SQLAlchemy Core – 多表删除
在本文中,我们将了解如何在 SQLAlchemy 中对Python中的 PostgreSQL 数据库执行多表删除。
为演示创建表 - 书籍
从 SQLAlchemy 包中导入必要的函数。使用 create_engine()函数与 PostgreSQL 数据库建立连接,如下所示,创建一个名为 books 的表,其中包含 book_id 和 book_price、genre、book_name 列。如图所示,使用 insert() 和 values()函数将记录插入表中。
Python3
# import necessary packages
import sqlalchemy
from sqlalchemy import create_engine, MetaData,
Table, Column, Numeric, Integer, VARCHAR, update, text, delete
from sqlalchemy.engine import result
# establish connections
engine = create_engine(
"database+dialect://username:password@hostname:port/databasename")
# initialize the Metadata Object
meta = MetaData(bind=engine)
MetaData.reflect(meta)
# create a table schema
books = Table(
'books', meta,
Column('book_id', Integer, primary_key=True),
Column('book_price', Numeric),
Column('genre', VARCHAR),
Column('book_name', VARCHAR)
)
meta.create_all(engine)
# insert records into the table
statement1 = books.insert().values(book_id=1,
book_price=12.2,
genre='fiction',
book_name='Old age')
statement2 = books.insert().values(book_id=2,
book_price=13.2,
genre='non-fiction',
book_name='Saturn rings')
statement3 = books.insert().values(book_id=3,
book_price=121.6,
genre='fiction',
book_name='Supernova')
statement4 = books.insert().values(book_id=4,
book_price=100,
genre='non-fiction',
book_name='History of the world')
statement5 = books.insert().values(book_id=5,
book_price=1112.2,
genre='fiction',
book_name='Sun city')
# execute the insert records statement
engine.execute(statement1)
engine.execute(statement2)
engine.execute(statement3)
engine.execute(statement4)
engine.execute(statement5)
# Get the `books` table from the Metadata object
BOOKS = meta.tables['books']
Python3
# import necessary packages
import sqlalchemy
from sqlalchemy import create_engine, MetaData,
Table, String, Column, Numeric, Integer, VARCHAR,
update, text, delete
from sqlalchemy.engine import result
# establish connection
engine = create_engine(
"database+dialect://username:password@hostname:port/databasename")
# store engine objects
meta = MetaData()
# create a table
book_publisher = Table(
'book_publisher', meta,
Column('publisher_id', Integer,
primary_key=True),
Column('publisher_name', String),
Column('publisher_estd', Integer),
)
# use create_all() function to create
# a table using objects stored in meta.
meta.create_all(engine)
# insert values
statement1 = book_publisher.insert().values(
publisher_id=1, publisher_name="Oxford",
publisher_estd=1900)
statement2 = book_publisher.insert().values(
publisher_id=2, publisher_name='Stanford',
publisher_estd=1910)
statement3 = book_publisher.insert().values(
publisher_id=3, publisher_name="MIT",
publisher_estd=1920)
statement4 = book_publisher.insert().values(
publisher_id=4, publisher_name="Springer",
publisher_estd=1930)
statement5 = book_publisher.insert().values(
publisher_id=5, publisher_name="Packt",
publisher_estd=1940)
engine.execute(statement1)
engine.execute(statement2)
engine.execute(statement3)
engine.execute(statement4)
engine.execute(statement5)
# Get the `book_publisher` table from the Metadata object
book_publisher = meta.tables['book_publisher']
Python3
from sqlalchemy import delete
# query to multiple table delete
delete_stmt = (delete(BOOKS).where(
BOOKS.c.book_id == book_publisher.c.publisher_id).where(
book_publisher.c.publisher_name == 'Springer'))
# execute the statement
engine.execute(delete_stmt)
# write the SQL query inside the
# text() block to fetch all records
sql = text("SELECT * from BOOKS")
# Fetch all the records
result = engine.execute(sql).fetchall()
# View the records
for record in result:
print("\n", record)
输出:
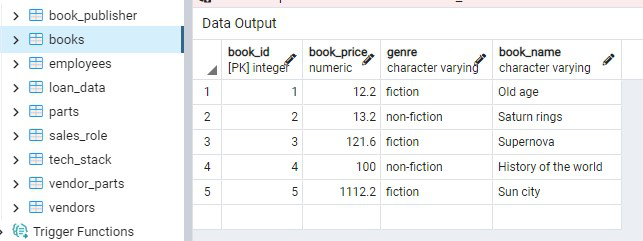
样品表 – 书籍
为演示创建表 – book_publisher
从 SQLAlchemy 包中导入必要的函数。使用 create_engine()函数与 PostgreSQL 数据库建立连接,如下所示,创建一个名为 book_publisher 的表,其中包含 publisher_id、publisher_name 和 publisher_estd 列。如图所示,使用 insert() 和 values()函数将记录插入表中。
Python3
# import necessary packages
import sqlalchemy
from sqlalchemy import create_engine, MetaData,
Table, String, Column, Numeric, Integer, VARCHAR,
update, text, delete
from sqlalchemy.engine import result
# establish connection
engine = create_engine(
"database+dialect://username:password@hostname:port/databasename")
# store engine objects
meta = MetaData()
# create a table
book_publisher = Table(
'book_publisher', meta,
Column('publisher_id', Integer,
primary_key=True),
Column('publisher_name', String),
Column('publisher_estd', Integer),
)
# use create_all() function to create
# a table using objects stored in meta.
meta.create_all(engine)
# insert values
statement1 = book_publisher.insert().values(
publisher_id=1, publisher_name="Oxford",
publisher_estd=1900)
statement2 = book_publisher.insert().values(
publisher_id=2, publisher_name='Stanford',
publisher_estd=1910)
statement3 = book_publisher.insert().values(
publisher_id=3, publisher_name="MIT",
publisher_estd=1920)
statement4 = book_publisher.insert().values(
publisher_id=4, publisher_name="Springer",
publisher_estd=1930)
statement5 = book_publisher.insert().values(
publisher_id=5, publisher_name="Packt",
publisher_estd=1940)
engine.execute(statement1)
engine.execute(statement2)
engine.execute(statement3)
engine.execute(statement4)
engine.execute(statement5)
# Get the `book_publisher` table from the Metadata object
book_publisher = meta.tables['book_publisher']
输出:
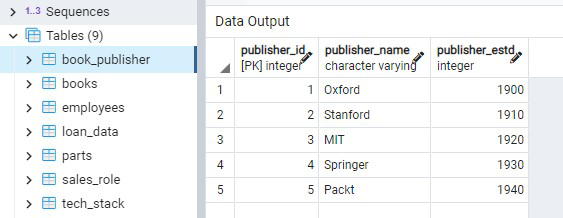
示例表 – book_publisher
在 SQLAlchemy 中实现查询以执行多表删除
执行多表删除的过程与常规 SQL 查询的过程略有不同,如下所示
from sqlalchemy import delete
delete(tablename_1).where(tablename_1.c.column_name== tablename_2.c.column_name).where(tablename_2.c.column_name== ‘value’)
从连接到数据库时初始化的元数据对象中获取书籍和 book_publisher 表。将删除查询传递给 execute()函数并使用 fetchall()函数获取所有结果。使用 for 循环遍历结果。
下面代码中显示的 SQLAlchemy 查询删除了 books 表中 book_id 与 book_publisher 表中的 publisher_name “Springer”相对应的记录。 .然后,我们可以编写一个常规的 SQL 查询,并使用 fetchall() 打印结果来检查表记录是否被正确删除。
Python3
from sqlalchemy import delete
# query to multiple table delete
delete_stmt = (delete(BOOKS).where(
BOOKS.c.book_id == book_publisher.c.publisher_id).where(
book_publisher.c.publisher_name == 'Springer'))
# execute the statement
engine.execute(delete_stmt)
# write the SQL query inside the
# text() block to fetch all records
sql = text("SELECT * from BOOKS")
# Fetch all the records
result = engine.execute(sql).fetchall()
# View the records
for record in result:
print("\n", record)
输出:
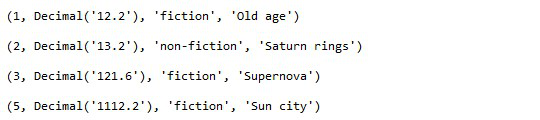
多表删除的输出