求方阵的特征多项式
在线性代数中,方阵的特征多项式是在矩阵相似性下不变且以特征值为根的多项式。它的系数之间有矩阵的行列式和迹。
3×3矩阵的特征多项式可以使用公式计算
x3 – (Trace of matrix)*x2 + (Sum of minors along diagonal)*x – determinant of matrix = 0
例子:
Input: mat[][] = { { 0, 1, 2 }, { 1, 0, -1 }, { 2, -1, 0 } }
Output: x^3 – 6x + 4
方法:让我们分解公式并一一找出每个术语的值:
矩阵的踪迹
Trace of a Matrix 沿其对角线元素的总和。
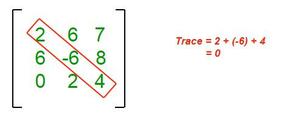
矩阵的痕迹
矩阵的小数
- 矩阵的小数是针对矩阵的每个元素的,它等于在排除包含该特定元素的行和列之后剩余的矩阵部分。由给定矩阵的每个元素的小数组成的新矩阵称为矩阵的小数。
- 次要矩阵的每个新元素可以如下实现:
矩阵的行列式
- 矩阵的行列式是一个特殊的数字,仅针对方阵(具有相同行数和列数的矩阵)定义。行列式在微积分和另一个与矩阵相关的代数中的许多地方使用,它实际上用实数表示矩阵,可用于求解线性方程组和求矩阵的逆。
求方阵特征多项式的程序
C++
// C++ code to calculate
// characteristic polynomial of 3x3 matrix
#include
using namespace std;
#define N 3
// Trace
int findTrace(int mat[N][N], int n)
{
int sum = 0;
for (int i = 0; i < n; i++)
sum += mat[i][i];
return sum;
}
// Sum of minors along diagonal
int sum_of_minors(int mat[N][N], int n)
{
return (
(mat[2][2] * mat[1][1] - mat[2][1] * mat[1][2])
+ (mat[2][2] * mat[0][0] - mat[2][0] * mat[0][2])
+ (mat[1][1] * mat[0][0] - mat[1][0] * mat[0][1]));
}
// Function to get cofactor of mat[p][q]
// in temp[][]. n is current dimension of mat[][]
// Cofactor will be used for calculating determinant
void getCofactor(int mat[N][N], int temp[N][N], int p,
int q, int n)
{
int i = 0, j = 0;
// Looping for each element of the matrix
for (int row = 0; row < n; row++) {
for (int col = 0; col < n; col++) {
// Copying into temporary matrix only those
// element which are not in given row and
// column
if (row != p && col != q) {
temp[i][j++] = mat[row][col];
// Row is filled, so increase row index
// and reset col index
if (j == n - 1) {
j = 0;
i++;
}
}
}
}
}
// Function for calculating
// determinant of matrix
int determinantOfMatrix(int mat[N][N], int n)
{
// Initialize result
int D = 0;
// Base case : if matrix
// contains single element
if (n == 1)
return mat[0][0];
// To store cofactors
int temp[N][N];
// To store sign multiplier
int sign = 1;
// Iterate for each element of first row
for (int f = 0; f < n; f++) {
// Getting Cofactor of mat[0][f]
getCofactor(mat, temp, 0, f, n);
D += sign * mat[0][f]
* determinantOfMatrix(temp, n - 1);
// Terms are to be added with alternate sign
sign = -sign;
}
return D;
}
// Driver Code
int main()
{
// Given matrix
int mat[N][N]
= { { 0, 1, 2 }, { 1, 0, -1 }, { 2, -1, 0 } };
int trace = findTrace(mat, 3);
int s_o_m = sum_of_minors(mat, 3);
int det = determinantOfMatrix(mat, 3);
cout << "x^3";
if (trace != 0) {
trace < 0 ? cout << " + " << trace * -1 << "x^2"
: cout << " - " << trace << "x^2";
}
if (s_o_m != 0) {
s_o_m < 0 ? cout << " - " << s_o_m * -1 << "x"
: cout << " + " << s_o_m << "x";
}
if (det != 0) {
det < 0 ? cout << " + " << det * -1
: cout << " - " << det;
}
return 0;
}
Java
// Java code to calculate
// characteristic polynomial of 3x3 matrix
import java.util.*;
class GFG{
static final int N =3;
// Trace
static int findTrace(int mat[][], int n)
{
int sum = 0;
for (int i = 0; i < n; i++)
sum += mat[i][i];
return sum;
}
// Sum of minors along diagonal
static int sum_of_minors(int mat[][], int n)
{
return (
(mat[2][2] * mat[1][1] - mat[2][1] * mat[1][2])
+ (mat[2][2] * mat[0][0] - mat[2][0] * mat[0][2])
+ (mat[1][1] * mat[0][0] - mat[1][0] * mat[0][1]));
}
// Function to get cofactor of mat[p][q]
// in temp[][]. n is current dimension of mat[][]
// Cofactor will be used for calculating determinant
static void getCofactor(int mat[][], int temp[][], int p,
int q, int n)
{
int i = 0, j = 0;
// Looping for each element of the matrix
for (int row = 0; row < n; row++) {
for (int col = 0; col < n; col++) {
// Copying into temporary matrix only those
// element which are not in given row and
// column
if (row != p && col != q) {
temp[i][j++] = mat[row][col];
// Row is filled, so increase row index
// and reset col index
if (j == n - 1) {
j = 0;
i++;
}
}
}
}
}
// Function for calculating
// determinant of matrix
static int determinantOfMatrix(int mat[][], int n)
{
// Initialize result
int D = 0;
// Base case : if matrix
// contains single element
if (n == 1)
return mat[0][0];
// To store cofactors
int [][]temp = new int[N][N];
// To store sign multiplier
int sign = 1;
// Iterate for each element of first row
for (int f = 0; f < n; f++) {
// Getting Cofactor of mat[0][f]
getCofactor(mat, temp, 0, f, n);
D += sign * mat[0][f]
* determinantOfMatrix(temp, n - 1);
// Terms are to be added with alternate sign
sign = -sign;
}
return D;
}
// Driver Code
public static void main(String[] args)
{
// Given matrix
int [][]mat
= { { 0, 1, 2 }, { 1, 0, -1 }, { 2, -1, 0 } };
int trace = findTrace(mat, 3);
int s_o_m = sum_of_minors(mat, 3);
int det = determinantOfMatrix(mat, 3);
System.out.print("x^3");
if (trace != 0) {
if(trace < 0)
System.out.print(" + " + trace * -1+ "x^2");
else
System.out.print(" - " + trace+ "x^2");
}
if (s_o_m != 0) {
if(s_o_m < 0 )
System.out.print(" - " + s_o_m * -1+ "x");
else
System.out.print(" + " + s_o_m+ "x");
}
if (det != 0) {
if(det < 0 )
System.out.print(" + " + det * -1);
else
System.out.print(" - " + det);
}
}
}
// This code is contributed by Rajput-Ji
Python3
# JavaScript code for the above approach
N = 3
# Trace
def findTrace(mat, n):
sum = 0
for i in range(n):
sum += mat[i][i]
return sum
# Sum of minors along diagonal
def sum_of_minors(mat, n):
return ((mat[2][2] * mat[1][1] - mat[2][1] * mat[1][2])
+ (mat[2][2] * mat[0][0] - mat[2][0] * mat[0][2])
+ (mat[1][1] * mat[0][0] - mat[1][0] * mat[0][1]))
# Function to get cofactor of mat[p][q]
# in temp[][]. n is current dimension of mat[][]
# Cofactor will be used for calculating determinant
def getCofactor(mat, temp, p, q, n):
i,j = 0,0
# Looping for each element of the matrix
for row in range(n):
for col in range(n):
# Copying into temporary matrix only those
# element which are not in given row and
# column
if (row != p and col != q):
temp[i][j] = mat[row][col]
j += 1
# Row is filled, so increase row index
# and reset col index
if (j == n - 1):
j = 0
i += 1
# Function for calculating
# determinant of matrix
def determinantOfMatrix(mat, n):
# Initialize result
D = 0
# Base case : if matrix
# contains single element
if (n == 1):
return mat[0][0]
# To store cofactors
temp = [[0 for i in range(n)] for j in range(n)]
# To store sign multiplier
sign = 1
# Iterate for each element of first row
for f in range(n):
# Getting Cofactor of mat[0][f]
getCofactor(mat, temp, 0, f, n)
D += sign * mat[0][f] * determinantOfMatrix(temp, n - 1)
# Terms are to be added with alternate sign
sign = -sign
return D
# Driver Code
# Given matrix
mat = [[0, 1, 2], [1, 0, -1], [2, -1, 0]]
trace = findTrace(mat, 3)
s_o_m = sum_of_minors(mat, 3)
det = determinantOfMatrix(mat, 3)
print("x^3",end="")
if (trace != 0):
print(f" {trace * -1}x^2",end="") if(trace < 0) else print(f" - {trace}x^2",end="")
if (s_o_m != 0):
print(f" - {s_o_m * -1}x",end="") if(s_o_m < 0) else print(f" + {s_o_m}x",end="")
if (det != 0):
print(f" + {det * -1}",end="") if (det < 0) else print(f" - {det}",end="")
# This code is contributed by shinjanpatra
C#
// C# code to calculate
// characteristic polynomial of 3x3 matrix
using System;
class GFG {
static int N = 3;
// Trace
static int findTrace(int[, ] mat, int n)
{
int sum = 0;
for (int i = 0; i < n; i++)
sum += mat[i, i];
return sum;
}
// Sum of minors along diagonal
static int sum_of_minors(int[, ] mat, int n)
{
return (
(mat[2, 2] * mat[1, 1] - mat[2, 1] * mat[1, 2])
+ (mat[2, 2] * mat[0, 0]
- mat[2, 0] * mat[0, 2])
+ (mat[1, 1] * mat[0, 0]
- mat[1, 0] * mat[0, 1]));
}
// Function to get cofactor of mat[p][q]
// in temp[][]. n is current dimension of mat[][]
// Cofactor will be used for calculating determinant
static void getCofactor(int[, ] mat, int[, ] temp,
int p, int q, int n)
{
int i = 0, j = 0;
// Looping for each element of the matrix
for (int row = 0; row < n; row++) {
for (int col = 0; col < n; col++) {
// Copying into temporary matrix only those
// element which are not in given row and
// column
if (row != p && col != q) {
temp[i, j++] = mat[row, col];
// Row is filled, so increase row index
// and reset col index
if (j == n - 1) {
j = 0;
i++;
}
}
}
}
}
// Function for calculating
// determinant of matrix
static int determinantOfMatrix(int[, ] mat, int n)
{
// Initialize result
int D = 0;
// Base case : if matrix
// contains single element
if (n == 1)
return mat[0, 0];
// To store cofactors
int[, ] temp = new int[N, N];
// To store sign multiplier
int sign = 1;
// Iterate for each element of first row
for (int f = 0; f < n; f++) {
// Getting Cofactor of mat[0][f]
getCofactor(mat, temp, 0, f, n);
D += sign * mat[0, f]
* determinantOfMatrix(temp, n - 1);
// Terms are to be added with alternate sign
sign = -sign;
}
return D;
}
// Driver Code
public static void Main()
{
// Given matrix
int[, ] mat
= { { 0, 1, 2 }, { 1, 0, -1 }, { 2, -1, 0 } };
int trace = findTrace(mat, 3);
int s_o_m = sum_of_minors(mat, 3);
int det = determinantOfMatrix(mat, 3);
Console.Write("x^3");
if (trace != 0) {
if (trace < 0)
Console.Write(" + " + trace * -1 + "x^2");
else
Console.Write(" - " + trace + "x^2");
}
if (s_o_m != 0) {
if (s_o_m < 0)
Console.Write(" - " + s_o_m * -1 + "x");
else
Console.Write(" + " + s_o_m + "x");
}
if (det != 0) {
if (det < 0)
Console.Write(" + " + det * -1);
else
Console.Write(" - " + det);
}
}
}
// This code is contributed by ukasp.
Javascript
输出
x^3 - 6x + 4
时间复杂度: O(N 3 ),其中 N 是方阵的大小
辅助空间: O(N)