给定一块3 X n的木板,找到最多使用4种颜色对其进行着色的方法,以使没有两个相邻的盒子具有相同的颜色。对角邻居不被视为相邻框。
随着答案的迅速增长,请输出%1000000007的方式。
限制条件:
1 <= n <100000
例子 :
输入:1输出:36我们可以使用3种颜色或2种颜色的组合。现在,从4种颜色中选择3种颜色是并将它们排列成3个!方式,类似地从4种颜色中选择2种是
而在安排时,我们只能选择其中哪一个位于中心位置,那就是两种方式。答案=
* 3! +
* 2! = 36输入:2输出:588
我们将使用动态方法解决此问题,因为当在板上添加新列时,填充颜色的方式仅取决于当前列中的颜色模式。一列中只能有两种颜色和三种颜色的组合。图像中给出了可以生成的所有可能的新列。请考虑A,B,C和D为4种颜色。
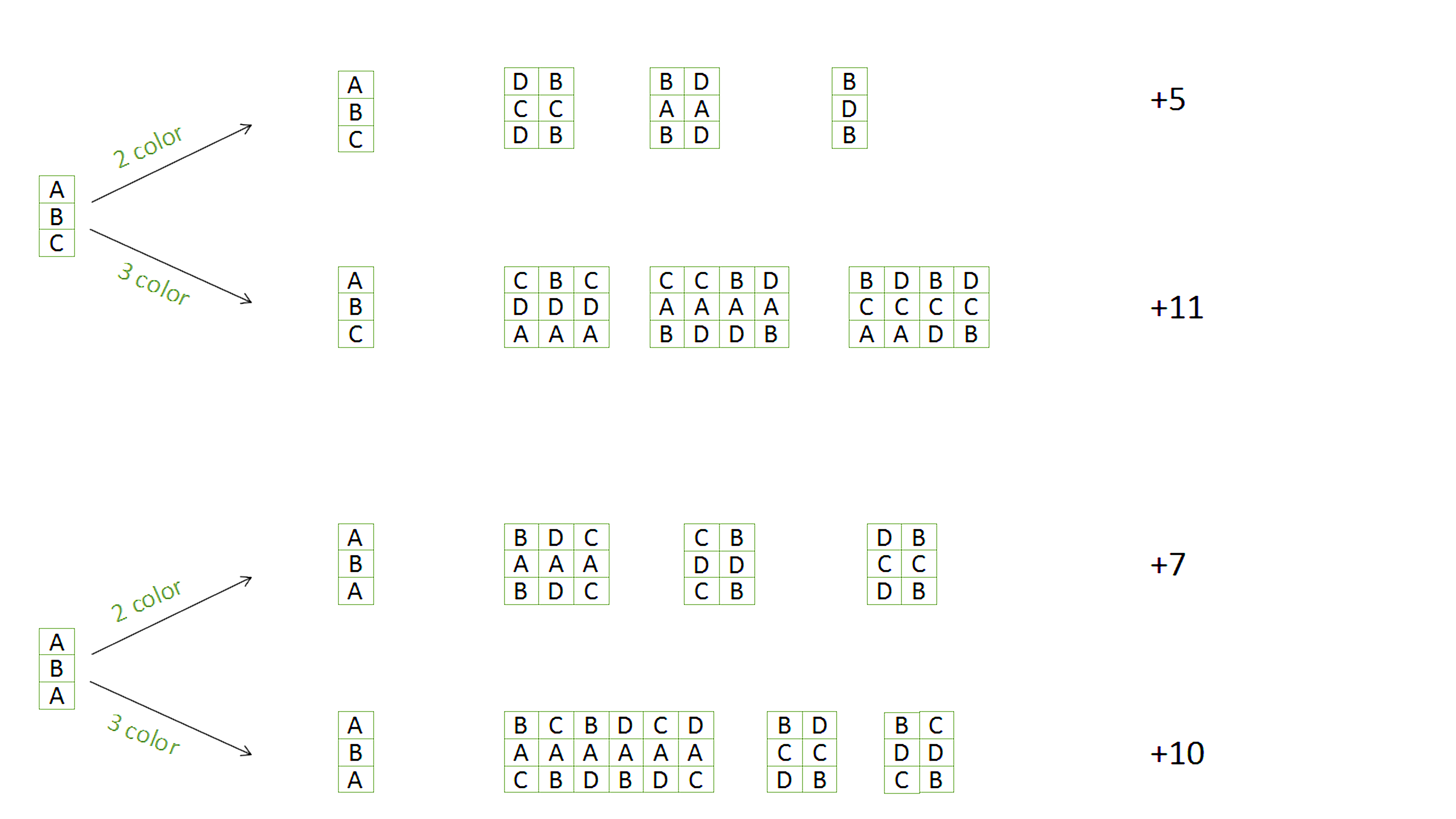
可以从当前列生成的所有可能的颜色组合。
从现在开始,我们将3 * N板第N列的3种颜色组合称为W(n),将2种颜色称为Y(n)。
我们可以看到每个W可以产生5Y和11W,每个Y可以产生7Y和10W。我们从这里得到两个方程
现在我们有两个方程,
W(n+1) = 10*Y(n)+11*W(n);
Y(n+1) = 7*Y(n)+5*W(n);
C++
// C++ program to find number of ways
// to color a 3 x n grid using 4 colors
// such that no two adjacent have same
// color
#include
using namespace std;
int solve(int A)
{
// When we to fill single column
long int color3 = 24;
long int color2 = 12;
long int temp = 0;
for (int i = 2; i <= A; i++)
{
temp = color3;
color3 = (11 * color3 + 10 *
color2 ) % 1000000007;
color2 = ( 5 * temp + 7 *
color2 ) % 1000000007;
}
long num = (color3 + color2)
% 1000000007;
return (int)num;
}
// Driver code
int main()
{
int num1 = 1;
cout << solve(num1) << endl;
int num2 = 2;
cout << solve(num2) << endl;
int num3 = 500;
cout << solve(num3) << endl;
int num4 = 10000;
cout << solve(num4);
return 0;
}
// This code is contributed by vt_m.
Java
// Java program to find number of ways to color
// a 3 x n grid using 4 colors such that no two
// adjacent have same color.
public class Solution {
public static int solve(int A) {
long color3 = 24; // When we to fill single column
long color2 = 12;
long temp = 0;
for (int i = 2; i <= A; i++)
{
long temp = color3;
color3 = (11 * color3 + 10 * color2 ) % 1000000007;
color2 = ( 5 * temp + 7 * color2 ) % 1000000007;
}
long num = (color3 + color2) % 1000000007;
return (int)num;
}
// Driver code
public static void main(String[] args)
{
int num1 = 1;
System.out.println(solve(num1));
int num2 = 2;
System.out.println(solve(num2));
int num3 = 500;
System.out.println(solve(num3));
int num4 = 10000;
System.out.println(solve(num4));
}
}
Python3
# Python 3 program to find number of ways
# to color a 3 x n grid using 4 colors
# such that no two adjacent have same
# color
def solve(A):
# When we to fill single column
color3 = 24
color2 = 12
temp = 0
for i in range(2, A + 1, 1):
temp = color3
color3 = (11 * color3 + 10 * color2 ) % 1000000007
color2 = ( 5 * temp + 7 * color2 ) % 1000000007
num = (color3 + color2) % 1000000007
return num
# Driver code
if __name__ == '__main__':
num1 = 1
print(solve(num1))
num2 = 2
print(solve(num2))
num3 = 500
print(solve(num3))
num4 = 10000
print(solve(num4))
# This code is contributed by
# Shashank_Sharma
C#
// C# program to find number of ways
// to color a 3 x n grid using 4
// colors such that no two adjacent
// have same color.
using System;
public class GFG {
public static int solve(int A)
{
// When we to fill single column
long color3 = 24;
long color2 = 12;
long temp = 0;
for (int i = 2; i <= A; i++)
{
temp = color3;
color3 = (11 * color3 + 10
* color2 ) % 1000000007;
color2 = ( 5 * temp + 7
* color2 ) % 1000000007;
}
long num = (color3 + color2)
% 1000000007;
return (int)num;
}
// Driver code
public static void Main()
{
int num1 = 1;
Console.WriteLine(solve(num1));
int num2 = 2;
Console.WriteLine(solve(num2));
int num3 = 500;
Console.WriteLine(solve(num3));
int num4 = 10000;
Console.WriteLine(solve(num4));
}
}
// This code is contributed by vt_m.
PHP
输出 :
36
588
178599516
540460643