Java Java类
此类提供线程局部变量。这些变量不同于它们的正常对应变量,因为每个访问一个(通过它的 get 或 set 方法)的线程都有它自己的、独立初始化的变量副本。基本上,这是除了编写不可变类之外实现线程安全的另一种方法。由于不再共享对象,因此不需要同步,这可以提高应用程序的可伸缩性和性能。 ThreadLocal 提供线程限制,它是局部变量的扩展。 ThreadLocal 仅在单个线程中可见。没有两个线程可以看到彼此的线程局部变量。这些变量通常是类中的私有静态字段,并在线程内维护它们的状态。
Note: ThreadLocal class extends Object class
构造函数: ThreadLocal() :这将创建一个线程局部变量。
ThreadLocal 类的方法
Method | Action Performed |
---|---|
get() | Returns the value in the current thread’s copy of this thread-local variable. If the variable has no value for the current thread, it is first initialized to the value returned by an invocation of the initialValue() method |
initialValue() | Returns the current thread initial value for the local thread variable. |
remove() | Removes the current thread’s value for this thread-local variable. If this thread-local variable is subsequently read by the current thread, its value will be reinitialized by invoking its initialValue() method, unless its value is set by the current thread in the interim. This may result in multiple invocations of the initialValue method in the current thread |
set() | Sets the current thread’s copy of this thread-local variable to the specified value. Most subclasses will have no need to override this method, relying solely on the initialValue() method to set the values of thread locals. |
示例 1:
Java
// Java Program to Illustrate ThreadLocal Class
// Via get() and set() Method
// Class
// ThreadLocalDemo
class GFG {
// Main driver method
public static void main(String[] args)
{
// Creatin objects of ThreadLocal class
ThreadLocal gfg_local
= new ThreadLocal();
ThreadLocal gfg = new ThreadLocal();
// Now setting custom value
gfg_local.set(100);
// Returns the current thread's value
System.out.println("value = " + gfg_local.get());
// Setting the value
gfg_local.set(90);
// Returns the current thread's value of
System.out.println("value = " + gfg_local.get());
// Setting the value
gfg_local.set(88.45);
// Returns the current thread's value of
System.out.println("value = " + gfg_local.get());
// Setting the value
gfg.set("GeeksforGeeks");
// Returning the current thread's value of
System.out.println("value = " + gfg.get());
}
}
Java
// Java Program to Illustrate ThreadLocal Class
// Via Illustrating remove() Method
// Class
// ThreadLocalDemo
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating objects of ThreadLocal class
ThreadLocal gfg_local
= new ThreadLocal();
ThreadLocal gfg = new ThreadLocal();
// Setting the value
gfg_local.set(100);
// Returning the current thread's value
System.out.println("value = " + gfg_local.get());
// Setting the value
gfg_local.set(90);
// Returns the current thread's value of
System.out.println("value = " + gfg_local.get());
// Setting the value
gfg_local.set(88.45);
// Returning the current thread's value of
System.out.println("value = " + gfg_local.get());
// Setting the value
gfg.set("GeeksforGeeks");
// Returning the current thread's value of
System.out.println("value = " + gfg.get());
// Removing value using remove() method
gfg.remove();
// Returning the current thread's value of
System.out.println("value = " + gfg.get());
// Removing vale
gfg_local.remove();
// Returns the current thread's value of
System.out.println("value = " + gfg_local.get());
}
}
Java
// Java Program to Illustrate ThreadLocal Class
// Via initialValue() Method
// Importing requried classes
import java.lang.*;
// Class 1
// Helper class extending Thread class
class NewThread extends Thread {
private static ThreadLocal gfg = new ThreadLocal() {
protected Object initialValue()
{
return new Integer(question--);
}
};
private static int question = 15;
NewThread(String name)
{
// super keyword refers to parent class instance
super(name);
start();
}
// Method
// run() method for Thread
public void run()
{
for (int i = 0; i < 2; i++)
System.out.println(getName() + " " + gfg.get());
}
}
// Class 2
// Main class
// ThreadLocalDemo
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating threads inside main() method
NewThread t1 = new NewThread("quiz1");
NewThread t2 = new NewThread("quiz2");
}
}
输出
value = 100
value = 90
value = 88.45
value = GeeksforGeeks
示例 2:
Java
// Java Program to Illustrate ThreadLocal Class
// Via Illustrating remove() Method
// Class
// ThreadLocalDemo
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating objects of ThreadLocal class
ThreadLocal gfg_local
= new ThreadLocal();
ThreadLocal gfg = new ThreadLocal();
// Setting the value
gfg_local.set(100);
// Returning the current thread's value
System.out.println("value = " + gfg_local.get());
// Setting the value
gfg_local.set(90);
// Returns the current thread's value of
System.out.println("value = " + gfg_local.get());
// Setting the value
gfg_local.set(88.45);
// Returning the current thread's value of
System.out.println("value = " + gfg_local.get());
// Setting the value
gfg.set("GeeksforGeeks");
// Returning the current thread's value of
System.out.println("value = " + gfg.get());
// Removing value using remove() method
gfg.remove();
// Returning the current thread's value of
System.out.println("value = " + gfg.get());
// Removing vale
gfg_local.remove();
// Returns the current thread's value of
System.out.println("value = " + gfg_local.get());
}
}
输出
value = 100
value = 90
value = 88.45
value = GeeksforGeeks
value = null
value = null
示例 3:
Java
// Java Program to Illustrate ThreadLocal Class
// Via initialValue() Method
// Importing requried classes
import java.lang.*;
// Class 1
// Helper class extending Thread class
class NewThread extends Thread {
private static ThreadLocal gfg = new ThreadLocal() {
protected Object initialValue()
{
return new Integer(question--);
}
};
private static int question = 15;
NewThread(String name)
{
// super keyword refers to parent class instance
super(name);
start();
}
// Method
// run() method for Thread
public void run()
{
for (int i = 0; i < 2; i++)
System.out.println(getName() + " " + gfg.get());
}
}
// Class 2
// Main class
// ThreadLocalDemo
public class GFG {
// Main driver method
public static void main(String[] args)
{
// Creating threads inside main() method
NewThread t1 = new NewThread("quiz1");
NewThread t2 = new NewThread("quiz2");
}
}
输出:
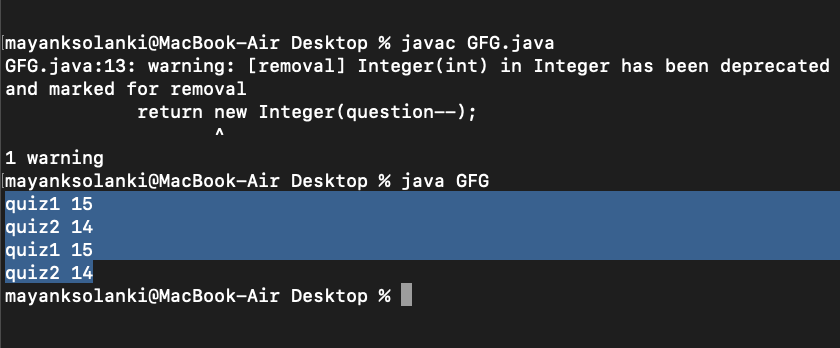