给定两个整数m&n,找到长度为n的可能序列数,以使下一个元素中的每个元素大于或等于前一个元素的两倍但小于或等于m。
例子 :
Input : m = 10, n = 4
Output : 4
There should be n elements and value of last
element should be at-most m.
The sequences are {1, 2, 4, 8}, {1, 2, 4, 9},
{1, 2, 4, 10}, {1, 2, 5, 10}
Input : m = 5, n = 2
Output : 6
The sequences are {1, 2}, {1, 3}, {1, 4},
{1, 5}, {2, 4}, {2, 5}
根据给定条件,序列的第n个值最多为m。第n个元素可能有两种情况:
- 如果为m,则第(n-1)个元素最多为m / 2。我们重复得到m / 2和n-1。
- 如果不是m,则最多为m-1。我们重复(m-1)和n。
序列总数是包括m的序列数与不包括m的序列数之和。因此,找到具有最大m的长度为n的序列数的原始问题可以细分为以下独立的子问题:找到具有最大m-1的长度为n的序列数和具有最大m /的长度为n-1的序列数。 2。
C++
// C program to count total number of special sequences
// of length n where
#include
// Recursive function to find the number of special
// sequences
int getTotalNumberOfSequences(int m, int n)
{
// A special sequence cannot exist if length
// n is more than the maximum value m.
if (m < n)
return 0;
// If n is 0, found an empty special sequence
if (n == 0)
return 1;
// There can be two possibilities : (1) Reduce
// last element value (2) Consider last element
// as m and reduce number of terms
return getTotalNumberOfSequences (m-1, n) +
getTotalNumberOfSequences (m/2, n-1);
}
// Driver Code
int main()
{
int m = 10;
int n = 4;
printf("Total number of possible sequences %d",
getTotalNumberOfSequences(m, n));
return 0;
}
Java
// Java program to count total number
// of special sequences of length n where
class Sequences
{
// Recursive function to find the number of special
// sequences
static int getTotalNumberOfSequences(int m, int n)
{
// A special sequence cannot exist if length
// n is more than the maximum value m.
if(m < n)
return 0;
// If n is 0, found an empty special sequence
if(n == 0)
return 1;
// There can be two possibilities : (1) Reduce
// last element value (2) Consider last element
// as m and reduce number of terms
return getTotalNumberOfSequences (m-1, n) +
getTotalNumberOfSequences (m/2, n-1);
}
// main function
public static void main (String[] args)
{
int m = 10;
int n = 4;
System.out.println("Total number of possible sequences "+
getTotalNumberOfSequences(m, n));
}
}
Python3
#Python3 program to count total number of
#special sequences of length n where
#Recursive function to find the number of
# special sequences
def getTotalNumberOfSequences(m,n):
#A special sequence cannot exist if length
#n is more than the maximum value m.
if m
C#
// C# program to count total number
// of special sequences of length n
// where every element is more than
// or equal to twice of previous
using System;
class GFG
{
// Recursive function to find
// the number of special sequences
static int getTotalNumberOfSequences(int m, int n)
{
// A special sequence cannot exist if length
// n is more than the maximum value m.
if(m < n)
return 0;
// If n is 0, found an empty special sequence
if(n == 0)
return 1;
// There can be two possibilities : (1) Reduce
// last element value (2) Consider last element
// as m and reduce number of terms
return getTotalNumberOfSequences (m-1, n) +
getTotalNumberOfSequences (m/2, n-1);
}
// Driver code
public static void Main ()
{
int m = 10;
int n = 4;
Console.Write("Total number of possible sequences " +
getTotalNumberOfSequences(m, n));
}
}
// This code is contributed by nitin mittal.
PHP
C++
// C program to count total number of special sequences
// of length N where
#include
// DP based function to find the number of special
// sequences
int getTotalNumberOfSequences(int m, int n)
{
// define T and build in bottom manner to store
// number of special sequences of length n and
// maximum value m
int T[m+1][n+1];
for (int i=0; i
Java
// Efficient java program to count total number
// of special sequences of length n where
class Sequences
{
// DP based function to find the number of special
// sequences
static int getTotalNumberOfSequences(int m, int n)
{
// define T and build in bottom manner to store
// number of special sequences of length n and
// maximum value m
int T[][]=new int[m+1][n+1];
for (int i=0; i
Python3
#Python3 program to count total number of
#special sequences of length N where
#DP based function to find the number
# of special sequence
def getTotalNumberOfSequences(m,n):
#define T and build in bottom manner to store
#number of special sequences of length n and
#maximum value m
T=[[0 for i in range(n+1)] for i in range(m+1)]
for i in range(m+1):
for j in range(n+1):
#Base case : If length of sequence is 0
# or maximum value is 0, there cannot
#exist any special sequence
if i==0 or j==0:
T[i][j]=0
#if length of sequence is more than
#the maximum value, special sequence
# cannot exist
elif i
C#
// Efficient C# program to count total number
// of special sequences of length n where
using System;
class Sequences {
// DP based function to find
// the number of special
// sequences
static int getTotalNumberOfSequences(int m, int n)
{
// define T and build in
// bottom manner to store
// number of special sequences
// of length n and maximum value m
int [,]T=new int[m + 1, n + 1];
for (int i = 0; i < m + 1; i++)
{
for (int j = 0; j < n + 1; j++)
{
// Base case : If length
// of sequence is 0
// or maximum value is
// 0, there cannot
// exist any special
// sequence
if (i == 0 || j == 0)
T[i, j] = 0;
// if length of sequence
// is more than the maximum
// value, special sequence
// cannot exist
else if (i < j)
T[i,j] = 0;
// If length of sequence is 1 then the
// number of special sequences is equal
// to the maximum value
// For example with maximum value 2 and
// length 1, there can be 2 special
// sequences {1}, {2}
else if (j == 1)
T[i,j] = i;
// otherwise calculate
else
T[i,j] = T[i - 1, j] + T[i / 2, j - 1];
}
}
return T[m,n];
}
// Driver Code
public static void Main ()
{
int m = 10;
int n = 4;
Console.WriteLine("Total number of possible sequences "+
getTotalNumberOfSequences(m, n));
}
}
// This code is contributed by anuj_67.
PHP
输出:
Total number of possible sequences 4
请注意,上面的函数一次又一次地计算相同的子问题。考虑以下f(10,4)的树。
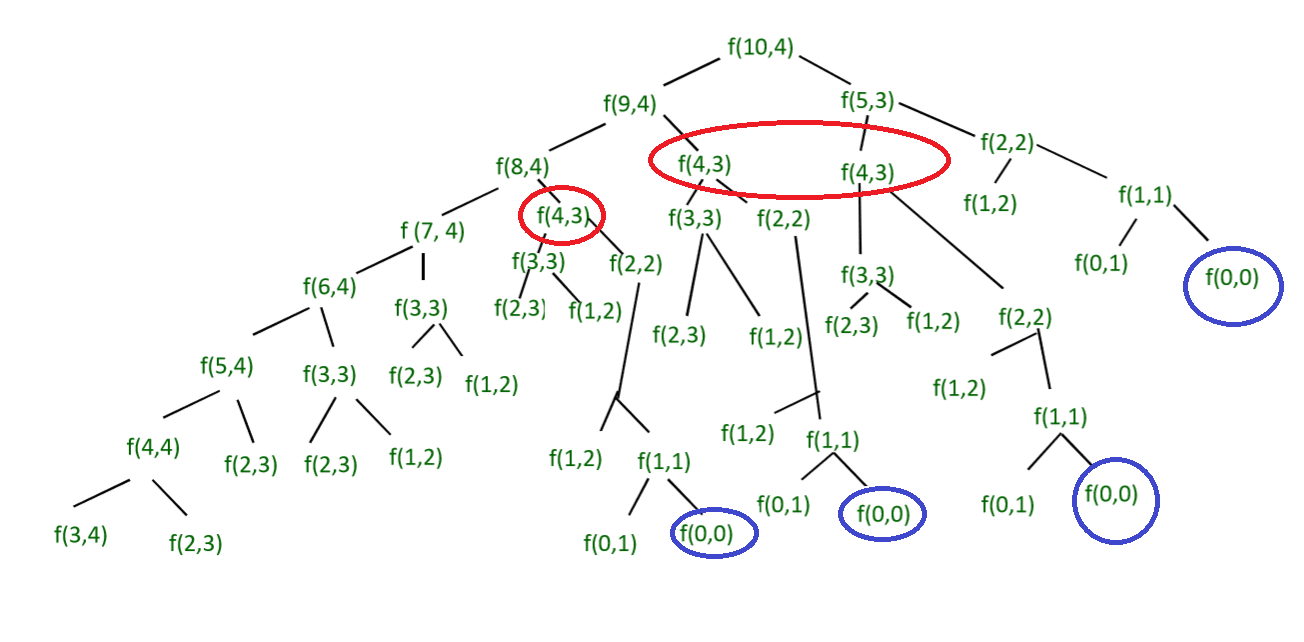
m = 10和N = 4的递归树
我们可以使用动态编程解决此问题。
C++
// C program to count total number of special sequences
// of length N where
#include
// DP based function to find the number of special
// sequences
int getTotalNumberOfSequences(int m, int n)
{
// define T and build in bottom manner to store
// number of special sequences of length n and
// maximum value m
int T[m+1][n+1];
for (int i=0; i
Java
// Efficient java program to count total number
// of special sequences of length n where
class Sequences
{
// DP based function to find the number of special
// sequences
static int getTotalNumberOfSequences(int m, int n)
{
// define T and build in bottom manner to store
// number of special sequences of length n and
// maximum value m
int T[][]=new int[m+1][n+1];
for (int i=0; i
Python3
#Python3 program to count total number of
#special sequences of length N where
#DP based function to find the number
# of special sequence
def getTotalNumberOfSequences(m,n):
#define T and build in bottom manner to store
#number of special sequences of length n and
#maximum value m
T=[[0 for i in range(n+1)] for i in range(m+1)]
for i in range(m+1):
for j in range(n+1):
#Base case : If length of sequence is 0
# or maximum value is 0, there cannot
#exist any special sequence
if i==0 or j==0:
T[i][j]=0
#if length of sequence is more than
#the maximum value, special sequence
# cannot exist
elif i
C#
// Efficient C# program to count total number
// of special sequences of length n where
using System;
class Sequences {
// DP based function to find
// the number of special
// sequences
static int getTotalNumberOfSequences(int m, int n)
{
// define T and build in
// bottom manner to store
// number of special sequences
// of length n and maximum value m
int [,]T=new int[m + 1, n + 1];
for (int i = 0; i < m + 1; i++)
{
for (int j = 0; j < n + 1; j++)
{
// Base case : If length
// of sequence is 0
// or maximum value is
// 0, there cannot
// exist any special
// sequence
if (i == 0 || j == 0)
T[i, j] = 0;
// if length of sequence
// is more than the maximum
// value, special sequence
// cannot exist
else if (i < j)
T[i,j] = 0;
// If length of sequence is 1 then the
// number of special sequences is equal
// to the maximum value
// For example with maximum value 2 and
// length 1, there can be 2 special
// sequences {1}, {2}
else if (j == 1)
T[i,j] = i;
// otherwise calculate
else
T[i,j] = T[i - 1, j] + T[i / 2, j - 1];
}
}
return T[m,n];
}
// Driver Code
public static void Main ()
{
int m = 10;
int n = 4;
Console.WriteLine("Total number of possible sequences "+
getTotalNumberOfSequences(m, n));
}
}
// This code is contributed by anuj_67.
的PHP
输出:
4
时间复杂度:O(mxn)
辅助空间:O(mxn)