你想计算百分比吗?如果是,那么您可以使用 HTML、CSS 和 JavaScript 制作一个简单的百分比计算器。在本文中,您必须输入在第一个输入字段中获得的分数和在第二个输入字段中获得的总分以计算百分比。输入值并单击“计算”按钮后,可以获得百分比。这个非常有用的项目用于计算分数、折扣等。百分比计算器对学生、店主以及解决与百分比相关的基本数学问题很有用。
文件结构
- 索引.html
- 样式文件
- 脚本.js
先决条件:需要 HTML、CSS 和 JavaScript 的基本知识。该项目包含 HTML、CSS 和 JavaScript 文件。 HTML 文件添加结构,然后使用 CSS 设置样式,JavaScript 为其添加功能并对项目的某些部分进行验证。
HTML 布局是使用 div 标签、id 属性、类、表单和用于提交表单的按钮创建的。它定义了网页的结构。
index.html
Percentage Calculator
Percentage Calculator
What is % of
?
is what Percent of
?
style.css
/* A margin and padding are provided 0
box-sizing border box is used to include
padding and border in the total width
and height of the element, and font-family
can be specified by the user */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Courier New', Courier, monospace;
}
/* The user display allows you to specify the
background colour and height. The
display:flex property, which is aligned at the
centre, is used to fill available free space
or to shrink them to prevent overflow. */
body {
background-color: #f7f7f7;
min-height: 100vh;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
/* font-weight Specifies weight of glyphs
in the font, their degree of blackness or
stroke */
h1 {
font-weight: 900;
margin-bottom: 12px;
}
div {
width: 480px;
background-color: #fff;
margin: 12px 0;
padding: 24px;
text-align: center;
box-shadow: 2px 2px 8px 2px #aaa;
}
input[type=number] {
width: 84px;
font-size: 18px;
padding: 8px;
margin: 0px 8px 8px 8px;
}
/* The text-transform:uppercase property
causes characters to be raised to uppercase.
The button's font-weight, font-size, and
cursor type can be customised by the user. */
button {
text-transform: uppercase;
font-weight: 900;
font-size: 20px;
margin: 12px 0;
padding: 8px;
cursor: pointer;
letter-spacing: 1px;
}
/* The font-weight, font-size, background-color,
and border can all be customized by the user.
The border-radius property allows you to give
an element rounded corners.*/
input[type=text] {
font-size: 22px;
padding: 8px 0;
font-weight: 900;
text-align: center;
background-color: #f7f7f7;
border: 2px solid #ccc;
border-radius: 6px;
}
script.js
function percentage_1() {
// Method returns the element of percent id
var percent = document.getElementById("percent").value;
// Method returns the element of num id
var num = document.getElementById("num").value;
document.getElementById("value1")
.value = (num / 100) * percent;
}
function percentage_2() {
// Method returns the element of num1 id
var num1 = document.getElementById("num1").value;
// Method returns the elements of num2 id
var num2 = document.getElementById("num2").value;
document.getElementById("value2")
.value = (num1 * 100) / num2 + "%";
}
通过使用 CSS 属性,我们将使用根据项目要求给出的颜色、宽度、高度和位置属性来装饰网页。 CSS 设计了计算器的界面。
样式文件
/* A margin and padding are provided 0
box-sizing border box is used to include
padding and border in the total width
and height of the element, and font-family
can be specified by the user */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Courier New', Courier, monospace;
}
/* The user display allows you to specify the
background colour and height. The
display:flex property, which is aligned at the
centre, is used to fill available free space
or to shrink them to prevent overflow. */
body {
background-color: #f7f7f7;
min-height: 100vh;
display: flex;
justify-content: center;
align-items: center;
flex-direction: column;
}
/* font-weight Specifies weight of glyphs
in the font, their degree of blackness or
stroke */
h1 {
font-weight: 900;
margin-bottom: 12px;
}
div {
width: 480px;
background-color: #fff;
margin: 12px 0;
padding: 24px;
text-align: center;
box-shadow: 2px 2px 8px 2px #aaa;
}
input[type=number] {
width: 84px;
font-size: 18px;
padding: 8px;
margin: 0px 8px 8px 8px;
}
/* The text-transform:uppercase property
causes characters to be raised to uppercase.
The button's font-weight, font-size, and
cursor type can be customised by the user. */
button {
text-transform: uppercase;
font-weight: 900;
font-size: 20px;
margin: 12px 0;
padding: 8px;
cursor: pointer;
letter-spacing: 1px;
}
/* The font-weight, font-size, background-color,
and border can all be customized by the user.
The border-radius property allows you to give
an element rounded corners.*/
input[type=text] {
font-size: 22px;
padding: 8px 0;
font-weight: 900;
text-align: center;
background-color: #f7f7f7;
border: 2px solid #ccc;
border-radius: 6px;
}
为了向网页添加功能,使用了 JavaScript 代码。在这种情况下,声明了两个函数,百分比 1() 和百分比 2(),并通过 onclick 事件传递给按钮。因此,当按下计算按钮时,就会显示结果。我们使用了 document.getElementById() 方法。它返回具有指定 ID 属性的元素,如果不存在具有指定 ID 的元素,则返回 null。
使用的公式:
What is X percent of Y is given by the formula:
X percent of Y =(X/100)* Y
And X is what percent of Y is given by the formula:
X is what percent of Y= (X/Y)×100%, where X, Y > 0
脚本.js
function percentage_1() {
// Method returns the element of percent id
var percent = document.getElementById("percent").value;
// Method returns the element of num id
var num = document.getElementById("num").value;
document.getElementById("value1")
.value = (num / 100) * percent;
}
function percentage_2() {
// Method returns the element of num1 id
var num1 = document.getElementById("num1").value;
// Method returns the elements of num2 id
var num2 = document.getElementById("num2").value;
document.getElementById("value2")
.value = (num1 * 100) / num2 + "%";
}
输出:
- 首先-
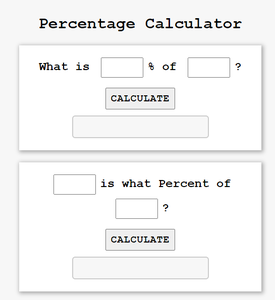
百分比计算器
- 输入一些数据并单击计算按钮后-
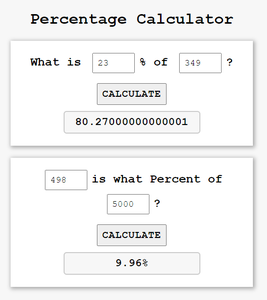
百分比计算器