聚合关系是“has-a” ,组合关系是“part-of”关系。
3.关联类型:组合是强关联,聚合是弱关联。
例子:
Java
// Java Program to Illustrate Difference between
// Aggregation and Composition
// Importing I/O classes
import java.io.*;
// Class 1
// Engine class which will
// be used by car. so 'Car'
// class will have a field
// of Engine type.
class Engine {
// Method to starting an engine
public void work()
{
// Print statement whenever this method is called
System.out.println(
"Engine of car has been started ");
}
}
// Class 2
// Engine class
final class Car {
// For a car to move,
// it needs to have an engine.
// Composition
private final Engine engine;
// Note: Uncommented part refers to Aggregation
// private Engine engine;
// Constructor of this class
Car(Engine engine)
{
// This keywords refers to same instance
this.engine = engine;
}
// Method
// Car start moving by starting engine
public void move()
{
// if(engine != null)
{
// Calling method for working of engine
engine.work();
// Print statement
System.out.println("Car is moving ");
}
}
}
// Class 3
// Main class
class GFG {
// Main driver method
public static void main(String[] args)
{
// Making an engine by creating
// an instance of Engine class.
Engine engine = new Engine();
// Making a car with engine so we are
// passing a engine instance as an argument
// while creating instance of Car
Car car = new Car(engine);
// Making car to move by calling
// move() method inside main()
car.move();
}
}
Engine of car has been started
Car is moving
在聚合的情况下,Car 还通过 Engine 执行其功能。但引擎并不总是汽车的内部部件。发动机可以更换,甚至可以从汽车上拆下。这就是我们将引擎类型字段设为非最终字段的原因。