抽象工厂方法Python设计模式
抽象工厂方法是一种创建设计模式,它允许您生成相关对象的系列,而无需指定它们的具体类。使用抽象工厂方法,我们有最简单的方法来产生许多类似类型的对象。
它提供了一种封装一组单独工厂的方法。基本上,这里我们尝试根据逻辑、业务、平台选择等抽象对象的创建。
没有抽象工厂方法我们面临的问题:
想象一下,您想加入 GeeksforGeeks 的精英批次之一。因此,您将去那里询问可用的课程、费用结构、时间安排和其他重要事项。他们只会查看他们的系统,并会为您提供所需的所有信息。看起来很简单?想想开发人员如何使系统如此有条理,以及他们的网站如何如此润滑。
开发人员将为每门课程制作独特的课程,其中将包含其属性,如费用结构、时间安排和其他内容。但是他们将如何调用它们以及它们将如何实例化它们的对象?
这里出现了问题,假设 GeeksforGeeks 最初只有 3-4 门课程,但后来他们增加了 5 门新课程。
因此,我们必须手动实例化它们的对象,根据开发人员的说法,这不是一件好事。
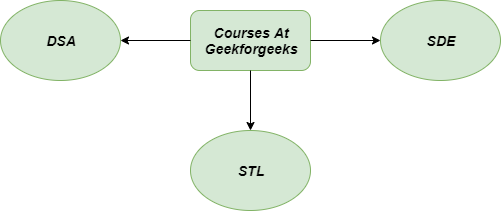
抽象工厂
不使用抽象工厂方法的问题的图解表示
注意:以下代码是在没有使用抽象工厂方法的情况下编写的
Python3
# Python Code for object
# oriented concepts without
# using the Abstract factory
# method in class
class DSA:
""" Class for Data Structure and Algorithms """
def price(self):
return 11000
def __str__(self):
return "DSA"
class STL:
"""Class for Standard Template Library"""
def price(self):
return 8000
def __str__(self):
return "STL"
class SDE:
"""Class for Software Development Engineer"""
def price(self):
return 15000
def __str__(self):
return 'SDE'
# main method
if __name__ == "__main__":
sde = SDE() # object for SDE class
dsa = DSA() # object for DSA class
stl = STL() # object for STL class
print(f'Name of the course is {sde} and its price is {sde.price()}')
print(f'Name of the course is {dsa} and its price is {dsa.price()}')
print(f'Name of the course is {stl} and its price is {stl.price()}')
Python3
# Python Code for object
# oriented concepts using
# the abstract factory
# design pattern
import random
class Course_At_GFG:
""" GeeksforGeeks portal for courses """
def __init__(self, courses_factory = None):
"""course factory is out abstract factory"""
self.course_factory = courses_factory
def show_course(self):
"""creates and shows courses using the abstract factory"""
course = self.course_factory()
print(f'We have a course named {course}')
print(f'its price is {course.Fee()}')
class DSA:
"""Class for Data Structure and Algorithms"""
def Fee(self):
return 11000
def __str__(self):
return "DSA"
class STL:
"""Class for Standard Template Library"""
def Fee(self):
return 8000
def __str__(self):
return "STL"
class SDE:
"""Class for Software Development Engineer"""
def Fee(self):
return 15000
def __str__(self):
return 'SDE'
def random_course():
"""A random class for choosing the course"""
return random.choice([SDE, STL, DSA])()
if __name__ == "__main__":
course = Course_At_GFG(random_course)
for i in range(5):
course.show_course()
使用抽象工厂方法的解决方案:
它的解决方案是将直接的对象构造调用替换为对特殊抽象工厂方法的调用。实际上,对象创建没有区别,但它们是在工厂方法中调用的。
现在我们将创建一个名为Course_At_GFG的唯一类,它将自动处理所有对象实例化。现在,我们不必担心一段时间后我们要添加多少课程。

使用抽象工厂模式的解决方案
Python3
# Python Code for object
# oriented concepts using
# the abstract factory
# design pattern
import random
class Course_At_GFG:
""" GeeksforGeeks portal for courses """
def __init__(self, courses_factory = None):
"""course factory is out abstract factory"""
self.course_factory = courses_factory
def show_course(self):
"""creates and shows courses using the abstract factory"""
course = self.course_factory()
print(f'We have a course named {course}')
print(f'its price is {course.Fee()}')
class DSA:
"""Class for Data Structure and Algorithms"""
def Fee(self):
return 11000
def __str__(self):
return "DSA"
class STL:
"""Class for Standard Template Library"""
def Fee(self):
return 8000
def __str__(self):
return "STL"
class SDE:
"""Class for Software Development Engineer"""
def Fee(self):
return 15000
def __str__(self):
return 'SDE'
def random_course():
"""A random class for choosing the course"""
return random.choice([SDE, STL, DSA])()
if __name__ == "__main__":
course = Course_At_GFG(random_course)
for i in range(5):
course.show_course()
抽象工厂方法的类图:
让我们看一下考虑 GeeksforGeeks 上的 Courses 示例的类图。
GeeksforGeeks 上所有可用课程的费用结构
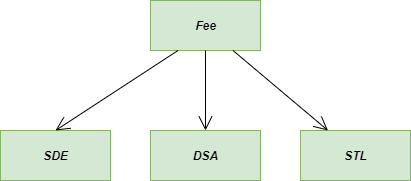
抽象工厂模式的类图
GeeksforGeeks 上所有可用课程的时间安排
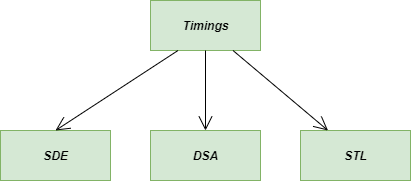
类图2抽象方法模式
使用抽象工厂方法的优点:
当客户端不确切知道要创建什么类型时,此模式特别有用。
- 在不破坏现有客户端代码的情况下,很容易引入产品的新变体。
- 我们从工厂得到的产品肯定是相互兼容的。
使用抽象工厂方法的缺点:
- 由于类的存在,我们的简单代码可能会变得复杂。
- 我们最终会得到大量的小文件,即文件杂乱无章。
适用性:
- 最常见的抽象工厂方法模式出现在汽车制造中使用的钣金冲压设备中。
- 它可用于必须处理不同类别报告的系统,例如与输入、输出和中间事务相关的报告。