Python - 将字节写入文件
文件用于永久存储数据。文件处理是对这些文件执行各种操作(读、写、删除、更新等)。在Python中,文件处理过程按以下步骤进行:
- 打开文件
- 执行操作
- 关闭文件
打开文件有四种基本模式——读、写、追加和独占创建。此外, Python允许您指定两种处理文件的模式——二进制和文本。二进制模式用于处理各种非文本数据,如图像文件和可执行文件。
Python将文件以字节的形式存储在磁盘上。因此,当以文本模式打开文件时,这些文件将从字节解码以返回字符串对象。虽然以二进制模式打开的文件将内容作为字节对象(单字节序列)返回,而无需任何解码。让我们看看如何在Python中将字节写入文件。
首先以二进制写入方式打开一个文件,然后以字节的形式指定要写入的内容。接下来,使用 write函数将字节内容写入二进制文件。
Python3
some_bytes = b'\xC3\xA9'
# Open in "wb" mode to
# write a new file, or
# "ab" mode to append
with open("my_file.txt", "wb") as binary_file:
# Write bytes to file
binary_file.write(some_bytes)
Python3
some_bytes = b'\x21'
# Open file in binary write mode
binary_file = open("my_file.txt", "wb")
# Write bytes to file
binary_file.write(some_bytes)
# Close file
binary_file.close()
Python3
# Create bytearray
# (sequence of values in
# the range 0-255 in binary form)
# ASCII for A,B,C,D
byte_arr = [65,66,67,68]
some_bytes = bytearray(byte_arr)
# Bytearray allows modification
# ASCII for exclamation mark
some_bytes.append(33)
# Bytearray can be cast to bytes
immutable_bytes = bytes(some_bytes)
# Write bytes to file
with open("my_file.txt", "wb") as binary_file:
binary_file.write(immutable_bytes)
输出:
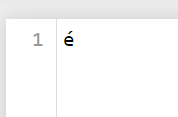
我的文件.txt
在上面的例子中,我们以二进制写入方式打开了一个文件,然后在二进制文件中写入了一些字节的内容作为字节。
或者,可以显式调用 open() 和 close(),如下所示。但是,这种方法需要你自己进行错误处理,即确保文件始终处于关闭状态,即使在写入过程中出现错误。所以,使用“with”语句 在这方面更好,因为它会在块结束时自动关闭文件。
蟒蛇3
some_bytes = b'\x21'
# Open file in binary write mode
binary_file = open("my_file.txt", "wb")
# Write bytes to file
binary_file.write(some_bytes)
# Close file
binary_file.close()
输出:
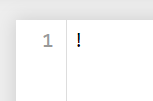
我的文件.txt
此外, some_bytes 可以是可变的 bytearray 形式,也可以是不可变的 bytes 对象,如下所示。
蟒蛇3
# Create bytearray
# (sequence of values in
# the range 0-255 in binary form)
# ASCII for A,B,C,D
byte_arr = [65,66,67,68]
some_bytes = bytearray(byte_arr)
# Bytearray allows modification
# ASCII for exclamation mark
some_bytes.append(33)
# Bytearray can be cast to bytes
immutable_bytes = bytes(some_bytes)
# Write bytes to file
with open("my_file.txt", "wb") as binary_file:
binary_file.write(immutable_bytes)
输出:
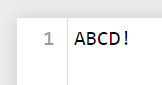
我的文件.txt