对象列表的所有组合
先决条件: Python Itertools
有几种方法可以获取Python中对象列表的所有组合。这个问题已经有递归解决方案了。 Python有一个 itertools 模块,它提供了两个名为组合()的函数 和组合_with_replacement() 这让我们的工作轻松了很多。下面是两种方法:
1. 使用 itertools.combinations():
Syntax : itertools.combination(iterable, r)
Where r is the length of output tuples.
此函数从输入迭代返回长度为 r 的子序列(元组)。它需要一个对象列表和输出元组(r)的长度作为输入。但是这个函数有几点需要注意,比如:
- 组合元组按字典顺序发出。因此,如果输入可迭代对象按排序顺序排列,则组合输出也将按排序顺序生成。
- 元素被视为唯一基于它们的位置而不是它们的值。因此,如果输入元素由重复值组成,则输出中将出现重复值。
- 返回的项目数为nCr = n! / (r! * (nr)!)当 0 <= r <= n;当 r > n 时为零。
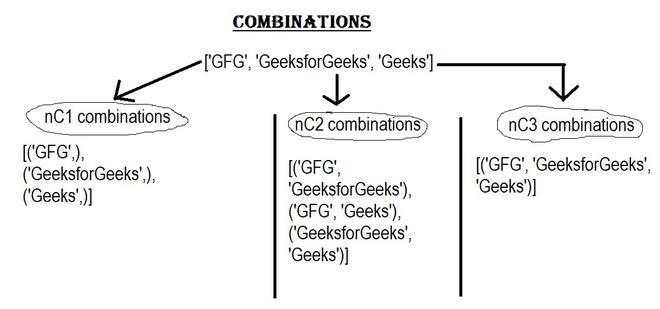
所有组合无需更换
下面是实现:
Python3
# code
from itertools import combinations
# m = list of objects.
# same method can be applied
# for list of integers.
m = ['GFG', 'GeeksforGeeks', 'Geeks']
# display
for i in range(len(m)):
print(list(combinations(m, i+1)))
Python3
# code
from itertools import combinations
# m = list of objects.
# 1st and 3rd elements are same.
# same method can be applied
# for list of integers.
m = ['GFG', 'GeeksforGeeks', 'GFG']
# output : list of combinations.
for i in range(len(m)):
print(list(combinations(m, i+1)))
Python3
# code
from itertools import combinations_with_replacement
# m = list of objects.
# same method can be applied
# for list of integers.
m = ['GFG', 'GeeksforGeeks', 'Geeks']
# output : list of combinations.
for i in range(len(m)):
print(list(combinations_with_replacement(m, i+1)))
输出:
[('GFG',), ('GeeksforGeeks',), ('Geeks',)]
[('GFG', 'GeeksforGeeks'), ('GFG', 'Geeks'), ('GeeksforGeeks', 'Geeks')]
[('GFG', 'GeeksforGeeks', 'Geeks')]
如果输入有重复元素:
蟒蛇3
# code
from itertools import combinations
# m = list of objects.
# 1st and 3rd elements are same.
# same method can be applied
# for list of integers.
m = ['GFG', 'GeeksforGeeks', 'GFG']
# output : list of combinations.
for i in range(len(m)):
print(list(combinations(m, i+1)))
输出:
[('GFG',), ('GeeksforGeeks',), ('GFG',)]
[('GFG', 'GeeksforGeeks'), ('GFG', 'GFG'), ('GeeksforGeeks', 'GFG')]
[('GFG', 'GeeksforGeeks', 'GFG')]
2. 使用 itertools.combinations_with_replacement():
Syntax: itertools.combination_with_replacement(iterable, r)
Where r is the length of output tuples.
该函数的工作原理与itertools.combinations() 相同。但是这个函数返回 r 个长度的子序列,包括重复多次的单个元素。还有几点需要注意:
- 组合元组按字典顺序发出。因此,如果输入可迭代对象按排序顺序排列,则组合输出也将按排序顺序生成。
- 元素被视为唯一基于它们的位置而不是它们的值。因此,如果输入元素由重复值组成,则输出中将出现重复值。
- 返回的项目数是(n+r-1)! /r! / (n-1)!当 n > 0 时。
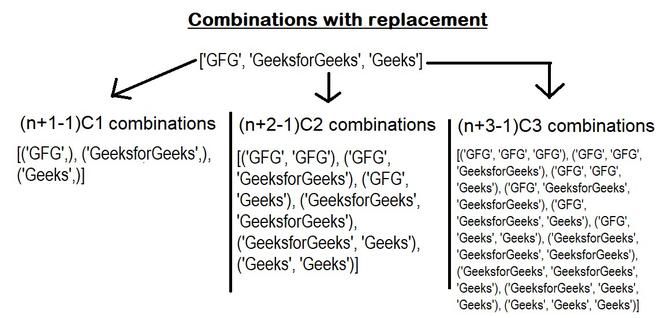
与替换组合。
下面是实现:
蟒蛇3
# code
from itertools import combinations_with_replacement
# m = list of objects.
# same method can be applied
# for list of integers.
m = ['GFG', 'GeeksforGeeks', 'Geeks']
# output : list of combinations.
for i in range(len(m)):
print(list(combinations_with_replacement(m, i+1)))
输出:
[(‘GFG’,), (‘GeeksforGeeks’,), (‘Geeks’,)]
[(‘GFG’, ‘GFG’), (‘GFG’, ‘GeeksforGeeks’), (‘GFG’, ‘Geeks’), (‘GeeksforGeeks’, ‘GeeksforGeeks’), (‘GeeksforGeeks’, ‘Geeks’), (‘Geeks’, ‘Geeks’)]
[(‘GFG’, ‘GFG’, ‘GFG’), (‘GFG’, ‘GFG’, ‘GeeksforGeeks’), (‘GFG’, ‘GFG’, ‘Geeks’), (‘GFG’, ‘GeeksforGeeks’, ‘GeeksforGeeks’), (‘GFG’, ‘GeeksforGeeks’, ‘Geeks’), (‘GFG’, ‘Geeks’, ‘Geeks’), (‘GeeksforGeeks’, ‘GeeksforGeeks’, ‘GeeksforGeeks’), (‘GeeksforGeeks’, ‘GeeksforGeeks’, ‘Geeks’), (‘GeeksforGeeks’, ‘Geeks’, ‘Geeks’), (‘Geeks’, ‘Geeks’, ‘Geeks’)]