SciPy - 空间距离矩阵
距离矩阵包含在矩阵/矩阵的向量之间成对计算的距离。 scipy.spatial包为我们提供了distance_matrix()方法来计算距离矩阵。通常矩阵是二维数组的形式,矩阵的向量是矩阵行(一维数组)。
Syntax: scipy.spatial.distance_matrix(x, y, p=2)
Parameters:
x : (M, K) Matrix of M vectors, each of dimension K.
y : (N, K) Matrix of N vectors, each of dimension K.
p : float, 1 <= p <= infinity, defines which Minkowski p-norm to use.
Reurns: (M, N) ndarray
/ matrix containing the distance from every vector in x to every vector in y.
注意: x和y矩阵的列尺寸必须相同。
我们可以使用不同的p值来应用不同类型的距离来计算距离矩阵。
p = 1, Manhattan Distance
p = 2, Euclidean Distance
p = ∞, Chebychev Distance
示例 1。
我们计算两个矩阵x和y的距离矩阵。两个矩阵都具有相同的维度 (3, 2)。所以距离矩阵的维数是 (3,3)。使用 p=2,距离计算为 Minkowski 2-范数(或欧几里得距离)。
Python3
# Python program to compute distance matrix
# import important libraries
import numpy as np
from scipy.spatial import distance_matrix
# Create the matrices
x = np.array([[1,2],[2,1],[2,2]])
y = np.array([[5,0],[1,2],[2,0]])
# Display the matrices
print("matrix x:\n", x)
print("matrix y:\n", y)
# compute the distance matrix
dist_mat = distance_matrix(x, y, p=2)
# display distance matrix
print("Distance Matrix:\n", dist_mat)
Python3
# Python program to compute distance matrix
# import important libraries
import numpy as np
from scipy.spatial import distance_matrix
# Create the matrices
x = np.array([[1,2],[2,1],[2,2]])
y = np.array([[0,0],[0,0],[1,1],[1,1],[1,2]])
# Display the matrices
print("matrix x:\n", x)
print("matrix y:\n", y)
# compute the distance matrix
dist_mat = distance_matrix(x, y, p=2)
# display distance matrix
print("Distance Matrix:\n", dist_mat)
Python3
# Python program to compute distance matrix
# import important libraries
import numpy as np
from scipy.spatial import distance_matrix
# Create the matrix
x = np.array([[1,2],[2,1],[2,2]])
# Display the matrix
print("matrix x:\n", x)
# compute the distance matrix
dist_mat = distance_matrix(x, x, p=2)
# display distance matrix
print("Distance Matrix:\n", dist_mat)
Python3
# Python program to compute distance matrix
# import important libraries
import numpy as np
from scipy.spatial import distance_matrix
# Create the matrices
x = np.array([[1,2],[2,1],[2,2]])
y = np.array([[5,0],[1,2],[2,0]])
# Display the matrices
print("matrix x:\n", x)
print("matrix y:\n", y)
# compute the distance matrix
dist_mat = distance_matrix(x, y, p=1)
# display distance matrix
print("Distance Matrix:\n", dist_mat)
Python3
# Python program to compute distance matrix
# import important libraries
import numpy as np
from scipy.spatial import distance_matrix
# Create the matrices
x = np.array([[1,2,3,4,5],[2,1,0,3,4]])
y = np.array([[0,0,0,0,1],[1,1,1,1,2]])
# Display the matrices
print("matrix x:\n", x)
print("matrix y:\n", y)
# compute the distance matrix
dist_mat = distance_matrix(x, y, p=2)
# display distance matrix
print("Distance Matrix:\n", dist_mat)
输出:
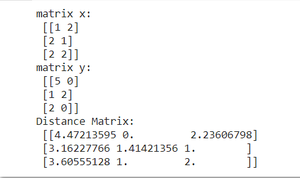
距离矩阵示例 1
示例 2。
我们计算两个矩阵x和y的距离矩阵。两个矩阵都有不同的维度。矩阵x 的维度为 (3,2),矩阵y 的维度为 (5,2)。所以距离矩阵的维数为 (3,5)。
蟒蛇3
# Python program to compute distance matrix
# import important libraries
import numpy as np
from scipy.spatial import distance_matrix
# Create the matrices
x = np.array([[1,2],[2,1],[2,2]])
y = np.array([[0,0],[0,0],[1,1],[1,1],[1,2]])
# Display the matrices
print("matrix x:\n", x)
print("matrix y:\n", y)
# compute the distance matrix
dist_mat = distance_matrix(x, y, p=2)
# display distance matrix
print("Distance Matrix:\n", dist_mat)
输出:
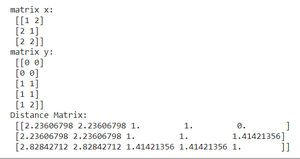
距离矩阵示例 2
例 3。
我们使用单个矩阵(即x )计算距离矩阵。矩阵x具有维度 (3,2)。相同的矩阵x作为参数y 给出。距离矩阵的维度为 (3,3)。
蟒蛇3
# Python program to compute distance matrix
# import important libraries
import numpy as np
from scipy.spatial import distance_matrix
# Create the matrix
x = np.array([[1,2],[2,1],[2,2]])
# Display the matrix
print("matrix x:\n", x)
# compute the distance matrix
dist_mat = distance_matrix(x, x, p=2)
# display distance matrix
print("Distance Matrix:\n", dist_mat)
输出:
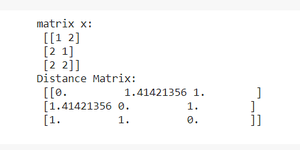
距离矩阵示例 3
注意:注意上面的距离矩阵是一个对称矩阵。当x和y矩阵相同时,距离矩阵是对称矩阵。
例 4。
我们计算两个矩阵x和y的距离矩阵。两个矩阵都有不同的维度。矩阵x 的维度为 (3,2),矩阵y 的维度为 (5,2)。所以距离矩阵的维数为 (3,5)。使用 p=1,距离计算为 Minkowski 1-范数(或曼哈顿距离)。
蟒蛇3
# Python program to compute distance matrix
# import important libraries
import numpy as np
from scipy.spatial import distance_matrix
# Create the matrices
x = np.array([[1,2],[2,1],[2,2]])
y = np.array([[5,0],[1,2],[2,0]])
# Display the matrices
print("matrix x:\n", x)
print("matrix y:\n", y)
# compute the distance matrix
dist_mat = distance_matrix(x, y, p=1)
# display distance matrix
print("Distance Matrix:\n", dist_mat)
输出:
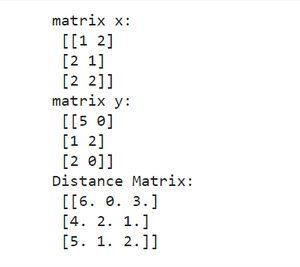
距离矩阵示例 4
例 5。
我们计算两个矩阵x和y的距离矩阵。两个矩阵都有维度 (2, 5)。所以距离矩阵的维数为 (3,5)。使用 p=2,距离计算为 Minkowski 2-范数(或欧几里得距离)。
蟒蛇3
# Python program to compute distance matrix
# import important libraries
import numpy as np
from scipy.spatial import distance_matrix
# Create the matrices
x = np.array([[1,2,3,4,5],[2,1,0,3,4]])
y = np.array([[0,0,0,0,1],[1,1,1,1,2]])
# Display the matrices
print("matrix x:\n", x)
print("matrix y:\n", y)
# compute the distance matrix
dist_mat = distance_matrix(x, y, p=2)
# display distance matrix
print("Distance Matrix:\n", dist_mat)
输出:
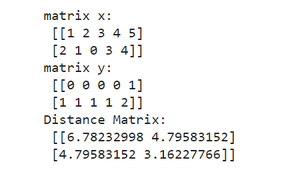
距离矩阵示例 5