Spring – MVC 表单下拉列表
在本文中,我们将了解 Spring MVC 下拉列表。我们将在Spring 工具套件(STS)中创建一个基本的 Spring MVC 项目,以使用form:select标签创建一个下拉列表。
spring-form.tld
我们可以使用Java Server Pages (JSP) 作为 Spring Framework 中的视图组件。为了帮助您使用 JSP 实现视图,Spring 框架提供了表单标签库spring-form.tld ,其中包含一些标签,用于评估错误、设置主题、格式化输入和输出国际化消息的字段。
“选择”标签
'select' 是 spring-form.tld 库提供的标签之一。它呈现一个 HTML 'select' 元素并支持对选定选项的数据绑定。我们可以直接使用 select 标签来显示下拉值,也可以使用 option 标签。
以下是“选择”标签中可用的各种属性。
“选择”标签中的属性
A. HTML 标准属性:HTML 标准属性也称为全局属性,可用于所有 HTML 元素。Name Description accesskey To specify a shortcut key to activate/focus on an element. id To specify a unique ID for the element. dir To specify text direction of elements content. lang To specify the language of the content. tabindex To specify tabbing order of an element. title To specify extra information about the element.
B. HTML 事件属性: HTML 事件属性用于在元素上发生特定事件时触发函数。Name Description onblur To execute a javascript function when a user leaves the text field. onchange To execute a javascript function when a user changes the text. onclick To execute a javascript function when the user clicks on the element. ondblclick To execute a javascript function when the user double click on the element. onfocus To execute a javascript function when the user focuses on the text box. onkeydown To execute a javascript function when the user is pressing a key on the keyboard. onkeypress To execute a javascript function when the user presses the key on the keyboard. onkeyup To execute a javascript function when the user is releasing the key on the keyboard. onmousedown To execute a javascript function when the user is pressing a mouse button. onmousemove To execute a javascript function when the user is moving the mouse pointer. onmouseout To execute a javascript function when the user is moving the mouse pointer out of the field. onmouseover To execute a javascript function when the user is moving the mouse pointer onto the field. onmouseup To execute a javascript function when the user is releasing the mouse button.
C. HTML 可选属性:Name Description cssClass To specify a class name for an HTML element to access it. cssErrorClass Used when the bounded element has errors. cssStyle To add styles to an element, such as color, font, size etc. disabled To specify the element to be disabled or not. multiple A boolean attribute to specify user can select multiple values. size To specify the number of visible options in a drop-down list.
D.其他 HTML 属性:Name Description htmlEscape To enable/disable HTML escaping of rendered values itemLabel To specify the name of the property that is mapped to the inner text of the ‘option’ tag. items The Collection, Map or array of objects used to generate the inner ‘option’ tags. itemValue To specify the name of the property mapped to ‘value’ attribute of the ‘option’ tag path To specify the path to the property for binding the data.
Spring MVC 应用程序
我们将创建以下应用程序:
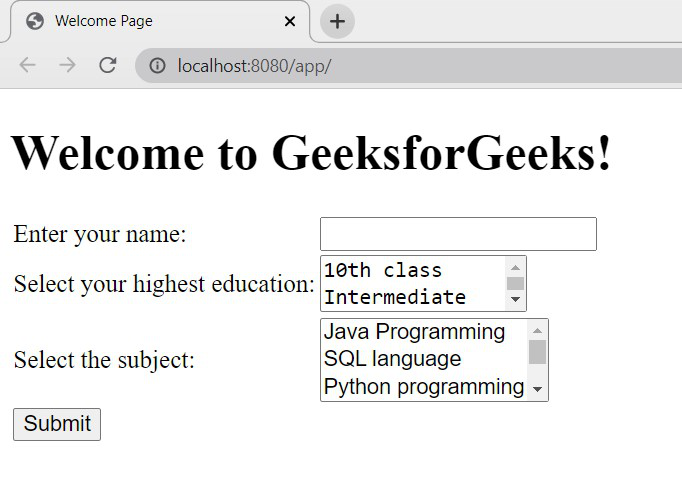
Spring MVC 下拉示例
创建应用程序的步骤
- 在 Spring Tool Suite 中创建一个 Spring MVC 项目。
- 在 STS 中,根据开发人员选择创建项目时,它将下载所有必需的 maven 依赖项、*.jar、lib 文件,并提供嵌入式服务器。
- 下面是 Spring MVC 项目创建后的最终项目结构 *. Java和 *.jsp 文件。
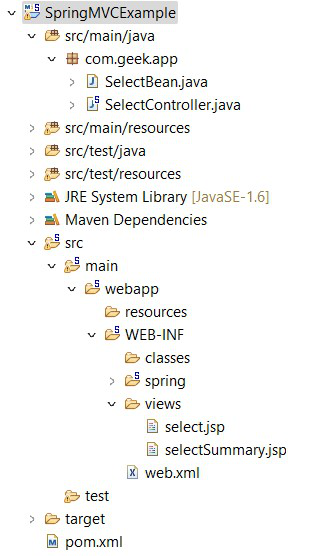
项目结构
实现:要创建的文件如下:
- 选择豆。 Java – Bean 类 – 定义字段属性和属性的 getter/setter 方法。
- 选择控制器。 Java – 控制器类 – 处理用户请求并生成输出。
- select.jsp – 与用户交互的 Jsp 文件以进行输入。
- selectSummary.jsp – Jsp 文件,用于向用户显示处理后的输出。
A.文件:select.jsp
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
Welcome Page
Welcome to GeeksforGeeks!
Enter your name:
Select your highest education:
Select the subject:
Submit
HTML
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
HTML
package com.geek.app;
public class SelectBean {
public String name;
public String education;
public String subject;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEducation() {
return education;
}
public void setEducation(String education) {
this.education = education;
}
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
}
Java
// Java Program to Illustrate SelectController Class
package com.geek.app;
// Importing required classes
import java.util.Arrays;
import java.util.List;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
// Annotation
@Controller
// Class
public class SelectController {
// Annotation
@RequestMapping(value = "/")
public String view(Model model)
{
SelectBean sel = new SelectBean();
model.addAttribute("select", sel);
return "select";
}
// Annotation
@ModelAttribute("educationDetails")
public List educationDetailsList()
{
List educationList = Arrays.asList(
"10th class", "Intermediate", "Graduation",
"Post Graduation");
return educationList;
}
// Annotation
@RequestMapping(value = "/submit",
method = RequestMethod.POST)
public String
submit(@ModelAttribute("select") SelectBean select)
{
return "selectSummary";
}
}
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
Summary page
Hello ${select.name}, details submitted successfully!!
Highest Education:
${select.education}
Subject Selected:
${select.subject}
- 这是应用程序运行时的欢迎页面。
- 要使用 Spring 标签,我们需要在 Jsp 中指定下面的 spring 标签库 URI,
HTML
<%@taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
- 如果你不包含这个标签库,那么容器不理解表单标签,那些将成为它的未知标签。
- 对于字段的正确结构,我们使用 table 标记。
- 正如我们上面讨论的,我们可以只使用选择标签创建一个下拉列表,也可以使用选项标签。
- 通常,当我们单独提供值时,将使用选项标签。
B.文件:SelectBean。Java
HTML
package com.geek.app;
public class SelectBean {
public String name;
public String education;
public String subject;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEducation() {
return education;
}
public void setEducation(String education) {
this.education = education;
}
public String getSubject() {
return subject;
}
public void setSubject(String subject) {
this.subject = subject;
}
}
- 这是定义所有属性以存储值的Java bean
- 以及它们的 getter/setter 方法来获取和设置属性的值。
C.选择控制器。Java
Java
// Java Program to Illustrate SelectController Class
package com.geek.app;
// Importing required classes
import java.util.Arrays;
import java.util.List;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
// Annotation
@Controller
// Class
public class SelectController {
// Annotation
@RequestMapping(value = "/")
public String view(Model model)
{
SelectBean sel = new SelectBean();
model.addAttribute("select", sel);
return "select";
}
// Annotation
@ModelAttribute("educationDetails")
public List educationDetailsList()
{
List educationList = Arrays.asList(
"10th class", "Intermediate", "Graduation",
"Post Graduation");
return educationList;
}
// Annotation
@RequestMapping(value = "/submit",
method = RequestMethod.POST)
public String
submit(@ModelAttribute("select") SelectBean select)
{
return "selectSummary";
}
}
- 这是控制器类,它根据请求 URL 的映射处理方法。
- 这里,@Controller 向容器传达这个类是 spring 控制器类。
- 注释 @RequestMapping 根据提供的值将请求 URL 映射到指定的方法。
- 注解@ModelAttribute,将方法参数或方法返回值绑定到命名模型属性。
D.文件:selectSummary.jsp
HTML
<%@ page language="java" contentType="text/html; charset=ISO-8859-1"
pageEncoding="ISO-8859-1"%>
Summary page
Hello ${select.name}, details submitted successfully!!
Highest Education:
${select.education}
Subject Selected:
${select.subject}
这是输出页面,我们只是在其中显示用户选择的值作为输入。
执行:
- 创建所有必需的Java和 jsp 文件后,在服务器上运行该项目。
- 在项目上,运行方式-> 在服务器上运行。
- 在 localhost 中选择服务器以运行应用程序。
- 在浏览器中打开 URL: http://localhost:8080/app/以获取以下屏幕。
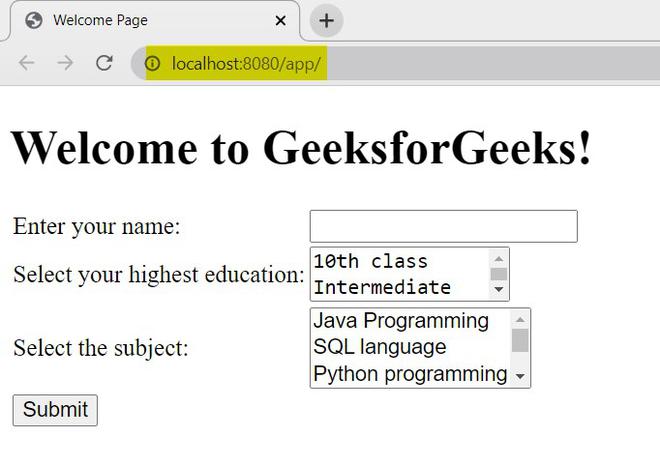
欢迎页面 – select.jsp
- 由于我们为 select 标签指定了不同的属性,因此可以在此处看到该功能。
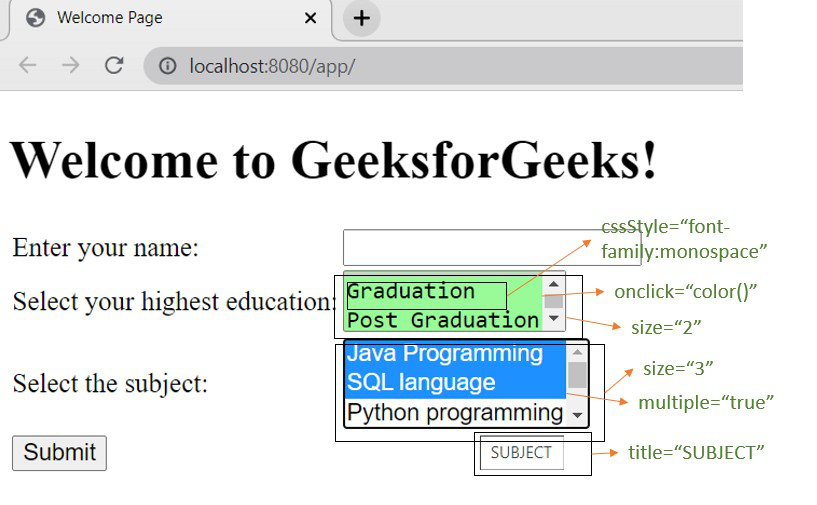
属性的功能
- 输入所有字段,然后单击提交按钮。
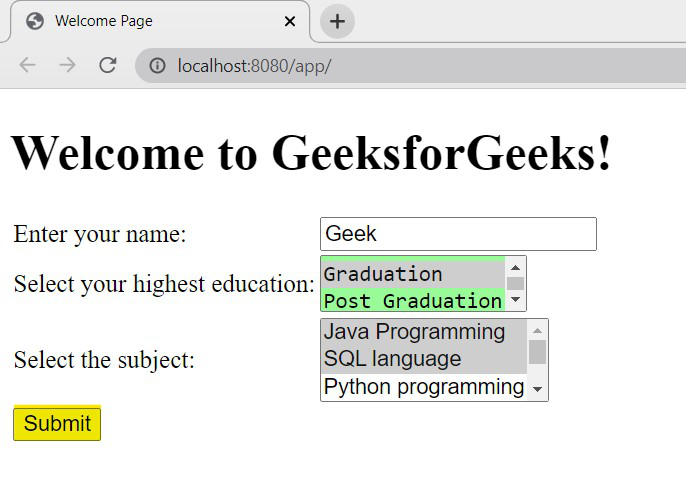
输入要提交的值
- 提交页面后,控制器类处理 URL 请求并执行相应的方法并显示以下输出。
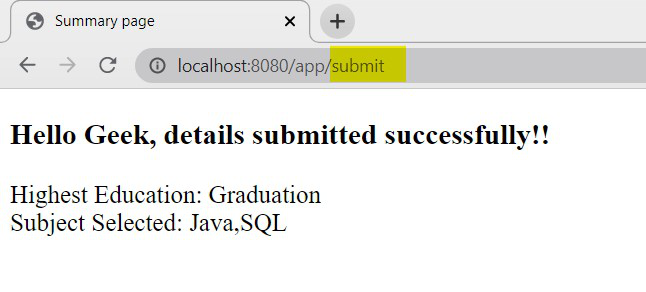
输出 - selectSummary.jsp