给定一个具有V个顶点和E 个边的无向图,任务是打印所有独立集并找到最大独立集。
Independent set is a set of vertices such that any two vertices in the set do not have a direct edge between them.
Maximal independent set is an independent set having highest number of vertices.
注意:对于给定的图形,可以有多个独立和最大独立集。
例子:
Input:
V = 3, E = 0
Graph:
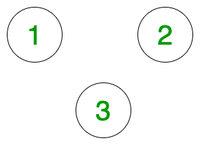
Graph for example 1
Output:
{ }{ 1 }{ 1 2 }{ 1 2 3 }{ 1 3 }{ 2 }{ 2 3 }{ 3 }
{ 1 2 3 }
Explanation:
The first line represents all the possible independent sets for the given graph. The second line has the maximal independent sets possible for the given graph.
Input:
V = 4, E = 4
Graph:
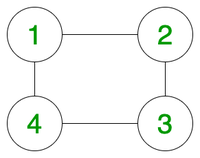
Graph for example 2
Output:
{ }{ 1 }{ 1 3 }{ 2 }{ 2 4 }{ 3 }{ 4 }
{ 1 3 }{ 2 4 }
方法:
这个想法是使用回溯来解决问题。在每一步,我们都需要检查当前节点是否与我们的独立集合中已经存在的任何节点有任何直接边。如果没有,我们可以将其添加到我们的独立集合中,并对所有节点递归重复相同的过程。
Illustration:
Recursion tree for the first example:
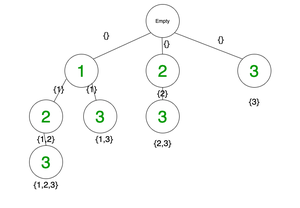
Backtracking tree for all possible sets
In the above backtracking tree we will choose only those sets which are produced after adding a safe node to maintain the set as an independent set.
下面是上述方法的实现:
CPP
// C++ Program to print the
// independent sets and
// maximal independent sets
// of the given graph
#include
using namespace std;
// To store all the independent
// sets of the graph
set > independentSets;
// To store all maximal independent
// sets in the graph
set > maximalIndependentSets;
map, int> edges;
vector vertices;
// Function to print all independent sets
void printAllIndependentSets()
{
for (auto iter : independentSets) {
cout << "{ ";
for (auto iter2 : iter) {
cout << iter2 << " ";
}
cout << "}";
}
cout << endl;
}
// Function to extract all
// maximal independent sets
void printMaximalIndependentSets()
{
int maxCount = 0;
int localCount = 0;
for (auto iter : independentSets) {
localCount = 0;
for (auto iter2 : iter) {
localCount++;
}
if (localCount > maxCount)
maxCount = localCount;
}
for (auto iter : independentSets) {
localCount = 0;
set tempMaximalSet;
for (auto iter2 : iter) {
localCount++;
tempMaximalSet.insert(iter2);
}
if (localCount == maxCount)
maximalIndependentSets
.insert(tempMaximalSet);
}
for (auto iter : maximalIndependentSets) {
cout << "{ ";
for (auto iter2 : iter) {
cout << iter2 << " ";
}
cout << "}";
}
cout << endl;
}
// Function to check if a
// node is safe node.
bool isSafeForIndependentSet(
int vertex,
set tempSolutionSet)
{
for (auto iter : tempSolutionSet) {
if (edges[make_pair(iter, vertex)]) {
return false;
}
}
return true;
}
// Recursive function to find
// all independent sets
void findAllIndependentSets(
int currV,
int setSize,
set tempSolutionSet)
{
for (int i = currV; i <= setSize; i++) {
if (isSafeForIndependentSet(
vertices[i - 1],
tempSolutionSet)) {
tempSolutionSet
.insert(vertices[i - 1]);
findAllIndependentSets(
i + 1,
setSize,
tempSolutionSet);
tempSolutionSet
.erase(vertices[i - 1]);
}
}
independentSets
.insert(tempSolutionSet);
}
// Driver Program
int main()
{
int V = 3, E = 0;
for (int i = 1; i <= V; i++)
vertices.push_back(i);
vector > inputEdges;
pair edge;
int x, y;
for (int i = 0; i < E; i++) {
edge.first = inputEdges[i].first;
edge.second = inputEdges[i].second;
edges[edge] = 1;
int t = edge.first;
edge.first = edge.second;
edge.second = t;
edges[edge] = 1;
}
set tempSolutionSet;
findAllIndependentSets(1,
V,
tempSolutionSet);
printAllIndependentSets();
printMaximalIndependentSets();
return 0;
}
{ }{ 1 }{ 1 2 }{ 1 2 3 }{ 1 3 }{ 2 }{ 2 3 }{ 3 }
{ 1 2 3 }