使用 PyMongo 更新集合中的所有文档
MongoDB 是一个开源的面向文档的数据库。 MongoDB以键值对的形式存储数据,是一个NoSQL数据库程序。 NoSQL 一词的意思是非关系型的。
PyMongo 包含用于与 MongoDB 数据库交互的工具。现在让我们看看如何更新集合中的所有文档。
更新集合中的所有文档
PyMongo 包含一个update_many()函数,它更新所有满足给定查询的文档。
update_many() 接受以下参数 -
- filter –它是第一个参数,它是更新满足查询的文档所依据的条件。
- 更新运算符-它是第二个参数,其中包含要在文档中更新的信息。
- upsert- (可选)它是一个布尔值。如果设置为 true 并且没有与过滤器匹配的文档,则会创建一个新文档。
- array_filters - (可选)它是一个过滤器文档数组,用于确定要修改哪些数组元素以对数组字段进行更新操作。
- bypass_document_validation – (可选)设置为True时跳过文档验证的布尔值。
- collation – (可选)指定操作的语言特定规则。
- session – (可选)一个 ClientSession。
MongoDB 中的更新运算符
设定值:
- $set:用于设置字段值。
- $setOnInsert:仅在插入新文档时更新值。
- $unset:删除字段及其值。
数字运算符:
- $inc:将值增加给定的数量。
- $min/$max:返回最小值或最大值。
- $mul:将值乘以给定的数量。
杂项运算符:
- $currentDate:将字段的值更新为当前日期。
- $rename : 重命名一个字段
现在让我们通过一些例子来理解。
样本数据库:
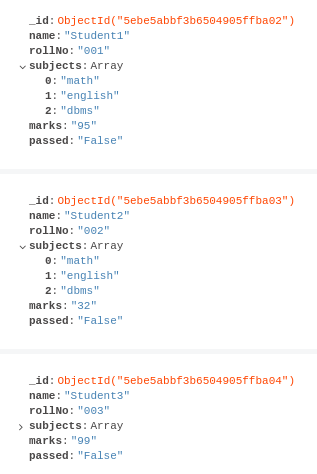
我们将在本文中看到一些用例,其中更新许多记录可能很有用:
- 根据条件更改或增加多个元素。
- 将新字段插入多个或所有文档。
示例 1:所有分数大于 35 的学生都通过了。
Python3
from pymongo import MongoClient
# Creating an instance of MongoClient
# on default localhost
client = MongoClient('mongodb://localhost:27017')
# Accessing desired database and collection
db = client.gfg
collection = db["classroom"]
# Update passed field to be true for all
# students with marks greater than 35
collection.update_many(
{"marks": { "$gt": "35" } },
{
"$set": { "passed" : "True" }
}
)
Python
from pymongo import MongoClient
# Creating an instance of MongoClient
# on default localhost
client = MongoClient('mongodb://localhost:27017')
# Accessing desired database and collection
db = client.gfg
collection = db["classroom"]
# Address filed to be added to all documents
collection.update_many(
{},
{"$set":
{
"Address": "value"
}
},
# don't insert if no document found
upsert=False,
array_filters=None
)
查询后的数据库:
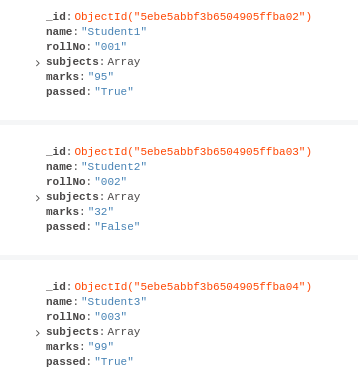
示例 2:将名为地址的新字段添加到所有文档
Python
from pymongo import MongoClient
# Creating an instance of MongoClient
# on default localhost
client = MongoClient('mongodb://localhost:27017')
# Accessing desired database and collection
db = client.gfg
collection = db["classroom"]
# Address filed to be added to all documents
collection.update_many(
{},
{"$set":
{
"Address": "value"
}
},
# don't insert if no document found
upsert=False,
array_filters=None
)
数据库查询后:
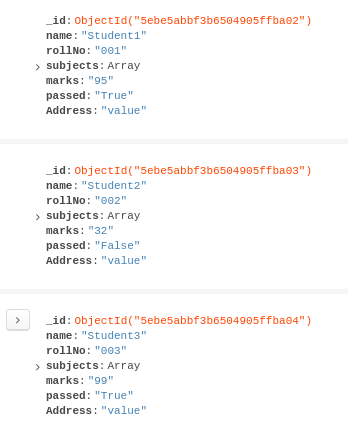