Rust 中的函数
函数是可以执行类似相关操作的可重用代码块。您已经看到了该语言中最重要的函数之一: main函数,它是许多程序的入口点。您还看到了“fn”关键字,它允许您声明新函数。 Rust 代码使用蛇形大小写作为函数和变量名称的常规样式。在蛇的情况下,所有字母都是小写,并下划线单独的单词。
句法:
fn functionname(arguments){
code
}
- 要创建一个函数,我们需要使用fn 关键字。
- 函数名写在 fn 关键字之后
- 参数在括号内的函数名之后传递
- 您可以在函数块中编写函数代码
例子:
我们将在下面的代码中研究一个简单的函数
Rust
fn main() {
greet("kushwanthreddy");
}
fn greet(name: &str) {
println!("hello {} welcome to geeksforgeeks",name);
}
Rust
use std::io;
fn main() {
println!("enter a number:");
let mut stra = String::new();
io::stdin()
.read_line(&mut stra)
.expect("failed to read input.");
println!("enter b number:");
let mut strb = String::new();
io::stdin()
.read_line(&mut strb)
.expect("failed to read input.");
let a: i32 = stra.trim().parse().expect("invalid input");
let b: i32 = strb.trim().parse().expect("invalid input");
sum(a,b);
}
fn sum(x: i32, y: i32) {
println!("sum = {}", x+y);
}
Rust
use std::io;
use std::process::exit;
fn main() {
println!("enter a number:");
let mut stra = String::new();
io::stdin()
.read_line(&mut stra)
.expect("failed to read input.");
println!("enter b number:");
let mut strb = String::new();
io::stdin()
.read_line(&mut strb)
.expect("failed to read input.");
let a: i32 = stra.trim().parse().expect("invalid input");
let b: i32 = strb.trim().parse().expect("invalid input");
println!("choose your calculation: \n1.sum
\n2.difference
\n3.product
\n4.quotient
\n5.remainder\n");
let mut choose = String::new();
io::stdin()
.read_line(&mut choose)
.expect("failed to read input.");
let c: i32 = choose.trim().parse().expect("invalid input");
// Select Operation using conditionals
if c==1{sum(a,b);}
else if c==2{sub(a,b);}
else if c==3{mul(a,b);}
else if c==4{quo(a,b);}
else if c==5{rem(a,b);}
else{println!("Invalid argument");exit(1);}
}
// Sum function
fn sum(x: i32, y: i32) {
println!("sum = {}", x+y);
}
// Difference function
fn sub(x: i32, y: i32) {
println!("difference = {}", x-y);
}
// Product function
fn mul(x: i32, y: i32) {
println!("product = {}", x*y);
}
// Division function
fn quo(x: i32, y: i32) {
println!("quotient = {}", x/y);
}
// Remainder function
fn rem(x: i32, y: i32) {
println!("remainder = {}", x%y);
}
输出:
hello kushwanthreddy welcome to geeksforgeeks
在上面的函数声明中,我们编写了一个欢迎程序,它接受一个参数并打印一条欢迎消息。我们给greet函数的参数是一个名字(字符串数据类型)。我们使用了以下方法:
- main函数有一个greet函数
- Greet函数将名称(字符串)作为参数
- 问候函数打印欢迎信息
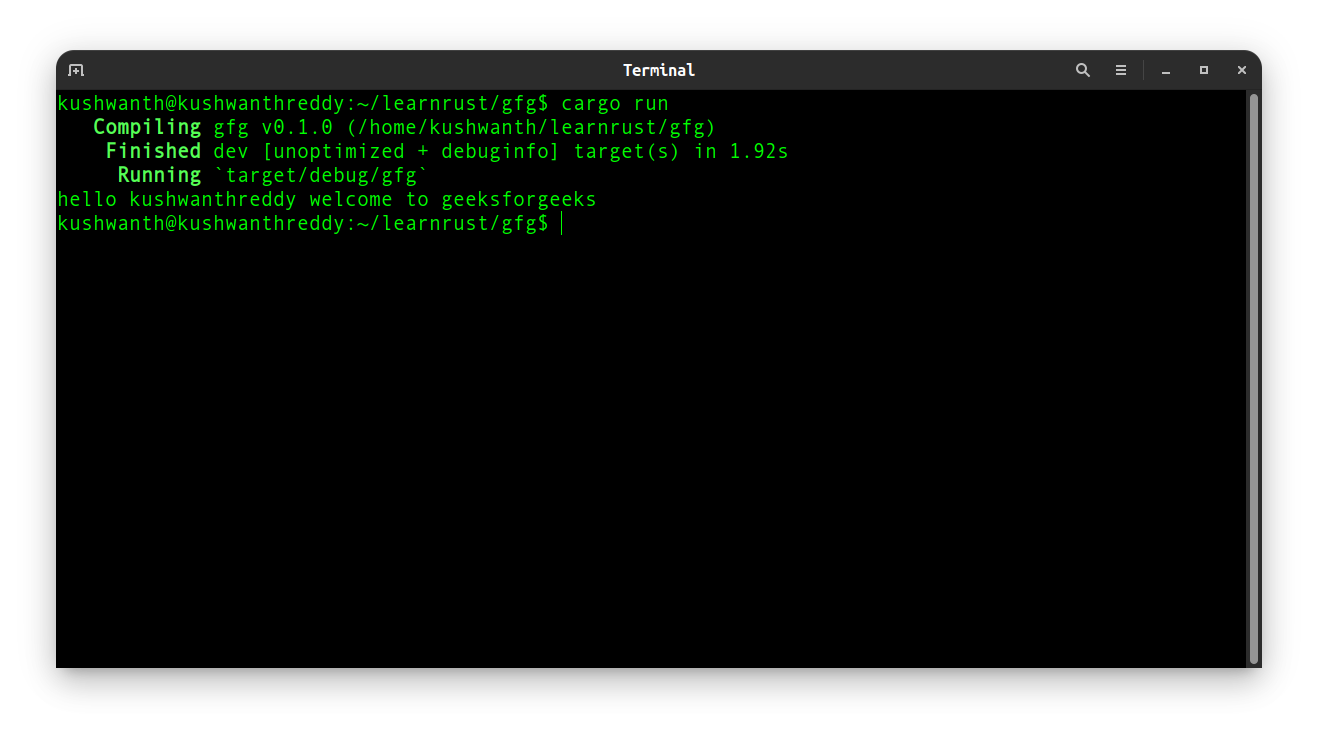
在 Rust 中的函数
函数参数
在上面的例子中,我们使用函数不带任何参数,参数是特殊变量,这些变量的函数签名的一部分参数。让我们看看下面的例子,它增加了 2 个数字。
锈
use std::io;
fn main() {
println!("enter a number:");
let mut stra = String::new();
io::stdin()
.read_line(&mut stra)
.expect("failed to read input.");
println!("enter b number:");
let mut strb = String::new();
io::stdin()
.read_line(&mut strb)
.expect("failed to read input.");
let a: i32 = stra.trim().parse().expect("invalid input");
let b: i32 = strb.trim().parse().expect("invalid input");
sum(a,b);
}
fn sum(x: i32, y: i32) {
println!("sum = {}", x+y);
}
输出:
enter a number: 1
enter b number: 2
sum = 3
如上述程序中的greet程序,该程序中的sum函数也接受2个参数,一般为整数,输出为参数中给出的整数之和。在这里,我们将使用以下方法:
- 程序要求输入一个
- 程序要求输入 b
- Sum 程序以 a, b 作为参数执行
- Sum 程序打印 a, b 的总和
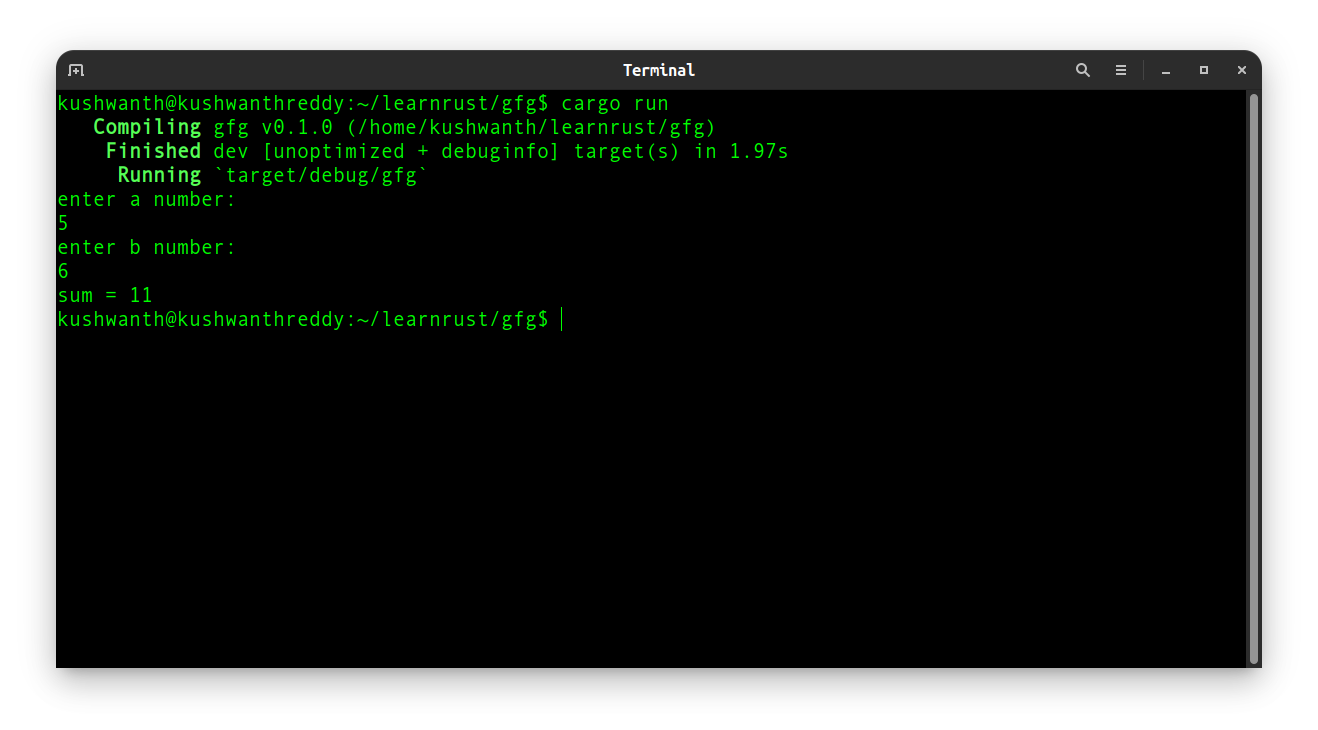
带参数的函数
用 RUST 构建一个简单的计算器
我们将使用函数、函数参数和条件语句构建一个简单的计算器。为此,我们将使用以下方法:
- 该程序要求编号 a
- 程序要求输入数字 b
- 该程序要求选择如何处理数字,无论是它们的总和、差、乘积还是提醒
- 根据用户输入各自的计算是计算器
- sum函数计算总和
- 子函数计算差值
- mul函数计算乘积
- quo函数找出商
- rem函数找出余数
- 对于无效参数程序退出并显示“无效”消息
锈
use std::io;
use std::process::exit;
fn main() {
println!("enter a number:");
let mut stra = String::new();
io::stdin()
.read_line(&mut stra)
.expect("failed to read input.");
println!("enter b number:");
let mut strb = String::new();
io::stdin()
.read_line(&mut strb)
.expect("failed to read input.");
let a: i32 = stra.trim().parse().expect("invalid input");
let b: i32 = strb.trim().parse().expect("invalid input");
println!("choose your calculation: \n1.sum
\n2.difference
\n3.product
\n4.quotient
\n5.remainder\n");
let mut choose = String::new();
io::stdin()
.read_line(&mut choose)
.expect("failed to read input.");
let c: i32 = choose.trim().parse().expect("invalid input");
// Select Operation using conditionals
if c==1{sum(a,b);}
else if c==2{sub(a,b);}
else if c==3{mul(a,b);}
else if c==4{quo(a,b);}
else if c==5{rem(a,b);}
else{println!("Invalid argument");exit(1);}
}
// Sum function
fn sum(x: i32, y: i32) {
println!("sum = {}", x+y);
}
// Difference function
fn sub(x: i32, y: i32) {
println!("difference = {}", x-y);
}
// Product function
fn mul(x: i32, y: i32) {
println!("product = {}", x*y);
}
// Division function
fn quo(x: i32, y: i32) {
println!("quotient = {}", x/y);
}
// Remainder function
fn rem(x: i32, y: i32) {
println!("remainder = {}", x%y);
}
输出:
enter a number:
2
enter b number:
4
choose your calculation:
1.sum
2.difference
3.product
4.quotient
5.remainder
3
product = 8