Python Pillow – 使用图像模块
在本文中,我们将看到如何在Python中使用 PIL 的图像模块。首先,让我们看看如何安装PIL。
安装:
Linux:在 Linux 终端上键入以下内容:
pip install Pillow
通过终端安装pip:
sudo apt-get update
sudo apt-get install python-pip
使用图像模块
在这里,我们将介绍图像模块提供的一些方法和属性,如下所示:
- 打开图片,
- 保存图片,
- 图像大小,
- 旋转图像,
- 裁剪图像,
- 调整图像大小等等。
让我们借助一些示例来一一讨论。
1. 打开图片:
要使用 PIL 打开图像,我们使用 open() 方法。
Syntax: PIL.Image.open(fp, mode=’r’, formats=None)
Python3
# importing Image from PIL
from PIL import Image
# open an image
img = Image.open('gfg.png')
Python3
from PIL import Image
with Image.open("gfg.png") as image:
width, height = image.size
print((width,height))
Python3
from PIL import Image
img = Image.open("gfg.png")
img.save("logo.jpg")
Python3
from PIL import Image
img = Image.open("gfg.png")
rot_img = img.rotate(180)
rot_img.save("rotated_gfg.png")
Python3
from PIL import Image
# open image and get size
img = Image.open("gfg.jpg")
width, height = img.size
# cropped image using corrdinates
area = (0, 0, width/2, height/2)
crop_img = img.crop(area)
crop_img.save("cropped_image.jpg")
Python3
from PIL import Image
img = Image.open("gfg.png")
width, height = img.size
#resizing the image
img = img.resize((width//2, height//2))
img.save("resized_picture.png")
Python3
from PIL import Image
img1 = Image.open("gfg.jpg")
#pasting img2 on img1
img2 = Image.open("gfg.png")
img1.paste(img2, (50, 50))
img1.save("pasted_picture.jpg")
Python3
from PIL import Image
img = Image.open("gfg.png")
#flipping the image by 180 degree horizontally
transposed_img = img.transpose(Image.FLIP_LEFT_RIGHT)
transposed_img.save("transposed.png")
Python3
from PIL import Image
img = Image.open("gfg.png")
img.thumbnail((200, 200))
img.save("thumb.png")
输出:
2.检索图像的大小:
要检索图像的大小,我们将使用 Image 对象提供的属性,即; Image.size 属性。
Syntax: Image.size
蟒蛇3
from PIL import Image
with Image.open("gfg.png") as image:
width, height = image.size
print((width,height))
输出:
(200, 200)
3. 保存图像中的更改:
为了保存图像,我们使用 Image.save() 方法。
Syntax: Image.save(fp, format=None, **params)
Parameters:
- fp – A filename (string), pathlib.Path object or file object.
- format – Optional format override. If omitted, the format to use is determined from the filename extension. If a file object was used instead of a filename, this parameter should always be used.
- options – Extra parameters to the image writer.
Returns: None
蟒蛇3
from PIL import Image
img = Image.open("gfg.png")
img.save("logo.jpg")
输出:
4. 旋转图像:
图像旋转需要一个角度作为参数来旋转图像。
Syntax: Image.rotate(angle, resample=0, expand=0, center=None, translate=None, fillcolor=None)
Parameters:
- angle – In degrees counterclockwise.
- resample – An optional resampling filter.
- expand – Optional expansion flag. If true, expands the output image to make it large enough to hold the entire rotated image.
- center – Optional center of rotation (a 2-tuple). Origin is the upper left corner. Default is the center of the image.
- translate – An optional post-rotate translation (a 2-tuple).
- fillcolor – An optional color for area outside the rotated image.
蟒蛇3
from PIL import Image
img = Image.open("gfg.png")
rot_img = img.rotate(180)
rot_img.save("rotated_gfg.png")
输出:
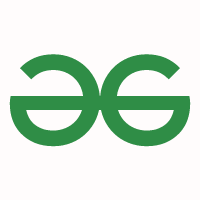
原图
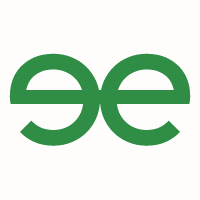
旋转图像
5.裁剪图像:
Image.crop(box) 采用 4 元组(左、上、右、下)像素坐标,并从使用的图像返回一个矩形区域。
Syntax: PIL.Image.crop(box = None)
Parameters:
- box – a 4-tuple defining the left, upper, right, and lower pixel coordinate.
Return type: Image (Returns a rectangular region as (left, upper, right, lower)-tuple).
Return: An Image object.
蟒蛇3
from PIL import Image
# open image and get size
img = Image.open("gfg.jpg")
width, height = img.size
# cropped image using corrdinates
area = (0, 0, width/2, height/2)
crop_img = img.crop(area)
crop_img.save("cropped_image.jpg")
输出:
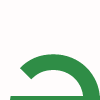
裁剪图像
6. 调整图像大小:
Image.resize(size) 用于调整大小。这里的大小以 2 元组的宽度和高度提供。
Syntax: Image.resize(size, resample=0)
Parameters:
- size – The requested size in pixels, as a 2-tuple: (width, height).
- resample – An optional resampling filter. This can be one of PIL.Image.NEAREST (use nearest neighbour), PIL.Image.BILINEAR (linear interpolation), PIL.Image.BICUBIC (cubic spline interpolation), or PIL.Image.LANCZOS (a high-quality downsampling filter). If omitted, or if the image has mode “1” or “P”, it is set PIL.Image.NEAREST.
Returns type: An Image object.
蟒蛇3
from PIL import Image
img = Image.open("gfg.png")
width, height = img.size
#resizing the image
img = img.resize((width//2, height//2))
img.save("resized_picture.png")
输出:
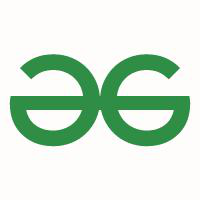
调整大小的图像
7. 将一张图片粘贴到另一张图片上:
第二个参数可以是 2 元组(指定左上角),也可以是 4 元组(左、上、右、下)——在这种情况下,粘贴图像的大小必须与此框区域的大小匹配或 None 等价于 (0, 0)。
Syntax: PIL.Image.Image.paste(image_1, image_2, box=None, mask=None)
OR
image_object.paste(image_2, box=None, mask=None)
Parameters:
- image_1/image_object : It the image on which other image is to be pasted.
- image_2: Source image or pixel value (integer or tuple).
- box: An optional 4-tuple giving the region to paste into. If a 2-tuple is used instead, it’s treated as the upper left corner. If omitted or None, the source is pasted into the upper left corner.
- mask: An optional mask image.
If an image is given as the second argument and there is no third, the box defaults to (0, 0), and the second argument is interpreted as a mask image.
蟒蛇3
from PIL import Image
img1 = Image.open("gfg.jpg")
#pasting img2 on img1
img2 = Image.open("gfg.png")
img1.paste(img2, (50, 50))
img1.save("pasted_picture.jpg")
输出:
8. 转置图像:
此功能为我们提供了图像的镜像
Syntax: Transpose image (flip or rotate in 90 degree steps)
Parameters:
- method – One of PIL.Image.FLIP_LEFT_RIGHT, PIL.Image.FLIP_TOP_BOTTOM, PIL.Image.ROTATE_90, PIL.Image.ROTATE_180, PIL.Image.ROTATE_270 or PIL.Image.TRANSPOSE.
Returns type: An Image object.
蟒蛇3
from PIL import Image
img = Image.open("gfg.png")
#flipping the image by 180 degree horizontally
transposed_img = img.transpose(Image.FLIP_LEFT_RIGHT)
transposed_img.save("transposed.png")
输出:

原来的

转置图像
9. 创建缩略图:
此方法创建打开的图像的缩略图。它不会返回新的图像对象,而是对当前打开的图像对象本身进行就地修改。如果您不想更改原始图像对象,请创建一个副本,然后应用此方法。该方法还根据传递的大小来评估适当地保持图像的纵横比。
Syntax: Image.thumbnail(size, resample=3)
Parameters:
- size – Requested size.
- resample – Optional resampling filter.
Returns Type: An Image object.
蟒蛇3
from PIL import Image
img = Image.open("gfg.png")
img.thumbnail((200, 200))
img.save("thumb.png")
输出:
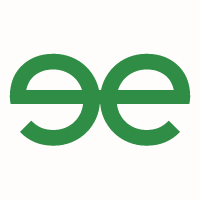
200 x 200 像素的缩略图