Java中的 MouseListener 和 MouseMotionListener
MouseListener 和 MouseMotionListener 是Java.awt.event 包中的一个接口。鼠标事件
有两种类型。 MouseListener 在鼠标不运动时处理事件。而 MouseMotionListener
处理鼠标移动时的事件。
MouseListener 可以生成五种类型的事件。有五个抽象函数代表这五个事件。抽象函数是:
- void mouseReleased(MouseEvent e) : 鼠标键被释放
- void mouseClicked(MouseEvent e) : 鼠标键被按下/释放
- void mouseExited(MouseEvent e) : 鼠标退出组件
- void mouseEntered(MouseEvent e) : 鼠标进入组件
- void mousepressed(MouseEvent e) : 鼠标键被按下
MouseMotionListener 可以生成两种类型的事件。有两个抽象函数代表这五个事件。抽象函数是:
- void mouseDragged(MouseEvent e) :在组件中按下鼠标按钮并拖动时调用。事件一直传递,直到用户释放鼠标按钮。
- void mouseMoved(MouseEvent e) :当鼠标光标在组件内从一个点移动到另一个点时调用,无需按下任何鼠标按钮。
以下程序是 MouseListener 和 MouseMotionListener 的说明。
1.处理MouseListener事件的程序
Java
// Java program to handle MouseListener events
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class Mouse extends Frame implements MouseListener {
// Jlabels to display the actions of events of mouseListener
// static JLabel label1, label2, label3;
// default constructor
Mouse()
{
}
// main class
public static void main(String[] args)
{
// create a frame
JFrame f = new JFrame("MouseListener");
// set the size of the frame
f.setSize(600, 100);
// close the frame when close button is pressed
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// create anew panel
JPanel p = new JPanel();
// set the layout of the panel
p.setLayout(new FlowLayout());
// initialize the labels
label1 = new JLabel("no event ");
label2 = new JLabel("no event ");
label3 = new JLabel("no event ");
// create an object of mouse class
Mouse m = new Mouse();
// add mouseListener to the frame
f.addMouseListener(m);
// add labels to the panel
p.add(label1);
p.add(label2);
p.add(label3);
// add panel to the frame
f.add(p);
f.show();
}
// getX() and getY() functions return the
// x and y coordinates of the current
// mouse position
// getClickCount() returns the number of
// quick consecutive clicks made by the user
// this function is invoked when the mouse is pressed
public void mousePressed(MouseEvent e)
{
// show the point where the user pressed the mouse
label1.setText("mouse pressed at point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse is released
public void mouseReleased(MouseEvent e)
{
// show the point where the user released the mouse click
label1.setText("mouse released at point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse exits the component
public void mouseExited(MouseEvent e)
{
// show the point through which the mouse exited the frame
label2.setText("mouse exited through point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse enters the component
public void mouseEntered(MouseEvent e)
{
// show the point through which the mouse entered the frame
label2.setText("mouse entered at point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse is pressed or released
public void mouseClicked(MouseEvent e)
{
// getClickCount gives the number of quick,
// consecutive clicks made by the user
// show the point where the mouse is i.e
// the x and y coordinates
label3.setText("mouse clicked at point:"
+ e.getX() + " "
+ e.getY() + "mouse clicked :" + e.getClickCount());
}
}
Java
//Program of an applet which
//displays x and y co-ordinate
//in it's status bar,whenever
//the user click anywhere in
//the applet window.
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class GFG extends Applet implements MouseListener
{
public void init()
{
this.addMouseListener (this);
//first "this" represent source
//(in this case it is applet which
//is current calling object) and
//second "this" represent
//listener(in this case it is GFG)
}
public void mouseClicked(MouseEvent m)
{
int x = m.getX();
int y = m.getY();
String str = "x =" +x+",y = "+y;
showStatus(str);
}
@Override
public void mousePressed(MouseEvent e) {
}
@Override
public void mouseReleased(MouseEvent e) {
}
@Override
public void mouseEntered(MouseEvent e) {
}
@Override
public void mouseExited(MouseEvent e) {
}
}
Java
//Co-ordinates should display
//at that point only wherever
//their is click on canvas
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class GFG extends Applet implements MouseListener
{
private int x,y;
private String str = " ";
public void init()
{
this.addMouseListener (this);
//first "this" represent source
//(in this case it is applet which
// is current calling object) and
// second "this" represent listener
//(in this case it is GFG)
}
public void paint(Graphics g)
{
g.drawString(str,x,y);
}
public void mouseClicked(MouseEvent m)
{
x = m.getX();
y = m.getY();
str = "x =" +x+",y = "+y;
repaint(); // we have made this
//call because repaint() will
//call paint() method for us.
//If we comment out this line,
//then we will see output
//only when focus is on the applet
//i.e on maximising the applet window
//because paint() method is called
//when applet screen gets the focus.
//repaint() is a method of Component
//class and prototype for this method is:
//public void repaint()
}
public void mouseEntered(MouseEvent m)
//over-riding all the methods given by
// MouseListener
{
}
public void mouseExited(MouseEvent m)
{
}
public void mousePressed(MouseEvent m)
{
}
public void mouseReleased(MouseEvent m)
{
}
}
Java
//Co-ordinates should display
//at that point only wherever
//their is click on canvas and also
//able to see the previous coordinates
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class GFG extends Applet implements MouseListener
{
private int x,y;
private String str = " ";
public void init()
{
this.addMouseListener (this);
}
public void paint(Graphics g)
{
g.drawString(str,x,y);
}
public void update(Graphics g)
{
paint(g);
}
public void mouseClicked(MouseEvent m)
{
x = m.getX();
y = m.getY();
str = "x =" +x+",y = "+y;
repaint();
}
public void mouseEntered(MouseEvent m)
{
}
public void mouseExited(MouseEvent m)
{
}
public void mousePressed(MouseEvent m)
{
}
public void mouseReleased(MouseEvent m)
{
}
}
Java
// Java Program to illustrate MouseMotionListener events
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class Mouse extends Frame implements MouseMotionListener {
// Jlabels to display the actions of events of MouseMotionListener
static JLabel label1, label2;
// default constructor
Mouse()
{
}
// main class
public static void main(String[] args)
{
// create a frame
JFrame f = new JFrame("MouseMotionListener");
// set the size of the frame
f.setSize(600, 400);
// close the frame when close button is pressed
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// create anew panel
JPanel p = new JPanel();
// set the layout of the panel
p.setLayout(new FlowLayout());
// initialize the labels
label1 = new JLabel("no event ");
label2 = new JLabel("no event ");
// create an object of mouse class
Mouse m = new Mouse();
// add mouseListener to the frame
f.addMouseMotionListener(m);
// add labels to the panel
p.add(label1);
p.add(label2);
// add panel to the frame
f.add(p);
f.show();
}
// getX() and getY() functions return the
// x and y coordinates of the current
// mouse position
// invoked when mouse button is pressed
// and dragged from one point to another
// in a component
public void mouseDragged(MouseEvent e)
{
// update the label to show the point
// through which point mouse is dragged
label1.setText("mouse is dragged through point "
+ e.getX() + " " + e.getY());
}
// invoked when the cursor is moved from
// one point to another within the component
public void mouseMoved(MouseEvent e)
{
// update the label to show the point to which the cursor moved
label2.setText("mouse is moved to point "
+ e.getX() + " " + e.getY());
}
}
Java
// Java program to illustrate MouseListener
// and MouseMotionListener events
// simultaneously
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class Mouse extends Frame implements MouseMotionListener, MouseListener {
// Jlabels to display the actions of events of MouseMotionListener and MouseListener
static JLabel label1, label2, label3, label4, label5;
// default constructor
Mouse()
{
}
// main class
public static void main(String[] args)
{
// create a frame
JFrame f = new JFrame("MouseListener and MouseMotionListener");
// set the size of the frame
f.setSize(900, 300);
// close the frame when close button is pressed
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// create anew panel
JPanel p = new JPanel();
JPanel p1 = new JPanel();
// set the layout of the frame
f.setLayout(new FlowLayout());
JLabel l1, l2;
l1 = new JLabel("MouseMotionListener events :");
l2 = new JLabel("MouseLIstener events :");
// initialize the labels
label1 = new JLabel("no event ");
label2 = new JLabel("no event ");
label3 = new JLabel("no event ");
label4 = new JLabel("no event ");
label5 = new JLabel("no event ");
// create an object of mouse class
Mouse m = new Mouse();
// add mouseListener and MouseMotionListenerto the frame
f.addMouseMotionListener(m);
f.addMouseListener(m);
// add labels to the panel
p.add(l1);
p.add(label1);
p.add(label2);
p1.add(l2);
p1.add(label3);
p1.add(label4);
p1.add(label5);
// add panel to the frame
f.add(p);
f.add(p1);
f.show();
}
// getX() and getY() functions return the
// x and y coordinates of the current
// mouse position
// getClickCount() returns the number of
// quick consecutive clicks made by the user
// MouseMotionListener events
// invoked when mouse button is pressed
// and dragged from one point to another
// in a component
public void mouseDragged(MouseEvent e)
{
// update the label to show the point
// through which point mouse is dragged
label1.setText("mouse is dragged through point "
+ e.getX() + " " + e.getY());
}
// invoked when the cursor is moved from
// one point to another within the component
public void mouseMoved(MouseEvent e)
{
// update the label to show the point to which the cursor moved
label2.setText("mouse is moved to point "
+ e.getX() + " " + e.getY());
}
// MouseListener events
// this function is invoked when the mouse is pressed
public void mousePressed(MouseEvent e)
{
// show the point where the user pressed the mouse
label3.setText("mouse pressed at point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse is released
public void mouseReleased(MouseEvent e)
{
// show the point where the user released the mouse click
label3.setText("mouse released at point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse exits the component
public void mouseExited(MouseEvent e)
{
// show the point through which the mouse exited the frame
label4.setText("mouse exited through point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse enters the component
public void mouseEntered(MouseEvent e)
{
// show the point through which the mouse entered the frame
label4.setText("mouse entered at point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse is pressed or released
public void mouseClicked(MouseEvent e)
{
// getClickCount gives the number of quick,
// consecutive clicks made by the user
// show the point where the mouse is i.e
// the x and y coordinates
label5.setText("mouse clicked at point:"
+ e.getX() + " "
+ e.getY() + "mouse clicked :" + e.getClickCount());
}
}
输出 :
注意:以下程序可能无法在在线编译器中运行,请使用离线 IDE
让我们在 MouseListener 上再举一个例子,问题是:
Q.编写一个小程序,只要用户单击小程序窗口中的任何位置,它就会在其状态栏中显示 x 和 y 坐标。
答。
注意:此代码与 Netbeans IDE 相关。
Java
//Program of an applet which
//displays x and y co-ordinate
//in it's status bar,whenever
//the user click anywhere in
//the applet window.
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class GFG extends Applet implements MouseListener
{
public void init()
{
this.addMouseListener (this);
//first "this" represent source
//(in this case it is applet which
//is current calling object) and
//second "this" represent
//listener(in this case it is GFG)
}
public void mouseClicked(MouseEvent m)
{
int x = m.getX();
int y = m.getY();
String str = "x =" +x+",y = "+y;
showStatus(str);
}
@Override
public void mousePressed(MouseEvent e) {
}
@Override
public void mouseReleased(MouseEvent e) {
}
@Override
public void mouseEntered(MouseEvent e) {
}
@Override
public void mouseExited(MouseEvent e) {
}
}
输出:
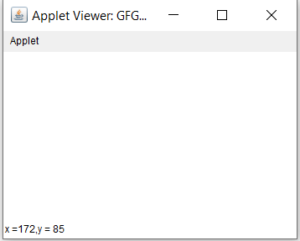
输出在状态栏中显示 (x,y)
修改:现在我们的目标是改进上面的程序,使坐标应该只显示在点击的那个点
注意:此代码与 Netbeans IDE 相关。
Java
//Co-ordinates should display
//at that point only wherever
//their is click on canvas
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class GFG extends Applet implements MouseListener
{
private int x,y;
private String str = " ";
public void init()
{
this.addMouseListener (this);
//first "this" represent source
//(in this case it is applet which
// is current calling object) and
// second "this" represent listener
//(in this case it is GFG)
}
public void paint(Graphics g)
{
g.drawString(str,x,y);
}
public void mouseClicked(MouseEvent m)
{
x = m.getX();
y = m.getY();
str = "x =" +x+",y = "+y;
repaint(); // we have made this
//call because repaint() will
//call paint() method for us.
//If we comment out this line,
//then we will see output
//only when focus is on the applet
//i.e on maximising the applet window
//because paint() method is called
//when applet screen gets the focus.
//repaint() is a method of Component
//class and prototype for this method is:
//public void repaint()
}
public void mouseEntered(MouseEvent m)
//over-riding all the methods given by
// MouseListener
{
}
public void mouseExited(MouseEvent m)
{
}
public void mousePressed(MouseEvent m)
{
}
public void mouseReleased(MouseEvent m)
{
}
}
输出
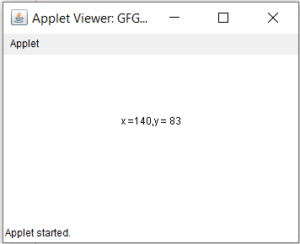
输出在画布中显示 (x,y)
现在还有一件不寻常的事情会出现在输出中,那就是,我们将无法看到以前的坐标。但是为什么呢?
在Java中,在调用 paint() 方法之前,它会调用另一个方法 update() 并执行以下操作:
- 它用当前颜色重新绘制小程序背景。
- 然后它调用paint()。
现在也可以查看以前的坐标:
我们还必须覆盖 update() 方法,它的原型类似于paint()。
进一步修改要查看以前的坐标:
注意:此代码与 Netbeans IDE 相关。
Java
//Co-ordinates should display
//at that point only wherever
//their is click on canvas and also
//able to see the previous coordinates
import java.awt.*;
import java.awt.event.*;
import java.applet.*;
public class GFG extends Applet implements MouseListener
{
private int x,y;
private String str = " ";
public void init()
{
this.addMouseListener (this);
}
public void paint(Graphics g)
{
g.drawString(str,x,y);
}
public void update(Graphics g)
{
paint(g);
}
public void mouseClicked(MouseEvent m)
{
x = m.getX();
y = m.getY();
str = "x =" +x+",y = "+y;
repaint();
}
public void mouseEntered(MouseEvent m)
{
}
public void mouseExited(MouseEvent m)
{
}
public void mousePressed(MouseEvent m)
{
}
public void mouseReleased(MouseEvent m)
{
}
}
输出
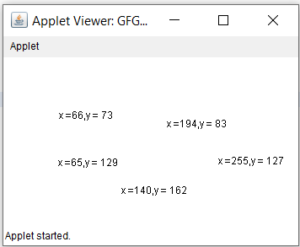
输出也显示以前的坐标
2.处理MouseMotionListener事件的程序
Java
// Java Program to illustrate MouseMotionListener events
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class Mouse extends Frame implements MouseMotionListener {
// Jlabels to display the actions of events of MouseMotionListener
static JLabel label1, label2;
// default constructor
Mouse()
{
}
// main class
public static void main(String[] args)
{
// create a frame
JFrame f = new JFrame("MouseMotionListener");
// set the size of the frame
f.setSize(600, 400);
// close the frame when close button is pressed
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// create anew panel
JPanel p = new JPanel();
// set the layout of the panel
p.setLayout(new FlowLayout());
// initialize the labels
label1 = new JLabel("no event ");
label2 = new JLabel("no event ");
// create an object of mouse class
Mouse m = new Mouse();
// add mouseListener to the frame
f.addMouseMotionListener(m);
// add labels to the panel
p.add(label1);
p.add(label2);
// add panel to the frame
f.add(p);
f.show();
}
// getX() and getY() functions return the
// x and y coordinates of the current
// mouse position
// invoked when mouse button is pressed
// and dragged from one point to another
// in a component
public void mouseDragged(MouseEvent e)
{
// update the label to show the point
// through which point mouse is dragged
label1.setText("mouse is dragged through point "
+ e.getX() + " " + e.getY());
}
// invoked when the cursor is moved from
// one point to another within the component
public void mouseMoved(MouseEvent e)
{
// update the label to show the point to which the cursor moved
label2.setText("mouse is moved to point "
+ e.getX() + " " + e.getY());
}
}
输出 :
3. 说明 MouseListener 和 MouseMotionListener 事件的Java程序
同时地
Java
// Java program to illustrate MouseListener
// and MouseMotionListener events
// simultaneously
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class Mouse extends Frame implements MouseMotionListener, MouseListener {
// Jlabels to display the actions of events of MouseMotionListener and MouseListener
static JLabel label1, label2, label3, label4, label5;
// default constructor
Mouse()
{
}
// main class
public static void main(String[] args)
{
// create a frame
JFrame f = new JFrame("MouseListener and MouseMotionListener");
// set the size of the frame
f.setSize(900, 300);
// close the frame when close button is pressed
f.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// create anew panel
JPanel p = new JPanel();
JPanel p1 = new JPanel();
// set the layout of the frame
f.setLayout(new FlowLayout());
JLabel l1, l2;
l1 = new JLabel("MouseMotionListener events :");
l2 = new JLabel("MouseLIstener events :");
// initialize the labels
label1 = new JLabel("no event ");
label2 = new JLabel("no event ");
label3 = new JLabel("no event ");
label4 = new JLabel("no event ");
label5 = new JLabel("no event ");
// create an object of mouse class
Mouse m = new Mouse();
// add mouseListener and MouseMotionListenerto the frame
f.addMouseMotionListener(m);
f.addMouseListener(m);
// add labels to the panel
p.add(l1);
p.add(label1);
p.add(label2);
p1.add(l2);
p1.add(label3);
p1.add(label4);
p1.add(label5);
// add panel to the frame
f.add(p);
f.add(p1);
f.show();
}
// getX() and getY() functions return the
// x and y coordinates of the current
// mouse position
// getClickCount() returns the number of
// quick consecutive clicks made by the user
// MouseMotionListener events
// invoked when mouse button is pressed
// and dragged from one point to another
// in a component
public void mouseDragged(MouseEvent e)
{
// update the label to show the point
// through which point mouse is dragged
label1.setText("mouse is dragged through point "
+ e.getX() + " " + e.getY());
}
// invoked when the cursor is moved from
// one point to another within the component
public void mouseMoved(MouseEvent e)
{
// update the label to show the point to which the cursor moved
label2.setText("mouse is moved to point "
+ e.getX() + " " + e.getY());
}
// MouseListener events
// this function is invoked when the mouse is pressed
public void mousePressed(MouseEvent e)
{
// show the point where the user pressed the mouse
label3.setText("mouse pressed at point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse is released
public void mouseReleased(MouseEvent e)
{
// show the point where the user released the mouse click
label3.setText("mouse released at point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse exits the component
public void mouseExited(MouseEvent e)
{
// show the point through which the mouse exited the frame
label4.setText("mouse exited through point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse enters the component
public void mouseEntered(MouseEvent e)
{
// show the point through which the mouse entered the frame
label4.setText("mouse entered at point:"
+ e.getX() + " " + e.getY());
}
// this function is invoked when the mouse is pressed or released
public void mouseClicked(MouseEvent e)
{
// getClickCount gives the number of quick,
// consecutive clicks made by the user
// show the point where the mouse is i.e
// the x and y coordinates
label5.setText("mouse clicked at point:"
+ e.getX() + " "
+ e.getY() + "mouse clicked :" + e.getClickCount());
}
}
输出 :
MouseListener 与 MouseMotionListener
- MouseListener: MouseListener 事件在鼠标不运动且稳定时被调用。它生成诸如mousePressed、mouseReleased、mouseClicked、mouseExited 和mouseEntered 等事件(即当鼠标按钮被按下或鼠标进入或退出组件时)。此类的对象必须在组件中注册,并使用 addMouseListener() 方法注册。
- MouseMotionListener:鼠标移动时调用 MouseMotionListener 事件。它生成诸如mouseMoved 和mouseDragged 之类的事件(即当鼠标在组件内从一个点移动到另一个点或鼠标按钮被按下并从一个点拖动到另一个点时)。此类的对象必须在组件中注册,并且使用 addMouseMotionListener() 方法注册。