📅  最后修改于: 2023-12-03 15:05:36.722000             🧑  作者: Mango
TOO LONG
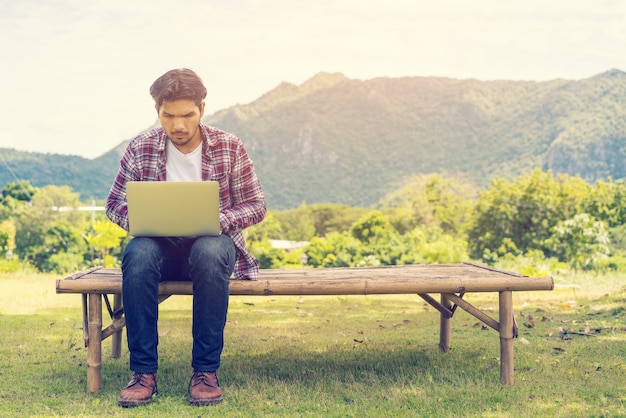
Introduction:
In the world of programming, the phrase "too long" often refers to lines of code, processes, or workflows that have become unnecessarily lengthy or complex. It implies a situation where the codebase has grown unmanageable and difficult to understand. As a programmer, it is essential to recognize and address these issues to ensure maintainable, efficient, and readable code.
Recognizing the Problem:
Identifying when code becomes "too long" can be subjective, but there are some common signs to watch out for. These include:
- Line length: Lines of code that extend far beyond a reasonable width (e.g., more than 80 characters) can hinder readability and understanding.
- Function length: Functions or methods that span numerous lines can become challenging to comprehend, debug, and maintain.
- Nested logic: Excessive nesting of conditional statements or loops can make code convoluted and error-prone.
- Repetitive code: Copy-pasting code instead of using reusable abstractions can lead to long, redundant code segments.
- File size: Very large files can negatively impact collaborative development, version control, and comprehension.
Consequences of "Too Long" Code:
When code becomes "too long," several issues arise, negatively impacting development and maintenance processes. These include:
- Readability: Lengthy, complex code becomes difficult to read, making it hard for developers to understand its flow, functionality, and purpose.
- Maintainability: As codebase size increases, it becomes harder to maintain, debug, and introduce new features or fix bugs.
- Efficiency: Lengthy code can result in slower execution times, decreased performance, and increased resource consumption.
- Bug propagation: Complex, hard-to-understand code may introduce more bugs, as developers find it challenging to comprehend its intricacies fully.
- Collaboration: Longer codebases make collaboration and code reviews more arduous, leading to decreased productivity and increased confusion.
Strategies to Tackle "Too Long" Code:
To overcome the challenges associated with "too long" code, here are some best practices programmers can follow:
1. Modularization:
- Break longer functions into smaller, reusable modules, each performing a specific task.
- Aim for functions that are concise, focused, and do one thing well.
- Utilize function parameters and return values effectively to improve code organization and reusability.
Example:
def calculate_average(numbers):
total = sum(numbers)
length = len(numbers)
return total / length
2. Code Refactoring:
- Analyze long code segments and look for opportunities to simplify, optimize, or eliminate redundancies.
- Identify patterns and extract common code into reusable functions or classes.
- Ensure meaningful and intention-revealing variable and function names to enhance readability.
Example:
// Long and repetitive code
if (conditionA) {
// code block A
}
else {
// code block B
}
// Refactored code using a ternary operator
conditionA ? code_block_A() : code_block_B();
function code_block_A() {
// code block A
}
function code_block_B() {
// code block B
}
3. Abstraction and Encapsulation:
- Identify repetitive tasks and abstract them into reusable functions or classes.
- Encapsulate complex logic within appropriate abstractions to simplify its usage and comprehension.
- Utilize object-oriented or functional programming principles to reduce the complexity of code segments.
Example:
// Lengthy logic within a method
if (conditionA) {
// code block A
// code block B
// code block C
}
else {
// code block B
// code block C
}
// Encapsulated logic within a separate method
if (conditionA) {
code_block_A();
} else {
code_block_B();
}
code_block_B();
code_block_C();
4. Use of Libraries and Frameworks:
- Leverage existing libraries and frameworks to reduce the amount of custom code needed.
- Utilize well-established design patterns and abstractions provided by these tools.
- Avoid reinventing the wheel and take advantage of community-driven solutions.
Example (Python):
import pandas as pd
# Long code segment to read a CSV file
data = []
with open("data.csv", "r") as file:
for line in file:
data.append(line.strip().split(","))
# Shorter code using pandas library
data = pd.read_csv("data.csv")
Conclusion:
Recognizing and addressing "too long" code is crucial for programmers to enhance code quality, maintainability, and productivity. By following best practices like modularization, code refactoring, abstraction, and utilizing libraries, developers can write more readable, maintainable, and efficient code in the long run. Keep striving for simplicity, clarity, and well-organized code to foster better software development practices.