Solidity 中的存储与内存
Solidity 中的 Storage 和 Memory 关键字类似于计算机的硬盘驱动器和计算机的 RAM。与 RAM 非常相似,Solidity 中的Memory是一个临时存储数据的地方,而Storage在函数调用之间保存数据。 Solidity 智能合约在执行期间可以使用任意数量的内存,但是一旦执行停止,内存将被完全擦除以备下次执行。而另一方面,存储是持久的,智能合约的每次执行都可以访问先前存储在存储区域中的数据。
以太坊虚拟机上的每笔交易都会花费我们一定数量的 Gas。 Gas 消耗越低,您的 Solidity 代码就越好。与 Storage 的 Gas 消耗相比,Memory 的 Gas 消耗不是很显着。因此,中间计算最好使用Memory,将最终结果存储在Storage中。
- 默认情况下,结构体、数组的状态变量和局部变量始终存储在存储中。
- 函数参数在内存中。
- 每当使用关键字“memory”创建数组的新实例时,都会创建该变量的新副本。更改新实例的数组值不会影响原始数组。
示例#1:在下面的示例中,创建了一个合同来演示“存储”关键字。
Solidity
pragma solidity ^0.4.17;
// Creating a contract
contract helloGeeks
{
// Initialising array numbers
int[] public numbers;
// Function to insert values
// in the array numbers
function Numbers() public
{
numbers.push(1);
numbers.push(2);
//Creating a new instance
int[] storage myArray = numbers;
// Adding value to the
// first index of the new Instance
myArray[0] = 0;
}
}
Solidity
pragma solidity ^0.4.17;
// Creating a contract
contract helloGeeks
{
// Initialising array numbers
int[] public numbers;
// Function to insert
// values in the array
// numbers
function Numbers() public
{
numbers.push(1);
numbers.push(2);
//creating a new instance
int[] memory myArray = numbers;
// Adding value to the first
// index of the array myArray
myArray[0] = 0;
}
}
输出:
当我们在上面的代码中检索数组 numbers 的值时,请注意数组的输出是[0,2]而不是[1,2] 。
这可以从下图理解——
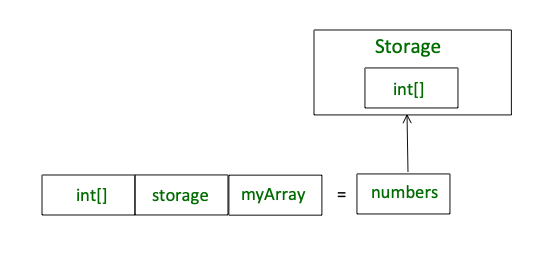
使用关键字“存储”
示例#2:在下面的示例中,创建了一个合约来演示关键字“内存”。
坚固性
pragma solidity ^0.4.17;
// Creating a contract
contract helloGeeks
{
// Initialising array numbers
int[] public numbers;
// Function to insert
// values in the array
// numbers
function Numbers() public
{
numbers.push(1);
numbers.push(2);
//creating a new instance
int[] memory myArray = numbers;
// Adding value to the first
// index of the array myArray
myArray[0] = 0;
}
}
输出:
当我们在上面的代码中检索数组 numbers 的值时,注意数组的输出是 [1,2]。在这种情况下,更改 myArray 的值不会影响数组 numbers 中的值。
这可以从下图理解——
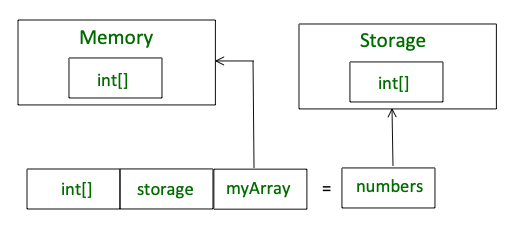
使用关键字“记忆”