使用 Playfair 密码 Enode 消息的Java程序
Playfair 密码是属于替代密码类别的传统密码之一。在 Playfair Cipher 中,与传统密码不同,我们加密一对字母(有向图)而不是单个字母。在 Playfair 密码中,最初会创建一个密钥表。密钥表是一个由字母组成的 5×5 矩阵,作为明文加密的密钥。 25 个字母中的每一个都必须是唯一的,并且表中省略了一个字母(通常是 'j'),因为我们只需要 25 个字母而不是 26 个。如果明文包含 'j',则将其替换为 '一世'。
Playfair 密码流程:
- 明文消息被分成两个字母对(有向图)。如果明文有奇数个字符,则在末尾附加“z”以使消息长度为偶数。
- 识别在明文中并排放置的任何双字母,并将第二个字母替换为“x”,例如“hello”->“he lx lo”。
- 现在,找到 5×5 密钥表中的字母。
- 使用以下规则加密明文:
- 如果这些字母出现在表格的同一行,请分别用它们紧邻的字母替换它们(如果原始对中的一个字母在该行的右侧,则绕到该行的左侧)。
- 如果字母出现在表格的同一列上,请分别用紧邻下方的字母替换它们(如果原始对中的字母在列的底部,则绕到列的顶部)
- 如果这些字母不在同一行或同一列,则将它们替换为位于其所在行但与另一个字母位于同一列的字母。
Playfair cipher 的解密过程与加密过程相同,但应用方式相反。接收者拥有相同的密钥并且可以创建相同的密钥表,他用它来解密使用该密钥生成的密文消息。
例子:
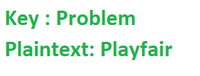
playfair 密码的输入

Playfair 密码密钥矩阵
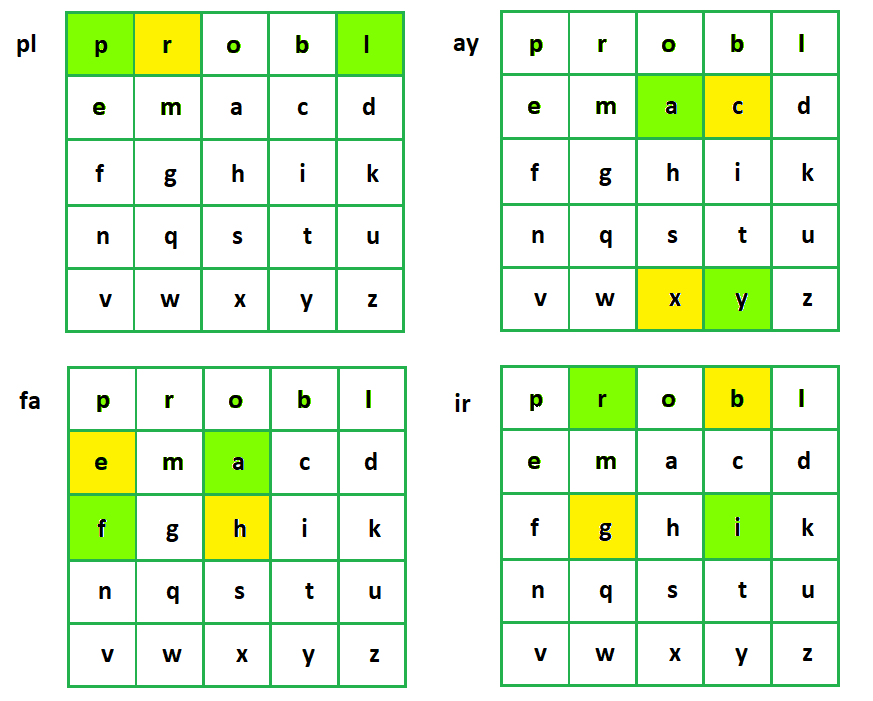
使用 Playfair Cipher 生成密文
Java
// Java Program to Enode a Message Using Playfair Cipher
import java.io.*;
import java.util.*;
class Playfair {
String key;
String plainText;
char[][] matrix = new char[5][5];
public Playfair(String key, String plainText)
{
// convert all the characters to lowercase
this.key = key.toLowerCase();
this.plainText = plainText.toLowerCase();
}
// function to remove duplicate characters from the key
public void cleanPlayFairKey()
{
LinkedHashSet set
= new LinkedHashSet();
String newKey = "";
for (int i = 0; i < key.length(); i++)
set.add(key.charAt(i));
Iterator it = set.iterator();
while (it.hasNext())
newKey += (Character)it.next();
key = newKey;
}
// function to generate playfair cipher key table
public void generateCipherKey()
{
Set set = new HashSet();
for (int i = 0; i < key.length(); i++)
{
if (key.charAt(i) == 'j')
continue;
set.add(key.charAt(i));
}
// remove repeated characters from the cipher key
String tempKey = new String(key);
for (int i = 0; i < 26; i++)
{
char ch = (char)(i + 97);
if (ch == 'j')
continue;
if (!set.contains(ch))
tempKey += ch;
}
// create cipher key table
for (int i = 0, idx = 0; i < 5; i++)
for (int j = 0; j < 5; j++)
matrix[i][j] = tempKey.charAt(idx++);
System.out.println("Playfair Cipher Key Matrix:");
for (int i = 0; i < 5; i++)
System.out.println(Arrays.toString(matrix[i]));
}
// function to preprocess plaintext
public String formatPlainText()
{
String message = "";
int len = plainText.length();
for (int i = 0; i < len; i++)
{
// if plaintext contains the character 'j',
// replace it with 'i'
if (plainText.charAt(i) == 'j')
message += 'i';
else
message += plainText.charAt(i);
}
// if two consecutive characters are same, then
// insert character 'x' in between them
for (int i = 0; i < message.length(); i += 2)
{
if (message.charAt(i) == message.charAt(i + 1))
message = message.substring(0, i + 1) + 'x'
+ message.substring(i + 1);
}
// make the plaintext of even length
if (len % 2 == 1)
message += 'x'; // dummy character
return message;
}
// function to group every two characters
public String[] formPairs(String message)
{
int len = message.length();
String[] pairs = new String[len / 2];
for (int i = 0, cnt = 0; i < len / 2; i++)
pairs[i] = message.substring(cnt, cnt += 2);
return pairs;
}
// function to get position of character in key table
public int[] getCharPos(char ch)
{
int[] keyPos = new int[2];
for (int i = 0; i < 5; i++)
{
for (int j = 0; j < 5; j++)
{
if (matrix[i][j] == ch)
{
keyPos[0] = i;
keyPos[1] = j;
break;
}
}
}
return keyPos;
}
public String encryptMessage()
{
String message = formatPlainText();
String[] msgPairs = formPairs(message);
String encText = "";
for (int i = 0; i < msgPairs.length; i++)
{
char ch1 = msgPairs[i].charAt(0);
char ch2 = msgPairs[i].charAt(1);
int[] ch1Pos = getCharPos(ch1);
int[] ch2Pos = getCharPos(ch2);
// if both the characters are in the same row
if (ch1Pos[0] == ch2Pos[0]) {
ch1Pos[1] = (ch1Pos[1] + 1) % 5;
ch2Pos[1] = (ch2Pos[1] + 1) % 5;
}
// if both the characters are in the same column
else if (ch1Pos[1] == ch2Pos[1])
{
ch1Pos[0] = (ch1Pos[0] + 1) % 5;
ch2Pos[0] = (ch2Pos[0] + 1) % 5;
}
// if both the characters are in different rows
// and columns
else {
int temp = ch1Pos[1];
ch1Pos[1] = ch2Pos[1];
ch2Pos[1] = temp;
}
// get the corresponding cipher characters from
// the key matrix
encText = encText + matrix[ch1Pos[0]][ch1Pos[1]]
+ matrix[ch2Pos[0]][ch2Pos[1]];
}
return encText;
}
}
public class GFG {
public static void main(String[] args)
{
System.out.println("Example-1\n");
String key1 = "Problem";
String plainText1 = "Playfair";
System.out.println("Key: " + key1);
System.out.println("PlainText: " + plainText1);
Playfair pfc1 = new Playfair(key1, plainText1);
pfc1.cleanPlayFairKey();
pfc1.generateCipherKey();
String encText1 = pfc1.encryptMessage();
System.out.println("Cipher Text is: " + encText1);
System.out.println("\nExample-2\n");
String key2 = "Problem";
String plainText2 = "Hello";
System.out.println("Key: " + key2);
System.out.println("PlainText: " + plainText2);
Playfair pfc2 = new Playfair(key2, plainText2);
pfc2.cleanPlayFairKey();
pfc2.generateCipherKey();
String encText2 = pfc2.encryptMessage();
System.out.println("Cipher Text is: " + encText2);
}
}
输出
Example-1
Key: Problem
PlainText: Playfair
Playfair Cipher Key Matrix:
[p, r, o, b, l]
[e, m, a, c, d]
[f, g, h, i, k]
[n, q, s, t, u]
[v, w, x, y, z]
Cipher Text is: rpcxhegb
Example-2
Key: Problem
PlainText: Hello
Playfair Cipher Key Matrix:
[p, r, o, b, l]
[e, m, a, c, d]
[f, g, h, i, k]
[n, q, s, t, u]
[v, w, x, y, z]
Cipher Text is: faozpb