GUI 工具包包含用于创建图形界面的小部件。 Python包括各种可用的接口实现,从 TkInter(它与Python)到各种跨平台解决方案,例如 PyQt5,它以其更复杂的小部件和时尚的外观而闻名。
PyQt
PyQt 是用于图形用户界面 (GUI) 小部件的工具包。它是从 Qt 库中提取的。 PyQt 是Python语言和 Qt 库结合的产物。 PyQt 与 Qt Builder 一起提供。我们将使用它从 Qt Creator 获取Python代码。在qt设计器的支持下,我们可以构建一个GUI,然后我们可以获得该GUI的Python代码。 PyQt 支持所有平台,包括 Windows、macOS 和 UNIX。 PyQt 可用于创建时尚的 GUI,这是一个现代且可移植的Python框架。
代码:
Python3
# Import sys for handle the
# exit status of the application.
import sys
# Importing required widgets
from PyQt5.QtWidgets import QApplication
from PyQt5.QtWidgets import QLabel
from PyQt5.QtWidgets import QWidget
# To create an instance of QApplication
# sys.argv contains the list of
# command-line argument
app = QApplication(sys.argv)
# To create an instance of application GUI
# root is an instance of QWidget,
# it provides all the features to
# create the application's window
root = QWidget()
# adding title to window
root.setWindowTitle('Geeks App')
# to place txt at the coordinates
root.move(60, 15)
# to display text
txt = QLabel('Welcome, Geeks!', parent = root)
txt.move(60, 15)
# Show application's GUI
root.show()
# Run application's main loop
sys.exit(app.exec_())
Python3
# importing the module tkinter
import tkinter as tk
# create main window (parent window)
root = tk.Tk()
# Label() it display box
# where you can put any text.
txt = tk.Label(root,
text="Welcome to GeekForGeeks")
# pack() It organizes the widgets
# in blocks before placing in the parent widget.
txt.pack()
# running the main loop
root.mainloop()
输出:
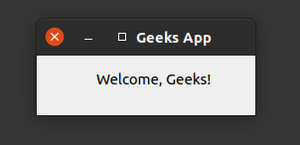
一个使用 PyQt 显示文本的简单应用程序。
使用 PyQt 的优势
- 编码多功能性——使用 Qt 进行 GUI 编程是围绕信号和插槽的思想构建的,用于在对象之间创建联系。这允许处理 GUI 事件的多功能性,从而产生更流畅的代码库。
- 不仅仅是一个框架:Qt 使用各种原生平台 API 来进行网络、数据库开发等。它通过特殊的 API 提供对它们的主要访问。
- 各种 UI 组件:Qt 提供了多个小部件,例如按钮或菜单,所有这些小部件都设计有适用于所有兼容平台的基本界面。
- 各种学习资源:由于 PyQt 是Python最常用的 UI 系统之一,您可以方便地访问各种文档。
使用 PyQt 的缺点
- PyQt5 中的类缺少 Python 特定的文档
- 掌握 PyQt 的所有细节需要花费大量时间,这意味着它的学习曲线非常陡峭。
- 如果应用程序不是开源的,您必须支付商业许可费用。
特金特
Tkinter 是一个开源Python图形用户界面 (GUI) 库,以其简单性而闻名。它预先安装在Python,因此您甚至不需要考虑安装它。这些特点使其成为初学者和中级初学者的有利位置。 Tkinter 不能用于大型项目。
代码:
蟒蛇3
# importing the module tkinter
import tkinter as tk
# create main window (parent window)
root = tk.Tk()
# Label() it display box
# where you can put any text.
txt = tk.Label(root,
text="Welcome to GeekForGeeks")
# pack() It organizes the widgets
# in blocks before placing in the parent widget.
txt.pack()
# running the main loop
root.mainloop()
输出:
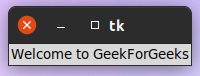
一个使用 tkinter 显示文本的简单应用程序。
使用 Tkinter 的优势
- 与任何其他 GUI 工具包相比,Tkinter 实现起来既简单又快速。
- Tkinter 更加灵活和稳定。
- Tkinter 包含在Python,因此无需额外下载。
- Tkinter 提供了一个简单的语法。
- Tkinter 真的很容易理解和掌握。
- Tkinter 提供了三种几何管理器:放置、打包和网格。这更强大且易于使用。
使用 Tkinter 的缺点
- Tkinter 不包括高级小部件。
- 它没有与 Qt Designer for Tkinter 类似的工具。
- 它没有可靠的用户界面。
- 有时,在 Tkinter 中很难调试。
- 它不是纯粹的 Pythonic。
PyQt 和 Tkinter 的区别
No. | Basis |
PyQt |
Tkinter |
---|---|---|---|
1. | License | PyQt is available under Riverbank Commercial License and GPL v3 (General Public License v 3.0) and if you do not wish to release your application under a GPL-compatible license, you must apply for a commercial license. | Tkinter is open source and free for any commercial use. |
2. | Ease of Understanding | It requires a lot of time for understanding all the details of PyQt. | Tkinter is easy to understand and master due to a small library. |
3. | Design | PyQt has a modern look and a good UI. | Tk had an older design and it looks outdated. |
4. | Widgets | thenPyQt comes with many powerful and advanced widgets. | TkInter does not come with advanced widgets. |
5. | UI Builder | PyQt have a Qt Designer tool which we can use to build GUIs than get python code of that GUI using Qt Designer. | It has no similar tool as Qt Designer for Tkinter. |
6. | Installation | PyQt is not included by default with Python installations. | It is included in the standard Python library so no need to install it separately. |