使用 Monte Carlo 估计 Pi 的值 |并行计算方法
给定两个整数N和K ,表示并行处理中的试验次数和总线程数。任务是使用 Monte Carlo 算法找到 PI 的估计值,该算法使用并行化程序部分的开放式多处理 (OpenMP) 技术。
例子:
Input: N = 100000, K = 8
Output: Final Estimation of Pi = 3.146600
Input: N = 10, K = 8
Output: Final Estimation of Pi = 3.24
Input: N = 100, K = 8
Output: Final Estimation of Pi = 3.0916
方法:上面给出的使用蒙特卡洛估计 Pi 值的问题已经使用标准算法解决了。这里的想法是使用 OpenMp 的并行计算来解决问题。请按照以下步骤解决问题:
- 初始化 3 个变量,例如x、y和d ,以存储随机点的X和Y坐标以及随机点到原点的距离的平方。
- 初始化 2 个变量,例如pCircle和pSquare ,其值为0以存储位于半径为 0.5的圆和边长为1的正方形内的点。
- 现在开始使用 OpenMp 和以下部分的 reduction() 进行并行处理:
- 迭代范围[0, N]并在每次迭代中使用srand48()和drand48()找到x和y ,然后找到点 ( x, y)与原点的距离的平方,然后如果距离小于或等于到1然后将pCircle增加1 。
- 在上述步骤的每次迭代中,将pSquare的计数增加1 。
- 最后,经过上述步骤计算估计的 pi 的值如下,然后打印得到的值。
- Pi = 4.0 * ((double)pCircle / (double)(pSquare))
下面是上述方法的实现:
C++
// C++ program for the above approach
#include
using namespace std;
// Function to find estimated
// value of PI using Monte
// Carlo algorithm
void monteCarlo(int N, int K)
{
// Stores X and Y coordinates
// of a random point
double x, y;
// Stores distance of a random
// point from origin
double d;
// Stores number of points
// lying inside circle
int pCircle = 0;
// Stores number of points
// lying inside square
int pSquare = 0;
int i = 0;
// Parallel calculation of random
// points lying inside a circle
#pragma omp parallel firstprivate(x, y, d, i) reduction(+ : pCircle, pSquare) num_threads(K)
{
// Initializes random points
// with a seed
srand48((int)time(NULL));
for (i = 0; i < N; i++)
{
// Finds random X co-ordinate
x = (double)drand48();
// Finds random X co-ordinate
y = (double)drand48();
// Finds the square of distance
// of point (x, y) from origin
d = ((x * x) + (y * y));
// If d is less than or
// equal to 1
if (d <= 1)
{
// Increment pCircle by 1
pCircle++;
}
// Increment pSquare by 1
pSquare++;
}
}
// Stores the estimated value of PI
double pi = 4.0 * ((double)pCircle / (double)(pSquare));
// Prints the value in pi
cout << "Final Estimation of Pi = "<< pi;
}
// Driver Code
int main()
{
// Input
int N = 100000;
int K = 8;
// Function call
monteCarlo(N, K);
}
// This code is contributed by shivanisinghss2110
C
// C program for the above approach
#include
#include
#include
#include
// Function to find estimated
// value of PI using Monte
// Carlo algorithm
void monteCarlo(int N, int K)
{
// Stores X and Y coordinates
// of a random point
double x, y;
// Stores distance of a random
// point from origin
double d;
// Stores number of points
// lying inside circle
int pCircle = 0;
// Stores number of points
// lying inside square
int pSquare = 0;
int i = 0;
// Parallel calculation of random
// points lying inside a circle
#pragma omp parallel firstprivate(x, y, d, i) reduction(+ : pCircle, pSquare) num_threads(K)
{
// Initializes random points
// with a seed
srand48((int)time(NULL));
for (i = 0; i < N; i++) {
// Finds random X co-ordinate
x = (double)drand48();
// Finds random X co-ordinate
y = (double)drand48();
// Finds the square of distance
// of point (x, y) from origin
d = ((x * x) + (y * y));
// If d is less than or
// equal to 1
if (d <= 1) {
// Increment pCircle by 1
pCircle++;
}
// Increment pSquare by 1
pSquare++;
}
}
// Stores the estimated value of PI
double pi = 4.0 * ((double)pCircle / (double)(pSquare));
// Prints the value in pi
printf("Final Estimation of Pi = %f\n", pi);
}
// Driver Code
int main()
{
// Input
int N = 100000;
int K = 8;
// Function call
monteCarlo(N, K);
}
输出
Final Estimation of Pi = 3.146600
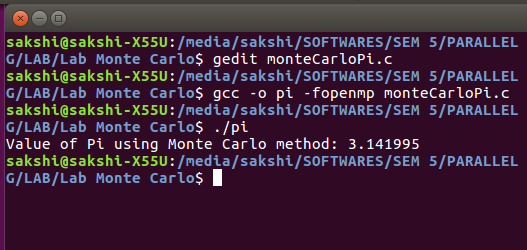
上述C程序的输出
时间复杂度: O(N*K)
辅助空间: O(1)