Tkinter 中的 RadioButton | Python
Radiobutton是一个标准的 Tkinter 小部件,用于实现多选之一。单选按钮可以包含文本或图像,您可以将Python函数或方法与每个按钮相关联。当按下按钮时,Tkinter 会自动调用该函数或方法。
句法:
button = Radiobutton(master, text=”Name on Button”, variable = “shared variable”, value = “values of each button”, options = values, …)
shared variable = A Tkinter variable shared among all Radio buttons
value = each radiobutton should have different value otherwise more than 1 radiobutton will get selected.
代码#1:
单选按钮,但不是按钮形式,按钮框形式。为了显示按钮框, indicatoron/indicator选项应该设置为 0。
Python3
# Importing Tkinter module
from tkinter import *
# from tkinter.ttk import *
# Creating master Tkinter window
master = Tk()
master.geometry("175x175")
# Tkinter string variable
# able to store any string value
v = StringVar(master, "1")
# Dictionary to create multiple buttons
values = {"RadioButton 1" : "1",
"RadioButton 2" : "2",
"RadioButton 3" : "3",
"RadioButton 4" : "4",
"RadioButton 5" : "5"}
# Loop is used to create multiple Radiobuttons
# rather than creating each button separately
for (text, value) in values.items():
Radiobutton(master, text = text, variable = v,
value = value, indicator = 0,
background = "light blue").pack(fill = X, ipady = 5)
# Infinite loop can be terminated by
# keyboard or mouse interrupt
# or by any predefined function (destroy())
mainloop()
Python3
# Importing Tkinter module
from tkinter import *
from tkinter.ttk import *
# Creating master Tkinter window
master = Tk()
master.geometry("175x175")
# Tkinter string variable
# able to store any string value
v = StringVar(master, "1")
# Dictionary to create multiple buttons
values = {"RadioButton 1" : "1",
"RadioButton 2" : "2",
"RadioButton 3" : "3",
"RadioButton 4" : "4",
"RadioButton 5" : "5"}
# Loop is used to create multiple Radiobuttons
# rather than creating each button separately
for (text, value) in values.items():
Radiobutton(master, text = text, variable = v,
value = value).pack(side = TOP, ipady = 5)
# Infinite loop can be terminated by
# keyboard or mouse interrupt
# or by any predefined function (destroy())
mainloop()
Python3
# Importing Tkinter module
from tkinter import *
from tkinter.ttk import *
# Creating master Tkinter window
master = Tk()
master.geometry('175x175')
# Tkinter string variable
# able to store any string value
v = StringVar(master, "1")
# Style class to add style to Radiobutton
# it can be used to style any ttk widget
style = Style(master)
style.configure("TRadiobutton", background = "light green",
foreground = "red", font = ("arial", 10, "bold"))
# Dictionary to create multiple buttons
values = {"RadioButton 1" : "1",
"RadioButton 2" : "2",
"RadioButton 3" : "3",
"RadioButton 4" : "4",
"RadioButton 5" : "5"}
# Loop is used to create multiple Radiobuttons
# rather than creating each button separately
for (text, value) in values.items():
Radiobutton(master, text = text, variable = v,
value = value).pack(side = TOP, ipady = 5)
# Infinite loop can be terminated by
# keyboard or mouse interrupt
# or by any predefined function (destroy())
mainloop()
输出:
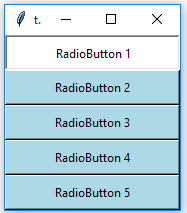
这些按钮框的背景是浅蓝色的。具有白色背景以及凹陷的按钮框被选中。
代码 #2:将按钮框更改为标准单选按钮。对于此删除指示选项。
Python3
# Importing Tkinter module
from tkinter import *
from tkinter.ttk import *
# Creating master Tkinter window
master = Tk()
master.geometry("175x175")
# Tkinter string variable
# able to store any string value
v = StringVar(master, "1")
# Dictionary to create multiple buttons
values = {"RadioButton 1" : "1",
"RadioButton 2" : "2",
"RadioButton 3" : "3",
"RadioButton 4" : "4",
"RadioButton 5" : "5"}
# Loop is used to create multiple Radiobuttons
# rather than creating each button separately
for (text, value) in values.items():
Radiobutton(master, text = text, variable = v,
value = value).pack(side = TOP, ipady = 5)
# Infinite loop can be terminated by
# keyboard or mouse interrupt
# or by any predefined function (destroy())
mainloop()
输出:
这些单选按钮是使用tkinter.ttk创建的,这就是为什么背景选项不可用但我们可以使用样式类来进行样式设置的原因。代码#3:使用样式类向单选按钮添加样式。
Python3
# Importing Tkinter module
from tkinter import *
from tkinter.ttk import *
# Creating master Tkinter window
master = Tk()
master.geometry('175x175')
# Tkinter string variable
# able to store any string value
v = StringVar(master, "1")
# Style class to add style to Radiobutton
# it can be used to style any ttk widget
style = Style(master)
style.configure("TRadiobutton", background = "light green",
foreground = "red", font = ("arial", 10, "bold"))
# Dictionary to create multiple buttons
values = {"RadioButton 1" : "1",
"RadioButton 2" : "2",
"RadioButton 3" : "3",
"RadioButton 4" : "4",
"RadioButton 5" : "5"}
# Loop is used to create multiple Radiobuttons
# rather than creating each button separately
for (text, value) in values.items():
Radiobutton(master, text = text, variable = v,
value = value).pack(side = TOP, ipady = 5)
# Infinite loop can be terminated by
# keyboard or mouse interrupt
# or by any predefined function (destroy())
mainloop()
输出:
您可能会观察到字体样式发生了变化,背景和前景色也发生了变化。在这里,TRAdiobutton 用于样式类,它会自动将样式应用于所有可用的 Radiobuttons。