PUT 和 PATCH 请求之间的区别
在本文中,我们将讨论PUT和PATCH HTTP 请求之间的区别。 PUT 和 PATCH 请求是 HTTP 动词,都与更新某个位置的资源有关。
PUT HTTP 请求: PUT 是一种修改资源的方法,客户端发送更新整个资源的数据。 PUT 与 POST 类似,因为它可以创建资源,但是当存在已定义的 URL 时,它会这样做,其中 PUT 如果存在则替换整个资源,如果不存在则创建新资源。
例如,当您要更新候选人姓名和电子邮件时,您必须发送候选人的所有参数,包括请求正文中不更新的参数,否则它将简单地将整个资源替换为姓名和电子邮件。
{
id: 8,
email: "lindsay.ferguson@reqres.in",
// field to be updated
first_name: "Lindsay",
// field to be updated
last_name: "Ferguson",
avatar: "https://reqres.in/img/faces/8-image.jpg"
}
让我们举个例子,通过只发送我们想要更新的字段来理解 PUT 请求。
示例:以下代码演示了PUT请求方法
Javascript
let PutRequest = () => {
// Sending PUT request with fetch API in javascript
fetch("https://reqres.in/api/users/2", {
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
method: "PUT",
// Sending only the fields that to be updated
body: JSON.stringify({
email: "hello@geeky.com",
first_name: "Geeky"
})
})
.then(function (response) {
// Console.log(response);
return response.json();
})
.then(function (data) {
console.log(data);
});
};
PutRequest();
Javascript
let PatchRequest = () => {
// sending PUT request with fetch API in javascript
fetch("https://reqres.in/api/users/2", {
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
method: "PATCH",
// Fields that to be updated are passed
body: JSON.stringify({
email: "hello@geeky.com",
first_name: "Geeky"
})
})
.then(function (response) {
// console.log(response);
return response.json();
})
.then(function (data) {
console.log(data);
});
};
PatchRequest();
输出:
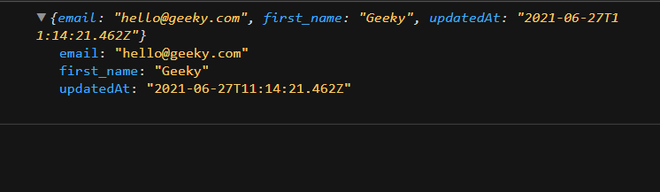
放置请求的示例
在上面的示例中,我们向服务器发出了一个 PUT 请求,并在正文中附加了一个有效负载。如果我们想更新姓名和电子邮件,通过 PUT 请求,我们必须将所有其他字段(例如 id、avatarlast_name)发送到服务器,否则,它会用传递的有效负载替换数据,如上例所示。
PATCH HTTP 请求:与 PUT 请求不同,PATCH 会部分更新例如需要由客户端更新的字段,仅更新该字段而不修改其他字段。
所以在前面的例子中,我们只需要在请求正文中发送名称和电子邮件字段。
{
"first_name":"Geeky", // field that to be updated
"email":"hello@geeky.com", // field that to be updated
}
示例:以下代码演示PATCH请求方法
Javascript
let PatchRequest = () => {
// sending PUT request with fetch API in javascript
fetch("https://reqres.in/api/users/2", {
headers: {
Accept: "application/json",
"Content-Type": "application/json"
},
method: "PATCH",
// Fields that to be updated are passed
body: JSON.stringify({
email: "hello@geeky.com",
first_name: "Geeky"
})
})
.then(function (response) {
// console.log(response);
return response.json();
})
.then(function (data) {
console.log(data);
});
};
PatchRequest();
输出:
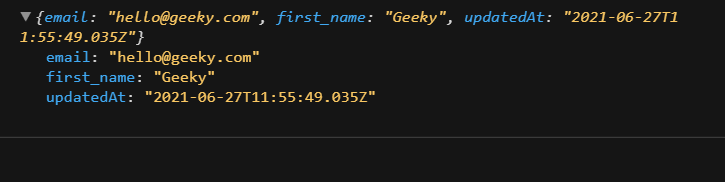
补丁请求
在上面的示例中,我们向服务器发出了一个PATCH请求,并在正文中附加了一个有效负载。如果我们想通过 PATCH 请求更新 email 和 first_name,那么我们必须只发送必须更新的字段,例如 first_name 和 email。
PUT 和 PATCH 请求的区别: PUT PATCH PUT is a method of modifying resource where the client sends data that updates the entire resource . PATCH is a method of modifying resources where the client sends partial data that is to be updated without modifying the entire data. In a PUT request, the enclosed entity is considered to be a modified version of the resource stored on the origin server, and the client is requesting that the stored version be replaced With PATCH, however, the enclosed entity contains a set of instructions describing how a resource currently residing on the origin server should be modified to produce a new version. HTTP PUT is said to be idempotent, So if you send retry a request multiple times, that should be equivalent to a single request modification HTTP PATCH is basically said to be non-idempotent. So if you retry the request N times, you will end up having N resources with N different URIs created on the server. It has High Bandwidth Since Only data that need to be modified if send in the request body as a payload , It has Low Bandwidth