Spring – MVC 文本区域
在这里,我们将学习 Spring MVC TextArea表单标签。我们将在 Spring 工具套件 (STS) 中创建一个基本的 Spring MVC 项目,以使用TextArea表单标签从用户那里获取一些数据。
spring-form.tld
我们可以使用Java Server Pages (JSP) 作为 Spring Framework 中的视图组件。为了帮助您使用 JSP 实现视图,Spring 框架提供了一个名为spring-form.tld的表单标记库,其中包含一些用于评估错误、设置主题、格式化输入和输出国际化消息的字段的标记。
‘textarea’ tag: The textarea is one of the tag provided by the spring-form.tld library. It renders an input HTML ‘textarea‘. Below are the various attributes available in textarea tag.
“textarea”标签中的属性
1. HTML 标准属性
HTML 标准属性也称为全局属性,可用于所有 HTML 元素。
Attribute Name | Description |
---|---|
accesskey | To specify a shortcut key to activate/focus on an element. |
id | To specify a unique ID for the element. |
lang | To specify the language of the content. |
tabindex | To specify tabbing order of an element. |
title | To specify extra information about the element. |
dir | To specify text direction of elements content. |
2. HTML 事件属性
HTML 事件属性用于在元素上发生特定事件时触发函数。Attribute Name Description onblur To execute a javascript function when a user leaves the text field. onchange To execute a javascript function when a user changes the text. onclick To execute a javascript function when the user clicks on the field. ondblclick To execute a javascript function when the user double clicks on the element. onfocus To execute a javascript function when the user focuses on the text box. onkeydown To execute a javascript function when the user is pressing a key on the keyboard. onkeypress To execute a javascript function when the user presses the key on the keyboard. onkeyup To execute a javascript function when the user is releasing the key on the keyboard. onmousedown To execute a javascript function when the user is pressing a mouse button. onmousemove To execute a javascript function when the user is moving the mouse pointer. onmouseout To execute a javascript function when the user is moving the mouse pointer out of the field. onmouseover To execute a javascript function when the user is moving the mouse pointer onto the field. onmouseup To execute a javascript function when the user is releasing the mouse button. onselect To execute a javascript function when the user selects the text.
3. HTML 必需属性Attribute Name Description cols To specify the column value of the text area. rows To specify the row value of the text area.
4.其他 HTML 属性Attribute Name Description cssClass To specify a class name for an HTML element to access it. cssStyle To add styles to an element, such as color, font, size, etc. cssErrorClass Used when the bounded element has errors. disabled To specify the element to be disabled or not. htmlEscape To enable/disable HTML escaping of rendered values. path To specify the path to a property for binding the data. readonly To make the HTML element read-only field.
Spring MVC 应用程序
下面是我们将要创建的应用程序。
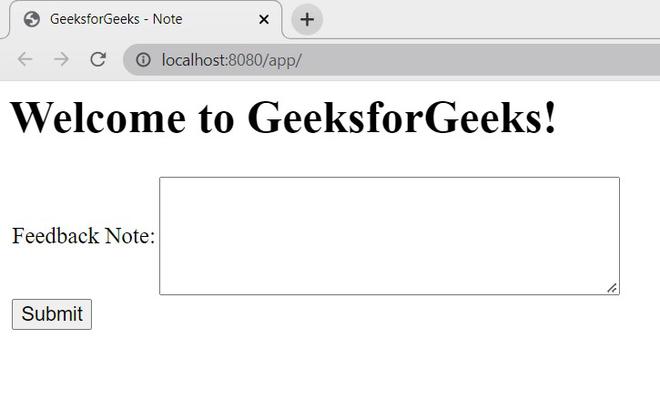
Spring MVC Textarea – 示例应用程序
程序
创建应用程序的步骤如下:
第 1 步:在 Spring Tool Suite 中创建一个 Spring MVC 项目。
第二步:在STS中,根据开发者选择创建项目时,会下载所有需要的maven依赖,*.jar,lib文件,并提供嵌入式服务器。
第三步:下面是Spring MVC项目创建后的最终项目结构 *. Java和 *.jsp 文件。
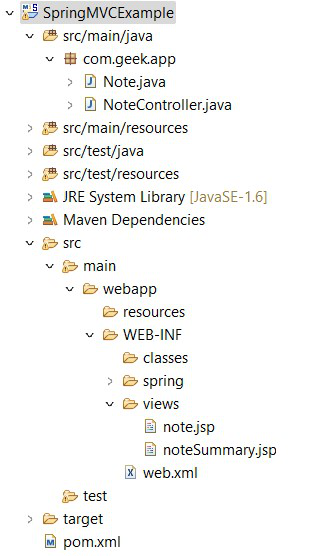
项目结构
执行
要创建的文件如下:
- 笔记。 Java:定义属性的 Bean 类和属性的 getter/setter 方法。
- 笔记控制器。 Java:处理请求并生成输出的控制器类。
- note.jsp:从浏览器获取用户输入的 Jsp 文件。
- noteSummary.jsp:将处理后的输出显示给用户的 Jsp 文件。
A.文件:note.jsp
HTML
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%>
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
<%@ page session="false"%>
GeeksforGeeks - Note
Welcome to GeeksforGeeks!
Feedback Note:
Submit
Java
// Java Program to Illustrate Note Class
package com.geek.app;
// Class
public class Note {
public String noteDetail;
// Method
public String getNoteDetail() { return noteDetail; }
// Method
public void setNoteDetail(String noteDetail)
{
this.noteDetail = noteDetail;
}
}
Java
package com.geek.app;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class NoteController {
@RequestMapping(value = "/")
public String viewNote(Model model) {
Note note = new Note();
model.addAttribute("note", note);
return "note";
}
@RequestMapping(value = "/submit", method = RequestMethod.POST)
public String submitNote(@ModelAttribute("note") Note note) {
return "noteSummary";
}
}
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
GeeksforGeeks - Feedback Summary
Feedback submitted successfully!
Feedback details:
${note.noteDetail}
HTML
这是与用户交互以获取信息的 JSP 页面。在表单内部,为了正确显示字段,我们使用了table标记。提供一个标签和文本区域以使用表单标签 - textarea 在其中输入一些数据。标签textarea将在处理时在内部提供一个 HTML textarea 框。
B. 档案:注意。Java
Java
// Java Program to Illustrate Note Class
package com.geek.app;
// Class
public class Note {
public String noteDetail;
// Method
public String getNoteDetail() { return noteDetail; }
// Method
public void setNoteDetail(String noteDetail)
{
this.noteDetail = noteDetail;
}
}
- 这是用于定义属性的Java Bean 类。
- 创建noteDetail属性以在其中输入用户输入的数据。
- 生成属性的 getter/setter 方法来获取/设置属性的值。
C. NoteController。Java
Java
package com.geek.app;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class NoteController {
@RequestMapping(value = "/")
public String viewNote(Model model) {
Note note = new Note();
model.addAttribute("note", note);
return "note";
}
@RequestMapping(value = "/submit", method = RequestMethod.POST)
public String submitNote(@ModelAttribute("note") Note note) {
return "noteSummary";
}
}
这是 Spring 控制器类,它根据请求的映射处理方法。这里, @ Controller 向容器传达这个类是 spring 控制器类。注释@RequestMapping根据提供的值将请求 URL 映射到指定的方法。
D. noteSummary.jsp
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
GeeksforGeeks - Feedback Summary
Feedback submitted successfully!
Feedback details:
${note.noteDetail}
- 这是显示处理输入的输出的 Jsp 页面。
- 当控制器处理输入时,我们使用这个 Jsp 页面向用户显示输出。
执行
- 创建所有必需的Java和 jsp 文件后,在服务器上运行该项目。
- 在项目上,运行方式-> 在服务器上运行。
- 在 localhost 中选择服务器以运行应用程序。
- 在浏览器中打开 URL: http://localhost:8080/app/以获取以下屏幕。
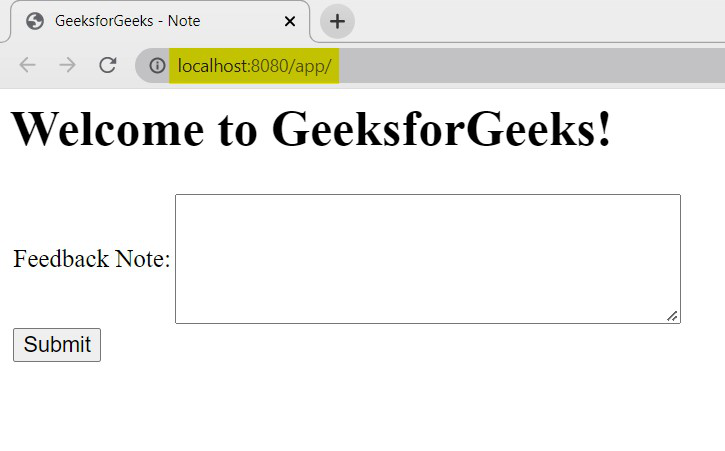
欢迎页面——note.jsp
- 由于我们为textarea提供了不同的属性,我们可以看到这些功能。
HTML
- 正如我们上面所讨论的, id属性指定了文本框的 ID。
- path属性指定输入数据必须绑定到的路径名。
- rows属性指定可以在文本框中输入的行数。
- cols属性指定可以在文本框中输入的列数。
- 标题属性显示给定的值 - GFG ,光标在文本框上。
- onfocus、onblur是事件发生时执行相应功能的事件属性。
- cssStyle属性可用于为文本框中输入的数据提供任何样式,如字体颜色、字体样式、字体系列等。
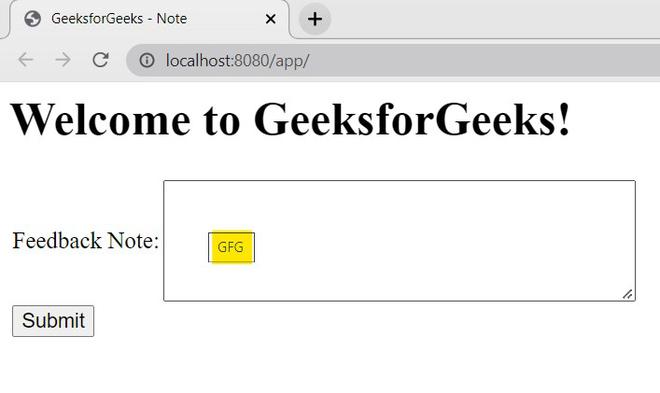
标题属性
- 当光标放在文本框上时,我们可以在文本框上看到值GFG ,因为我们指定了属性title=”GFG”。
- 单击文本框后,颜色将根据onfocus属性更改,如下所示。
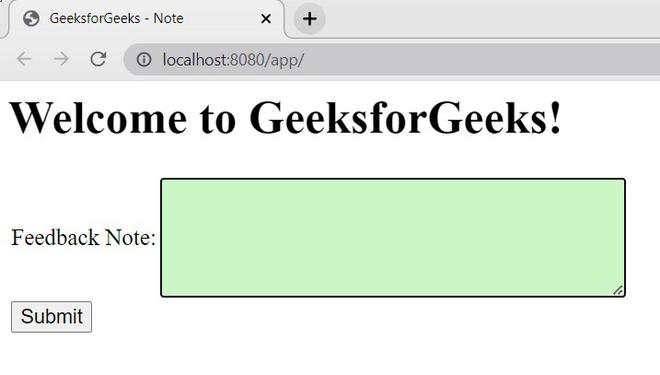
onfocus 属性
- 在文本框中输入数据,然后单击提交。
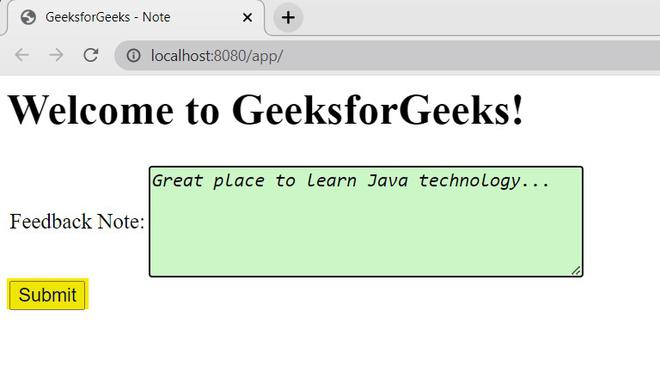
cssStyle 属性
- 当我们指定属性cssStyle = “font-style:italic” 时,用户输入的文本具有斜体字体。
- 提交页面后,控制器类将根据 URL 映射执行其中的submitNote方法并提供以下输出。
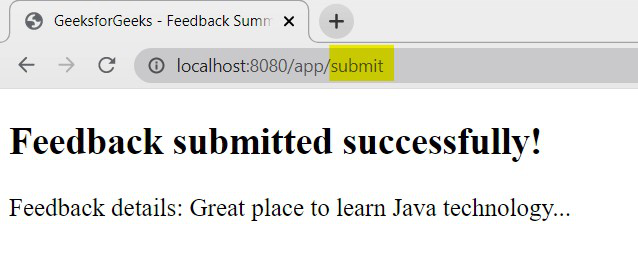
输出——noteSummary.jsp
This way we can use the tag – textarea in forms with the required attributes based on the requirement provided while developing Spring MVC applications.