字符串format()
方法的语法为:
String.format(String format, Object... args)
这里,
-
format()
是静态方法。我们使用类名称String
调用format()
方法。 -
...
在上面的代码中表示您可以将多个对象传递给format()
。
format()参数
format()
方法采用两个参数。
- 格式 -格式字符串
- args -0个或多个参数
format()返回值
- 返回格式化的字符串
示例1:Java String format()
class Main {
public static void main(String[] args) {
String language = "Java";
int number = 30;
String result;
// format object as a string
result = String.format("Language: %s", language);
System.out.println(result); // Language: Java
// format number as a hexadecimal number
result = String.format("Hexadecimal Number: %x", number); // 1e
System.out.println(result); // Hexadecimal Number: 1e
}
}
在上面的程序中,注意代码
result = String.format("Language: %s", language);
此处, "Language: %s"
是格式字符串 。
格式字符串中的%s
替换为language的内容。 %s
是格式说明符。
同样, %x
替换为String.format("Number: %x", number)
中number的十六进制值。
格式说明符
以下是常用的格式说明符:
Specifier | Description |
---|---|
%b , %B |
"true" or "false" based on the argument |
%s , %S |
a string |
%c , %C |
a Unicode character |
%d |
a decimal integer (used for integers only) |
%o |
an octal integer (used for integers only) |
%x , %X |
a hexadecimal integer (used for integers only) |
%e , %E |
for scientific notation (used for floating-point numbers) |
%f |
for decimal numbers (used for floating-point numbers) |
示例2:数字的字符串格式
class Main {
public static void main(String[] args) {
int n1 = 47;
float n2 = 35.864f;
double n3 = 44534345.76d;
// format as an octal number
System.out.println(String.format("n1 in octal: %o", n1)); // 57
// format as hexadecimal numbers
System.out.println(String.format("n1 in hexadecimal: %x", n1)); // 2f
System.out.println(String.format("n1 in hexadecimal: %X", n1)); // 2F
// format as strings
System.out.println(String.format("n1 as string: %s", n1)); // 47
System.out.println(String.format("n2 as string: %s", n2)); // 35.864
// format in scientific notation
System.out.println(String.format("n3 in scientific notation: %g", n3)); // 4.45343e+07
}
}
输出
n1 in octal: 57
n1 in hexadecimal: 2f
n1 in hexadecimal: 2F
n1 as string: 47
n2 as string: 35.864
n3 in scientific notation: 4.45343e+07
您可以在格式字符串使用多个格式说明符。
示例3:使用多个格式说明符
// using more than one format specifiers
// in a format string
class Main {
public static void main(String[] args) {
int n1 = 47;
String text = "Result";
System.out.println(String.format("%s\nhexadecimal: %x", text, n1));
}
}
输出
Result
hexadecimal: 2f
在这里, %s
替换为text的值。类似地, %o
被替换为n1的十六进制值。
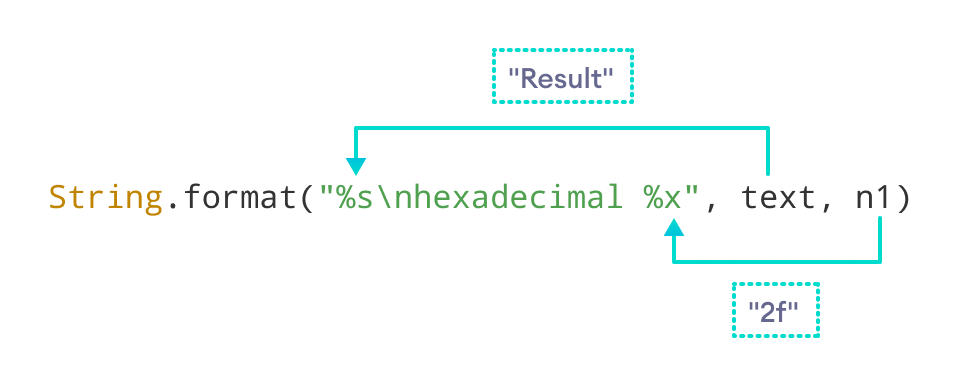
示例4:十进制数的格式
class Main {
public static void main(String[] args) {
float n1 = -452.534f;
double n2 = -345.766d;
// format floating-point as it is
System.out.println(String.format("n1 = %f", n1)); // -452.533997
System.out.println(String.format("n2 = %f", n2)); // -345.766000
// show up to two decimal places
System.out.println(String.format("n1 = %.2f", n1)); // -452.53
System.out.println(String.format("n2 = %.2f", n2)); // -345.77
}
}
输出
n1 = -452.533997
n2 = -345.766000
n1 = -452.53
n2 = -345.77
注意:当我们使用%f
格式化-452.534时 ,我们得到-452.533997 。这不是因为format()
方法。 Java不会返回浮点数的确切表示形式。
使用%.2f
格式说明符时, format()
在小数点后给出两个数字。
示例5:用空格和0填充数字
// using more than one format specifiers
// in a format string
class Main {
public static void main(String[] args) {
int n1 = 46, n2 = -46;
String result;
// padding number with spaces
// the length of the string will be 5
result = String.format("|%5d|", n1); // | 46|
System.out.println(result);
// padding number with numbers 0
// the length of the string will be 5
result = String.format("|%05d|", n1); // |00046|
System.out.println(result);
// using signs before numbers
result = String.format("%+d", n1); // +46
System.out.println(result);
result = String.format("%+d", n2); // -46
System.out.println(result);
// enclose negative number within parenthesis
// and removing the sign
result = String.format("%(d", n2); // (46)
System.out.println(result);
}
}
示例6:在十六进制和八进制之前使用0x和0
// using 0x before hexadecimal
// using 0 before octal
class Main {
public static void main(String[] args) {
int n = 46;
System.out.println(String.format("%#o", n)); // 056
System.out.println(String.format("%#x", n)); // 0x2e
}
}
带有语言环境的Java字符串format()
如果必须使用指定的语言环境,则String format()
方法还具有另一种语法。
String.format(Locale l,
String format,
Object... args)
示例7:在format()中使用GERMAN语言环境
// to use Locale
import java.util.Locale;
class Main {
public static void main(String[] args) {
int number = 8652145;
String result;
// using the current locale
result = String.format("Number: %,d", number);
System.out.println(result);
// using the GERMAN locale as the first argument
result = String.format(Locale.GERMAN, "Number in German: %,d", number);
System.out.println(result);
}
}
输出
Number: 8,652,145
Number in German: 8.652.145
注意:在德国,整数用分隔.
而不是,
。