在Python中使用 NetworkX 介绍社交网络
先决条件Python基础
有没有想过最受欢迎的社交网站 Facebook 是如何运作的?我们如何仅使用 Facebook 与朋友联系?因此,Facebook 和其他社交网站使用一种称为社交网络的方法。社交网络主要用于所有社交媒体网站,如 Facebook、Instagram 和 LinkedIn 等。它对营销人员吸引客户有显着影响。社交网络使用图表来创建网络。它们的节点是人,边是它们彼此之间的连接。连接边的两个节点是朋友。现在让我们看一个例子来了解什么是社交网络。
一个班级50名学生的网络
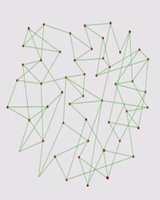
50人的网络
社交网络中使用的最重要的Python库是Networkx。
网络X
NetworkX 是一个图形包,用于创建和修改不同类型的图形。它为协作的多学科项目提供了一个快速的开发环境。
安装:
pip install networkx
启动Python后,我们必须导入 networkx 模块:
import networkx as nx
基本的内置图形类型是:
- 图:这种类型的图存储节点和边,边是无向的。它可以有自环,但不能有平行边。
- Di-Graph:这种类型的图是有向图的基类。它可以有节点和边,边在本质上是有向的。它可以有自环,但在有向图中不允许有平行边。
- Multi-Graph:这种类型的图是一种无向图类,可以存储多条或平行边。它也可以有自循环。多边是两个节点之间的多条边。
- Multi-DiGraph:这种类型的图是可以存储多条边的有向图类。它也可以有自循环。多边是两个节点之间的多条边。
图表创建示例:
Python3
# import networkx library
import networkx as nx
# create an empty undirected graph
G = nx.Graph()
# adding edge in graph G
G.add_edge(1, 2)
G.add_edge(2, 3, weight=0.9)
Python3
# import matplotlib.pyplot library
import matplotlib.pyplot as plt
# import networkx library
import networkx as nx
# create a cubical empty graph
G = nx.cubical_graph()
# plotting the graph
plt.subplot(122)
# draw a graph with red
# node and value edge color
nx.draw(G, pos = nx.circular_layout(G),
node_color = 'r',
edge_color = 'b')
Python3
# import networkx library
import netwokx as nx
# create an empty undirected graph
G = nx.Graph()
# add edge to the graph
G.add_edge('1', '2')
G.add_edge('2', '3')
# print the adjacent vertices
print(G.adj)
图表绘制:
绘图可以使用 Matplotlib.pyplot 库完成。
Python3
# import matplotlib.pyplot library
import matplotlib.pyplot as plt
# import networkx library
import networkx as nx
# create a cubical empty graph
G = nx.cubical_graph()
# plotting the graph
plt.subplot(122)
# draw a graph with red
# node and value edge color
nx.draw(G, pos = nx.circular_layout(G),
node_color = 'r',
edge_color = 'b')
输出:
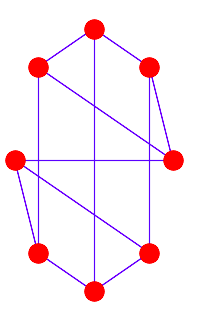
圆形图
图形边缘去除:
要从图中删除一条边,请使用图形对象的remove_edge()方法。
Syntax: G.remove_edge(u, v)
Parameters:
- u: first node
- v: second node
Return: None
图形节点删除:
要从图中删除节点,请使用图形对象的remove_node()方法。
Syntax: G.remove_node(u)
Parameter: Node to remove
Return: None
显示相邻顶点:
Python3
# import networkx library
import netwokx as nx
# create an empty undirected graph
G = nx.Graph()
# add edge to the graph
G.add_edge('1', '2')
G.add_edge('2', '3')
# print the adjacent vertices
print(G.adj)
输出:
{'1': {'2': {}}, '2': {'1': {}, '3': {}}, '3': {'2': {}}}