在Flutter格式化日期
Dart内置的方法,用于根据要求在Flutter格式化日期是非常有限和限制性的。在处理日期时,它应该采用人类可读的格式,但不幸的是,除非您使用第三方包,否则无法在flutter中格式化日期。
在本文中,我们将研究一种称为intl 包的此类包。
使用国际包:
将以下依赖项添加到您的pubspec.yaml文件中,您可以在此处找到最新的依赖项。
dependencies:
intl: ^0.17.0
使用终端添加:
您还可以使用终端轻松获取最新的 intl 库:
flutter pub add intl
导入它:
现在在你的Dart代码中导入 intl 包:
import 'package:intl/intl.dart';
尽管如此,如果您在使用 intl 时遇到任何错误,只需使用以下命令:
flutter pub get
现在让我们看看下面的例子。
例子:
在下面的代码中,我们不会使用 intl 包进行格式化。另外,看看下面代码的输出。
Dart
import 'package:flutter/material.dart';
void main() {
runApp(dateDemo());
}
class dateDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
// browser tab title
title: "Geeksforgeeks",
// Body
home: Scaffold(
// AppBar
appBar: AppBar(
// AppBar color
backgroundColor: Colors.green.shade900,
// AppBar title
title: Text("Geeksforgeeks"),
),
// Container or Wrapper
body: Container(
margin: EdgeInsets.fromLTRB(95, 80, 0, 0),
// printing text on screen
child: Text(
// Formatted Date
// Builtin fomat / without formatting
DateTime.now().toString(),
style: TextStyle(
// Styling text
fontWeight: FontWeight.bold, fontSize: 30),
),
)),
);
}
}
Dart
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
void main() {
runApp(dateDemo());
}
class dateDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
// browser tab title
title: "Geeksforgeeks",
// Body
home: Scaffold(
// AppBar
appBar: AppBar(
// AppBar color
backgroundColor: Colors.green.shade900,
// AppBar title
title: Text("Geeksforgeeks"),
),
// Container or Wrapper
body: Container(
margin: EdgeInsets.fromLTRB(95, 80, 0, 0),
// printing text on screen
child: Text(
// Formatted Date
DateFormat.yMMMEd()
// displaying formatted date
.format(DateTime.now()),
style: TextStyle(
// Styling text
fontWeight: FontWeight.bold, fontSize: 30),
),
)),
);
}
}
Dart
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
void main() {
runApp(dateDemo());
}
class dateDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: "Date-Demo",
home: Scaffold(
appBar: AppBar(
// AppBar
backgroundColor: Colors.green.shade900,
// AppBar title
title: Text("Geeksforgeeks"),
),
body: Container(
// Container/Wrapper
width: double.infinity,
child:
Column(
// use Column for more Text()
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
// Day,Month Date,Year
DateFormat.yMMMEd().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Day,Month/Date in Numbers
DateFormat.MEd().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// DayName,MonthName Date
DateFormat.MMMMEEEEd().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// DayName,MonthName Date,Year
DateFormat.yMMMMEEEEd().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Full DayName only
DateFormat.EEEE().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Short DayName
DateFormat.E().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Month-Number
DateFormat.M().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// short MonthName
DateFormat.MMM().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// full MonthName
DateFormat.LLLL().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Current Time only
DateFormat.j().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
]),
)),
);
}
}
输出:
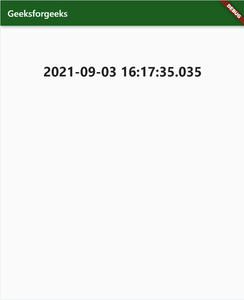
Dart内置格式
现在让我们使用 intl 包来格式化日期。
Dart
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
void main() {
runApp(dateDemo());
}
class dateDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
// browser tab title
title: "Geeksforgeeks",
// Body
home: Scaffold(
// AppBar
appBar: AppBar(
// AppBar color
backgroundColor: Colors.green.shade900,
// AppBar title
title: Text("Geeksforgeeks"),
),
// Container or Wrapper
body: Container(
margin: EdgeInsets.fromLTRB(95, 80, 0, 0),
// printing text on screen
child: Text(
// Formatted Date
DateFormat.yMMMEd()
// displaying formatted date
.format(DateTime.now()),
style: TextStyle(
// Styling text
fontWeight: FontWeight.bold, fontSize: 30),
),
)),
);
}
}
输出:
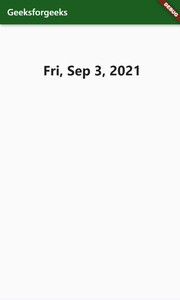
格式化日期
更多 DateFormat 函数:
intl 中的 DateFormat 类提供了许多函数来相应地格式化日期,如下所示: Use Description Example DateFormat.yMMMEd().format(date) Day Name ,Month Name ,Date,Year Fri, Sep 3, 2021 DateFormat.MEd().format(date) Day Name,Month/Date in Numbers Fri , 9/3 DateFormat.MMMMEEEEd().format(date)) DayName,MonthName Date Friday ,September 3 DateFormat.yMMMMEEEEd().format(date)) DayName ,MonthName Date,Year Friday ,September 3,2021 DateFormat.EEEE().format(date) Full DayName only Friday DateFormat.E().format(date) Short DayName Fri DateFormat.M().format(date) Month-Number 9 DateFormat.MMM().format(date) Short MonthName Sep DateFormat.LLLL().format(date) Full MonthName September DateFormat.j().format(date) Current Time only 4 PM
例子:
Dart
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
void main() {
runApp(dateDemo());
}
class dateDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: "Date-Demo",
home: Scaffold(
appBar: AppBar(
// AppBar
backgroundColor: Colors.green.shade900,
// AppBar title
title: Text("Geeksforgeeks"),
),
body: Container(
// Container/Wrapper
width: double.infinity,
child:
Column(
// use Column for more Text()
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
// Day,Month Date,Year
DateFormat.yMMMEd().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Day,Month/Date in Numbers
DateFormat.MEd().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// DayName,MonthName Date
DateFormat.MMMMEEEEd().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// DayName,MonthName Date,Year
DateFormat.yMMMMEEEEd().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Full DayName only
DateFormat.EEEE().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Short DayName
DateFormat.E().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Month-Number
DateFormat.M().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// short MonthName
DateFormat.MMM().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// full MonthName
DateFormat.LLLL().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
Text(
// Current Time only
DateFormat.j().format(DateTime.now()),
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 20),
),
]),
)),
);
}
}
输出:
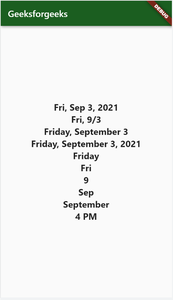
更多 DateFormat 函数
intl是一个具有很多功能的库,所以不要在这里结束并从他们的文档中探索更多。