提取 SQL 查询的正则表达式
正则表达式是搜索与复杂条件匹配的数据的更简单的机制。例如,从字母数字值中,仅提取字母值或数字值或检查字符匹配的特定模式并检索记录等。
让我们通过一些示例场景来一一看看;
第 1 步:创建数据库
询问:
SELECT * FROM sys.databases WHERE name = 'GEEKSFORGEEKS'
BEGIN
CREATE DATABASE [GEEKSFORGEEKS]
END
第 2 步:使用数据库
询问:
USE GEEKSFORGEEKS
第 3 步:在 GEEKSFORGEEKS 下创建 TABLE Country 并插入几条记录
询问:
INSERT INTO Country(CountryID,CountryName) VALUES (1,'United States');
INSERT INTO Country(CountryID,CountryName) VALUES (2,'United States');
INSERT INTO Country(CountryID,CountryName) VALUES (3,'United Kingdom');
INSERT INTO Country(CountryID,CountryName) VALUES (4,'Canada');
INSERT INTO Country(CountryID,CountryName) VALUES (5,'United Kingdom');
INSERT INTO Country(CountryID,CountryName) VALUES (6,'Canada');
INSERT INTO Country(CountryID,CountryName) VALUES (7,'United States');
INSERT INTO Country(CountryID,CountryName) VALUES (8,'Australia');
INSERT INTO Country(CountryID,CountryName) VALUES (9,'Canada');
INSERT INTO Country(CountryID,CountryName) VALUES (10,'United States');
SELECT * FROM Country
输出 :
示例 1:
要获取从 A - D 开始的记录和 U 到 Z 之间的第二个字母,其余字母可以是任何内容。
询问:
--Find Country Names having:
--First character should be A and D alphabets.
--The second character should be from U and Z alphabet
SELECT * FROM Country
WHERE CountryName like '[A-D][U-Z]%'
--regular expession is used here
输出 :
如果我们需要单独检查第一个字符,其余字符可以是任何字符,那么
询问:
--Find Country Names having:
--First character should be A and D alphabets.
--Rest letters can be any character
SELECT * FROM Country
WHERE CountryName like '[A-D]%'
--regular expession
输出 :
看到上面两个输出,我们可以理解,只是给出不同的正则表达式,我们得到了不同的输出。
假设如果我们想单独查找以“U”开头的国家名称,那么查询将如下所示:
询问:
--Find country names starting with 'U' alone
SELECT * FROM Country
WHERE CountryName like 'U%'
--regular expession
输出 :
假设如果我们要查找以“U”开头的国家名称和其他信息,那么查询将如下所示:
询问:
--Find country names starting with
-- U and additonal condition is given
SELECT * FROM Country
WHERE CountryName like 'U% [S]%'
--regular expession
输出 :
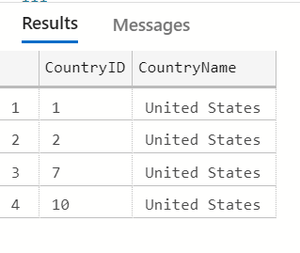
'
与 Like运算符一起使用时,我们还需要了解以下因素Wildcard character Description % A string of 0 or more characters will be retrieved [ ] Within the specified range, any single character alone will be retrieved [^] Within the specified range, none of the characters will be retrieved
我们也可以在其他函数中使用正则表达式。
示例 2:
让我们先看看 PATINDEX函数。它是一个函数,它接受搜索模式和输入字符串,并返回与模式不匹配的字符的起始位置。
--pattern to check is A-Z or a-z(search pattern)
-- in the input string and
--position of the non-matching pattern
-- It checks for numeric value position
--and it is displaying position of the character
SELECT 'GFGVersion1' as InputString,
PATINDEX('%[^A-Za-z]%', 'GFGVersion1') as
NumericCharacterPosition;
输出 :
要仅从输入字符串中获取数字,我们也可以使用以下方式
SELECT 'GFGVersion1' as InputString,
PATINDEX('%[0-9]%', 'GFGVersion1') as
NumericCharacterPosition;
即不是使用 [^A-Za-z],而是使用 [0-9] 并获得与上述相同的结果
-- 0 will indicate no numeric value present
SELECT 'VERSION' as InputString,
PATINDEX('%[^A-Za-z]%', 'VERSION')
as NumericPosition;
输出 :

如果没有数字,NumericPosition 将显示 0
我们可以将正则表达式与 PATINDEX 等函数结合使用,我们可以解决仅从输入字符串中获取字符/仅从输入字符串中获取数字等问题,
为此,让我们也看看 STUFF函数
东西函数
--remove the integer from
-- position 3 in the input string.
/* As only one character need to be removed,
we need to send params like this
1st Param -- Input string
2nd Param -- Start location
3rd Param -- Number of characters to be replaced
4th Param - Replacing value
*/
SELECT STUFF('GE098EKS9VER1', 3, 1, '' );
输出 :
示例 3:
通过使用 PATINDEX 和 STUFF 函数,我们只能从输入字符串中获取字符值。
- 我们需要使用正则表达式来应用 PATINDEX
- 使用 STUFF函数找出数字位置并删除数字
- 必须重复第 2 步,直到没有数值
询问:
DECLARE @inputData NVARCHAR(MAX) = 'GE098EKS9VER1'
--input string
DECLARE @intPosition INT
--get the position of the integer from the input string
SET @intPosition = PATINDEX('%[^A-Za-z]%', @inputData)
print @intPosition
--run loop until no integer is found in the input string
WHILE @intPosition > 0
BEGIN
--remove the integer from that position
SET @inputData = STUFF(@inputData, @intPosition, 1, '' )
--PRINT @inputData
SET @intPosition = PATINDEX('%[^A-Za-z]%', @inputData )
--Again get the position of the next integer in the input string
--PRINT @intPosition
END
SELECT 'GE098EKS9VER1' as InputString, @inputData AS AlphabetsOnly
输出 :
同样,我们可以使用正则表达式作为最佳搜索模式实践。
在整个 SQL 中,我们可以使用正则表达式根据需要提取不同的输出。它也可以与其他函数一起使用,它有助于仅从输入字符串中获取字母/从输入字符串中获取数字。