Rust – For 和 Range
假设您有一个项目列表,并且您想要遍历列表中的每个项目。我们可以做的一件事是使用 for 循环。使用 for 循环,我们可以遍历项目列表。在 Rust 中,我们使用关键字 for in 后跟变量名或我们想要迭代的项目范围。
让我们看一个for 循环的例子。
假设我们有一个数字列表,我们想要遍历列表中的每个数字。
Rust
let numbers = [1, 2, 3, 5];
for i in numbers {
println!("{}", i);
}
Rust
// Using for and range in Rust
fn main() { // main function
// define a range of numbers from 20 to 30
for i in 20..31 { // for loop
println!("The number is {}", i); // print the number
} // end of for loop
} // end of main function
Rust
// using range and for loop
fn main() { // main function
let colors = ["red", "green", "blue"]; // define a list of colors
// iterate over the list of colors
for i in 0..colors.len() { // for loop
println!("The color name is {}", colors[i]); // print the color name
} // end of for loop
} // end of main function
Rust
// Using for loop and vectors in Rust
fn main() { // main function
let countries = vec!["India", "China", "USA", "Russia"]; // define a vector of countries
for country in countries.iter() { // for loop
println!("The country name is {}", country); // print the country name
} // end of for loop
} // end of main function
Rust
// using enumerate and for loop in Rust
fn main() { // main function
let programming_languages = ["Rust", "Python", "Java", "C++"]; // define a vector of programming languages
for (index, language) in programming_languages.iter().enumerate() { // for loop
println!("The programming language at index {} is {}", index, language); // print the programming language at index
} // end of for loop
} // end of main function
Rust
// Using for loop with step_by() in Rust
fn main() { // main function
let programming_languages = ["Rust", "Python", "Java", "C++"]; // define a vector of programming languages
for (index, language) in programming_languages.iter().enumerate().step_by(2) { // for loop
println!("The programming language at index {} is {}", index, language); // print the programming language at index
} // end of for loop
} // end of main function
在这里,for 是我们用来迭代项目列表的关键字。变量名是 i。项目列表是数字。这将打印出列表中的每个数字。
输出:
1
2
3
5
对于和范围:
for 循环具有以下结构:
for iterator in range or vector{
// statement
}
在这里,我们使用 for 循环来完成重复性任务。 for 循环总是以关键字 for 开头,我们可以使用 for 循环而不是 while 循环来使我们的代码看起来更干净。迭代器可以是任何变量。 Rust 中的 range 类似于Python语言中的 range函数。使用范围,我们可以定义一个数字范围。例如,我们可以使用以下语法定义从 1 到 10 的数字范围:
0..11
这里,符号a..b用于定义范围。范围包括开始但不包括结束。如果我们想让范围包含结尾,我们可以使用范围语法a..=b。
示例 1:
锈
// Using for and range in Rust
fn main() { // main function
// define a range of numbers from 20 to 30
for i in 20..31 { // for loop
println!("The number is {}", i); // print the number
} // end of for loop
} // end of main function
输出:
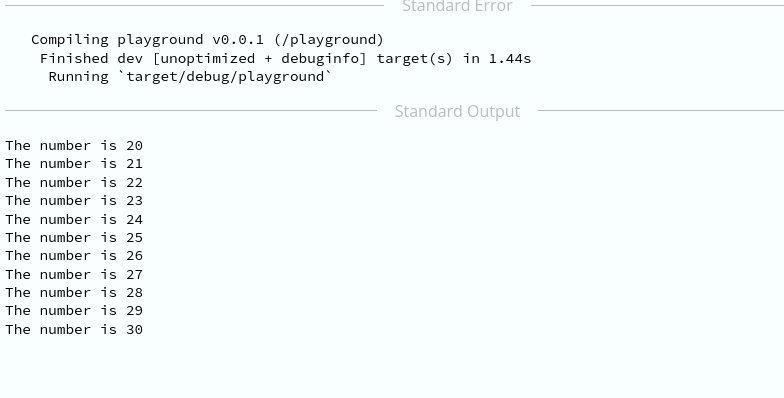
带范围的 for 循环
示例 2:
锈
// using range and for loop
fn main() { // main function
let colors = ["red", "green", "blue"]; // define a list of colors
// iterate over the list of colors
for i in 0..colors.len() { // for loop
println!("The color name is {}", colors[i]); // print the color name
} // end of for loop
} // end of main function
输出:
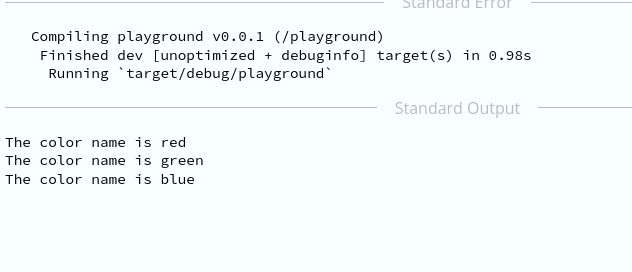
范围和 len()
在这里,我们 0..colors.len() 是颜色的范围。范围包括开始但不包括结束。这里颜色的个数是3。llen ()是String类型的方法,它返回列表中字符串的个数。
示例 3:
锈
// Using for loop and vectors in Rust
fn main() { // main function
let countries = vec!["India", "China", "USA", "Russia"]; // define a vector of countries
for country in countries.iter() { // for loop
println!("The country name is {}", country); // print the country name
} // end of for loop
} // end of main function
输出:
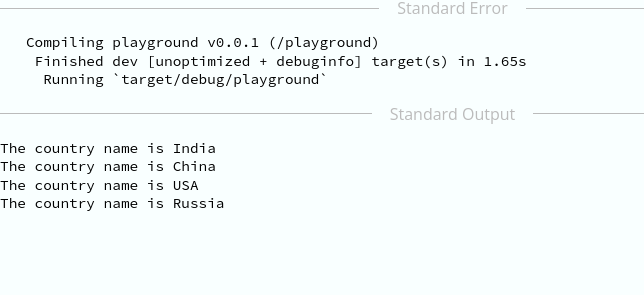
范围和 iter()
这里, iter()是一个向量类型的方法,它返回一个迭代器。 country 是一个变量名,用于遍历向量。通常,iter() 通过每次迭代借用向量的每个元素。
示例 4:
锈
// using enumerate and for loop in Rust
fn main() { // main function
let programming_languages = ["Rust", "Python", "Java", "C++"]; // define a vector of programming languages
for (index, language) in programming_languages.iter().enumerate() { // for loop
println!("The programming language at index {} is {}", index, language); // print the programming language at index
} // end of for loop
} // end of main function
输出:
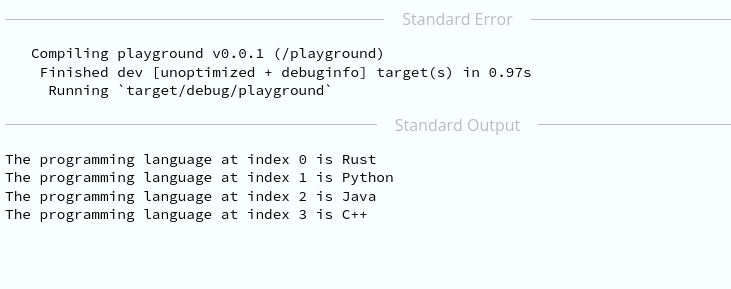
使用枚举()
在这里,我们使用了向量类型的enumerate()方法。 enumerate() 方法允许我们遍历向量并获取元素的索引。索引是从 0 开始的,即枚举的起始索引是 0,而不是 1。
示例 5:
锈
// Using for loop with step_by() in Rust
fn main() { // main function
let programming_languages = ["Rust", "Python", "Java", "C++"]; // define a vector of programming languages
for (index, language) in programming_languages.iter().enumerate().step_by(2) { // for loop
println!("The programming language at index {} is {}", index, language); // print the programming language at index
} // end of for loop
} // end of main function
输出:
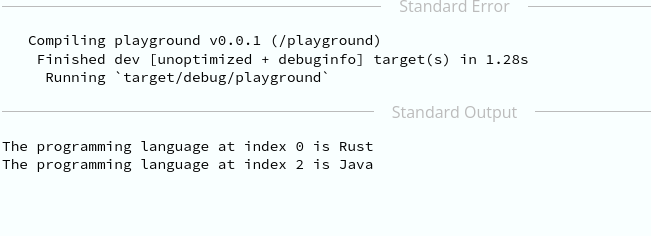
使用 step_by()
在这里, step_by()方法允许我们以步长迭代向量。在这种情况下,步长为 2。