Kotlin 中的动态图像切换器
Android ImageSwitcher是一个用户界面小部件,它为图像提供平滑的过渡动画效果,同时在它们之间切换以显示在视图中。
ImageSwitcher 是 View Switcher 的子类,用于为一张图像设置动画并显示下一张。
在这里,我们以编程方式在 Kotlin 文件中创建 ImageSwitcher。
首先,我们按照以下步骤创建一个新项目:
- 单击File ,然后单击New => New Project 。
- 之后包括 Kotlin 支持,然后单击下一步。
- 根据方便选择最小的 SDK,然后单击下一步按钮。
- 然后选择Empty activity => next => finish 。
修改activity_main.xml文件
在这个文件中,我们使用带有 ImageSwitcher 和 Buttons 的约束布局。
XML
XML
ImageSwitcherInKotlin
Next
Prev
Kotlin
package com.geeksforgeeks.myfirstkotlinapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.animation.AnimationUtils
import android.widget.Button
import android.widget.ImageSwitcher
import android.widget.ImageView
import androidx.constraintlayout.widget.ConstraintLayout
class MainActivity : AppCompatActivity() {
private val flowers = intArrayOf(R.drawable.flower1, R.drawable.flower2,
R.drawable.flower4)
private var index = 0
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// create the ImageSwitcher
val imgSwitcher = ImageSwitcher(this)
imgSwitcher?.setFactory({
val imgView = ImageView(applicationContext)
imgView.scaleType = ImageView.ScaleType.FIT_CENTER
imgView.setPadding(20, 20, 20, 20)
imgView
})
val c_Layout = findViewById(R.id.constraint_layout)
//add ImageSwitcher in constraint layout
c_Layout?.addView(imgSwitcher)
// set the method and pass array as a parameter
imgSwitcher?.setImageResource(flowers[index])
val imgIn = AnimationUtils.loadAnimation(
this, android.R.anim.slide_in_left)
imgSwitcher?.inAnimation = imgIn
val imgOut = AnimationUtils.loadAnimation(
this, android.R.anim.slide_out_right)
imgSwitcher?.outAnimation = imgOut
// previous button functionality
val prev = findViewById
XML
更新字符串.xml 文件
在这里,我们使用字符串标签更新应用程序的名称。
XML
ImageSwitcherInKotlin
Next
Prev
ImageSwitcher 小部件的不同方法
Methods | Description |
---|---|
setImageDrawable | It is used to set a new drawable on the next ImageView in the switcher. |
setImageResource | It is used to set a new image on the ImageSwitcher with the given resource id. |
setImageURI | It is used to set a new image on the ImageSwitcher with the given URI. |
在 MainActivity.kt 文件中创建 ImageSwitcher
首先,我们声明一个数组flowers ,其中包含用于ImageView 的图像资源。
private val flowers = intArrayOf(R.drawable.flower1,
R.drawable.flower2, R.drawable.flower4)
然后,我们在MainActivity.kt文件中创建ImageSwitcher并设置 ImageView 显示图像。
val imgSwitcher = ImageSwitcher(this)
imgSwitcher?.setFactory({
val imgView = ImageView(applicationContext)
imgView.scaleType = ImageView.ScaleType.FIT_CENTER
imgView.setPadding(8, 8, 8, 8)
imgView
})
此外,我们应该将 ImageSwitcher 添加到使用的布局中。
val c_Layout = findViewById(R.id.constraint_layout)
//add ImageSwitcher in constraint layout
c_Layout?.addView(imgSwitcher)
科特林
package com.geeksforgeeks.myfirstkotlinapp
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.view.animation.AnimationUtils
import android.widget.Button
import android.widget.ImageSwitcher
import android.widget.ImageView
import androidx.constraintlayout.widget.ConstraintLayout
class MainActivity : AppCompatActivity() {
private val flowers = intArrayOf(R.drawable.flower1, R.drawable.flower2,
R.drawable.flower4)
private var index = 0
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// create the ImageSwitcher
val imgSwitcher = ImageSwitcher(this)
imgSwitcher?.setFactory({
val imgView = ImageView(applicationContext)
imgView.scaleType = ImageView.ScaleType.FIT_CENTER
imgView.setPadding(20, 20, 20, 20)
imgView
})
val c_Layout = findViewById(R.id.constraint_layout)
//add ImageSwitcher in constraint layout
c_Layout?.addView(imgSwitcher)
// set the method and pass array as a parameter
imgSwitcher?.setImageResource(flowers[index])
val imgIn = AnimationUtils.loadAnimation(
this, android.R.anim.slide_in_left)
imgSwitcher?.inAnimation = imgIn
val imgOut = AnimationUtils.loadAnimation(
this, android.R.anim.slide_out_right)
imgSwitcher?.outAnimation = imgOut
// previous button functionality
val prev = findViewById(R.id.prev)
prev.setOnClickListener {
index = if (index - 1 >= 0) index - 1 else 1
imgSwitcher?.setImageResource(flowers[index])
}
// next button functionality
val next = findViewById(R.id.next)
next.setOnClickListener {
index = if (index + 1 < flowers.size) index +1 else 0
imgSwitcher?.setImageResource(flowers[index])
}
}
}
AndroidManifest.xml 文件
XML
作为模拟器运行:
单击下一步按钮,然后我们在视图中获得另一个动画图像。
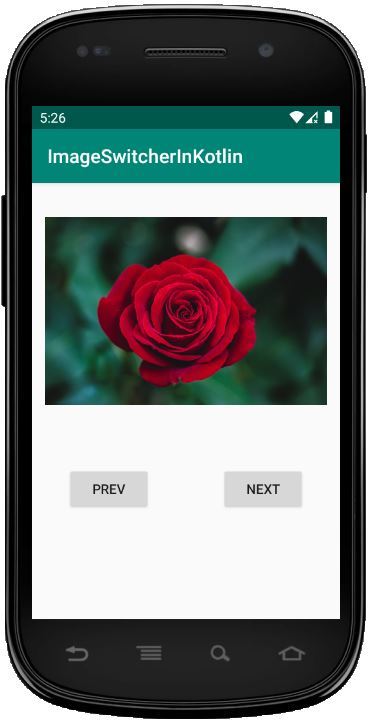
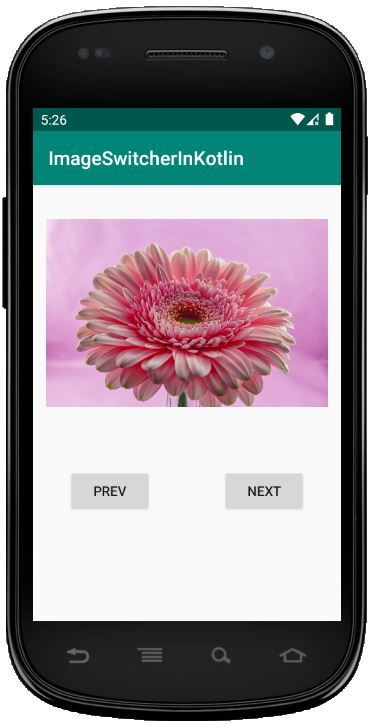
在评论中写代码?请使用 ide.geeksforgeeks.org,生成链接并在此处分享链接。