Java.lang.Boolean 类方法
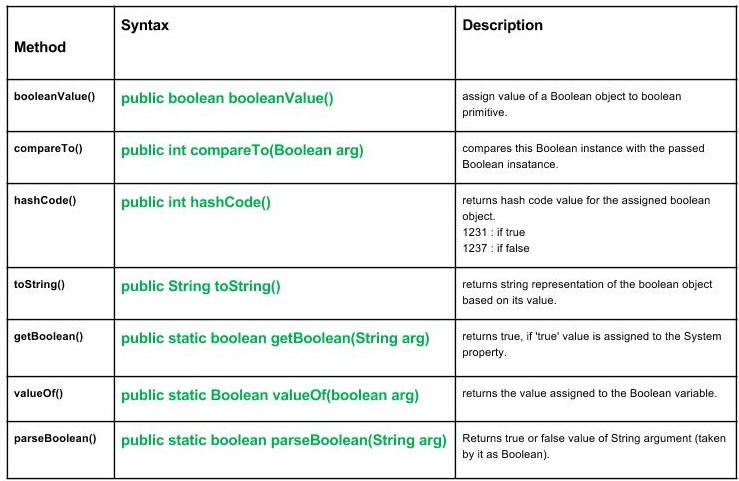
布尔类方法。
关于 :
Java.lang.Boolean 类将原始类型的布尔值包装在一个对象中。
类声明
public final class Boolean
extends Object
implements Serializable, Comparable
构造函数:
Boolean(boolean val) : Assigning Boolean object representing the val argument.
Boolean(String str) : Assigning Boolean object representing the value true or false
according to the string.
方法 :
- booleanValue() : Java.lang.Boolean.booleanValue()用于将布尔对象的值分配给布尔原语。
句法 :
public boolean booleanValue()
Returns :
primitive boolean value of the boolean object.
- compareTo() : Java.lang.Boolean.compareTo(Boolean arg)将此布尔实例与传递的布尔实例进行比较。
句法 :
public int compareTo(Boolean arg)
Parameter :
arg : boolean instance to be compared with this instance.
Returns :
0 : if this instance = argumented instance.
+ve value : if this instance > argumented instance.
-ve value : if this instance < argumented instance.
- hashCode() : Java.lang.Boolean.hashCode()返回分配的布尔对象的哈希码值。
句法 :
public int hashCode()
Returns :
1231 : if the boolean value of object is true.
1237 : if the boolean value of object is false.
- toString() : Java.lang.Boolean.toString()根据其值返回布尔对象的字符串表示形式。
句法 :
public String toString()
Returns :
string value - 'true' if boolean object is true, else false.
执行:
Java
// Java program illustrating Boolean class methods
// booleanValue(), compareTo(), hashCode(), toString()
import java.lang.*;
public class NewClass
{
public static void main(String[] args)
{
// Creating a boolean object and assigning value to it.
Boolean bool1 = new Boolean(true);
Boolean bool2 = new Boolean(false);
System.out.println("Boolean object - bool1 : "+bool1);
System.out.println("Boolean object - bool2 : "+bool2);
// Creating a boolean primitive bool2
boolean bool3, bool4 ;
// Use of booleanValue()
// Assigning object value to primitive variable.
bool3 = bool1.booleanValue();
System.out.println("Primitive value of object i.e. bool3 : "+bool3);
bool4 = bool2.booleanValue();
System.out.println("Primitive value of object i.e. bool4 : "+bool4);
System.out.println("");
// Comparing two boolean instances bool1 and bool2
// Use of compareTo() method
int comp = bool1.compareTo(bool2);
if (comp > 0)
System.out.println("bool1 is greater than bool2 as comp = "+comp);
if (comp == 0)
System.out.println("bool1 is equal to bool2 as comp = "+comp);
if (comp < 0)
System.out.println("bool1 is less than bool2 as comp = "+comp);
System.out.println("");
// HashCode value of the boolean object.
// use of hashCode() method
int h1 = bool1.hashCode();
int h2 = bool2.hashCode();
System.out.println("Hash Code value of bool1 : " + h1);
System.out.println("Hash Code value of bool2 : " + h2);
System.out.println("");
// String representation of the boolean object
// Use of toString() method.
String s1, s2;
s1 = bool1.toString();
s2 = bool2.toString();
System.out.println("String value of bool1 : " + s1);
System.out.println("String value of bool2 : " + s2);
}
}
Java
// Java program illustrating getBoolean() method
import java.lang.*; // Using Boolean and System classes
public class NewClass
{
public static void main(String[] args)
{
/* Use of getBoolean() to check whether
any value is assigned to Property - p1, p2 or not */
boolean b1 = Boolean.getBoolean("p1");
boolean b2 = Boolean.getBoolean("p2");
System.out.println("Bool Value of p1 : "+b1);
System.out.println("Bool Value of p2 : "+b2);
System.out.println("");
System.out.println("Since, no value assigned to p1, p2, Bool value is false");
System.out.println("Assign value to p1,p2 using java.lang.System.setProperty()");
System.out.println("");
System.setProperty("p1","true");
System.setProperty("p2","Cool");
boolean b3 = Boolean.getBoolean("p1");
boolean b4 = Boolean.getBoolean("p2");
System.out.println("Bool Value of p1 : " + b3);
System.out.println("Bool Value of p2 : " + b4);
}
}
Java
// Java program illustrating parseBoolean() and valueOf() method
import java.lang.*;
public class NewClass
{
public static void main(String[] args)
{
boolean b1 = false;
boolean b2 = true;
// Use of valueOf() method
boolean val1 = Boolean.valueOf(b1);
boolean val2 = Boolean.valueOf(b2);
System.out.println("Value of b1 : "+ val1);
System.out.println("Value of b2 : " +val2);
System.out.println("");
// Use of parseBoolean() method
String st1, st2, st3;
st1 = "True";
st2 = "yes";
st3 = "true"; // Case insensitive
boolean p1 = Boolean.parseBoolean(st1);
boolean p2 = Boolean.parseBoolean(st2);
boolean p3 = Boolean.parseBoolean(st3);
System.out.println("Value of String st1 as Boolean : "+p1);
System.out.println("Value of String st2 as Boolean : "+p2);
System.out.println("Value of String st3 as Boolean : "+p3);
}
}
输出:
Boolean object - bool1 : true
Boolean object - bool2 : false
Primitive value of object i.e. bool3 : true
Primitive value of object i.e. bool4 : false
bool1 is greater than bool2 as comp = 1
Hash Code value of bool1 : 1231
Hash Code value of bool2 : 1237
String value of bool1 : true
String value of bool2 : false
- getBoolean() : Java.lang.Boolean.getBoolean(String arg)返回真,如果“真”值被分配给系统属性。
要为属性分配任何值,我们使用 System 类的 setProperty() 方法。
句法 :
public static boolean getBoolean(String arg)
Parameters :
arg - name of the property
Returns :
true : if 'true' value is assigned to the System property.
false : if no such property exists or if exists then no value is assigned to it.
执行:
Java
// Java program illustrating getBoolean() method
import java.lang.*; // Using Boolean and System classes
public class NewClass
{
public static void main(String[] args)
{
/* Use of getBoolean() to check whether
any value is assigned to Property - p1, p2 or not */
boolean b1 = Boolean.getBoolean("p1");
boolean b2 = Boolean.getBoolean("p2");
System.out.println("Bool Value of p1 : "+b1);
System.out.println("Bool Value of p2 : "+b2);
System.out.println("");
System.out.println("Since, no value assigned to p1, p2, Bool value is false");
System.out.println("Assign value to p1,p2 using java.lang.System.setProperty()");
System.out.println("");
System.setProperty("p1","true");
System.setProperty("p2","Cool");
boolean b3 = Boolean.getBoolean("p1");
boolean b4 = Boolean.getBoolean("p2");
System.out.println("Bool Value of p1 : " + b3);
System.out.println("Bool Value of p2 : " + b4);
}
}
输出:
Bool Value of p1 : false
Bool Value of p2 : false
Since, no value assigned to p1, p2, Bool value is false
Assign value to p1,p2 using java.lang.System.setProperty()
Bool Value of p1 : true
Bool Value of p2 : false
- 价值(): Java。 Java.lang.Boolean.valueOf(boolean arg)返回分配给布尔变量的值。
如果分配了真值,则返回真,否则返回假。
要为属性分配任何值,我们使用 System 类的 setProperty() 方法。
句法 :
public static Boolean valueOf(boolean arg)
Parameters :
arg - boolean variable
Returns :
True : if true value is assigned to the boolean variable, else false
- parseBoolean() : Java.lang.Boolean.parseBoolean(String s)返回字符串参数的真或假值(被它作为布尔值)。
这是不区分大小写的方法。
句法 :
public static boolean parseBoolean(String arg)
Parameters :
arg - String argument taken as Boolean
Returns :
Boolean value of a String argument
执行:
Java
// Java program illustrating parseBoolean() and valueOf() method
import java.lang.*;
public class NewClass
{
public static void main(String[] args)
{
boolean b1 = false;
boolean b2 = true;
// Use of valueOf() method
boolean val1 = Boolean.valueOf(b1);
boolean val2 = Boolean.valueOf(b2);
System.out.println("Value of b1 : "+ val1);
System.out.println("Value of b2 : " +val2);
System.out.println("");
// Use of parseBoolean() method
String st1, st2, st3;
st1 = "True";
st2 = "yes";
st3 = "true"; // Case insensitive
boolean p1 = Boolean.parseBoolean(st1);
boolean p2 = Boolean.parseBoolean(st2);
boolean p3 = Boolean.parseBoolean(st3);
System.out.println("Value of String st1 as Boolean : "+p1);
System.out.println("Value of String st2 as Boolean : "+p2);
System.out.println("Value of String st3 as Boolean : "+p3);
}
}
输出:
Value of b1 : false
Value of b2 : true
Value of String st1 as Boolean : true
Value of String st2 as Boolean : false
Value of String st3 as Boolean : true