Angular Material Sort Header 组件
Angular Material是由 Google 开发的 UI 组件库,旨在由 Angular 开发人员以结构化和响应式的方式开发现代应用程序。通过使用这个库,我们可以极大地提高最终用户的用户体验,从而为我们的应用程序赢得人气。该库包含现代即用型元素,可以直接使用最少或无需额外代码。
我们可以通过两种方式禁用表格的排序:
- 我们可以在matSort指令上添加属性matSortDisabled ,或者
- 在任何单个表头上添加禁用属性
句法:
Column 1
Data
安装语法:
为了使用 Angular Material 中的 Basic Card 部分,我们必须在本地机器上安装 Angular CLI,这将有助于添加和配置 Angular 材质库。满足所需条件后,在 Angular CLI 上键入以下命令:
ng add @angular/material
有关详细的安装过程,请参阅将 Angular 材质组件添加到 Angular 应用程序一文。
添加排序标题组件:
要使用 sort-header 组件,我们需要将其导入到我们的组件中,如下所示:
import { Sort } from '@angular/material/sort';
然后,我们需要将该组件导入到app.module.ts文件中。
import {MatSortModule} from '@angular/material/sort';
项目结构:
安装成功后,项目结构如下图所示:
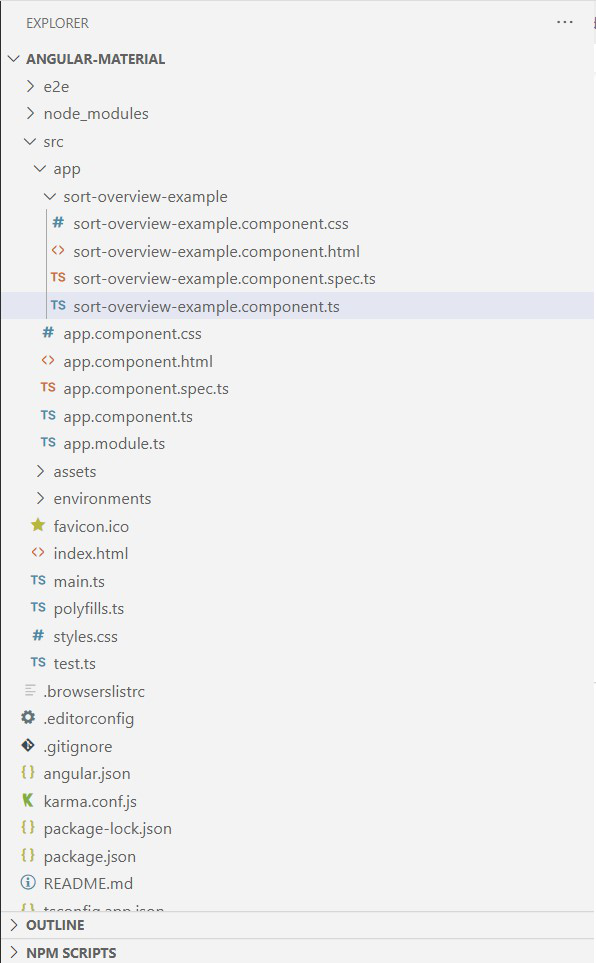
项目结构
示例:下面的示例说明了 Angular Material Sort Header 的实现。
app.module.ts
import { CommonModule } from "@angular/common";
import { NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
import { BrowserAnimationsModule } from
"@angular/platform-browser/animations";
import { SortOverviewExampleComponent } from
"./sort-overview-example/sort-overview-example.component";
import { MatSortModule } from "@angular/material/sort";
@NgModule({
declarations:
[AppComponent,
SortOverviewExampleComponent],
exports: [AppComponent],
imports:
[ CommonModule,
BrowserAnimationsModule,
BrowserModule,
MatSortModule,
],
bootstrap: [AppComponent],
})
export class AppModule {}
sort-overview-example.component.ts
import { Component, OnInit } from "@angular/core";
import { Sort } from "@angular/material/sort";
export interface Student {
physics: number;
maths: number;
chemistry: number;
name: string;
roll_number: number;
}
@Component({
selector: "app-sort-overview-example",
templateUrl: "sort-overview-example.component.html",
styleUrls: ["./sort-overview-example.component.css"],
})
export class SortOverviewExampleComponent implements OnInit {
ngOnInit(): void {}
school: Student[] = [
{ name: "Niall", physics: 91, chemistry: 86, maths: 84, roll_number: 4 },
{ name: "Liam", physics: 73, chemistry: 71, maths: 89, roll_number: 2 },
{ name: "Zayn", physics: 74, chemistry: 91, maths: 99, roll_number: 5 },
{ name: "Harry", physics: 82, chemistry: 80, maths: 92, roll_number: 3 },
{ name: "Louis", physics: 96, chemistry: 76, maths: 93, roll_number: 1 },
];
sortedData: Student[];
constructor() {
this.sortedData = this.school.slice();
}
sortData(sort: Sort) {
const data = this.school.slice();
if (!sort.active || sort.direction === "") {
this.sortedData = data;
return;
}
this.sortedData = data.sort((a, b) => {
const isAsc = sort.direction === "asc";
switch (sort.active) {
case "name":
return compare(a.name, b.name, isAsc);
case "roll_number":
return compare(a.roll_number, b.roll_number, isAsc);
case "maths":
return compare(a.maths, b.maths, isAsc);
case "physics":
return compare(a.physics, b.physics, isAsc);
case "chemistry":
return compare(a.chemistry, b.chemistry, isAsc);
default:
return 0;
}
});
}
}
function compare(a: number | string, b: number | string, isAsc: boolean) {
return (a < b ? -1 : 1) * (isAsc ? 1 : -1);
}
sort-overview-example.component.html
GeeksforGeeks
matSort & mat-sort-header Example
Roll No.
Student Name
Mathematics
Chemistry
Physics
{{ student.roll_number }}
{{ student.name }}
{{ student.maths }}
{{ student.chemistry }}
{{ student.physics }}
sort-overview-example-component.css
h1,
h3 {
color: green;
font-family: "Roboto", sans-serif;
text-align: center;
}
table {
font-family: "Open Sans", sans-serif;
margin-left: 50px;
}
app.component.html
排序概述-example.component.ts
import { Component, OnInit } from "@angular/core";
import { Sort } from "@angular/material/sort";
export interface Student {
physics: number;
maths: number;
chemistry: number;
name: string;
roll_number: number;
}
@Component({
selector: "app-sort-overview-example",
templateUrl: "sort-overview-example.component.html",
styleUrls: ["./sort-overview-example.component.css"],
})
export class SortOverviewExampleComponent implements OnInit {
ngOnInit(): void {}
school: Student[] = [
{ name: "Niall", physics: 91, chemistry: 86, maths: 84, roll_number: 4 },
{ name: "Liam", physics: 73, chemistry: 71, maths: 89, roll_number: 2 },
{ name: "Zayn", physics: 74, chemistry: 91, maths: 99, roll_number: 5 },
{ name: "Harry", physics: 82, chemistry: 80, maths: 92, roll_number: 3 },
{ name: "Louis", physics: 96, chemistry: 76, maths: 93, roll_number: 1 },
];
sortedData: Student[];
constructor() {
this.sortedData = this.school.slice();
}
sortData(sort: Sort) {
const data = this.school.slice();
if (!sort.active || sort.direction === "") {
this.sortedData = data;
return;
}
this.sortedData = data.sort((a, b) => {
const isAsc = sort.direction === "asc";
switch (sort.active) {
case "name":
return compare(a.name, b.name, isAsc);
case "roll_number":
return compare(a.roll_number, b.roll_number, isAsc);
case "maths":
return compare(a.maths, b.maths, isAsc);
case "physics":
return compare(a.physics, b.physics, isAsc);
case "chemistry":
return compare(a.chemistry, b.chemistry, isAsc);
default:
return 0;
}
});
}
}
function compare(a: number | string, b: number | string, isAsc: boolean) {
return (a < b ? -1 : 1) * (isAsc ? 1 : -1);
}
排序概述-example.component.html
GeeksforGeeks
matSort & mat-sort-header Example
Roll No.
Student Name
Mathematics
Chemistry
Physics
{{ student.roll_number }}
{{ student.name }}
{{ student.maths }}
{{ student.chemistry }}
{{ student.physics }}
排序概述示例组件.css
h1,
h3 {
color: green;
font-family: "Roboto", sans-serif;
text-align: center;
}
table {
font-family: "Open Sans", sans-serif;
margin-left: 50px;
}
app.component.html
输出:
参考: https ://material.angular.io/components/sort/overview