Java摇摆 | JFileChooser
JFileChooser 是Java Swing 包的一部分。 Java Swing 包是 JavaTM Foundation Classes(JFC) 的一部分。 JFC 包含许多有助于在Java中构建图形用户界面的特性。 Java Swing 提供了按钮、面板、对话框等组件。 JFileChooser 是一种提示用户选择文件或目录的简单而有效的方法。
在本文中,我们将了解如何在Java swing 中使用 JFileChooser。
JFileChooser 的构造函数是:
1. JFileChooser() - 指向用户默认目录的空构造函数
Java
// Using this process to invoke the constructor,
// JFileChooser points to user's default directory
JFileChooser j = new JFileChooser();
// Open the save dialog
j.showSaveDialog(null);
Java
// Using this process to invoke the constructor,
// JFileChooser points to the mentioned path
JFileChooser j = new JFileChooser("d:");
// Open the save dialog
j.showSaveDialog(null);
Java
// Using this process to invoke the constructor,
// JFileChooser points to the mentioned path
// of the file passed
JFileChooser j = new JFileChooser(new File("C:\\Users\\pc\\Documents\\New folder\\"));
// Open the save dialog
j.showSaveDialog(null);
Java
// In this process argument passed
// is an object of File System View
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView());
// Open the save dialog
j.showSaveDialog(null);
Java
// In this process argument passed is an object
// of File System View, and a path
JFileChooser j = new JFileChooser("d:", FileSystemView.getFileSystemView());
// Open the save dialog
j.showSaveDialog(null);
Java
// In this process argument passed is an object
// of File System View and a object of
// File class
File f = new File("C:\\Users\\pc\\Documents\\New folder\\");
JFileChooser j = new JFileChooser(f, FileSystemView.getFileSystemView());
// Open the save dialog
j.showSaveDialog(null);
Java
// Java program to create open or
// save dialog using JFileChooser
import java.io.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.filechooser.*;
class filechooser extends JFrame implements ActionListener {
// Jlabel to show the files user selects
static JLabel l;
// a default constructor
filechooser()
{
}
public static void main(String args[])
{
// frame to contains GUI elements
JFrame f = new JFrame("file chooser");
// set the size of the frame
f.setSize(400, 400);
// set the frame's visibility
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// button to open save dialog
JButton button1 = new JButton("save");
// button to open open dialog
JButton button2 = new JButton("open");
// make an object of the class filechooser
filechooser f1 = new filechooser();
// add action listener to the button to capture user
// response on buttons
button1.addActionListener(f1);
button2.addActionListener(f1);
// make a panel to add the buttons and labels
JPanel p = new JPanel();
// add buttons to the frame
p.add(button1);
p.add(button2);
// set the label to its initial value
l = new JLabel("no file selected");
// add panel to the frame
p.add(l);
f.add(p);
f.show();
}
public void actionPerformed(ActionEvent evt)
{
// if the user presses the save button show the save dialog
String com = evt.getActionCommand();
if (com.equals("save")) {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// invoke the showsSaveDialog function to show the save dialog
int r = j.showSaveDialog(null);
// if the user selects a file
if (r == JFileChooser.APPROVE_OPTION)
{
// set the label to the path of the selected file
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
// if the user presses the open dialog show the open dialog
else {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// invoke the showsOpenDialog function to show the save dialog
int r = j.showOpenDialog(null);
// if the user selects a file
if (r == JFileChooser.APPROVE_OPTION)
{
// set the label to the path of the selected file
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
}
}
Java
// Java program to use JFileChooser
// to select directory only
import java.io.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.filechooser.*;
class filechooser extends JFrame implements ActionListener {
// Jlabel to show the files user selects
static JLabel l;
// a default constructor
filechooser()
{
}
public static void main(String args[])
{
// frame to contains GUI elements
JFrame f = new JFrame("file chooser to select directories");
// set the size of the frame
f.setSize(400, 400);
// set the frame's visibility
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// button to open save dialog
JButton button1 = new JButton("save");
// button to open open dialog
JButton button2 = new JButton("open");
// make an object of the class filechooser
filechooser f1 = new filechooser();
// add action listener to the button to capture user
// response on buttons
button1.addActionListener(f1);
button2.addActionListener(f1);
// make a panel to add the buttons and labels
JPanel p = new JPanel();
// add buttons to the frame
p.add(button1);
p.add(button2);
// set the label to its initial value
l = new JLabel("no file selected");
// add panel to the frame
p.add(l);
f.add(p);
f.show();
}
public void actionPerformed(ActionEvent evt)
{
// if the user presses the save button show the save dialog
String com = evt.getActionCommand();
if (com.equals("save")) {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// set the selection mode to directories only
j.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
// invoke the showsSaveDialog function to show the save dialog
int r = j.showSaveDialog(null);
if (r == JFileChooser.APPROVE_OPTION) {
// set the label to the path of the selected directory
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
// if the user presses the open dialog show the open dialog
else {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// set the selection mode to directories only
j.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
// invoke the showsOpenDialog function to show the save dialog
int r = j.showOpenDialog(null);
if (r == JFileChooser.APPROVE_OPTION) {
// set the label to the path of the selected directory
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
}
}
Java
// Java program to use JFileChooser to allow multiple selection of files
import java.io.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.filechooser.*;
class filechooser extends JFrame implements ActionListener {
// Jlabel to show the files user selects
static JLabel l;
// a default constructor
filechooser()
{
}
public static void main(String args[])
{
// frame to contains GUI elements
JFrame f = new JFrame("file chooser to select multiple files at a time");
// set the size of the frame
f.setSize(400, 400);
// set the frame's visibility
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// button to open save dialog
JButton button1 = new JButton("save");
// button to open open dialog
JButton button2 = new JButton("open");
// make an object of the class filechooser
filechooser f1 = new filechooser();
// add action listener to the button to capture user
// response on buttons
button1.addActionListener(f1);
button2.addActionListener(f1);
// make a panel to add the buttons and labels
JPanel p = new JPanel();
// add buttons to the frame
p.add(button1);
p.add(button2);
// set the label to its initial value
l = new JLabel("no file selected");
// add panel to the frame
p.add(l);
f.add(p);
f.show();
}
public void actionPerformed(ActionEvent evt)
{
// if the user presses the save button show the save dialog
String com = evt.getActionCommand();
if (com.equals("save")) {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// allow multiple file selection
j.setMultiSelectionEnabled(true);
// invoke the showsSaveDialog function to show the save dialog
int r = j.showSaveDialog(null);
if (r == JFileChooser.APPROVE_OPTION) {
// get the selelcted files
File files[] = j.getSelectedFiles();
int t = 0;
// set text to blank
l.setText("");
// set the label to the path of the selected files
while (t++ < files.length)
l.setText(l.getText() + " " + files[t - 1].getName());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
// if the user presses the open dialog show the open dialog
else {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// allow multiple file selection
j.setMultiSelectionEnabled(true);
// invoke the showsOpenDialog function to show the save dialog
int r = j.showOpenDialog(null);
if (r == JFileChooser.APPROVE_OPTION) {
// get the selelcted files
File files[] = j.getSelectedFiles();
// set text to blank
l.setText("");
int t = 0;
// set the label to the path of the selected files
while (t++ < files.length)
l.setText(l.getText() + " " + files[t - 1].getName());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
}
}
Java
// Java program to use JFileChooser to restrict
// the type of files shown to the user
import java.io.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.filechooser.*;
class filechooser extends JFrame implements ActionListener {
// Jlabel to show the files user selects
static JLabel l;
// a default constructor
filechooser()
{
}
public static void main(String args[])
{
// frame to contains GUI elements
JFrame f = new JFrame("file chooser");
// set the size of the frame
f.setSize(400, 400);
// set the frame's visibility
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// button to open save dialog
JButton button1 = new JButton("save");
// button to open open dialog
JButton button2 = new JButton("open");
// make an object of the class filechooser
filechooser f1 = new filechooser();
// add action listener to the button to capture user
// response on buttons
button1.addActionListener(f1);
button2.addActionListener(f1);
// make a panel to add the buttons and labels
JPanel p = new JPanel();
// add buttons to the frame
p.add(button1);
p.add(button2);
// set the label to its initial value
l = new JLabel("no file selected");
// add panel to the frame
p.add(l);
f.add(p);
f.show();
}
public void actionPerformed(ActionEvent evt)
{
// if the user presses the save button show the save dialog
String com = evt.getActionCommand();
if (com.equals("save")) {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// resctrict the user to select files of all types
j.setAcceptAllFileFilterUsed(false);
// set a title for the dialog
j.setDialogTitle("Select a .txt file");
// only allow files of .txt extension
FileNameExtensionFilter restrict = new FileNameExtensionFilter("Only .txt files", "txt");
j.addChoosableFileFilter(restrict);
// invoke the showsSaveDialog function to show the save dialog
int r = j.showSaveDialog(null);
// if the user selects a file
if (r == JFileChooser.APPROVE_OPTION)
{
// set the label to the path of the selected file
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
// if the user presses the open dialog show the open dialog
else {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// resctrict the user to select files of all types
j.setAcceptAllFileFilterUsed(false);
// set a title for the dialog
j.setDialogTitle("Select a .txt file");
// only allow files of .txt extension
FileNameExtensionFilter restrict = new FileNameExtensionFilter("Only .txt files", "txt");
j.addChoosableFileFilter(restrict);
// invoke the showsOpenDialog function to show the save dialog
int r = j.showOpenDialog(null);
// if the user selects a file
if (r == JFileChooser.APPROVE_OPTION) {
// set the label to the path of the selected file
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
}
}
代码片段的输出:
2. JFileChooser(String) – 使用给定的路径
Java
// Using this process to invoke the constructor,
// JFileChooser points to the mentioned path
JFileChooser j = new JFileChooser("d:");
// Open the save dialog
j.showSaveDialog(null);
代码片段的输出:
3. JFileChooser(File) – 使用给定的文件作为路径
Java
// Using this process to invoke the constructor,
// JFileChooser points to the mentioned path
// of the file passed
JFileChooser j = new JFileChooser(new File("C:\\Users\\pc\\Documents\\New folder\\"));
// Open the save dialog
j.showSaveDialog(null);
代码片段的输出:
4. JFileChooser(FileSystemView) – 使用给定的 FileSystemView
Java
// In this process argument passed
// is an object of File System View
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView());
// Open the save dialog
j.showSaveDialog(null);
代码片段的输出:
5. JFileChooser(String, FileSystemView) – 使用给定的路径和 FileSystemView
Java
// In this process argument passed is an object
// of File System View, and a path
JFileChooser j = new JFileChooser("d:", FileSystemView.getFileSystemView());
// Open the save dialog
j.showSaveDialog(null);
代码片段的输出:
6. JFileChooser(File, FileSystemView) – 使用给定的当前目录和 FileSystemView
Java
// In this process argument passed is an object
// of File System View and a object of
// File class
File f = new File("C:\\Users\\pc\\Documents\\New folder\\");
JFileChooser j = new JFileChooser(f, FileSystemView.getFileSystemView());
// Open the save dialog
j.showSaveDialog(null);
代码片段的输出:
注意:上面给出的代码是代码片段而不是完整的代码,上面给出的代码片段应该根据程序员的需要和判断来调用构造函数,上面提到的路径是任意的。用户应根据需要设置路径。
JFileChooser 的实际应用
以下代码不会在在线编译器中执行。请使用离线 IDE
1. 使用 JFileChooser 创建打开或保存对话框
Java
// Java program to create open or
// save dialog using JFileChooser
import java.io.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.filechooser.*;
class filechooser extends JFrame implements ActionListener {
// Jlabel to show the files user selects
static JLabel l;
// a default constructor
filechooser()
{
}
public static void main(String args[])
{
// frame to contains GUI elements
JFrame f = new JFrame("file chooser");
// set the size of the frame
f.setSize(400, 400);
// set the frame's visibility
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// button to open save dialog
JButton button1 = new JButton("save");
// button to open open dialog
JButton button2 = new JButton("open");
// make an object of the class filechooser
filechooser f1 = new filechooser();
// add action listener to the button to capture user
// response on buttons
button1.addActionListener(f1);
button2.addActionListener(f1);
// make a panel to add the buttons and labels
JPanel p = new JPanel();
// add buttons to the frame
p.add(button1);
p.add(button2);
// set the label to its initial value
l = new JLabel("no file selected");
// add panel to the frame
p.add(l);
f.add(p);
f.show();
}
public void actionPerformed(ActionEvent evt)
{
// if the user presses the save button show the save dialog
String com = evt.getActionCommand();
if (com.equals("save")) {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// invoke the showsSaveDialog function to show the save dialog
int r = j.showSaveDialog(null);
// if the user selects a file
if (r == JFileChooser.APPROVE_OPTION)
{
// set the label to the path of the selected file
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
// if the user presses the open dialog show the open dialog
else {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// invoke the showsOpenDialog function to show the save dialog
int r = j.showOpenDialog(null);
// if the user selects a file
if (r == JFileChooser.APPROVE_OPTION)
{
// set the label to the path of the selected file
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
}
}
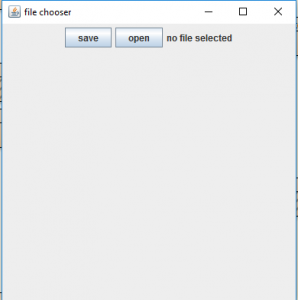
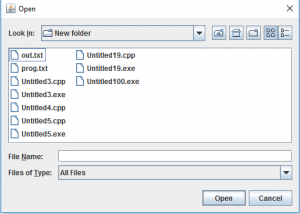
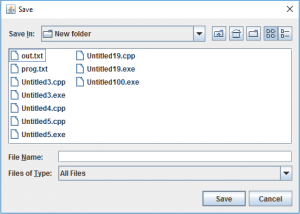
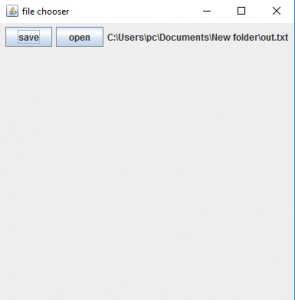
2. 使用 JFileChooser 只选择目录
Java
// Java program to use JFileChooser
// to select directory only
import java.io.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.filechooser.*;
class filechooser extends JFrame implements ActionListener {
// Jlabel to show the files user selects
static JLabel l;
// a default constructor
filechooser()
{
}
public static void main(String args[])
{
// frame to contains GUI elements
JFrame f = new JFrame("file chooser to select directories");
// set the size of the frame
f.setSize(400, 400);
// set the frame's visibility
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// button to open save dialog
JButton button1 = new JButton("save");
// button to open open dialog
JButton button2 = new JButton("open");
// make an object of the class filechooser
filechooser f1 = new filechooser();
// add action listener to the button to capture user
// response on buttons
button1.addActionListener(f1);
button2.addActionListener(f1);
// make a panel to add the buttons and labels
JPanel p = new JPanel();
// add buttons to the frame
p.add(button1);
p.add(button2);
// set the label to its initial value
l = new JLabel("no file selected");
// add panel to the frame
p.add(l);
f.add(p);
f.show();
}
public void actionPerformed(ActionEvent evt)
{
// if the user presses the save button show the save dialog
String com = evt.getActionCommand();
if (com.equals("save")) {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// set the selection mode to directories only
j.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
// invoke the showsSaveDialog function to show the save dialog
int r = j.showSaveDialog(null);
if (r == JFileChooser.APPROVE_OPTION) {
// set the label to the path of the selected directory
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
// if the user presses the open dialog show the open dialog
else {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// set the selection mode to directories only
j.setFileSelectionMode(JFileChooser.DIRECTORIES_ONLY);
// invoke the showsOpenDialog function to show the save dialog
int r = j.showOpenDialog(null);
if (r == JFileChooser.APPROVE_OPTION) {
// set the label to the path of the selected directory
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
}
}
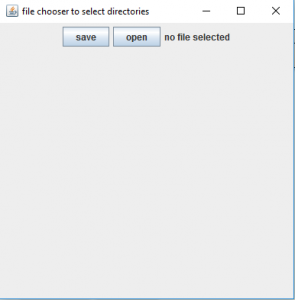
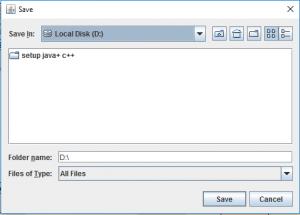
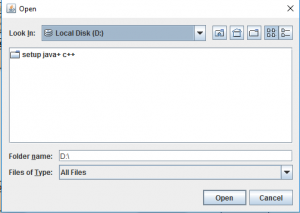
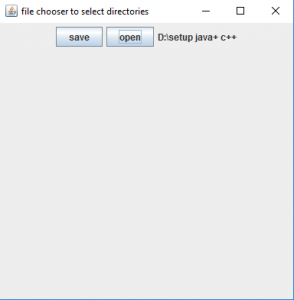
3.使用JFileChooser允许多选文件
Java
// Java program to use JFileChooser to allow multiple selection of files
import java.io.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.filechooser.*;
class filechooser extends JFrame implements ActionListener {
// Jlabel to show the files user selects
static JLabel l;
// a default constructor
filechooser()
{
}
public static void main(String args[])
{
// frame to contains GUI elements
JFrame f = new JFrame("file chooser to select multiple files at a time");
// set the size of the frame
f.setSize(400, 400);
// set the frame's visibility
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// button to open save dialog
JButton button1 = new JButton("save");
// button to open open dialog
JButton button2 = new JButton("open");
// make an object of the class filechooser
filechooser f1 = new filechooser();
// add action listener to the button to capture user
// response on buttons
button1.addActionListener(f1);
button2.addActionListener(f1);
// make a panel to add the buttons and labels
JPanel p = new JPanel();
// add buttons to the frame
p.add(button1);
p.add(button2);
// set the label to its initial value
l = new JLabel("no file selected");
// add panel to the frame
p.add(l);
f.add(p);
f.show();
}
public void actionPerformed(ActionEvent evt)
{
// if the user presses the save button show the save dialog
String com = evt.getActionCommand();
if (com.equals("save")) {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// allow multiple file selection
j.setMultiSelectionEnabled(true);
// invoke the showsSaveDialog function to show the save dialog
int r = j.showSaveDialog(null);
if (r == JFileChooser.APPROVE_OPTION) {
// get the selelcted files
File files[] = j.getSelectedFiles();
int t = 0;
// set text to blank
l.setText("");
// set the label to the path of the selected files
while (t++ < files.length)
l.setText(l.getText() + " " + files[t - 1].getName());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
// if the user presses the open dialog show the open dialog
else {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// allow multiple file selection
j.setMultiSelectionEnabled(true);
// invoke the showsOpenDialog function to show the save dialog
int r = j.showOpenDialog(null);
if (r == JFileChooser.APPROVE_OPTION) {
// get the selelcted files
File files[] = j.getSelectedFiles();
// set text to blank
l.setText("");
int t = 0;
// set the label to the path of the selected files
while (t++ < files.length)
l.setText(l.getText() + " " + files[t - 1].getName());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
}
}
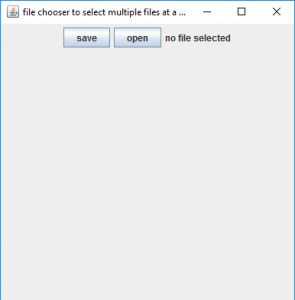
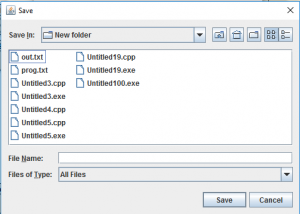
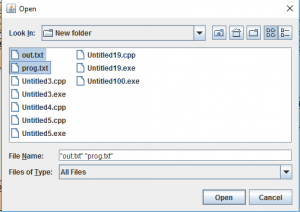
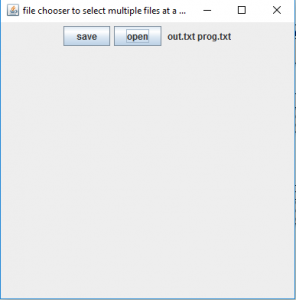
4. 使用 JFileChooser 限制向用户显示的文件类型
Java
// Java program to use JFileChooser to restrict
// the type of files shown to the user
import java.io.*;
import javax.swing.*;
import java.awt.event.*;
import javax.swing.filechooser.*;
class filechooser extends JFrame implements ActionListener {
// Jlabel to show the files user selects
static JLabel l;
// a default constructor
filechooser()
{
}
public static void main(String args[])
{
// frame to contains GUI elements
JFrame f = new JFrame("file chooser");
// set the size of the frame
f.setSize(400, 400);
// set the frame's visibility
f.setVisible(true);
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// button to open save dialog
JButton button1 = new JButton("save");
// button to open open dialog
JButton button2 = new JButton("open");
// make an object of the class filechooser
filechooser f1 = new filechooser();
// add action listener to the button to capture user
// response on buttons
button1.addActionListener(f1);
button2.addActionListener(f1);
// make a panel to add the buttons and labels
JPanel p = new JPanel();
// add buttons to the frame
p.add(button1);
p.add(button2);
// set the label to its initial value
l = new JLabel("no file selected");
// add panel to the frame
p.add(l);
f.add(p);
f.show();
}
public void actionPerformed(ActionEvent evt)
{
// if the user presses the save button show the save dialog
String com = evt.getActionCommand();
if (com.equals("save")) {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// resctrict the user to select files of all types
j.setAcceptAllFileFilterUsed(false);
// set a title for the dialog
j.setDialogTitle("Select a .txt file");
// only allow files of .txt extension
FileNameExtensionFilter restrict = new FileNameExtensionFilter("Only .txt files", "txt");
j.addChoosableFileFilter(restrict);
// invoke the showsSaveDialog function to show the save dialog
int r = j.showSaveDialog(null);
// if the user selects a file
if (r == JFileChooser.APPROVE_OPTION)
{
// set the label to the path of the selected file
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
// if the user presses the open dialog show the open dialog
else {
// create an object of JFileChooser class
JFileChooser j = new JFileChooser(FileSystemView.getFileSystemView().getHomeDirectory());
// resctrict the user to select files of all types
j.setAcceptAllFileFilterUsed(false);
// set a title for the dialog
j.setDialogTitle("Select a .txt file");
// only allow files of .txt extension
FileNameExtensionFilter restrict = new FileNameExtensionFilter("Only .txt files", "txt");
j.addChoosableFileFilter(restrict);
// invoke the showsOpenDialog function to show the save dialog
int r = j.showOpenDialog(null);
// if the user selects a file
if (r == JFileChooser.APPROVE_OPTION) {
// set the label to the path of the selected file
l.setText(j.getSelectedFile().getAbsolutePath());
}
// if the user cancelled the operation
else
l.setText("the user cancelled the operation");
}
}
}
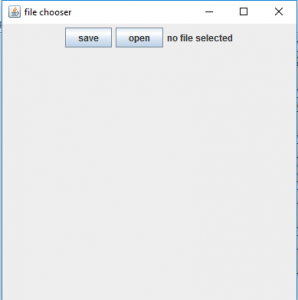
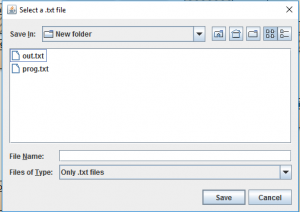
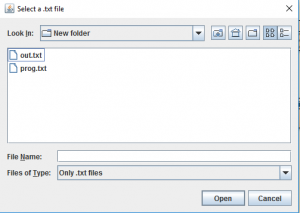
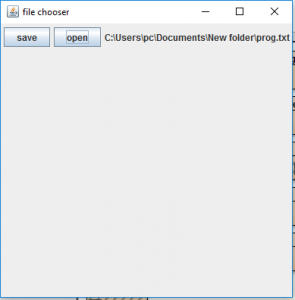
笔记 :
您还可以使用函数setApproveButtonText(String) 自定义批准按钮。这将设置已批准按钮的文本
到所需的文本。