Spring – RestTemplate
由于高流量和对服务的快速访问,REST API 越来越受欢迎。 REST 不是一种协议或一种标准方式,而是一组架构约束。它也称为 RESTful API 或 Web API。当发出客户端请求时,它只是将资源状态的表示通过 HTTP 传输给请求者或端点。传递给客户的信息可以采用以下几种格式:
- JSON(JavaScript 对象表示法)
- XML
- HTML
- XLT
- Python
- PHP
- 纯文本
Pre-requisites: The ‘Client’ can be of any front-end framework like Angular, React for reasons like developing Single Page Application (SPA ), etc. or it can be a back-end internal/external Spring application itself.
为了与 REST 交互,客户端需要创建客户端实例和请求对象,执行请求,解释响应,将响应映射到域对象,以及处理异常。 Spring 框架通常会创建 API 并使用内部或外部应用程序的 API。这一优势也有助于我们开发微服务。为了避免这种样板代码,Spring 提供了一种使用 REST API 的便捷方式——通过“RestTemplate”。
使用 REST API 如下:
“RestTemplate”是核心 Spring Framework 提供的同步 REST 客户端。
小路:
org.springframework.web.client.RestTemplate
构造函数:
- RestTemplate()
- RestTemplate(ClientHttpRequestFactory requestFactory)
- RestTemplate(List> messageConverters)
它总共提供了 41 种与 REST 资源交互的方法。但是只有十几个独特的方法,每个都被重载以形成一整套 41 个方法。 Operation Method Action Performed DELETE GET getForEntity() getForObject() Sends an HTTP GET request, returning a ResponseEntity containing an object mapped from the response body. Sends an HTTP GET request, returning an object mapped from a response body. POST postForEntity() postForLocation() postForObject() POSTs data to a URL, returning a ResponseEntity containing an object mapped from the response body. POSTs data to a URL, returning the URL of the newly created resource. POSTs data to a URL, returning an object mapped from the response body. PUT PATCH HEAD ANY exchange() execute() Executes a specified HTTP method against a URL, returning a ResponseEntity containing an object. Executes a specified HTTP method against a URL, returning an object mapped from the response body. OPTIONSdelete() Performs an HTTP DELETE request on a resource at a specified URL. put() PUTs resource data to the specified URL. patchForObject() Sends an HTTP PATCH request, returning the resulting object mapped from the response body. headForHeaders() Sends an HTTP HEAD request, returning the HTTP headers for the specified resource URL. optionsForAllow() – Sends an HTTP OPTIONS request, returning the Allow header for the specified URL.
除了 TRACE,RestTemplate 对每个标准 HTTP 方法都至少有一个方法。 execute() 和 exchange() 为使用任何 HTTP 方法发送请求提供了较低级别的通用方法。上述大多数方法都以这 3 种形式重载:
- 一种接受带有在变量参数列表中指定的 URL 参数的字符串 URL 规范。
- 一个接受带有在 Map
中指定的 URL 参数的字符串 URL 规范。 - 一种接受Java.net.URI 作为 URL 规范,不支持参数化 URL。
为了使用 RestTemplate,我们可以通过如下所示创建一个实例:
RestTemplate rest = new RestTemplate();
此外,您可以将其声明为 bean 并注入它,如下所示:
// Annotation
@Bean
// Method
public RestTemplate restTemplate()
{
return new RestTemplate();
}
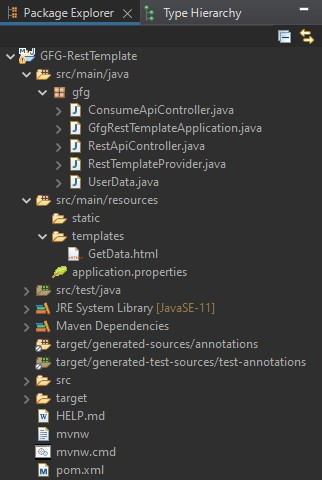
项目结构——Maven
文件: pom.xml (配置)
XML
4.0.0
org.springframework.boot
spring-boot-starter-parent
2.6.3
sia
GFG-RestTemplate
0.0.1-SNAPSHOT
GFG-RestTemplate
Rest-Template
11
org.springframework.boot
spring-boot-starter-thymeleaf
org.springframework.boot
spring-boot-starter-web
org.projectlombok
lombok
true
org.springframework.boot
spring-boot-starter-test
test
org.springframework.boot
spring-boot-starter
org.springframework.boot
spring-boot-devtools
runtime
true
org.springframework.boot
spring-boot-maven-plugin
org.projectlombok
lombok
Java
// Java Program to Illustrate Bootstrapping of Application
package gfg;
// Importing required classes
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
// Annotation
@SpringBootApplication
// Main class
public class GfgRestTemplateApplication {
// Main driver method
public static void main(String[] args)
{
SpringApplication.run(
GfgRestTemplateApplication.class, args);
}
}
Java
package gfg;
import lombok.Data;
@Data
public class UserData {
public String id;
public String userName;
public String data;
}
Java
// Java Program to illustrate Rest Controller REST API
package gfg;
// Importing required classes
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
// Annotation
@RestController
@RequestMapping(path = "/RestApi",
produces = "application/json")
@CrossOrigin(origins = "*")
// Class
public class RestApiController {
@GetMapping("/getData") public UserData get()
{
UserData userData = new UserData();
userData.setId("1");
userData.setUserName("darshanGPawar@geek");
userData.setData("Data send by Rest-API");
return userData;
}
// Annotation
@PostMapping
public ResponseEntity
post(@RequestBody UserData userData)
{
HttpHeaders headers = new HttpHeaders();
return new ResponseEntity<>(userData, headers,
HttpStatus.CREATED);
}
}
Java
// Java Program to Implementation of RestTemplate
package gfg;
// Importing required classes
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
// Class
public class RestTemplateProvider {
// Creating an instance of RestTemplate class
RestTemplate rest = new RestTemplate();
// Method
public UserData getUserData()
{
return rest.getForObject(
"http://localhost:8080/RestApi/getData",
UserData.class);
}
// Method
public ResponseEntity post(UserData user)
{
return rest.postForEntity(
"http://localhost:8080/RestApi", user,
UserData.class, "");
}
}
Java
// Java Program to illustrate Regular Controller
// Consume REST API
package gfg;
// Importing required classes
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
// Annotation
@Controller
@RequestMapping("/Api")
// Class
public class ConsumeApiController {
// Annotation
@GetMapping public String get(Model model)
{
// Creating an instance of RestTemplateProvider
// class
RestTemplateProvider restTemplate
= new RestTemplateProvider();
model.addAttribute("user",
restTemplate.getUserData());
model.addAttribute("model", new UserData());
return "GetData";
}
// Annotation
@PostMapping
public String post(@ModelAttribute("model")
UserData user, Model model)
{
RestTemplateProvider restTemplate = new RestTemplateProvider();
ResponseEntity response = restTemplate.post(user);
model.addAttribute("user", response.getBody());
model.addAttribute("headers",
response.getHeaders() + " "
+ response.getStatusCode());
return "GetData";
}
}
HTML
GFG-REST-TEMPLATE
Hello Geek
Replaceable text
Replaceable text
Replaceable text
文件: GfgRestTemplateApplication。 Java (应用程序的引导)
Java
// Java Program to Illustrate Bootstrapping of Application
package gfg;
// Importing required classes
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
// Annotation
@SpringBootApplication
// Main class
public class GfgRestTemplateApplication {
// Main driver method
public static void main(String[] args)
{
SpringApplication.run(
GfgRestTemplateApplication.class, args);
}
}
A.文件:用户数据。 Java (域类)
- 此类使用 Lombok 库自动生成带有 @Data 注释的 Getter/Setter 方法。
- Lombok 的依赖关系如下图所示:
Maven – pom.xml
org.projectlombok
lombok
true
例子:
Java
package gfg;
import lombok.Data;
@Data
public class UserData {
public String id;
public String userName;
public String data;
}
B. RestApiController。 Java (Rest Controller – REST API)
- GET – 以 JSON 格式返回域数据。
- POST - 返回包装在 ResponseEntity 中的域数据以及标头。
例子:
Java
// Java Program to illustrate Rest Controller REST API
package gfg;
// Importing required classes
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
// Annotation
@RestController
@RequestMapping(path = "/RestApi",
produces = "application/json")
@CrossOrigin(origins = "*")
// Class
public class RestApiController {
@GetMapping("/getData") public UserData get()
{
UserData userData = new UserData();
userData.setId("1");
userData.setUserName("darshanGPawar@geek");
userData.setData("Data send by Rest-API");
return userData;
}
// Annotation
@PostMapping
public ResponseEntity
post(@RequestBody UserData userData)
{
HttpHeaders headers = new HttpHeaders();
return new ResponseEntity<>(userData, headers,
HttpStatus.CREATED);
}
}
C.文件:RestTemplateProvider。 Java (RestTemplate 实现)
- GET – 使用 REST API 的 GET 映射响应并返回域对象。
- POST – 使用 REST API 的 POST 映射响应并返回 ResponseEntity 对象。
例子:
Java
// Java Program to Implementation of RestTemplate
package gfg;
// Importing required classes
import org.springframework.http.ResponseEntity;
import org.springframework.web.client.RestTemplate;
// Class
public class RestTemplateProvider {
// Creating an instance of RestTemplate class
RestTemplate rest = new RestTemplate();
// Method
public UserData getUserData()
{
return rest.getForObject(
"http://localhost:8080/RestApi/getData",
UserData.class);
}
// Method
public ResponseEntity post(UserData user)
{
return rest.postForEntity(
"http://localhost:8080/RestApi", user,
UserData.class, "");
}
}
D.文件:ConsumeApiController。 Java (常规控制器 - 使用 REST API)
使用 RestTemplate 从 REST API 获取数据并相应地更改并返回视图。
Java
// Java Program to illustrate Regular Controller
// Consume REST API
package gfg;
// Importing required classes
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
// Annotation
@Controller
@RequestMapping("/Api")
// Class
public class ConsumeApiController {
// Annotation
@GetMapping public String get(Model model)
{
// Creating an instance of RestTemplateProvider
// class
RestTemplateProvider restTemplate
= new RestTemplateProvider();
model.addAttribute("user",
restTemplate.getUserData());
model.addAttribute("model", new UserData());
return "GetData";
}
// Annotation
@PostMapping
public String post(@ModelAttribute("model")
UserData user, Model model)
{
RestTemplateProvider restTemplate = new RestTemplateProvider();
ResponseEntity response = restTemplate.post(user);
model.addAttribute("user", response.getBody());
model.addAttribute("headers",
response.getHeaders() + " "
+ response.getStatusCode());
return "GetData";
}
}
E.文件:GetData.html(显示结果 - Thymeleaf 模板)
HTML
GFG-REST-TEMPLATE
Hello Geek
Replaceable text
Replaceable text
Replaceable text
输出:依次如下
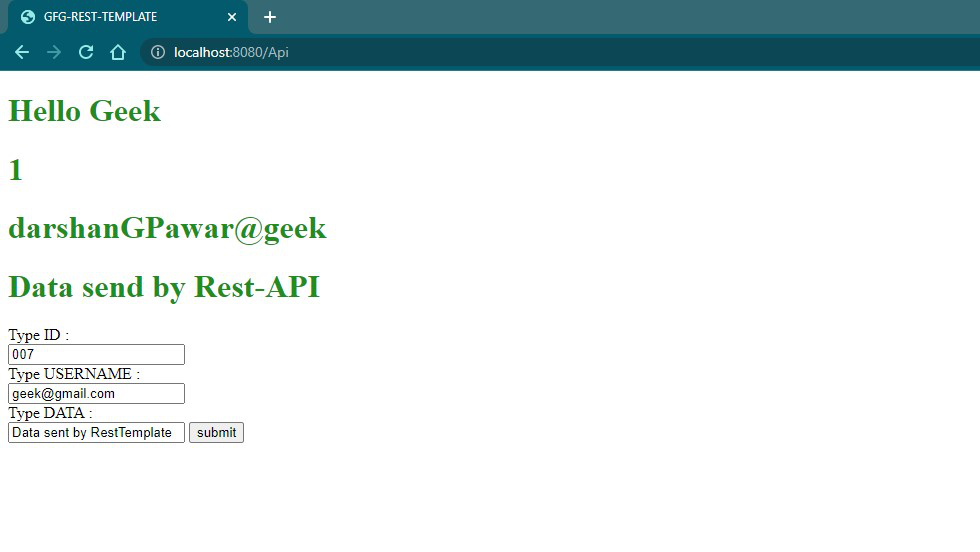
(获取请求)
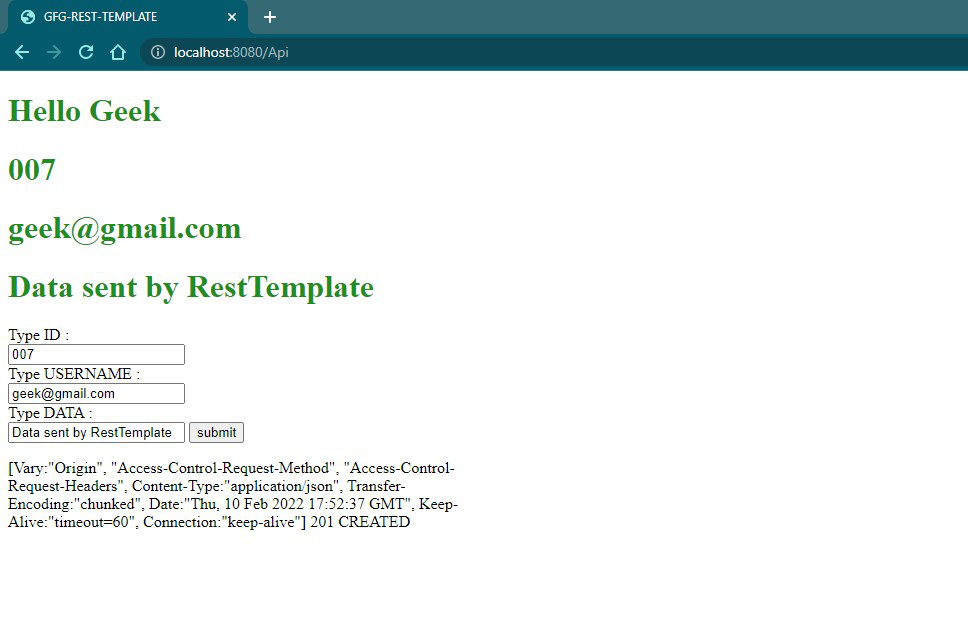
(发布请求)
笔记:
- 当您的后端 Spring 应用程序充当同一个或另一个 Spring 应用程序的 REST API 的客户端时,RestTemplate 使其方便并避免繁琐的工作。
- 在处理 HTTPS URL 时,如果您使用的是自签名证书,则会出现错误。出于开发目的使用 HTTP 会更好。